How to Create or Update Invoices with the Quickbooks API in PHP
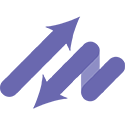
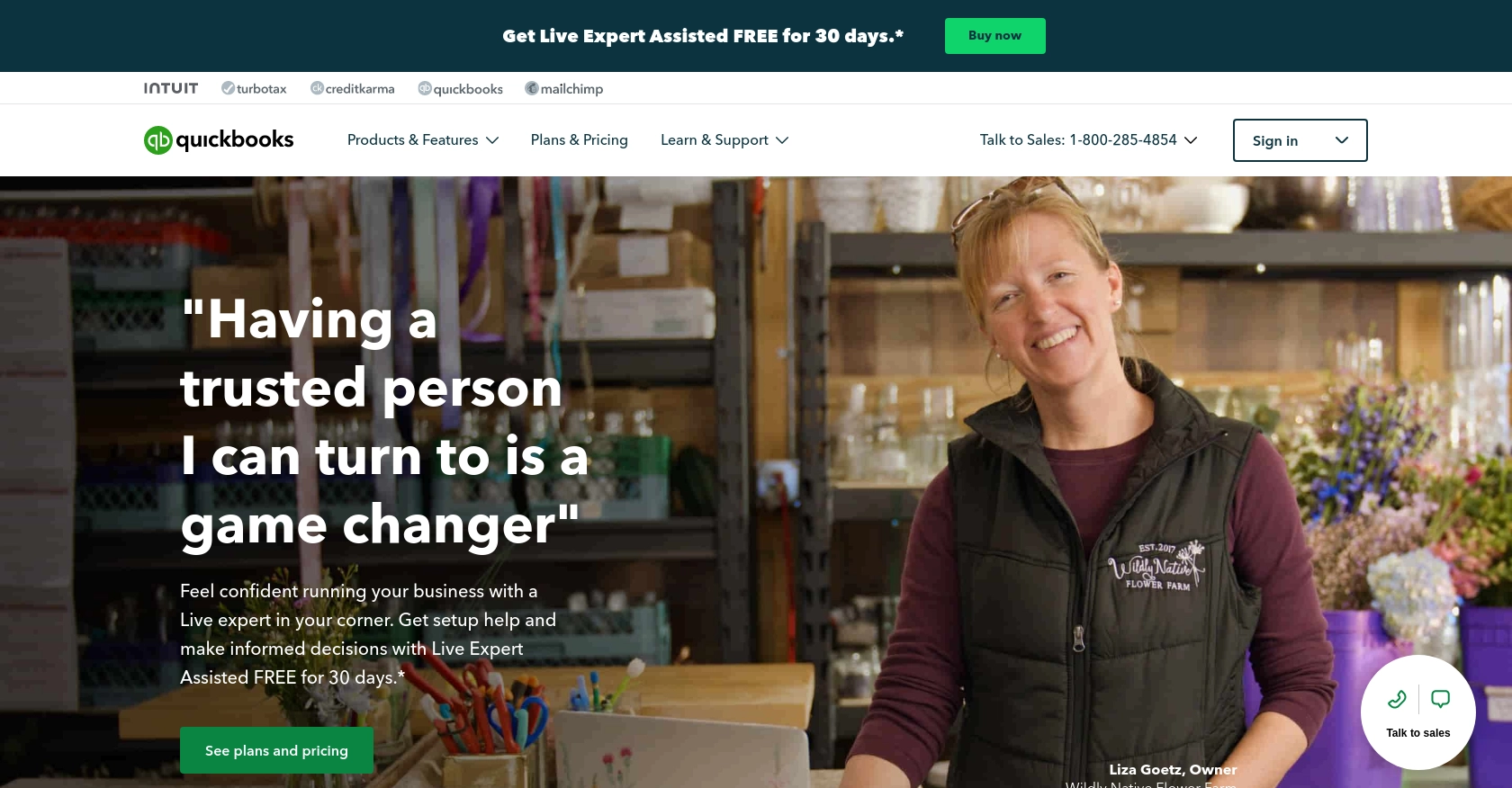
Introduction to QuickBooks API Integration
QuickBooks is a widely-used accounting software that offers comprehensive solutions for managing business finances. It provides tools for invoicing, expense tracking, payroll, and financial reporting, making it an essential platform for businesses of all sizes.
Integrating with the QuickBooks API allows developers to automate and streamline accounting processes, such as creating or updating invoices. For example, a developer might use the QuickBooks API to automatically generate invoices from an e-commerce platform, ensuring that financial records are always up-to-date and accurate.
Setting Up Your QuickBooks Sandbox Account for API Integration
Before you can start creating or updating invoices with the QuickBooks API, you'll need to set up a sandbox account. This allows you to test your integration without affecting live data. QuickBooks provides a sandbox environment specifically for developers to experiment and ensure their applications work seamlessly.
Step 1: Sign Up for a QuickBooks Developer Account
If you don't already have a QuickBooks Developer account, you'll need to create one. Visit the Intuit Developer Portal and follow the instructions to sign up. This account will give you access to the sandbox environment and the necessary tools to build your integration.
Step 2: Create a New App in the QuickBooks Developer Portal
Once your developer account is set up, log in to the QuickBooks Developer Portal and create a new app. This app will be used to generate the credentials needed for OAuth authentication.
- Navigate to the "My Apps" section and click on "Create an App."
- Select "QuickBooks Online and Payments" as the platform.
- Fill in the required details such as app name and description.
Step 3: Obtain Client ID and Client Secret
After creating your app, you'll need to obtain the Client ID and Client Secret, which are essential for OAuth authentication. These credentials allow your application to securely connect to the QuickBooks API.
- Go to the "Keys & OAuth" section of your app settings.
- Copy the Client ID and Client Secret. Keep these credentials secure as they are sensitive information.
- Refer to the Intuit Developer Documentation for more details.
Step 4: Configure OAuth Authentication
QuickBooks uses OAuth 2.0 for authentication. You'll need to set up OAuth to authorize your app to access QuickBooks data.
- In your app settings, configure the redirect URI. This is the URL where users will be redirected after they authorize your app.
- Ensure that your app has the necessary scopes to create and update invoices.
- For detailed instructions, refer to the Intuit Developer Documentation.
With your sandbox account and app configured, you're now ready to start making API calls to QuickBooks. In the next section, we'll explore how to create or update invoices using PHP.
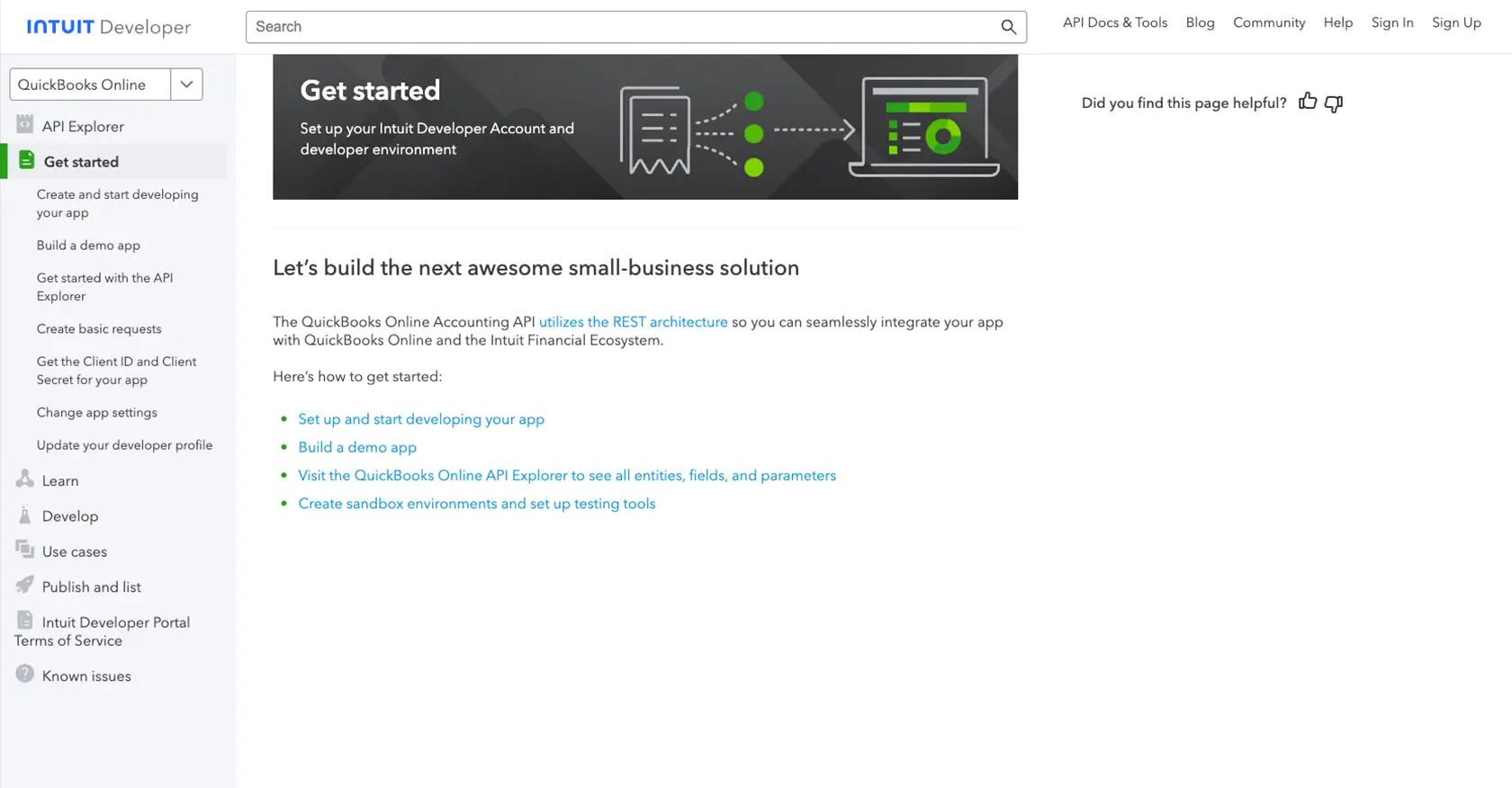
sbb-itb-96038d7
Making API Calls to Create or Update Invoices with QuickBooks API in PHP
To interact with the QuickBooks API for creating or updating invoices, you'll need to use PHP to make HTTP requests. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code to make API calls, and handling responses.
Setting Up Your PHP Environment for QuickBooks API
Before you begin, ensure that you have PHP installed on your machine. You will also need the cURL
extension enabled, as it is essential for making HTTP requests.
- Verify your PHP installation by running
php -v
in your terminal. - Ensure
cURL
is enabled by checking yourphp.ini
file or runningphp -m | grep curl
.
Installing Required PHP Libraries
To simplify OAuth authentication and API requests, you can use the league/oauth2-client
library. Install it using Composer:
composer require league/oauth2-client
Creating or Updating Invoices with QuickBooks API
Now that your environment is ready, you can proceed to write the PHP code to create or update invoices. Below is a sample script demonstrating how to achieve this:
require 'vendor/autoload.php';
use League\OAuth2\Client\Provider\GenericProvider;
// Set up the OAuth 2.0 provider
$provider = new GenericProvider([
'clientId' => 'Your_Client_ID',
'clientSecret' => 'Your_Client_Secret',
'redirectUri' => 'Your_Redirect_URI',
'urlAuthorize' => 'https://appcenter.intuit.com/connect/oauth2',
'urlAccessToken' => 'https://oauth.platform.intuit.com/oauth2/v1/tokens/bearer',
'urlResourceOwnerDetails' => 'https://sandbox-quickbooks.api.intuit.com/v3/company/Your_Company_ID/invoice'
]);
// Obtain an access token
$accessToken = $provider->getAccessToken('authorization_code', [
'code' => 'Authorization_Code_From_QuickBooks'
]);
// Prepare the invoice data
$invoiceData = [
"Line" => [
[
"Amount" => 100.00,
"DetailType" => "SalesItemLineDetail",
"SalesItemLineDetail" => [
"ItemRef" => [
"value" => "1",
"name" => "Services"
]
]
]
],
"CustomerRef" => [
"value" => "1"
]
];
// Make the API request to create or update an invoice
$response = $provider->getAuthenticatedRequest(
'POST',
'https://sandbox-quickbooks.api.intuit.com/v3/company/Your_Company_ID/invoice',
$accessToken,
['json' => $invoiceData]
);
// Handle the response
if ($response->getStatusCode() == 200) {
echo "Invoice created or updated successfully.";
} else {
echo "Failed to create or update invoice. Error: " . $response->getBody();
}
Replace Your_Client_ID
, Your_Client_Secret
, Your_Redirect_URI
, Your_Company_ID
, and Authorization_Code_From_QuickBooks
with your actual credentials and authorization code.
Verifying API Call Success in QuickBooks Sandbox
After running the script, you can verify the success of your API call by checking the QuickBooks sandbox environment. Navigate to the invoices section to see if the invoice has been created or updated as expected.
Handling Errors and QuickBooks API Error Codes
When making API calls, it's crucial to handle potential errors. The QuickBooks API may return various error codes, such as 400 for bad requests or 401 for unauthorized access. Ensure your code checks the response status and handles errors gracefully.
For more detailed error information, refer to the Intuit Developer Documentation.
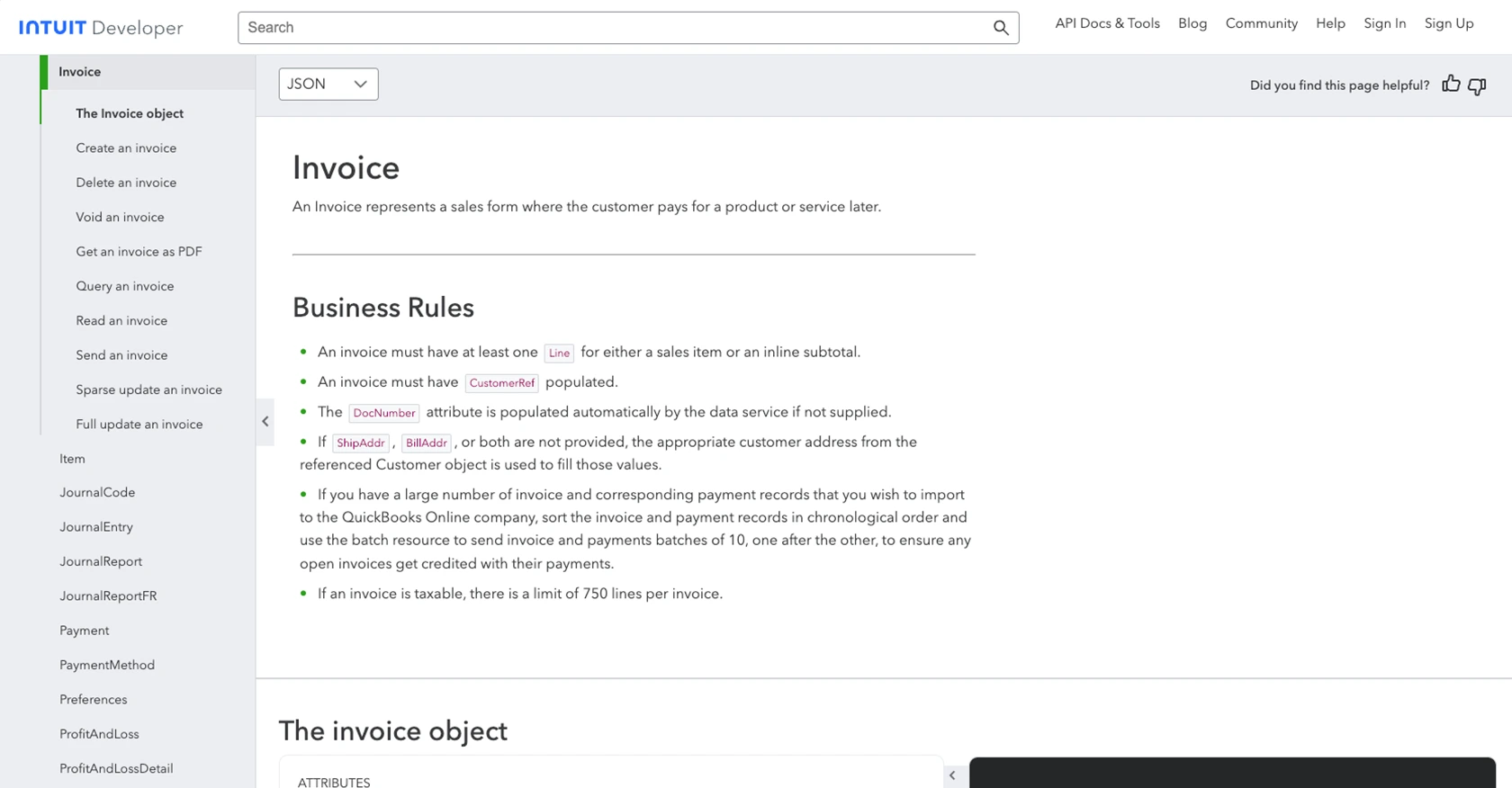
Best Practices for QuickBooks API Integration in PHP
When working with the QuickBooks API, it's essential to follow best practices to ensure a secure and efficient integration. Here are some key recommendations:
- Securely Store Credentials: Always store your Client ID, Client Secret, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: QuickBooks API has rate limits to prevent abuse. Be sure to implement logic to handle rate limit responses and retry requests if necessary. Refer to the Intuit Developer Documentation for specific rate limit details.
- Standardize Data Fields: Ensure that the data you send to QuickBooks is standardized and validated to prevent errors and maintain data integrity.
- Error Handling: Implement comprehensive error handling to manage various API error codes, such as 400 for bad requests or 401 for unauthorized access. This will help in diagnosing issues quickly and maintaining a robust integration.
Streamlining Your Integration Process with Endgrate
Integrating with multiple platforms like QuickBooks can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including QuickBooks.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the complexities of integration.
- Build Once, Deploy Everywhere: Create a single integration that works across multiple platforms, reducing development time and effort.
- Enhance Customer Experience: Offer your customers a seamless and intuitive integration experience with minimal effort.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/all-entities/invoice
Ready to get started?