Using the Sage Accounting API to Get Customers in PHP
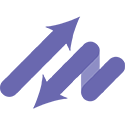
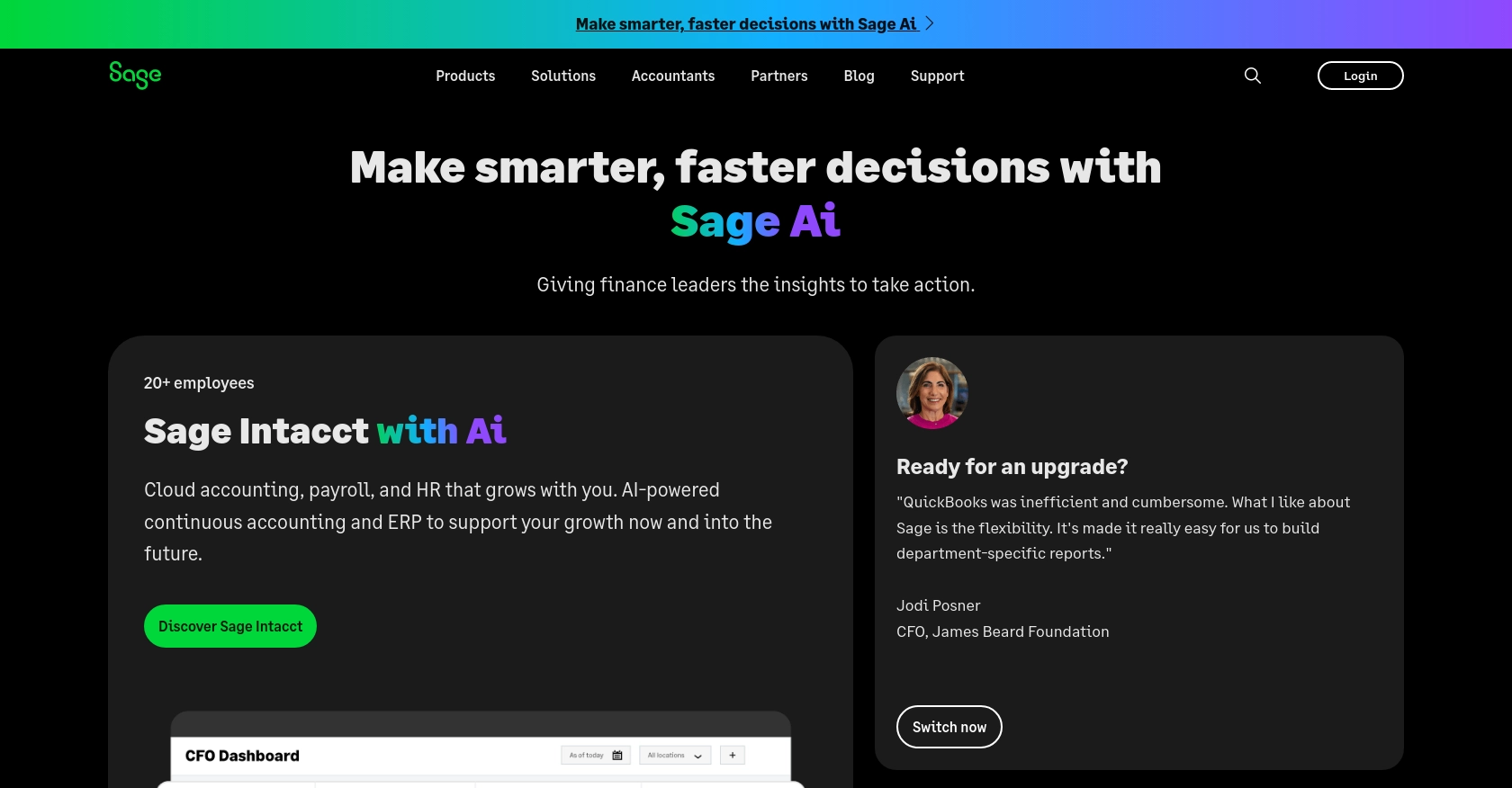
Introduction to Sage Accounting API
Sage Accounting is a robust cloud-based accounting solution tailored for small to medium-sized businesses. It offers a comprehensive suite of tools to manage financial operations, including invoicing, expense tracking, and financial reporting. Sage Accounting's flexibility and ease of use make it a popular choice for businesses aiming to streamline their accounting processes.
Integrating with the Sage Accounting API allows developers to automate and enhance financial workflows. For example, by using the Sage Accounting API, developers can programmatically retrieve customer data, enabling seamless integration with CRM systems or custom applications. This capability is particularly useful for businesses looking to maintain up-to-date customer records and improve their financial operations.
Setting Up Your Sage Accounting Test/Sandbox Account
Before you can start integrating with the Sage Accounting API, you'll need to set up a test or sandbox account. This environment allows you to safely develop and test your application without affecting live data. Follow these steps to get started:
Create a Sage Developer Account
To begin, you'll need a Sage Developer account. This account will enable you to register and manage your applications, obtain client credentials, and specify key details such as callback URLs.
- Visit the Sage Developer Portal.
- Sign up using your GitHub account or an email address.
- Follow the guide on signing up for a developer account.
Register a Trial Business for Development
Sage allows you to create trial businesses for various regions and subscription tiers. This is essential for testing your integration:
- Navigate to the trial business setup page.
- Select the appropriate region and subscription tier for your needs.
- Sign up for a trial account using the provided links.
Create a Sage Accounting App
Once your developer account is ready, the next step is to create an app to obtain the necessary credentials for API access:
- Log in to your Sage Developer account.
- Click on "Create App" and enter the required details such as the app name and callback URL.
- Save your app to generate a Client ID and Client Secret.
- Refer to the Sage guide on creating an app for more details.
Upgrade to a Developer Account
To fully utilize the Sage Accounting API, you may need to upgrade your trial account to a developer account:
- Submit a request through the upgrade form.
- Provide details such as your name, email address, app name, and Client ID.
- Wait for confirmation, which typically takes 3-5 working days.
With your Sage Accounting sandbox account set up, you're now ready to start integrating and testing the API in a safe environment.
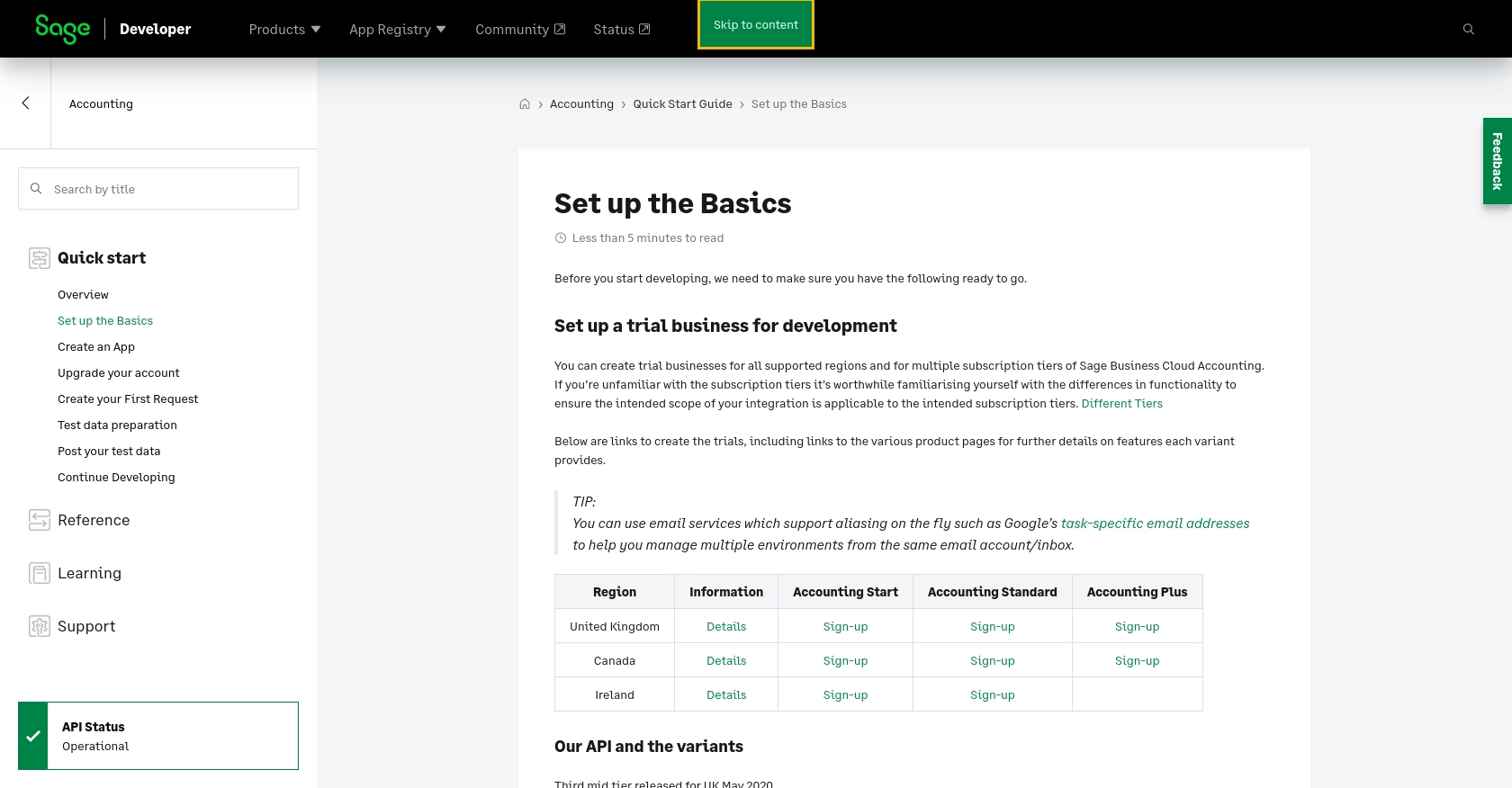
sbb-itb-96038d7
Making API Calls to Retrieve Customers Using Sage Accounting API in PHP
To interact with the Sage Accounting API and retrieve customer data, you'll need to set up your PHP environment and make authenticated requests. This section will guide you through the process of making API calls using PHP, ensuring you can efficiently access customer information from Sage Accounting.
Setting Up Your PHP Environment
Before making API calls, ensure your development environment is ready:
- Install PHP 7.4 or later on your machine.
- Ensure you have Composer installed for managing dependencies.
- Use Composer to install the Guzzle HTTP client, which simplifies making HTTP requests:
composer require guzzlehttp/guzzle
Authenticating with Sage Accounting API
The Sage Accounting API uses OAuth 2.0 for authentication. Follow these steps to authenticate your requests:
- Use your Client ID and Client Secret obtained from your Sage app to request an access token.
- Make a POST request to the Sage OAuth token endpoint to obtain the access token.
use GuzzleHttp\Client;
$client = new Client();
$response = $client->post('https://oauth.accounting.sage.com/token', [
'form_params' => [
'grant_type' => 'client_credentials',
'client_id' => 'Your_Client_ID',
'client_secret' => 'Your_Client_Secret',
],
]);
$tokenData = json_decode($response->getBody(), true);
$accessToken = $tokenData['access_token'];
Retrieving Customers from Sage Accounting API
With the access token, you can now make requests to the Sage Accounting API to retrieve customer data:
$response = $client->get('https://api.accounting.sage.com/v3.1/contacts', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Accept' => 'application/json',
],
]);
$customers = json_decode($response->getBody(), true);
foreach ($customers['contacts'] as $customer) {
echo 'Customer Name: ' . $customer['contact']['name'] . "\n";
}
This code snippet sends a GET request to the Sage Accounting API to fetch customer details. The response is parsed, and customer names are printed to the console.
Handling API Response and Errors
It's crucial to handle potential errors when making API calls. Check the response status code to ensure the request was successful:
if ($response->getStatusCode() === 200) {
// Process the data
} else {
echo 'Error: ' . $response->getStatusCode();
}
Refer to the Sage API documentation for detailed information on error codes and handling strategies.
By following these steps, you can efficiently retrieve customer data from Sage Accounting using PHP, enabling seamless integration with your applications.
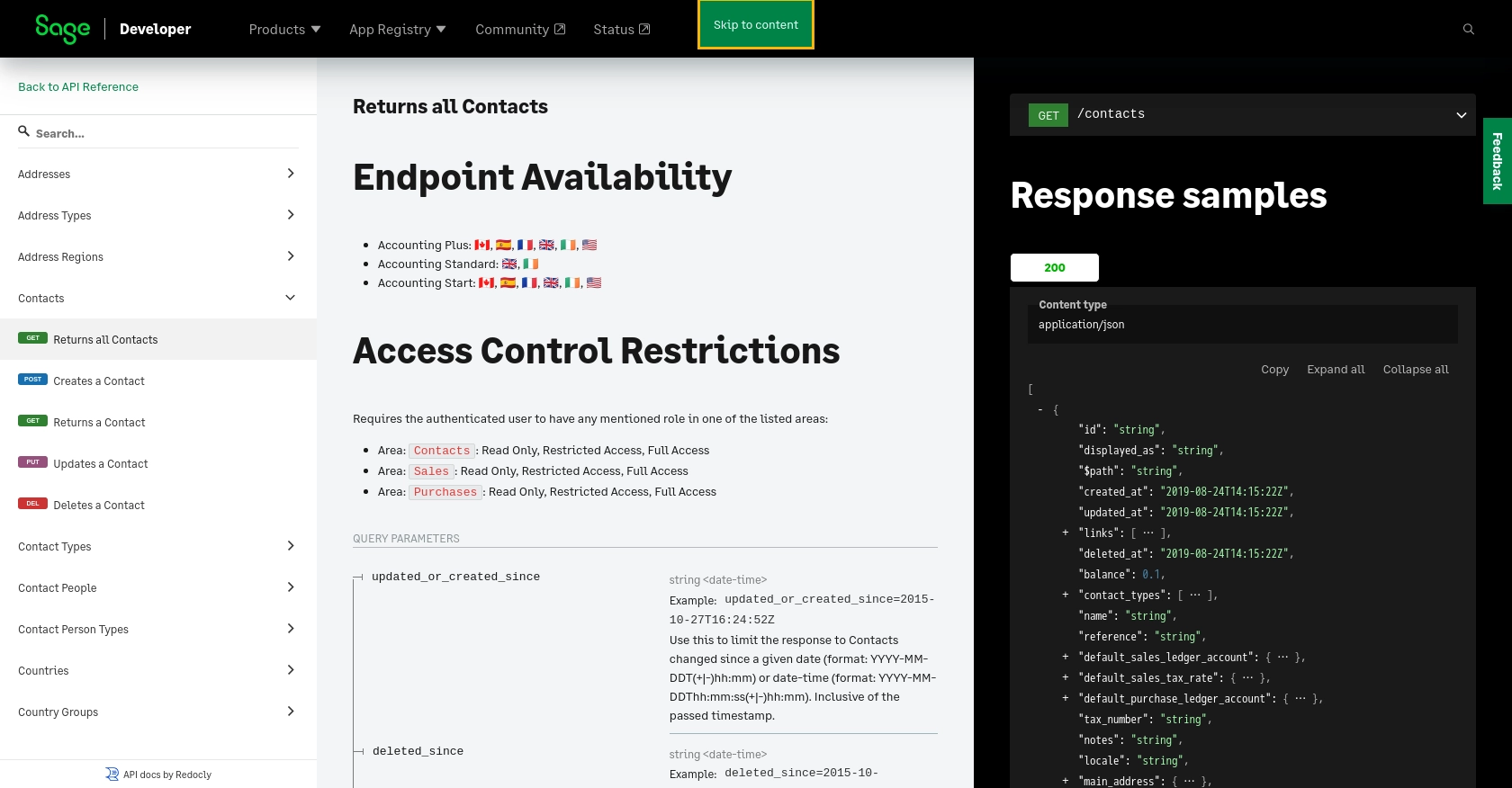
Best Practices for Using Sage Accounting API in PHP
When integrating with the Sage Accounting API, it's essential to follow best practices to ensure secure and efficient operations. Here are some recommendations:
- Securely Store Credentials: Always store your Client ID, Client Secret, and access tokens securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of the API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully. Check the Sage API documentation for specific rate limit details.
- Implement Error Handling: Ensure robust error handling by checking response status codes and handling exceptions. Log errors for troubleshooting and refer to the Sage API documentation for guidance on error codes.
- Data Transformation and Standardization: When retrieving customer data, consider transforming and standardizing fields to match your application's data model. This ensures consistency and simplifies integration with other systems.
Streamlining Integrations with Endgrate
While integrating with Sage Accounting API can enhance your application's capabilities, managing multiple integrations can be complex and time-consuming. This is where Endgrate can help.
Endgrate provides a unified API endpoint that connects to various platforms, including Sage Accounting. By leveraging Endgrate, you can streamline your integration process, reduce development time, and focus on your core product. With Endgrate, you build once for each use case and gain an intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration needs by visiting Endgrate and discover the benefits of outsourcing your integration efforts.
Read More
- https://endgrate.com/provider/sage
- https://developer.sage.com/accounting/quick-start/set-up-the-basics/
- https://developer.sage.com/accounting/quick-start/create-an-app/
- https://developer.sage.com/accounting/quick-start/upgrade-your-account/
- https://developer.sage.com/accounting/reference/contacts/#tag/Contacts/operation/getContacts
Ready to get started?