Circuit Breaker Pattern in Service Mesh: Guide
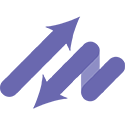
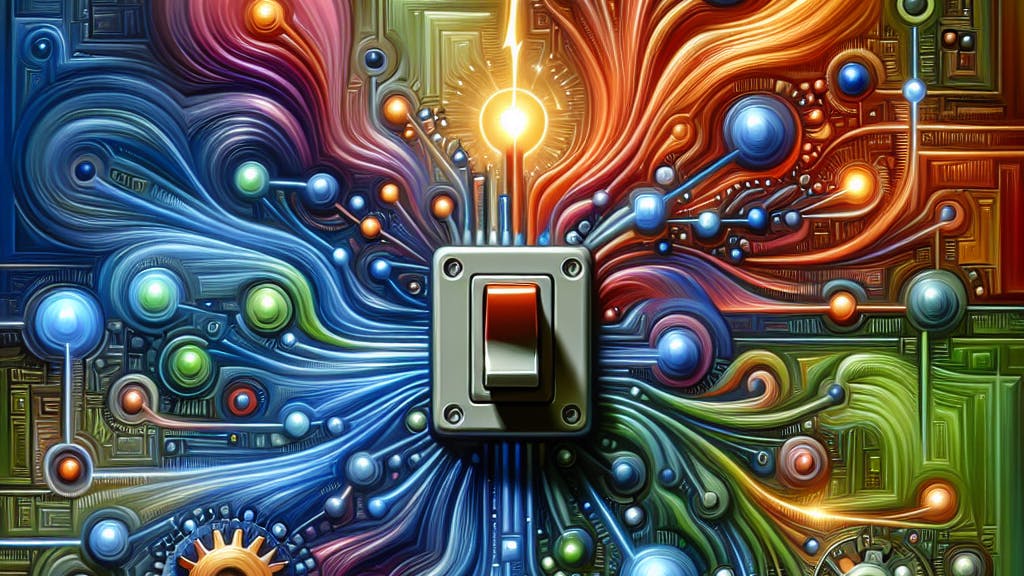
The Circuit Breaker pattern is a crucial safeguard in service mesh architectures that prevents system-wide failures by:
- Monitoring service calls
- Detecting failures or slow responses
- Temporarily halting requests to problematic services
It operates in three states:
- Closed: Normal operation
- Open: Requests blocked for recovery
- Half-Open: Limited requests to test service health
Key benefits:
- Prevents cascading failures
- Allows failing services time to recover
- Maintains system stability under load
To implement circuit breakers effectively:
Parameter | Description | Example Value |
---|---|---|
maxConnections | Max concurrent connections | 100 |
maxPendingRequests | Max queued requests | 1024 |
maxRequests | Max concurrent requests (HTTP/2) | 1000 |
maxRetries | Max number of retries | 3 |
This guide covers setup in Istio, Linkerd, and Consul, along with testing strategies and troubleshooting tips.
Related video from YouTube
What is the Circuit Breaker Pattern?
The Circuit Breaker pattern is a key design approach in microservices architecture that helps prevent system-wide failures. It's like a safety switch for your services, monitoring their health and cutting off connections when things go south.
Key Ideas
The Circuit Breaker operates in three states:
- Closed: Normal operation, requests flow through
- Open: Requests are blocked to allow recovery
- Half-Open: Limited requests to test service health
It's all about failing fast and applying back pressure when needed. This way, you're not wasting resources on requests that are likely to fail.
How Circuit Breakers Work
Let's break it down:
- In the Closed state, it's business as usual. The Circuit Breaker keeps an eye on failures.
- If failures hit a certain threshold, it flips to the Open state. Now, it's returning errors right away instead of trying to execute doomed operations.
- After a timeout, it moves to Half-Open. This is where it lets a few requests through to see if the service is back on its feet.
Why Use Circuit Breakers
Circuit Breakers are your first line of defense against cascading failures in microservices. They:
- Stop the domino effect of one service failure bringing down the whole system
- Give failing services time to recover without getting bombarded by requests
- Help your system stay stable under load
Here's a real-world example:
"At Netflix, we use circuit breakers extensively to handle service disruptions", says a Netflix engineer. "When a service starts failing, our circuit breakers kick in, preventing cascading failures and enabling fallback strategies. This keeps the user experience smooth even when certain features are unavailable."
To implement circuit breaking effectively, you need to set up some key parameters:
Parameter | Description | Example Value |
---|---|---|
tcp.maxConnections | Max HTTP1/TCP connections to a host | 100 |
http.http1MaxPendingRequests | Max pending HTTP requests | 1024 |
http2MaxRequests | Max requests to a backend | 1000 |
By tweaking these settings, you can control how your services interact and respond to potential issues.
What You Need Before Starting
Before diving into circuit breaker implementation in your service mesh, you'll need to set up a few key components:
1. Kubernetes Cluster
A running Kubernetes cluster is essential. This forms the foundation for deploying and managing your microservices.
2. Service Mesh Tool
Choose and install one of these popular service mesh options:
Service Mesh | Version | Key Features |
---|---|---|
Istio | 1.14+ | Built-in circuit breaking, robust traffic management |
Linkerd | 2.11+ | Lightweight, easy to use, supports consecutive failure accrual |
Consul | 1.9+ | Integrates well with HashiCorp stack, flexible circuit breaking options |
3. Sample Application
Deploy a test application to experiment with circuit breaking. For Istio users, the httpbin
sample app works well:
kubectl apply -f samples/httpbin/httpbin.yaml
4. CLI Tools
Install these command-line tools:
kubectl
: For interacting with your Kubernetes clusteristioctl
,linkerd
, orconsul
: CLI for your chosen service meshcurl
orfortio
: For testing and load generation
5. Understanding of Key Concepts
Familiarize yourself with these circuit breaker parameters:
Parameter | Description | Example Value |
---|---|---|
maxConnections | Max HTTP1/TCP connections to a host | 100 |
maxPendingRequests | Max queued requests | 1024 |
maxRequests | Max concurrent requests (HTTP/2) | 1000 |
maxRetries | Max number of retries | 3 |
6. Infrastructure as Code (Optional)
For production setups, consider using Terraform or similar tools. For example, to set up a Consul-based environment:
git clone https://github.com/hashicorp-education/learn-consul-circuit-breaking.git
terraform init
terraform apply
7. Monitoring Tools
Set up monitoring to observe your circuit breakers in action. Options include:
- Prometheus for metrics collection
- Grafana for visualization
- Kiali (for Istio) for service mesh observability
How to Set Up Circuit Breakers in Different Service Meshes
Setting up circuit breakers in service meshes can help improve the reliability of your distributed applications. Let's look at how to implement this pattern in three popular service mesh platforms: Istio, Linkerd, and Consul.
Setting Up in Istio
Istio uses DestinationRules to configure circuit breaking. Here's how to set it up:
1. Create a DestinationRule YAML file:
apiVersion: networking.istio.io/v1alpha3
kind: DestinationRule
metadata:
name: httpbin-circuit-breaker
spec:
host: httpbin
trafficPolicy:
connectionPool:
tcp:
maxConnections: 1
http:
http1MaxPendingRequests: 1
maxRequestsPerConnection: 1
outlierDetection:
consecutive5xxErrors: 1
interval: 1s
baseEjectionTime: 3m
maxEjectionPercent: 100
2. Apply the DestinationRule:
kubectl apply -f httpbin-circuit-breaker.yaml
This configuration limits connections and requests, and ejects failing instances for 3 minutes after a single 5xx error.
Setting Up in Linkerd
Linkerd uses annotations on Kubernetes Services for circuit breaking. Here's how to enable it:
1. Add the following annotation to your Service:
kubectl annotate -n your-namespace svc/your-service balancer.linkerd.io/failure-accrual=consecutive
2. Configure circuit breaking parameters:
kubectl annotate -n your-namespace svc/your-service \
balancer.linkerd.io/failure-accrual-consecutive-max-failures=7 \
balancer.linkerd.io/failure-accrual-consecutive-max-penalty=60s \
balancer.linkerd.io/failure-accrual-consecutive-min-penalty=1s \
balancer.linkerd.io/failure-accrual-consecutive-jitter-ratio=0.5
These settings will mark an endpoint as unavailable after 7 consecutive failures, with a penalty duration between 1 and 60 seconds.
Setting Up in Consul
Consul uses ServiceDefaults to configure circuit breaking. Here's how to set it up:
1. Create a ServiceDefaults configuration file:
Kind = "service-defaults"
Name = "your-service"
Protocol = "http"
CircuitBreaker = {
MaxFailures = 10
Interval = "5s"
BaseEjectionTime = "30s"
MaxEjectionTime = "300s"
MaxConcurrentRequests = 100
}
2. Apply the configuration:
consul config write service-defaults.hcl
This configuration allows 10 failures within a 5-second interval before ejecting the instance for 30 to 300 seconds.
When implementing circuit breakers, remember to test thoroughly and adjust settings based on your specific application needs and traffic patterns.
Tips for Good Circuit Breaker Setup
Setting up circuit breakers in your service mesh can be tricky. Here are some key tips to help you get it right:
Choose the Right Thresholds
Pick thresholds that match your system's needs:
- Failure rate: Start with a 50% failure rate threshold. This means the circuit opens if half of the requests fail.
- Request volume: Set a minimum request volume (e.g., 20 requests) before the circuit can open. This prevents premature tripping.
- Sleep window: Use a short sleep window (e.g., 30 seconds) to allow quick recovery attempts.
Monitor and Adjust
Keep an eye on your circuit breakers:
- Set up alerts for when circuits open
- Track how often circuits trip
- Adjust thresholds based on real-world performance
Use Fallbacks
Always have a plan B:
- Implement fallback mechanisms for when circuits open
- Cache data where possible to serve during outages
- Return default responses when services are unavailable
Test Thoroughly
Don't wait for production issues:
- Simulate failures in your test environment
- Use tools like Fortio to load test your services
- Verify that circuit breakers open and close as expected
Configure Wisely
Here's a table of key settings to consider:
Setting | Description | Recommended Starting Value |
---|---|---|
maxConnections | Max concurrent connections | 100 |
http1MaxPendingRequests | Max queued requests | 1 |
maxRequestsPerConnection | Max requests per connection | 1 |
consecutive5xxErrors | Errors before circuit opens | 5 |
interval | Health check interval | 10s |
baseEjectionTime | Initial ejection duration | 30s |
Real-world Example
"At Netflix, we found that setting our circuit breaker threshold to 50% failure rate over a 10-second interval worked well for most of our services. This allowed us to quickly isolate failing instances without being too sensitive to occasional hiccups", says a Netflix engineer.
sbb-itb-96038d7
How to Test Circuit Breakers
Testing circuit breakers is crucial to ensure they work as expected in your service mesh. Here's how to do it effectively:
Simulate Failures
To test circuit breakers, you need to create failure scenarios:
1. Service Outages
Set up a test environment where you can deliberately shut down services. For example:
- Use Istio's fault injection to simulate a service failure
- Monitor how quickly the circuit breaker detects the outage and opens
2. High Latency
Introduce artificial delays in your services:
- Use tools like Toxiproxy to add network latency
- Check if the circuit breaker trips when response times exceed thresholds
3. Resource Exhaustion
Push your services to their limits:
- Use load testing tools like siege or Apache JMeter
- Gradually increase load until resources are exhausted
- Verify that the circuit breaker prevents system-wide failures
Configure for Testing
Set up your circuit breakers with test-friendly configurations. Here's an example Istio DestinationRule:
apiVersion: networking.istio.io/v1alpha3
kind: DestinationRule
metadata:
name: web-test
spec:
host: web-test
trafficPolicy:
connectionPool:
tcp:
maxConnections: 1
http:
http1MaxPendingRequests: 1
maxRequestsPerConnection: 1
outlierDetection:
consecutive5xxErrors: 1
interval: 1s
baseEjectionTime: 3m
maxEjectionPercent: 100
This configuration:
- Limits connections and requests
- Opens the circuit after just one error
- Checks every second
- Keeps the circuit open for 3 minutes
Use Automated Testing
Set up automated tests to regularly check your circuit breakers:
- Create unit tests for individual components
- Develop integration tests for service interactions
- Use chaos engineering tools to randomly introduce failures
Monitor and Analyze
During testing, keep a close eye on:
- Number of requests
- Success and failure rates
- Response times
- Circuit state changes (open, closed, half-open)
Use tools like Grafana or Prometheus to visualize these metrics.
Real-World Testing Example
Let's look at how Netflix tests their circuit breakers:
"We use a tool called Chaos Monkey to randomly terminate instances in production. This helps us ensure our circuit breakers can handle real outages", says a Netflix engineer. "We found that setting our circuit breaker threshold to 50% failure rate over a 10-second interval worked well for most of our services."
Performance Considerations
Remember that circuit breakers can impact system performance. In Istio:
- Each Envoy proxy uses about 0.35 vCPU and 40 MB memory per 1000 requests/second
- The control plane (Istiod) uses 1 vCPU and 1.5 GB of memory
Factor these resource needs into your testing plans.
Fixing Common Problems
When implementing circuit breakers in service meshes, you might encounter several issues. Here's how to address some common problems:
Misconfigured Circuit Breakers
Often, circuit breakers don't work as expected due to incorrect configuration. To fix this:
1. Check Your DestinationRule
Make sure your DestinationRule
is properly set up. Here's an example of a correct configuration:
apiVersion: networking.istio.io/v1alpha3
kind: DestinationRule
metadata:
name: reviews-destination-rule
spec:
host: reviews
trafficPolicy:
outlierDetection:
baseEjectionTime: 1m
consecutiveErrors: 1
interval: 1s
maxEjectionPercent: 100
2. Verify VirtualService
Ensure your VirtualService
is correctly routing traffic to the intended service:
apiVersion: networking.istio.io/v1alpha3
kind: VirtualService
metadata:
name: reviews-virtual-service
spec:
hosts:
- reviews
http:
- route:
- destination:
host: reviews-service
Continuous Error Logs
If you're seeing non-stop error logs despite setting up circuit breakers, try these steps:
1. Check Service Health: Ensure your service is running correctly.
2. Adjust Thresholds: Your circuit breaker might be too sensitive. Try increasing the consecutiveErrors
value.
3. Monitor Closely: Use tools like Grafana or Prometheus to track circuit breaker states and service health.
"No Such Hosts" Errors
This error often occurs when Istio can't find your service. To resolve:
1. Check if Istiod pods are running:
kubectl -n istio-system get pod -lapp=istiod
2. Verify endpoints are ready:
kubectl -n istio-system get endpoints istiod
Envoy Request Rejections
If Envoy is rejecting requests, inspect the Envoy access logs:
kubectl logs PODNAME -c istio-proxy -n NAMESPACE
Look for error codes and adjust your circuit breaker settings accordingly.
CrashLoopBackOff Errors
These errors can occur due to misconfigurations or resource issues. To troubleshoot:
1. Check pod logs:
kubectl logs PODNAME -n NAMESPACE
2. Verify resource limits and requests in your deployment YAML.
3. Ensure all required environment variables are set.
Protocol Mismatch
If you're sending HTTPS requests to an HTTP port, change the port protocol to HTTPS in your configuration.
Half-Closed Connections
These can lead to 502 errors. To fix:
1. Update your app to close connections promptly.
2. Increase the connection tracker's timeout using the --close-wait-timeout
flag with linkerd inject
.
Linkerd-Specific Issues
For Linkerd users:
1. Always start troubleshooting with linkerd check
.
2. For HTTP 502 errors, check for connection issues between proxies.
3. For HTTP 503 and 504 errors, look for overloaded workloads.
Advanced Circuit Breaker Techniques
Circuit breakers are powerful tools for managing failures in service mesh architectures. Let's explore some advanced techniques to enhance their effectiveness.
Adding Retry Options
Combining retries with circuit breakers can help handle transient failures more effectively. Here's how to implement this strategy:
1. Configure retry attempts: Set a maximum number of retry attempts before the circuit breaker opens. For example, in a Spring application, you can configure:
retry:
maxAttempts: 4
waitDuration: 1000
enableExponentialBackoff: true
2. Use exponential backoff: This increases the delay between retry attempts, reducing load on the failing service.
3. Set appropriate thresholds: Ensure your circuit breaker threshold is higher than your retry attempts to allow for automatic retries before opening the circuit.
Using Fallback Options
Fallbacks provide alternatives when a service is unavailable. Here are some approaches:
1. Custom fallback: Use locally available data or a simplified version of the service to generate a response.
2. Fail silent: Return a null value for optional data.
3. Fail fast: Return a 5xx error for required data to maintain API server health.
Example fallback in a payment service:
@CircuitBreaker(name = "paymentService", fallbackMethod = "fallbackPayment")
public boolean processPayment(Order order) {
// Normal payment processing logic
}
public boolean fallbackPayment(Order order, Exception e) {
// Fallback logic: Assume transaction is not fraudulent
return true;
}
Warning: Be cautious when implementing fallbacks. In the payment example, assuming all transactions are not fraudulent could lead to security risks.
Monitoring Circuit Breakers
Effective monitoring is crucial for optimizing circuit breaker performance. Here's what to track:
Metric | Description | Importance |
---|---|---|
Request count | Number of requests processed | Helps identify traffic patterns |
Error rate | Percentage of failed requests | Indicates service health |
Response time | Time taken to process requests | Helps set appropriate timeouts |
Circuit state | Current state (open, closed, half-open) | Shows circuit breaker behavior |
To implement monitoring:
1. Use built-in tools: Many service meshes offer integrated monitoring solutions.
2. Set up alerts: Configure notifications for when circuits open or close frequently.
3. Visualize data: Use dashboards to track circuit breaker metrics over time.
Wrap-Up
The Circuit Breaker pattern is a key component in building strong, fault-tolerant service mesh architectures. By implementing this pattern, you can protect your system from cascading failures and improve overall stability.
Here's why circuit breakers are so important:
- Failure isolation: They stop issues in one service from affecting others
- Recovery time: Struggling services get a chance to bounce back
- User experience: Customers see fewer errors and faster responses
To get the most out of circuit breakers in your service mesh:
- Start small: Begin with non-critical services to gain experience
- Monitor closely: Keep an eye on circuit breaker metrics to fine-tune settings
- Test thoroughly: Simulate failures to ensure your circuit breakers work as expected
Remember, circuit breakers aren't just for large-scale systems. Even smaller applications can benefit from this pattern. As your service mesh grows, you'll find circuit breakers becoming more and more useful.
"The Circuit Breaker pattern significantly enhances the system's ability to handle failures gracefully, ensuring that sudden service disruptions do not escalate into major outages."
By using circuit breakers, you're taking a proactive approach to system reliability. This can lead to:
Benefit | Impact |
---|---|
Reduced downtime | Fewer system-wide outages |
Improved performance | Faster response times during partial failures |
Better resource management | Less strain on struggling services |
As you continue to work with service meshes, keep the Circuit Breaker pattern in mind. It's a powerful tool that can help you build more robust, reliable systems that can handle the challenges of modern distributed architectures.
Comparison of Circuit Breakers in Service Meshes
Let's compare circuit breaker features across popular service mesh solutions:
Service Mesh | Circuit Breaking | Setup Complexity | Default Settings | Key Features |
---|---|---|---|---|
Istio | Yes | High | Configurable | - Black-box implementation - Uses Envoy sidecar - Supports max connections and pending requests |
Linkerd | Yes | Low | On by default | - Consecutive failure accrual - Automatic load balancing - Simple annotation-based setup |
Consul | Yes | Medium | Configurable | - Uses Envoy proxy - Supports bulkhead pattern and outlier detection - Integrates with other HashiCorp tools |
AWS App Mesh | Yes | Medium | Integrated with AWS services | - Seamless AWS integration - Envoy-based proxy |
Traefik Mesh | Yes | Low | Basic configuration | - Lightweight - Easy to set up |
Istio offers the most advanced circuit breaking capabilities but comes with a steeper learning curve. For example, you can set up circuit breaking in Istio using a DestinationRule
:
apiVersion: networking.istio.io/v1alpha3
kind: DestinationRule
metadata:
name: redis-cb
namespace: hipster-app
spec:
host: redis-cart
trafficPolicy:
connectionPool:
tcp:
maxConnections: 1
http:
http1MaxPendingRequests: 1
maxRequestsPerConnection: 1
outlierDetection:
consecutiveErrors: 1
interval: 1s
baseEjectionTime: 3m
maxEjectionPercent: 100
This configuration limits connections and pending requests, ejecting failing instances for 3 minutes.
Linkerd, on the other hand, focuses on ease of use. You can enable circuit breaking with a simple annotation:
kubectl annotate -n circuit-breaking-demo svc/bb balancer.linkerd.io/failure-accrual=consecutive
Linkerd's approach led to clear improvements in a test scenario. Before circuit breaking, a "bad" pod had a 6.43% success rate with 4.7 RPS. After enabling circuit breaking, its success rate jumped to 94.74%, albeit with reduced traffic (0.3 RPS).
Consul takes a middle ground, offering configurable circuit breaking through its ServiceDefaults
:
Kind = "service-defaults"
Name = "web"
Protocol = "http"
MeshGateway = {
Mode = "local"
}
Expose = {
Checks = true
Paths = [
{
Path = "/health"
LocalPathPort = 8080
ListenerPort = 21500
}
]
}
MaxConnections = 100
MaxPendingRequests = 100
MaxConcurrentRequests = 100
This setup allows fine-tuning of connection limits and failure detection parameters.
When choosing a service mesh for circuit breaking, consider your team's expertise and specific needs. Istio offers power but requires more management, while Linkerd prioritizes simplicity. Consul provides a balance, especially if you're already using other HashiCorp products.
FAQs
When to use a Circuit Breaker pattern?
The Circuit Breaker pattern is best used in these scenarios:
-
High failure likelihood: When a service call is likely to fail, implementing a circuit breaker can prevent cascading failures.
-
Latency issues: If a service experiences high latency, such as slow database connections, causing timeouts.
-
Microservice instability: When dealing with microservices that crash or respond slowly.
-
Long-lasting errors: For handling persistent faults in distributed systems where simple retry mechanisms aren't enough.
Here's a quick reference table for when to consider using the Circuit Breaker pattern:
Scenario | Use Circuit Breaker? |
---|---|
Frequent service failures | Yes |
High latency in service calls | Yes |
Unstable microservices | Yes |
Persistent distributed system faults | Yes |
Simple, quick-to-resolve errors | No |
The Circuit Breaker pattern acts like a proxy between services, monitoring conditions and rerouting traffic when issues arise. It's a pessimistic approach that brings stability to applications by preventing repeated calls to failing services.
For example, if a database service is experiencing slow connections, the circuit breaker can "trip" after a set number of slow responses. This action prevents further calls to the slow service for a predetermined time, allowing it to recover without being overwhelmed by requests.
Related posts
Ready to get started?