Using the Slack API to Send Messages (User DM) in Python
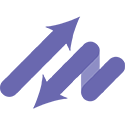
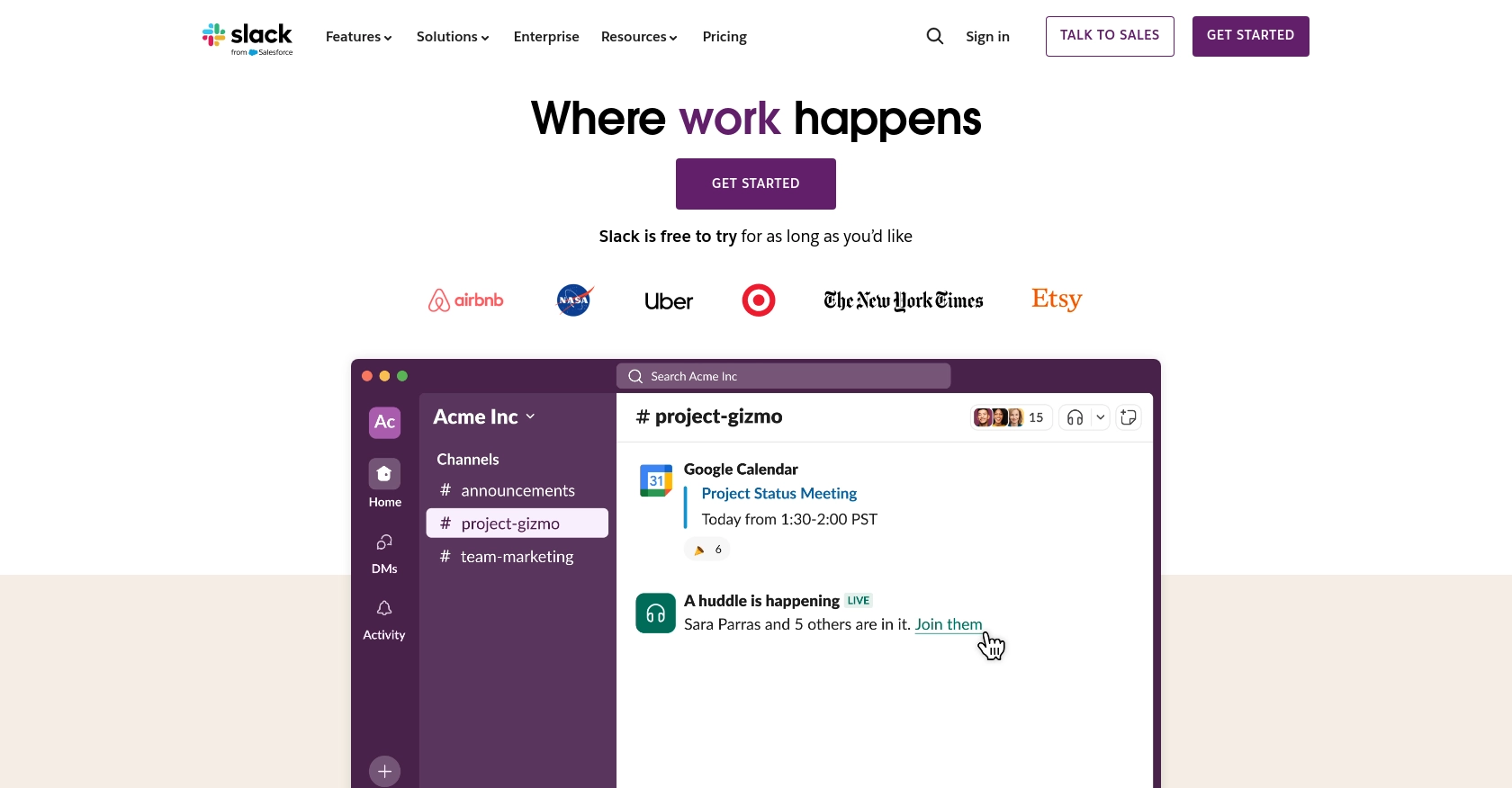
Introduction to Slack API for Direct Messaging
Slack is a powerful collaboration platform that enables teams to communicate and work together efficiently. With its extensive API, developers can build custom integrations to enhance productivity and streamline workflows within Slack workspaces.
Integrating with the Slack API allows developers to automate messaging tasks, such as sending direct messages to users. For example, you could create a bot that sends personalized reminders or notifications directly to a user's Slack inbox, improving communication and engagement within your team.
Setting Up Your Slack Developer Account for API Integration
Before you can start sending direct messages using the Slack API, you'll need to set up a Slack developer account and create an app. This process involves obtaining the necessary credentials and permissions to interact with Slack's API.
Creating a Slack App for API Access
-
Visit the Slack API Quickstart page and log in with your Slack account.
-
Navigate to the "Your Apps" section and click on "Create New App."
-
Select "From scratch" and enter a name for your app. Choose the workspace where you want to develop your app and click "Create App."
Configuring OAuth for Slack API Authentication
Slack uses OAuth 2.0 for authentication, which requires setting up scopes and obtaining an access token.
-
Go to the "OAuth & Permissions" section of your app settings.
-
Under "Scopes," add the following:
- chat:write - Allows your app to send messages.
- channels:read - (Optional) Allows your app to read channel information.
-
Click "Save Changes" and then "Install App to Workspace." Follow the prompts to authorize your app, granting it the necessary permissions.
-
After installation, copy the OAuth access token provided. This token is crucial for making API calls.
Testing Your Slack App in a Sandbox Environment
To ensure your app functions correctly, use a test or sandbox workspace within Slack:
-
Create a new Slack workspace dedicated to testing, or use an existing one that allows for experimentation without affecting live data.
-
Invite your app to a channel within this workspace to test sending messages.
With your Slack app set up and authorized, you're ready to start coding and interacting with the Slack API to send direct messages. In the next section, we'll cover how to make these API calls using Python.
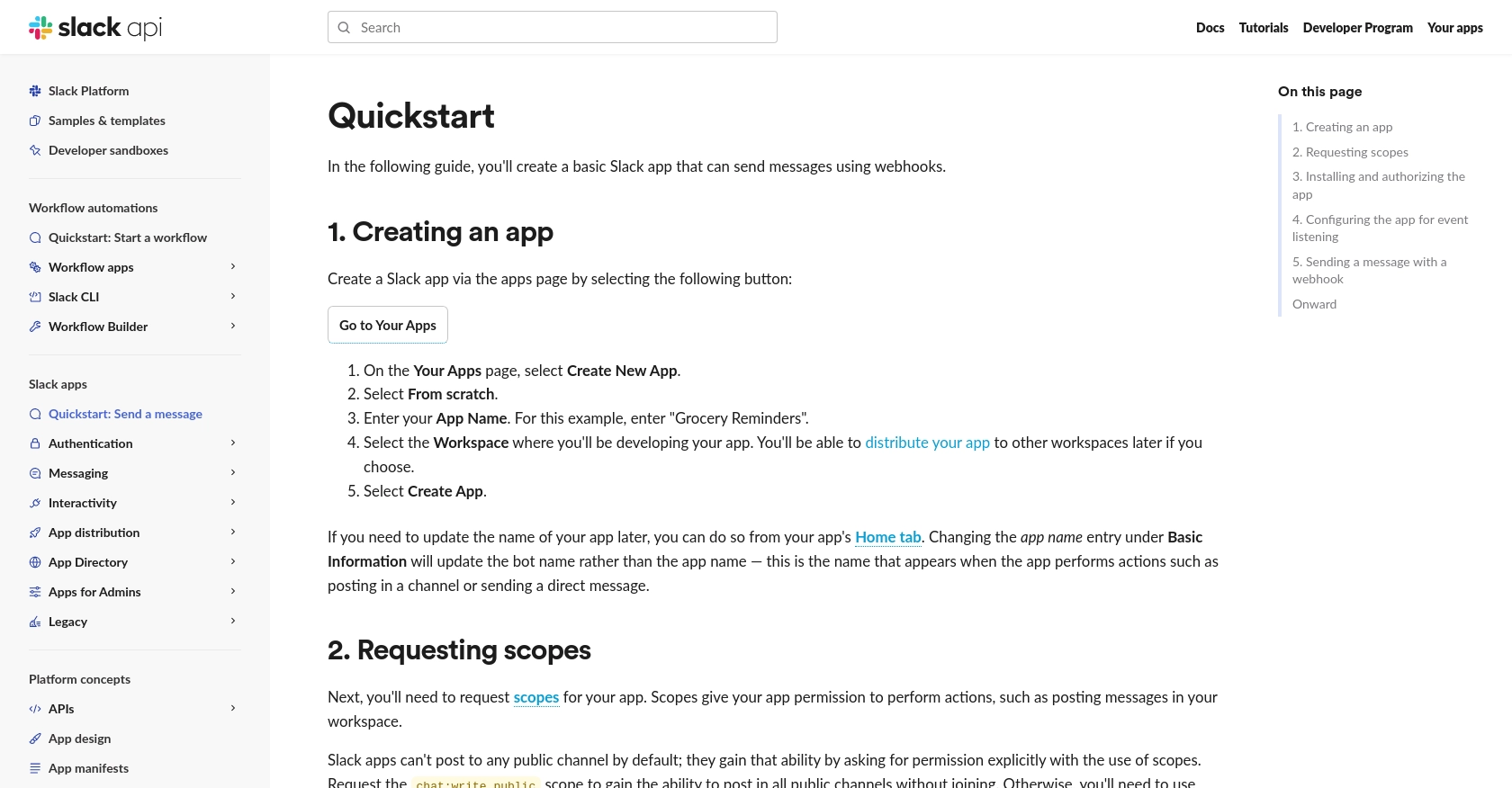
sbb-itb-96038d7
How to Make API Calls to Send Direct Messages Using Slack API in Python
Now that your Slack app is set up and authorized, it's time to dive into coding. We'll use Python to interact with the Slack API and send direct messages to users. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for Slack API Integration
Before you start coding, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Next, install the Slack SDK for Python, which provides a convenient way to interact with the Slack API:
pip install slack_sdk
Writing Python Code to Send Direct Messages via Slack API
With your environment ready, you can now write the Python code to send a direct message using the Slack API. Create a file named send_slack_dm.py
and add the following code:
import os
from slack_sdk import WebClient
from slack_sdk.errors import SlackApiError
# Initialize a WebClient
client = WebClient(token=os.environ['SLACK_BOT_TOKEN'])
def send_direct_message(user_id, message):
try:
# Call the chat.postMessage method using the WebClient
response = client.chat_postMessage(
channel=user_id,
text=message
)
print(f"Message sent to {user_id}: {response['message']['text']}")
except SlackApiError as e:
print(f"Error sending message: {e.response['error']}")
# Replace 'USER_ID' with the actual user ID and 'Hello!' with your message
send_direct_message('USER_ID', 'Hello!')
Replace 'USER_ID'
with the actual Slack user ID you want to message and 'Hello!'
with your desired message content.
Verifying Successful API Calls and Handling Errors
After running your script, check the Slack workspace to verify that the message was delivered to the intended user. If the message appears in the user's direct messages, the API call was successful.
In case of errors, the SlackApiError
will provide details about what went wrong. Common errors include invalid tokens or user IDs. Ensure your OAuth token is correct and that the user ID exists in the workspace.
Best Practices for Slack API Integration in Python
Here are some best practices to consider when integrating with the Slack API:
- Store your OAuth token securely, such as in environment variables, to prevent unauthorized access.
- Handle rate limits by implementing retries with exponential backoff. Slack's API rate limits are documented here.
- Standardize message formats to maintain consistency across different integrations.
By following these practices, you can ensure a robust and secure integration with the Slack API.
.webp)
Conclusion: Best Practices for Using Slack API in Python
Integrating with the Slack API to send direct messages in Python can significantly enhance team communication and automate routine tasks. By following the steps outlined in this guide, you can efficiently set up your Slack app, authenticate using OAuth, and send messages directly to users.
To ensure a successful integration, consider these best practices:
- Secure Token Management: Always store your OAuth tokens securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle API Rate Limits: Implement retries with exponential backoff to manage Slack's API rate limits effectively. This ensures your app remains responsive even under heavy load.
- Consistent Message Formatting: Standardize your message formats to maintain a consistent user experience across different Slack integrations.
- Error Handling: Use the
SlackApiError
to catch and address errors promptly, ensuring smooth operation of your integration.
By adhering to these practices, you can build a robust and secure integration with Slack, enhancing productivity and communication within your team.
Streamline Your Integrations with Endgrate
While building custom integrations can be rewarding, it can also be time-consuming and complex. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including Slack. This allows you to focus on your core product while outsourcing the intricacies of integration development.
With Endgrate, you can build once for each use case instead of multiple times for different integrations, saving time and resources. Take advantage of an easy, intuitive integration experience for your customers and streamline your development process.
Explore how Endgrate can simplify your integration needs by visiting Endgrate today.
Read More
Ready to get started?