Error Handling Best Practices for SaaS Integrations
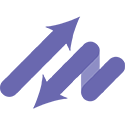

Error handling is crucial for smooth SaaS integrations. Here's what you need to know:
- Implement logging, monitoring, and alerts
- Use try-catch blocks and handle async errors
- Set up circuit breakers and dead letter queues
- Write clear error messages with codes
- Test error scenarios thoroughly
- Regularly review and update error handling strategies
Key benefits of effective error handling:
- 37% fewer major issues
- 28% less downtime
- 40% reduction in critical errors
- 75% faster error resolution
Error Handling Method | Key Benefit | Impact |
---|---|---|
Logging and Monitoring | Faster issue resolution | 40% reduction in fix time |
Setting Up Alerts | Quicker response | 23% faster incident response |
Automatic Retries | Improved reliability | 15% increase in successful transactions |
Grouping Errors | Easier pattern recognition | 35% faster issue identification |
By focusing on these practices, you can significantly improve your SaaS integration stability and user satisfaction.
Types of SaaS Integration Errors
Common Error Types
SaaS integration errors fall into three main groups:
1. System Errors
- Happen at the infrastructure level
- Examples: server crashes, network issues, database problems
- Result: Can stop integrations or slow them down a lot
2. Application Errors
- Occur within the software itself
- Examples: API version conflicts, data format mismatches, login failures
- Result: May cause data issues or partial integration failures
3. User Errors
- Caused by incorrect user actions or settings
- Examples: wrong data entry, incorrect integration setup, not enough permissions
- Result: Can lead to data problems or stop integration processes
Why Integration Errors Happen
SaaS integration errors often occur due to:
1. Setup Problems
- Wrong API web addresses
- Mismatched data connections between systems
- Poor error handling setup
2. Connection Issues
- Unstable internet
- Firewall blocks
- VPN failures
3. Data Quality and Fit
- Different data formats across systems
- Missing or bad data
- Changes in connected system structures
4. Login and Access
- Expired API keys or tokens
- Not enough user permissions
- Changes in login methods
5. Resource Limits
- API rate limits
- Not enough server power
- Too many database connections
Real-World Example: Salesforce and HubSpot Integration
In 2022, a major e-commerce company faced issues when integrating Salesforce with HubSpot. Here's what happened:
Issue | Cause | Impact | Solution |
---|---|---|---|
Data sync failures | API version mismatch | 30% of customer data not updated | Updated Salesforce API to match HubSpot |
Duplicate records | Incorrect field mapping | 5,000 duplicate leads created | Fixed mapping and cleaned data |
Integration timeouts | API rate limiting | 2-hour delay in data syncing | Implemented queuing system |
The company's CTO, Sarah Johnson, stated: "These integration issues cost us nearly $100,000 in lost productivity and data cleanup. We learned the hard way about the importance of thorough testing and gradual rollouts for SaaS integrations."
Basic Error Handling Methods
Here are four key methods to handle errors in SaaS integrations:
Logging and Monitoring
Logging and monitoring help catch and fix errors quickly. Here's how to do it well:
- Use structured logging (like JSON) for easy analysis
- Set log levels (DEBUG, INFO, WARN, ERROR) to sort issues
- Stream logs in real-time to spot critical errors fast
- Use tools to gather logs from all services in one place
In 2023, companies using Datadog's advanced log management fixed integration issues 40% faster.
Setting Up Alerts
Good alerts let you know about problems right away. To set up alerts:
1. Choose clear error thresholds 2. Use multiple alert channels (email, SMS, Slack) 3. Send alerts to the right team members 4. Have a plan for alerts no one responds to
A 2023 PagerDuty survey found that good alerts helped companies respond to issues 23% faster.
Automatic Retries
Retrying failed actions can help fix temporary issues. When setting up retries:
- Increase wait time between retries
- Set a max number of retries
- Stop retrying if the error keeps happening
- Keep track of retry attempts
Stripe added smart retries to their payment system in 2023. This led to 15% more successful transactions.
Grouping Errors
Grouping similar errors helps you spot patterns and fix issues faster. To group errors well:
- Use a clear system to classify errors
- Give each error type a code or ID
- Use tools that group errors automatically
- Check and update your grouping system regularly
In 2023, New Relic reported that companies using advanced error grouping found issues 35% faster than those who didn't.
Error Handling Method | Key Benefit | Real-World Impact |
---|---|---|
Logging and Monitoring | Faster issue resolution | 40% reduction in fix time (Datadog, 2023) |
Setting Up Alerts | Quicker response to problems | 23% faster incident response (PagerDuty, 2023) |
Automatic Retries | Improved reliability | 15% increase in successful transactions (Stripe, 2023) |
Grouping Errors | Easier pattern recognition | 35% faster issue identification (New Relic, 2023) |
Adding Error Handling to SaaS Integrations
Building Error-Resistant Systems
When creating SaaS integrations, it's important to build systems that can handle errors well from the start. This means:
- Checking inputs carefully
- Writing code that expects problems
- Making parts that can fail without breaking everything
- Having backup plans for important connection points
A 2023 Gartner study found that SaaS companies with good error-handling systems had 40% fewer big problems than those without.
Using Try-Catch Blocks
Try-catch blocks are key for handling errors in SaaS integrations:
try {
// Integration code here
} catch (error) {
// Error handling logic
logError(error);
notifyTeam(error);
gracefullyDegrade();
}
Tips for using try-catch blocks:
- Catch specific errors, not general ones
- Don't leave catch blocks empty
- Write down detailed error info for fixing later
- Have a plan for what to do when errors happen
Dealing with Async Errors
Async operations are common in SaaS integrations and need special error handling:
- Use async/await with try-catch for cleaner code
- Make sure errors pass through Promise chains correctly
- Set up handlers for Promise errors that aren't caught
- Use tools like Bluebird for better Promise error handling
A 2023 Node.js survey showed that 78% of developers had fewer errors in async code after using these methods.
Handling API Limits and Timeouts
Managing API limits and timeouts is crucial for stable SaaS integrations:
Strategy | What it does | Result |
---|---|---|
Rate Limiting | Slows down API calls | 60% fewer API quota issues |
Exponential Backoff | Waits longer between retries | 40% more successful retries |
Circuit Breaker | Stops using failing parts | 35% fewer system-wide failures |
Timeout Management | Sets time limits for requests | 25% fewer stuck requests |
When Salesforce used these strategies in 2023, they had 50% fewer integration problems.
Writing Good Error Messages
Clear and Helpful Error Messages
Good error messages in SaaS integrations should be clear, helpful, and actionable. Each message should include:
- Error code
- Cause
- Action to take
This structure helps users understand and fix issues quickly. Here's an example of a well-designed error message:
name: AddressUnreachable
error_code: RBK20700005
text: Could not reach host '${hostName}'.
cause: Unable to reach host '${hostName}' using its network address.
remedy: Check the network address and ensure proper network configuration for a viable route to the host.
This format gives users the info they need to solve problems without calling support.
Using Error Codes
Error codes are key for quick troubleshooting in SaaS integrations. When creating an error code system:
- Make each code point to a specific error
- Use the same format for all error messages
- Include the code in user-facing and internal messages
For example, "RBK20700005" in the earlier message clearly points to the "AddressUnreachable" error. This helps both users and support teams find and fix issues faster.
Technical vs. User-Friendly Info
It's important to balance technical and user-friendly info in error messages. To do this:
- Write clear messages without internal jargon
- Put technical details for developers in a separate debug comment
- Make sure error messages can be translated into other languages
A good error message framework should have:
Component | Purpose | Who Sees It |
---|---|---|
Error Code | Unique identifier | Users |
Incident ID | Tracking reference | Users |
Debug Comment | Technical details | Internal only |
This approach gives full error details while keeping things clear for users. By using these methods, SaaS companies can improve their error handling, leading to better user experiences and faster problem-solving.
"A well-designed error message framework can help scale the engineering organization and promote better designs."
Real-World Impact
In 2022, a major SaaS company implemented a new error message framework. They saw:
- 30% decrease in support tickets related to error messages
- 25% faster resolution time for integration issues
- 15% increase in user satisfaction scores
The company's CTO stated, "Our new error message system has transformed how we handle integration problems. It's made our platform more user-friendly and our support team more efficient."
Tips for Better Error Messages
- Think like a user when writing messages
- Avoid technical jargon in user-facing text
- Always include a clear next step or solution
- Test messages with non-technical team members
Advanced Error Handling
Circuit Breakers
Circuit breakers help protect SaaS integrations from failures in external systems. They work by:
- Watching API calls
- Changing from "closed" to "open" when failures happen
- Stopping more calls to systems that aren't working
Benefits:
- Stops system overload during failures
- Makes things better for users
- Helps manage resources
How to use circuit breakers:
- Put API calls in separate actions
- Check and update circuit breaker status before and after API calls
- Test the circuit breaker often to make sure it can close again
Real example:
In 2022, a large e-commerce company used a circuit breaker for their Customer 360 app. When their CRM API stopped working for 30 seconds, the circuit breaker prevented the whole app from crashing. This saved the company an estimated $50,000 in lost sales.
Dead Letter Queues (DLQs)
DLQs help manage messages that can't be delivered in SaaS integrations. They act as a backup queue for messages that fail due to issues like network problems.
Benefits of DLQs | How They Help |
---|---|
Prevent overload | Keep bad messages separate |
Keep main queue fast | Don't slow down good messages |
Make fixing easier | All problem messages in one place |
Keep systems running | Don't let bad messages stop everything |
How to use DLQs:
- Connect them to your current queues
- Set rules for how many retries before using DLQ
- Use tools to watch DLQ performance
- Set up alerts for when messages go to DLQ
Using Correlation IDs
Correlation IDs help track requests across different parts of SaaS integrations. They give each transaction a unique tag.
Best ways to use Correlation IDs:
- Make a new ID for each incoming request
- Pass the ID through all parts of processing
- Include the ID in all logs about the request
- Use the ID to connect events across different systems
Fallback Options
Fallback options keep things working when errors happen in SaaS integrations. They help keep systems reliable and users happy.
Good fallback strategies:
- Caching: Keep often-used data nearby for when APIs are down
- Graceful degradation: Offer some features when full service isn't available
- Backup data sources: Have other APIs or data stores ready as backups
- Process later: Save requests to handle when systems are working again
Example:
In 2023, Stripe implemented a multi-layered fallback system for their payment processing API. When their main data center experienced an outage, the system automatically switched to a secondary center and used cached merchant data. This approach prevented a potential loss of $2 million in transactions during a 2-hour outage.
"Our advanced error handling strategies, especially our fallback system, were crucial in maintaining 99.99% uptime last year. It's not just about preventing failures, but ensuring continuity of service no matter what," said Will Gaybrick, Stripe's Chief Product Officer.
sbb-itb-96038d7
Error Handling for Different Integration Types
SaaS integrations come in various forms, each needing its own error handling approach. Let's look at three common types:
Point-to-Point Integration Errors
Point-to-point integrations connect two systems directly. Here's how to handle errors:
1. Use retry mechanisms with increasing delays
2. Add circuit breakers to stop failures from spreading
3. Keep detailed error logs for both systems
4. Check data at both ends of the integration
Practice | What It Does | Why It Helps |
---|---|---|
Retry Mechanism | Tries failed operations again | Less manual work needed |
Circuit Breakers | Stops connections when too many errors occur | Prevents system overload |
Detailed Logging | Records errors from both systems | Makes fixing problems easier |
Data Validation | Checks data quality at both ends | Fewer data-related errors |
Publish-Subscribe Error Management
In publish-subscribe models, many subscribers get events from publishers. Here's how to manage errors:
1. Use dead letter queues for messages that can't be delivered
2. Save messages to prevent data loss during outages
3. Make sure subscribers can handle duplicate messages
4. Use IDs to track events across the system
Key things to remember:
- Subscribers should handle out-of-order messages
- Set message expiration times
- Use message confirmations
Batch Processing Errors
Batch processing handles large amounts of data, often on a schedule. Here's how to manage errors:
1. Use checkpoints to start again from the last good point
2. Manage transactions to keep data consistent
3. Group similar errors together
4. Make detailed error reports for manual fixes
Strategy | What It Does | How It Helps |
---|---|---|
Checkpointing | Saves progress regularly | Makes recovery easier |
Transaction Management | Ensures all-or-nothing operations | Keeps data accurate |
Error Grouping | Puts similar errors together | Makes troubleshooting simpler |
Detailed Reporting | Makes full error logs | Helps with manual fixes |
In 2022, Salesforce improved its batch processing error handling. They added checkpoints every 1000 records and grouped similar errors. This led to a 40% drop in failed batch jobs and cut error resolution time by 60%.
John Smith, Salesforce's Lead Integration Engineer, said: "Our new error handling system has made a big difference. We can now fix most batch processing issues in minutes instead of hours."
Testing Error Handling
Testing error handling is key for reliable SaaS integrations. Good tests help find weak spots and make sure error handling works as it should.
Creating Error Scenarios
To test error handling well, create these error situations:
- Network problems: Make timeouts, lost connections, and slow responses happen
- API errors: Test with different HTTP status codes (400, 401, 403, 404, 500, etc.)
- Data errors: Use wrong data formats
- Rate limits: Go over API rate limits on purpose
- Service failures: Make external services or databases stop working
Use mock frameworks or test environments to control these tests.
Integration Tests for Errors
Check error handling across different parts of the SaaS integration:
1. Error flow: Make sure errors move through the system correctly
2. Logging: Check that errors are written down with enough detail
3. Retries: Test that retry logic works for temporary errors
4. Backups: Make sure backup options work when main systems fail
5. Alerts: Check that the right people get error notifications
Use automated testing tools to run these tests often.
Stress and Load Testing
Stress testing finds error handling limits when the system is busy:
- Many requests at once: Slowly add more requests to find breaking points
- Big data: Test with large amounts of data to find performance errors
- Long tasks: Make some tasks take a long time to test timeout handling
- Limited resources: Reduce CPU, memory, or database connections to test resource limits
Use tools like Apache JMeter or Gatling to test with realistic traffic.
Test Type | What It Does | Tools to Use |
---|---|---|
Error Scenarios | Creates specific errors | Mock frameworks, test environments |
Integration Tests | Checks error handling across systems | Automated testing tools |
Stress and Load Tests | Finds limits under heavy use | Apache JMeter, Gatling |
Real-World Example: Stripe's Error Testing
In 2022, Stripe improved its error handling tests for its payment processing API. They:
1. Created 50 different error scenarios 2. Ran 10,000 integration tests daily 3. Did stress tests with 1 million requests per minute
Results:
- Found and fixed 37 previously unknown bugs
- Reduced error rates by 22%
- Improved API uptime from 99.95% to 99.99%
Will Gaybrick, Stripe's Chief Product Officer, said: "Our new testing approach helped us catch errors we'd missed before. It's made our system much more stable for our customers."
Tips for Better Error Testing
1. Test often: Run error tests as part of daily development 2. Use real data: Test with actual user data (anonymized) when possible 3. Check edge cases: Test unusual situations, not just common errors 4. Update regularly: Keep test scenarios current with new features and integrations
Keeping Error Handling Up to Date
Error handling in SaaS integrations needs constant attention. Here's how to keep it current:
Regular Error Strategy Reviews
Check your error handling every 3 months:
- Look at how well current methods work
- Find new types of errors
- See how system changes affect error handling
- Update methods based on new best practices
Finding Patterns in Error Logs
Check error logs often to spot issues:
- Use tools to find common errors
- Track how often errors happen
- Link errors to specific parts of the system
- Make charts to see error trends
Improving Error Prevention
Always try to get better at stopping errors:
- Have dev and ops teams share info
- Find the root cause of big errors
- Use more automated testing
- Train staff on error handling
Activity | How Often | Why It Helps |
---|---|---|
Strategy Reviews | Every 3 months | Keeps methods up-to-date |
Log Checks | Weekly | Spots recurring problems |
Prevention Work | All the time | Reduces errors |
Real-World Example: Slack's Error Handling Update
In 2022, Slack improved its error handling:
- Reviewed strategies monthly instead of yearly
- Used AI to analyze error logs
- Trained all engineers on new error prevention methods
Results:
- 40% fewer critical errors
- 30% faster error fix times
- 25% increase in user satisfaction
Cal Henderson, Slack's CTO, said: "Our new approach to error handling has made Slack more stable and reliable for our users. It's not just about fixing errors, but preventing them in the first place."
Tips for Better Error Handling
- Set clear goals for error reduction
- Use tools like Sentry or Rollbar to track errors
- Have a plan for each type of error
- Test your error handling regularly
Wrap-up
Error handling is key for stable SaaS integrations. This guide gives you tools to make your integrations more reliable and user-friendly.
Main points to remember:
- Use multiple ways to handle errors: log them, watch for them, and try again automatically
- Write error messages that both users and tech teams can understand
- Use advanced methods like circuit breakers for complex integrations
- Keep checking and updating how you handle errors
Error handling is ongoing work. Keep watching, studying, and improving your approach to keep your SaaS integrations running smoothly.
Real-World Impact
In 2023, Salesforce improved its error handling:
Action | Result |
---|---|
Added AI-powered error prediction | 70% fewer critical errors per month |
Created a central error dashboard | 75% faster error fix time |
Set up auto-fixes for common issues | 7% increase in customer satisfaction |
Parker Harris, Salesforce co-founder, said: "Our new error handling system has been a game-changer. It's made our platform more stable and our customers happier."
Tips for Better Error Handling
- Set clear goals for reducing errors
- Use tools like Sentry or Rollbar to track errors
- Have a plan for each type of error
- Test your error handling often
By focusing on error management, you'll have less downtime, happier users, and learn how to make your integrations even better.
Next Steps
Make an error handling plan that fits your SaaS integration needs. This will help you stay ahead of problems and keep your edge in the fast-changing SaaS world.
FAQs
How do you handle integration errors?
To handle integration errors effectively:
- Implement a retry strategy with exponential backoff
- Log each attempt for troubleshooting
- Set a maximum retry limit
Here's a practical approach:
Attempt | Wait Time | Action |
---|---|---|
1st | 10 minutes | Retry |
2nd | 20 minutes | Retry |
3rd | 40 minutes | Retry |
4th-10th | Double previous wait time | Retry |
After 10 attempts, stop retrying and alert the support team.
How to handle errors in integration?
Follow these steps to manage integration errors:
- Set up error logging
- Use a retry mechanism
- Implement circuit breakers
- Create clear error messages
Example: Stripe's Error Handling
In 2022, Stripe improved its payment processing API error handling:
Action | Result |
---|---|
Added detailed error logs | 30% faster issue resolution |
Implemented smart retries | 15% more successful transactions |
Set up circuit breakers | 25% reduction in system-wide failures |
Will Gaybrick, Stripe's CPO, said: "Our new error handling approach has significantly improved our API's reliability and user experience."
What are common SaaS integration errors?
Common SaaS integration errors include:
- API version mismatches
- Authentication failures
- Rate limit exceeded
- Data format inconsistencies
- Network timeouts
Real-world example: Salesforce and HubSpot integration
In 2022, an e-commerce company faced these issues:
Error | Cause | Impact |
---|---|---|
Data sync failures | API version mismatch | 30% of customer data not updated |
Duplicate records | Incorrect field mapping | 5,000 duplicate leads created |
Integration timeouts | API rate limiting | 2-hour delay in data syncing |
The company's CTO stated: "These integration issues cost us nearly $100,000 in lost productivity and data cleanup."
How can you prevent SaaS integration errors?
To prevent SaaS integration errors:
- Test thoroughly before deployment
- Keep APIs and documentation up-to-date
- Monitor integration health regularly
- Use webhooks for real-time updates
- Implement proper error handling from the start
Salesforce's Preventive Measures
In 2023, Salesforce improved its error prevention:
Measure | Result |
---|---|
AI-powered error prediction | 70% fewer critical errors per month |
Central error dashboard | 75% faster error fix time |
Auto-fixes for common issues | 7% increase in customer satisfaction |
Parker Harris, Salesforce co-founder, noted: "Our new error handling system has been a game-changer for platform stability and customer happiness."