10 API Performance Optimization Tips
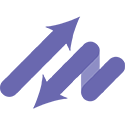
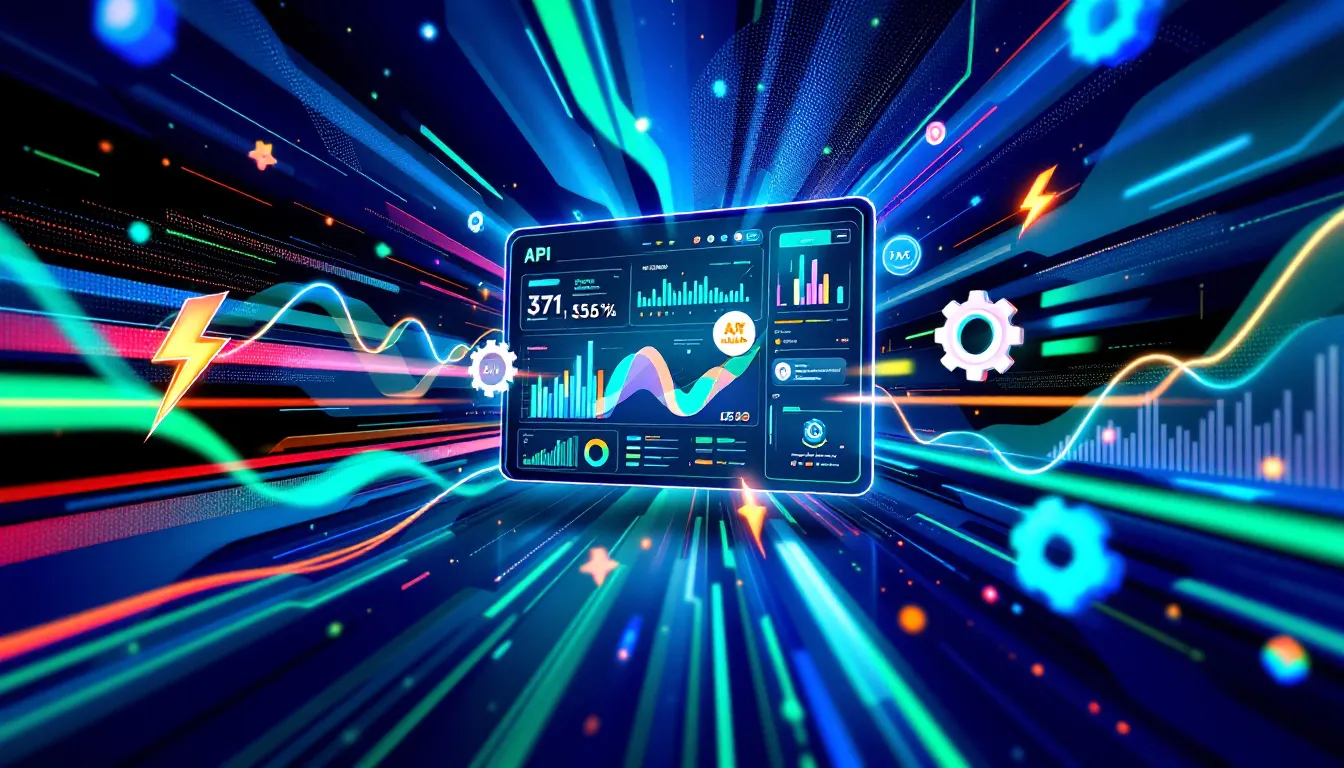
Want to supercharge your API? Here's how to make it faster and more efficient:
- Use caching strategies
- Improve database queries
- Compress data
- Implement pagination and filtering
- Use asynchronous processing
- Set rate limits
- Improve API design
- Adopt HTTP/2
- Track API performance
- Keep connections open
These tactics can dramatically boost your API's speed and reliability. For example, Lob saw a 50% increase in max request throughput just by keeping connections open.
Quick Comparison:
Tip | Main Benefit | Ease of Implementation |
---|---|---|
Caching | Reduces server load | Moderate |
Database optimization | Improves query speed | Challenging |
Data compression | Smaller payloads | Easy |
Pagination | Efficient data handling | Moderate |
Async processing | Better responsiveness | Challenging |
Rate limiting | Prevents abuse | Easy |
API design improvements | Easier data access | Moderate |
HTTP/2 | More efficient connections | Moderate |
Performance tracking | Spots issues early | Easy |
Open connections | Less overhead | Easy |
Ready to dive in? Let's explore each tip in detail.
Related video from YouTube
Use Caching Strategies
Caching is your secret weapon for speeding up APIs. It's like having a cheat sheet for your data. Let's break down three types of caching:
Browser Caching
This is like giving users their own personal data stash. It's fast and cuts down on server chatter.
Here's how to set it up:
res.set('Cache-Control', 'public, max-age=3600');
This tells browsers to hang onto the data for an hour.
Server Caching
Server caching is like the API's memory. It remembers recent requests so it doesn't have to think too hard. Here's a quick example:
const cache = require('memory-cache');
app.get('/api/data', (req, res) => {
const key = req.url;
const cachedData = cache.get(key);
if (cachedData) {
return res.json(cachedData);
}
const data = fetchDataFromDB();
cache.put(key, data, 5 * 60 * 1000);
res.json(data);
});
This keeps data fresh for 5 minutes, saving your database from a workout.
CDN Caching
CDNs are like having data outposts around the world. They bring your API closer to users, no matter where they are.
To use a CDN:
- Pick a provider
- Set up your API
- Tweak your caching rules
You might cache static stuff for a day, but keep API responses fresher.
Caching Type | Good Stuff | Not-So-Good Stuff |
---|---|---|
Browser | User-side speed boost | Less control, might get stale |
Server | You're in charge, database gets a break | Eats server memory |
CDN | Global speed, scales well | More setup, might cost you |
Each type has its place. Mix and match for the best results.
2. Improve Database Queries
Let's make your API faster by tweaking database queries:
Query Smarter, Not Harder
Forget SELECT *
. It's overkill. Pick only what you need:
SELECT id, name, email FROM users WHERE active = TRUE;
This cuts the fat, making your API zippier.
Use EXISTS
instead of IN
for subset checks. It's quicker:
SELECT * FROM orders o
WHERE EXISTS (SELECT 1 FROM customers c
WHERE c.id = o.customer_id AND c.active = TRUE);
Indexing: Your Database's Best Friend
Indexes are like shortcuts. They help find data fast:
CREATE INDEX idx_customer_name ON customers(name);
This speeds up customer name searches. But don't go overboard - too many indexes can slow things down.
"Leave out columns that will never be used by data analysts or business users."
This applies to APIs too. Less data = faster responses.
Connection Pooling: Reuse and Recycle
Think of connection pooling as a relay team. Instead of new runners each time, you reuse the same team:
Without Pooling | With Pooling |
---|---|
New connection per query | Reuse connections |
Slow, resource-heavy | Fast, efficient |
Limited scalability | Handles more traffic |
To set up pooling, focus on:
- Maximum pool size
- Minimum idle connections
- Connection timeout
- Idle connection test period
Tweak these to supercharge your API's database game.
3. Compress Data
Want faster APIs and less bandwidth? Compress your data. Here's how:
GZIP Compression
GZIP is your best friend for squeezing API data. It's quick, effective, and everyone uses it.
What can GZIP do? It can shrink your JSON data by 75% without touching your code. A 181 KB file? GZIP turns it into a 45.9 KB lightweight.
For Spring Boot apps, just add this to your YAML:
server:
compression:
enabled: true
mime-types: text/html,text/plain,text/css,application/javascript,application/json
min-response-size: 1024
Slim Down Responses
Smaller responses = faster APIs. Try these tricks:
- Ditch null values
- Use short field names
- Serialize as arrays
Combine these with GZIP, and your data shrinks to 22-25% of its original size. Not bad, right?
Pick the Right Format
Format matters. Check out GZIP vs Brotli:
Compression | File Size | Reduction |
---|---|---|
None | 173 KB | 0% |
GZIP | 61 KB | 65% |
Brotli | 52 KB | 70% |
Brotli's the new kid on the block. It often beats GZIP, but not everyone supports it yet.
Twitter saw an 80% size drop when they used GZIP on their Streaming API.
That's the power of good compression. Use it wisely!
4. Use Pagination and Filtering
Pagination and filtering are game-changers for API speed and user satisfaction. Here's why:
Why Use Pagination
Loading a million records at once? Your API would crawl. Pagination breaks data into manageable chunks.
Benefits:
- Faster responses
- Less server load
- No more endless loading screens
Effective Filtering
Filtering is like a custom search for your API. Users get only what they need.
It helps by:
- Reducing data processing
- Speeding up queries
- Delivering precise results
How to Implement
Here's how to add pagination and filtering:
1. Pagination Basics
Use query params:
GET /api/users?page=2&pageSize=50
This grabs page 2 with 50 users.
2. Smart Filtering
Let users filter key fields:
GET /api/products?category=electronics&price_min=100&price_max=500
3. Cursor-Based Pagination
For big, dynamic datasets, use cursors:
GET /api/events?since_id=12345&limit=10
This fetches 10 events after ID 12345.
4. Metadata Matters
Include pagination info:
Key | Value |
---|---|
total_items | 1000 |
items_per_page | 50 |
current_page | 2 |
total_pages | 20 |
5. Set Limits
Cap the max page size to prevent overload.
"Implementing API pagination can lead to improved performance, reduced resource usage, enhanced user experience, efficient data transfer, scalability, and better error handling."
Remember: Clear documentation is key. Your users will thank you!
5. Use Asynchronous Processing
Async processing can make your API faster and more efficient. Here's the deal:
Why Async?
Async APIs let your server juggle lots of requests at once. This means:
- Quicker responses
- Server resources used better
- Happier users
How to Do It
1. Go Non-Blocking
Swap out blocking calls for non-blocking ones. Think async database queries instead of synchronous.
2. Webhooks Are Your Friend
Let your API give clients a heads up when data changes. No more constant checking.
3. Queue It Up
Got time-consuming tasks? Toss them in a queue for later. Keeps your API snappy.
Real-World Examples
Company | What They Did | Result |
---|---|---|
Async messaging API | Handles billions of requests like a champ | |
GitHub | Webhooks for code pushes | Kicks off automated builds in CI/CD |
World of Warcraft | Async multiplayer API | Players swap data in real-time |
"Async APIs are great for real-time stuff, but don't rush into it."
sbb-itb-96038d7
6. Set Rate Limits
Think of rate limits as traffic cops for your API. They keep things running smoothly and stop resource hogs.
Why Rate Limiting Matters
Rate limiting is crucial for:
- API stability
- DDoS protection
- Fair access for all users
Without it, a few users could slow everything down.
How to Add Throttling
Here's a quick guide:
1. Pick Your Limit
Choose your request allowance. For example:
Time Frame | Request Limit |
---|---|
Per Second | 5 |
Per Minute | 30 |
Per Hour | 1,000 |
2. Choose Your Method
Options include:
- Fixed window
- Sliding window
- Leaky bucket
3. Set Up Responses
When users hit the limit, send a 429 "Too Many Requests" status code.
Balance Speed and Fair Use
Finding the right balance is key:
- Monitor usage
- Adjust limits as needed
- Offer paid tiers for power users
"API rate limiting is like setting up speed limits on the road – it ensures that everyone gets a fair chance to use the API without causing gridlock."
Real-World Example: Twitter allows 900 requests per 15 minutes on some endpoints. This keeps their API running smoothly even with millions of users.
Rate limits = happy users + healthy API.
7. Improve API Design
Good API design boosts performance. Here's how to make your API faster and more user-friendly:
RESTful Design Tips
-
Clear resource names: Use nouns, not verbs.
/products
instead of/getProducts
. -
Keep it simple: Avoid deep nesting. Stick to 2-3 levels in your URL structure.
-
Use HTTP methods right: Match actions to HTTP verbs:
HTTP Method | Use Case |
---|---|
GET | Fetch data |
POST | Create new data |
PUT | Update existing data |
DELETE | Remove data |
PATCH | Partial updates |
Organize Endpoints
Group related endpoints and use versioning. It makes your API easier to use and maintain.
Example:
/api/v1/users
/api/v1/products
/api/v2/users
/api/v2/products
For large data sets, use pagination to improve response times.
HTTP Methods: Speed and Clarity
- GET: Fast and cacheable. Use for reading data.
- POST: Not cacheable. Use for creating new resources.
- PUT: Replaces entire resources. Use for full updates.
- PATCH: Faster than PUT for small changes. Use for partial updates.
- DELETE: Keep it simple. Use to remove resources.
Remember: The right HTTP method can make your API faster and clearer.
8. Use HTTP/2
HTTP/2 is a big deal for API speed. It's way faster than HTTP/1.1 and fixes problems the old version didn't see coming.
Here's why HTTP/2 rocks:
-
Multiplexing: Sends multiple requests at once over one connection. No more waiting in line.
-
Header Compression: Squeezes headers to cut down on data.
-
Server Push: Servers can send stuff before you ask for it. Faster loading, anyone?
-
Binary Protocol: Uses computer-friendly binary format instead of text.
Want to switch? Here's how:
- Get HTTPS (it's required)
- Update your server
- Use a CDN like Cloudflare
- Tweak your API for HTTP/2
Check out these speed gains:
Feature | HTTP/1.1 | HTTP/2 | What It Means |
---|---|---|---|
Connections | One per request | Many per connection | Less waiting |
Headers | No compression | Compressed | Smaller data packets |
Prioritization | Nope | Yep | Important stuff loads first |
Server Push | Not a thing | It's a thing | Faster resource delivery |
"HTTP/2's speed boost is likely to beat any HTTPS slowdown", says a web speed guru.
Quick tips:
- Don't split domains (it's an old HTTP/1.1 trick)
- Use small, separate files instead of big combined ones
- Keep it under 50 files per URL to avoid slowdowns
HTTP/2 is faster, smarter, and ready to supercharge your API. Time to make the switch!
9. Track API Performance
Keeping your API fast and reliable? It's all about tracking performance. Here's what you need to know:
Key Metrics to Watch
Four numbers tell you how your API's doing:
- Response Time: How fast does it answer?
- Throughput: How many requests per minute?
- Error Rate: What percentage fails?
- CPU and Memory Usage: How much server power does it eat?
Monitoring Tools
Don't count by hand. Use these tools:
Tool | What It's Good For | Starting Cost |
---|---|---|
Better Stack | All-around monitoring | $25/month |
Datadog | Deep synthetic checks | $5 per 10k test runs |
Assertible | Cheap API checks | Free for 10 tests |
API Science | Lots of API tests | $29/month for 100k calls |
Regular Performance Tests
Catch problems early:
- Set up auto-tests to run often
- Use real-world scenarios
- Look for trends over time
"API monitoring isn't 'set and forget'. You need to keep an eye on it", says a Datadog performance pro.
Remember: Track, test, and tweak. That's how you keep your API in top shape.
10. Keep Connections Open
Keeping connections open is a simple trick to speed up your API. Here's the scoop:
Why It Works
Every new connection takes time. DNS lookups, TCP connections, SSL handshakes - they all add up. Open connections skip these steps for follow-up requests.
How to Do It
1. Use HTTP Keep-Alive
For HTTP/1.1, it's automatic. For HTTP/1.0, add this:
Connection: keep-alive
2. Tweak Server Settings
Setting | Purpose | Example |
---|---|---|
MaxKeepAliveRequests | Max requests per connection | 1000 |
KeepAliveTimeout | Idle connection time | 5 seconds |
3. Use Connection Pooling
Python:
import requests
session = requests.Session()
session.headers.update({'Connection': 'keep-alive'})
response = session.get('https://api.example.com')
PHP (Guzzle):
use GuzzleHttp\Client;
$client = new Client(['headers' => ['Connection' => 'keep-alive']]);
$response = $client->get('https://api.example.com');
The Results
Open connections make a BIG difference:
- Lob saw 50% more max request throughput
- Akamai had 22% fewer new TCP connections with HTTP/2
- Datadog found it saved 206ms per call
"Enabling Keepalive saved a whopping 206ms per invocation when compared to the same call without HTTP Keepalive", says a Datadog performance expert.
Conclusion
API performance optimization is an ongoing process. Let's recap the key strategies:
Strategy | Impact |
---|---|
Caching | Less server load, faster responses |
Database optimization | Better query efficiency |
Data compression | Smaller payloads |
Pagination and filtering | Efficient data handling |
Asynchronous processing | Improved responsiveness |
Rate limiting | Prevents abuse |
API design improvements | Easier data access |
HTTP/2 adoption | More efficient connections |
Performance tracking | Spots issues early |
Open connections | Less connection overhead |
Small tweaks can make a big difference. Lob saw a 50% boost in max request throughput by keeping connections open. Datadog shaved off 206ms per call with the same trick.
To stay sharp:
- Keep testing your API
- Use tools like Postman or Swagger
- Set up alerts for key metrics
- Listen to user feedback
Your API users can offer real-world insights. Use their experiences to guide your optimization efforts.
FAQs
How to optimize API performance?
To boost API speed:
1. Cut the fat: Trim unnecessary data from responses.
2. Cache it: Store common data to avoid hitting the database every time.
3. Chunk it up: Use pagination for large datasets.
4. Tune your queries: Simplify and index database queries.
5. Squeeze it: Compress data to reduce transfer sizes.
How do I make API calls efficiently?
For slick API calls:
- Cache smart: Store and reuse data to cut down on requests.
- Batch 'em up: Combine multiple calls when you can.
- Go HTTP/2: It's faster and more efficient.
- Set limits: Use rate limiting to keep things fair and prevent abuse.
How to reduce API response time?
Speed up those responses:
1. Optimize queries: Index well and avoid complex joins.
2. Cache it: Keep hot data in memory.
3. Go async: Handle time-sucks in the background.
4. Use CDNs: Bring content closer to users.
How can you speed up an API call?
Turbocharge your API calls:
- Pool connections: Reuse database connections to cut overhead.
- Handle errors right: Quickly spot and fix issues.
- Tune your server: Optimize those settings for speed.
- Keep an eye out: Regularly check performance to catch bottlenecks.
What is caching API?
API caching stores frequently requested data in fast storage. It's like a cheat sheet for your API, reducing database queries and speeding up responses.
Caching Type | What It Does | Why It's Great |
---|---|---|
Client-side | Stores data on user devices | Fewer network requests |
Server-side | Keeps data in server memory | Less database strain |
CDN caching | Stores content on distributed servers | Faster global access |
Related posts
Ready to get started?