How to Create or Update Payments with the Younium API in PHP
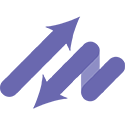
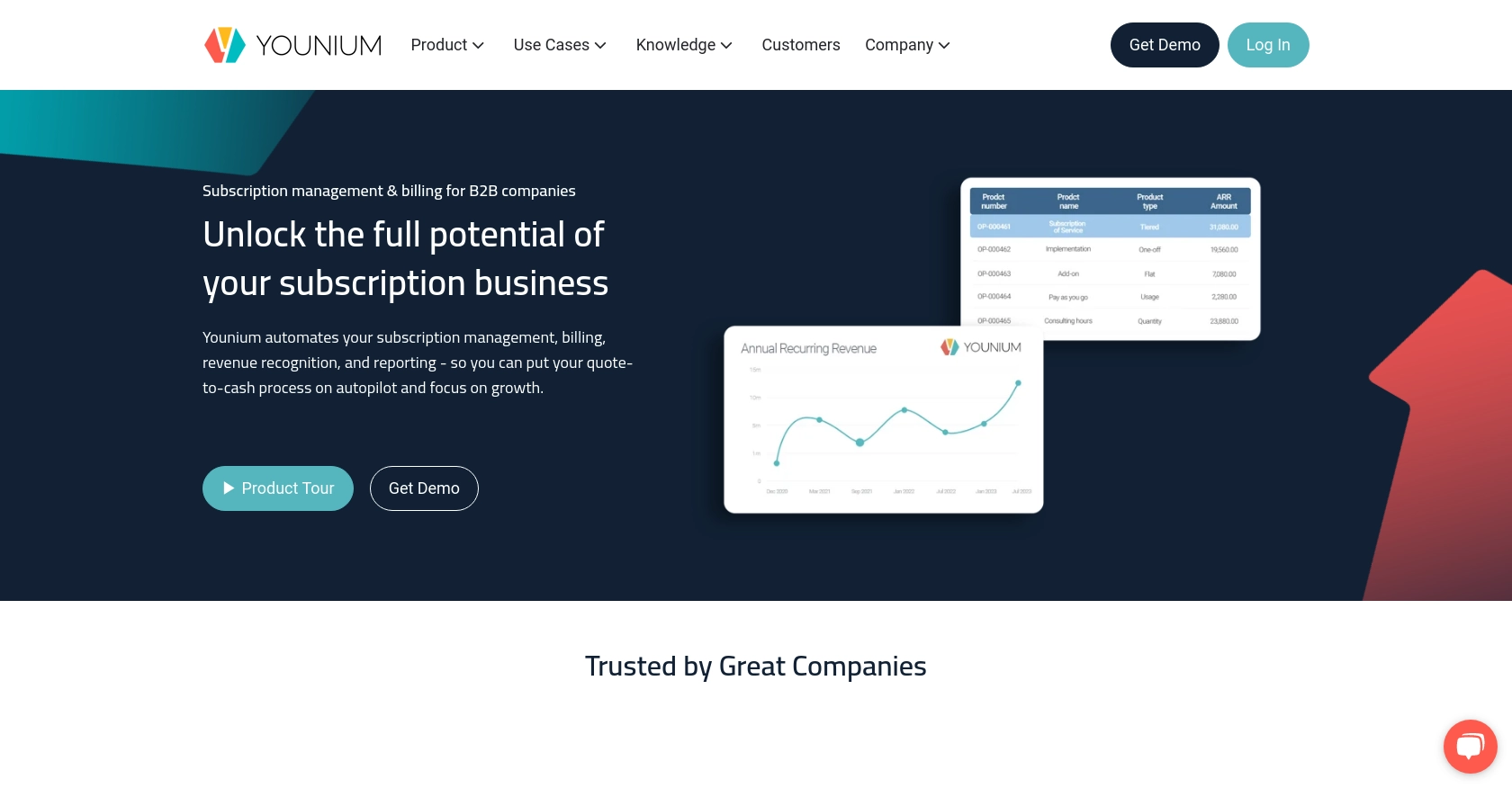
Introduction to Younium API for Payment Management
Younium is a comprehensive subscription management platform designed to streamline billing and financial operations for B2B SaaS companies. It offers a robust API that enables developers to automate and manage various financial processes, including invoicing, payments, and subscriptions.
Integrating with the Younium API allows developers to efficiently create or update payment records, ensuring seamless financial transactions and accurate accounting. For example, a developer might use the Younium API to automate the process of updating payment statuses in real-time, enhancing the financial workflow and reducing manual errors.
Setting Up a Younium Sandbox Account for API Integration
Before you can start creating or updating payments with the Younium API, you'll need to set up a sandbox account. This environment allows you to test and develop your integration without affecting live data, ensuring a smooth and error-free implementation.
Creating a Younium Sandbox Account
To begin, you'll need to create a sandbox account on the Younium platform. Follow these steps to get started:
- Visit the Younium Developer Portal and sign up for a developer account.
- Once registered, log in to your account and navigate to the sandbox environment setup section.
- Follow the on-screen instructions to create your sandbox account, ensuring you have access to all necessary features for testing the API.
Generating API Tokens and Client Credentials
Younium uses JWT access tokens for API authentication. Here’s how to generate the necessary credentials:
- Open the user profile menu by clicking your name in the top right corner and select “Privacy & Security.”
- In the left panel, click on “Personal Tokens” and then “Generate Token.”
- Provide a relevant description for the token and click “Create.”
- Copy the generated Client ID and Secret Key immediately, as they will not be visible again.
Acquiring a JWT Access Token
With your client credentials, you can now acquire a JWT access token:
// Set up the request to acquire the JWT token
$url = 'https://api.sandbox.younium.com/auth/token';
$headers = ['Content-Type: application/json'];
$body = json_encode([
'clientId' => 'Your_Client_ID',
'secret' => 'Your_Secret_Key'
]);
// Use cURL to make the POST request
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $body);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
// Decode the response to get the access token
$data = json_decode($response, true);
$accessToken = $data['accessToken'];
Replace Your_Client_ID
and Your_Secret_Key
with the credentials you generated earlier. If successful, the response will include an accessToken
which is valid for 24 hours.
Handling Authentication Errors
If you encounter errors during authentication, check the following:
- A 401 Unauthorized response indicates an expired, missing, or incorrect access token.
- A 403 Forbidden response may occur if the legal entity specified in the header is incorrect or if the user lacks necessary permissions.
For more details, refer to the Younium Authentication Documentation.
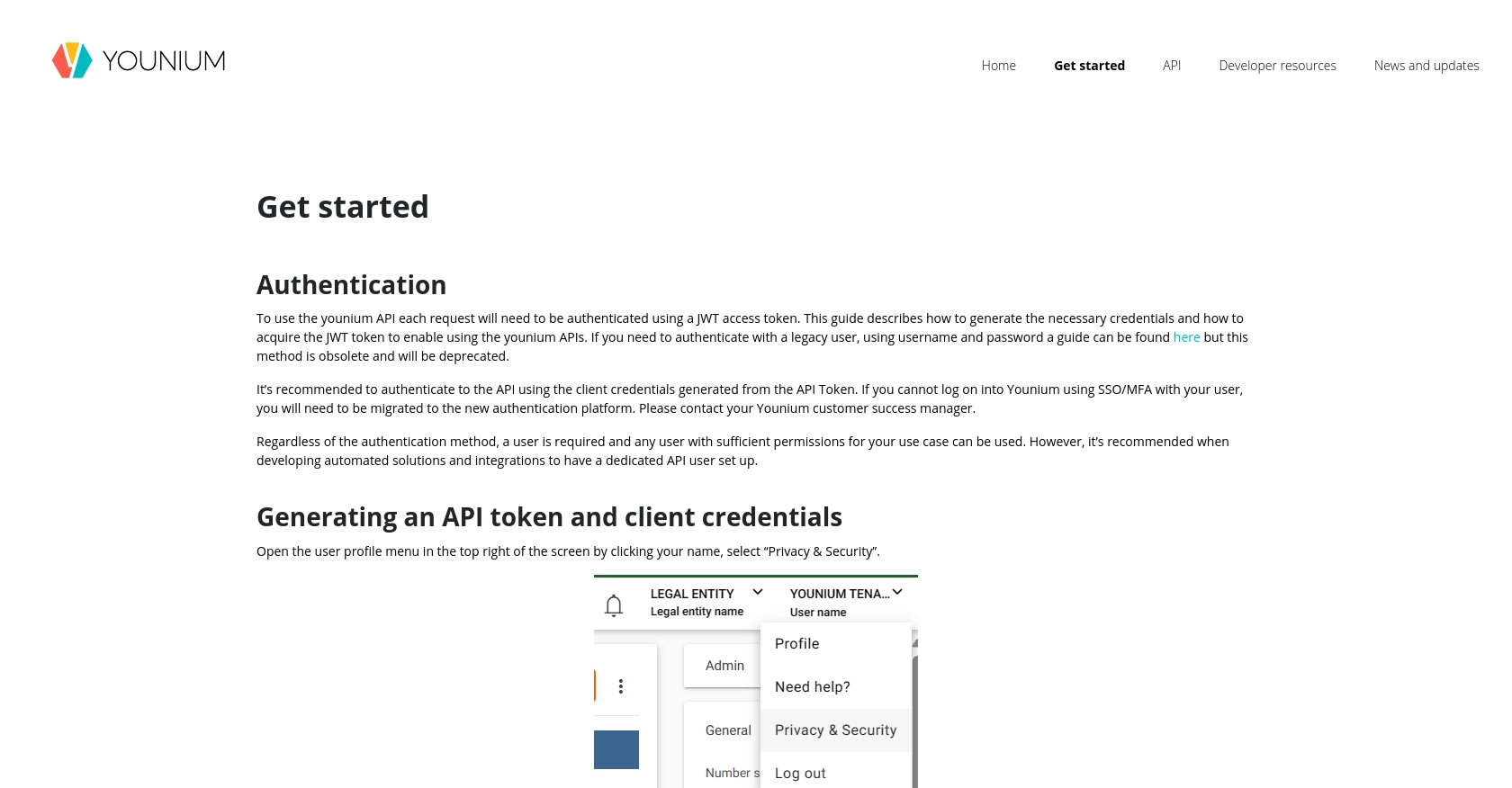
sbb-itb-96038d7
Making API Calls to Create or Update Payments with Younium in PHP
To interact with the Younium API for creating or updating payments, you'll need to make authenticated API calls using PHP. This section will guide you through the process, including setting up your PHP environment, writing the necessary code, and handling potential errors.
Setting Up Your PHP Environment for Younium API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need:
- PHP 7.4 or later
- cURL extension enabled
Verify your PHP version and cURL installation by running the following commands:
php -v
php -m | grep curl
Creating or Updating Payments with Younium API
Once your environment is ready, you can proceed to create or update payments using the Younium API. Here's a step-by-step guide:
// Define the API endpoint for creating or updating payments
$url = 'https://api.sandbox.younium.com/payments';
// Set up the request headers
$headers = [
'Authorization: Bearer ' . $accessToken,
'Content-Type: application/json'
];
// Define the payment data
$paymentData = json_encode([
'account' => 'A-000001',
'amount' => 1000,
'currency' => 'USD',
'paymentDate' => '2023-10-01',
'description' => 'Payment for Invoice #12345'
]);
// Initialize cURL session
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $paymentData);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
curl_close($ch);
// Decode the response
$responseData = json_decode($response, true);
// Check if the payment was successfully created or updated
if ($responseData['successful']) {
echo "Payment processed successfully. Payment ID: " . $responseData['id'];
} else {
echo "Failed to process payment: " . $responseData['message'];
}
Replace the placeholder values in the $paymentData
array with your actual payment details. The $accessToken
should be the JWT token obtained during authentication.
Verifying API Call Success in Younium Sandbox
After executing the API call, verify the success of the operation by checking the response. A successful response will include a successful
flag set to true
and a payment ID. You can also log into your Younium sandbox account to confirm the payment record.
Handling Errors and Common Issues
When making API calls, you may encounter errors. Here are some common issues and how to address them:
- 400 Bad Request: Check the request body for missing or incorrect fields.
- 401 Unauthorized: Ensure your access token is valid and not expired.
- 403 Forbidden: Verify the legal entity specified in the headers and check user permissions.
For more detailed error handling, refer to the Younium API Documentation.
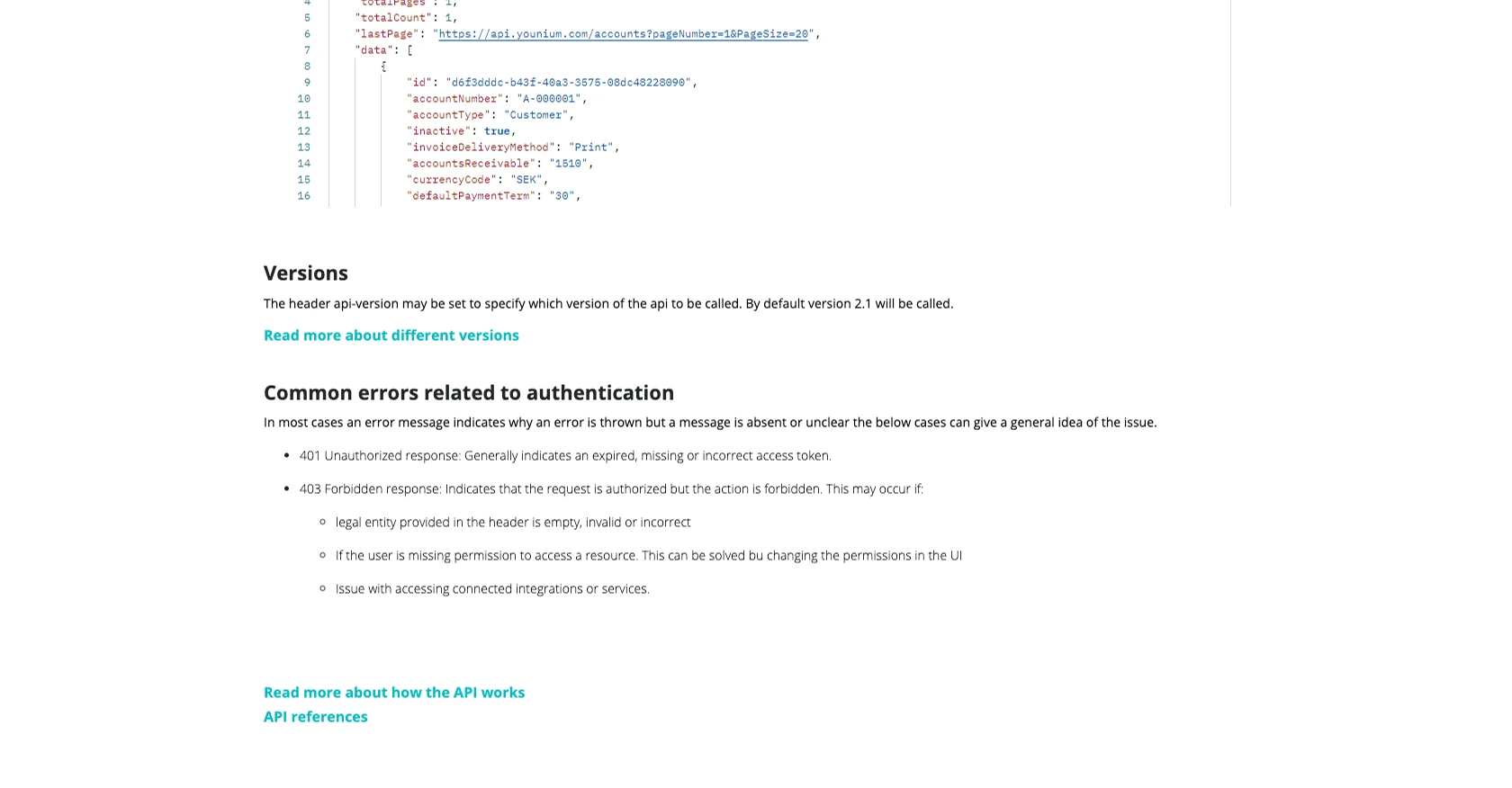
Conclusion and Best Practices for Using Younium API in PHP
Integrating with the Younium API allows developers to streamline payment processes and enhance financial operations within B2B SaaS environments. By following the steps outlined in this guide, you can efficiently create or update payments using PHP, ensuring accurate and timely financial transactions.
Best Practices for Secure and Efficient Younium API Integration
- Securely Store Credentials: Always store your API credentials securely. Consider using environment variables or secure vaults to manage sensitive information like client IDs and secret keys.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Younium API. Implement retry logic and exponential backoff strategies to handle rate limit responses gracefully.
- Data Standardization: Ensure that data fields are standardized across your application to maintain consistency and avoid errors during API interactions.
- Regularly Refresh Tokens: Since JWT tokens are valid for 24 hours, implement a mechanism to refresh tokens automatically to avoid authentication failures.
Enhancing Integration Efficiency with Endgrate
For developers looking to simplify and scale their integration efforts, consider leveraging Endgrate. By using Endgrate, you can:
- Save time and resources by outsourcing complex integrations, allowing your team to focus on core product development.
- Build once for each use case, reducing the need for multiple integrations across different platforms.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website.
Read More
Ready to get started?