Using the Outreach API to Create or Update Prospects in PHP
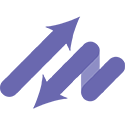
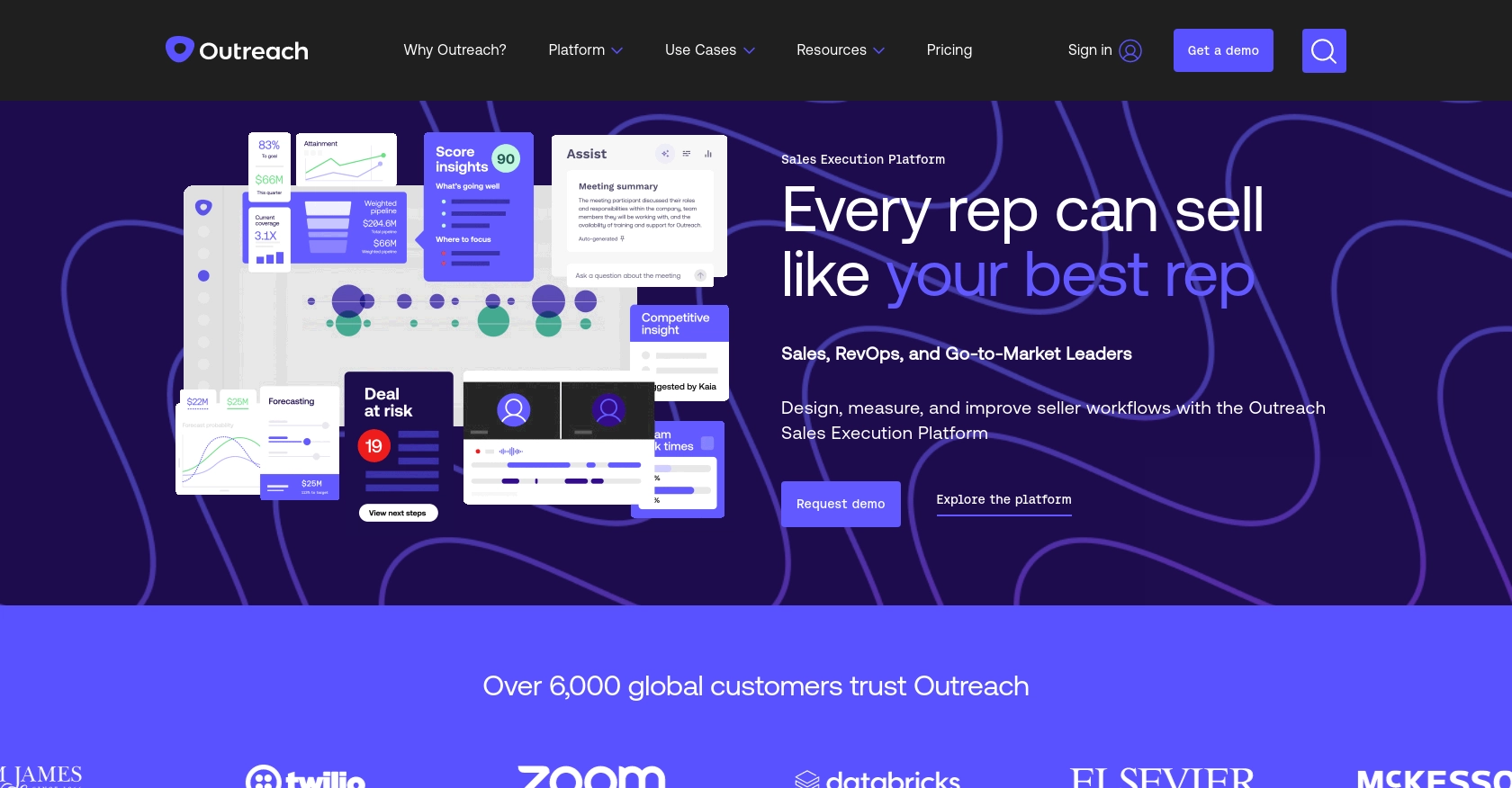
Introduction to Outreach API for Prospect Management
Outreach is a leading sales engagement platform that empowers sales teams to streamline their workflows and enhance productivity. By providing tools for managing customer interactions, automating repetitive tasks, and analyzing sales data, Outreach helps businesses optimize their sales processes.
Integrating with the Outreach API allows developers to automate and manage prospect data efficiently. For example, using the Outreach API, a developer can create or update prospect information directly from a CRM system, ensuring that sales teams have access to the most up-to-date data without manual entry.
This article will guide you through using PHP to interact with the Outreach API, focusing on creating or updating prospects. By following this tutorial, you'll learn how to streamline your sales operations and enhance data accuracy within your Outreach platform.
Setting Up Your Outreach Test or Sandbox Account
Before you can start integrating with the Outreach API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Outreach provides a development environment where you can create and test applications using OAuth 2.0 authentication.
Creating an Outreach Developer Account
If you don't have an Outreach account, begin by signing up for a developer account on the Outreach website. This will give you access to the necessary tools and resources to start building your integration.
- Visit the Outreach Developer Portal.
- Follow the instructions to create a new account.
- Once your account is set up, log in to access the developer dashboard.
Creating an Outreach App for OAuth Authentication
To interact with the Outreach API, you'll need to create an app within your developer account. This app will provide the OAuth credentials required for authentication.
- Navigate to the "My Apps" section in the Outreach Developer Portal.
- Click on "Create New App" and fill in the necessary details, such as the app name and description.
- Specify one or more redirect URIs where Outreach will send the authorization code after user consent.
- Select the OAuth scopes your application will need. Ensure you include scopes related to prospects, such as
prospects.read
andprospects.write
. - Save the app to generate your client ID and client secret. Note that the client secret will only be displayed once, so store it securely.
Obtaining OAuth Tokens
With your app created, you can now obtain OAuth tokens to authenticate API requests. Follow these steps to get your access token:
- Redirect users to the following URL to request authorization:
https://api.outreach.io/oauth/authorize?client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&response_type=code&scope=prospects.read+prospects.write
. - After user consent, Outreach will redirect to your specified URI with an authorization code.
- Exchange this code for an access token by making a POST request to
https://api.outreach.io/oauth/token
with the following parameters:
curl -X POST https://api.outreach.io/oauth/token \
-d client_id=YOUR_CLIENT_ID \
-d client_secret=YOUR_CLIENT_SECRET \
-d redirect_uri=YOUR_REDIRECT_URI \
-d grant_type=authorization_code \
-d code=AUTHORIZATION_CODE
This request will return a JSON response containing your access token and refresh token. Store these tokens securely, as they are required for making authenticated API calls.
For more detailed information on setting up OAuth, refer to the Outreach OAuth Documentation.
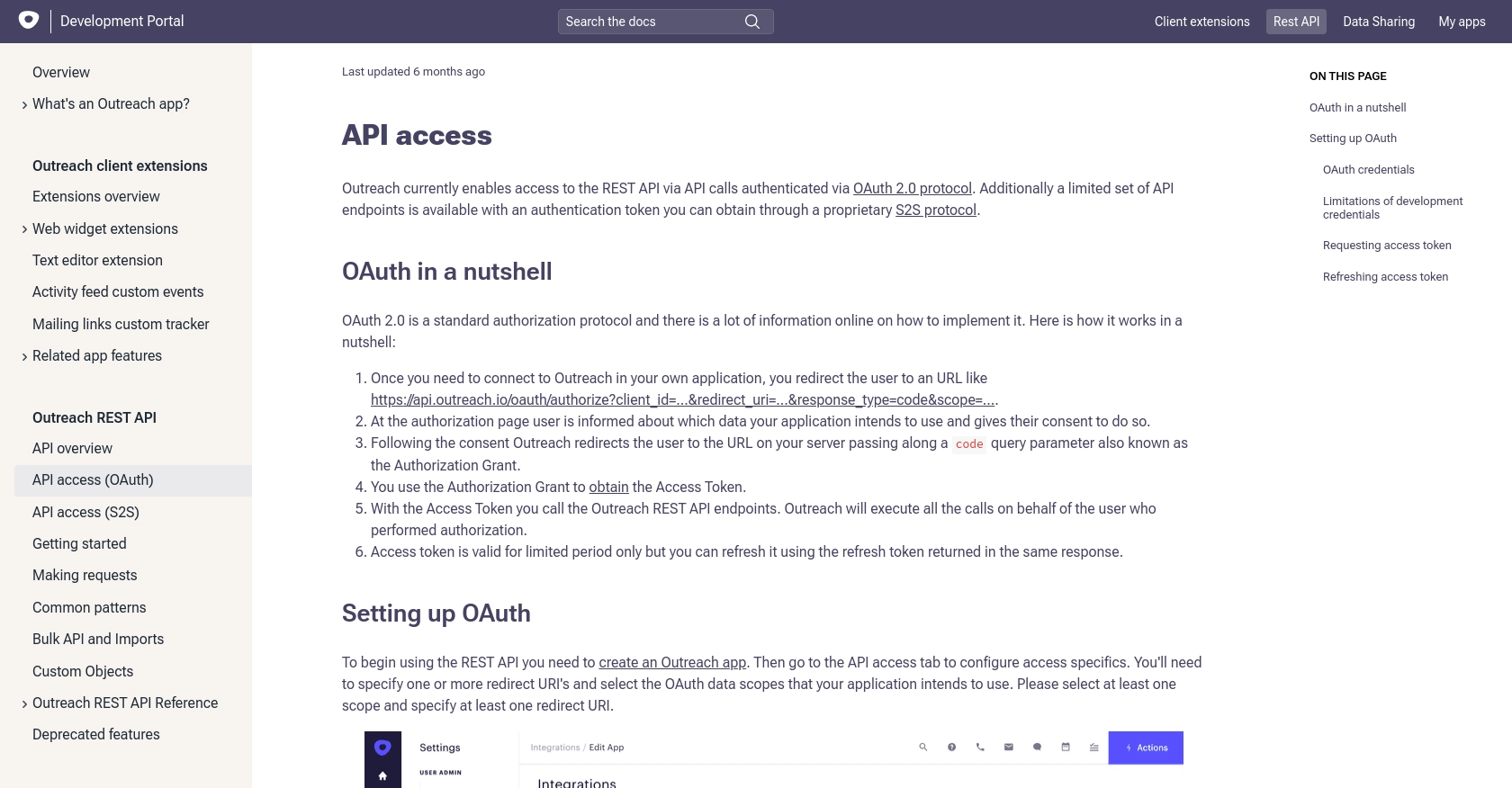
sbb-itb-96038d7
Making API Calls to Create or Update Prospects in Outreach Using PHP
To interact with the Outreach API for creating or updating prospects, you'll need to use PHP to make HTTP requests. This section will guide you through the process of setting up your PHP environment, writing the necessary code, and handling responses from the API.
Setting Up Your PHP Environment for Outreach API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need the following:
- PHP 7.4 or later
- cURL extension enabled
To check your PHP version and cURL extension, run the following command in your terminal:
php -v && php -m | grep curl
Creating a New Prospect in Outreach Using PHP
To create a new prospect, you'll need to send a POST request to the Outreach API. Here's a sample PHP script to achieve this:
<?php
$accessToken = 'YOUR_ACCESS_TOKEN';
$url = 'https://api.outreach.io/api/v2/prospects';
$data = [
'data' => [
'type' => 'prospect',
'attributes' => [
'firstName' => 'John',
'lastName' => 'Doe',
'emails' => ['john.doe@example.com']
]
]
];
$options = [
CURLOPT_URL => $url,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_HTTPHEADER => [
'Authorization: Bearer ' . $accessToken,
'Content-Type: application/vnd.api+json'
],
CURLOPT_POST => true,
CURLOPT_POSTFIELDS => json_encode($data)
];
$ch = curl_init();
curl_setopt_array($ch, $options);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
?>
Replace YOUR_ACCESS_TOKEN
with the access token obtained from the OAuth process. This script sends a POST request to create a new prospect with the specified attributes.
Updating an Existing Prospect in Outreach Using PHP
To update an existing prospect, you'll need to send a PATCH request. Here's how you can do it:
<?php
$accessToken = 'YOUR_ACCESS_TOKEN';
$prospectId = 'PROSPECT_ID';
$url = 'https://api.outreach.io/api/v2/prospects/' . $prospectId;
$data = [
'data' => [
'type' => 'prospect',
'id' => $prospectId,
'attributes' => [
'firstName' => 'Jane',
'lastName' => 'Doe'
]
]
];
$options = [
CURLOPT_URL => $url,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_HTTPHEADER => [
'Authorization: Bearer ' . $accessToken,
'Content-Type: application/vnd.api+json'
],
CURLOPT_CUSTOMREQUEST => 'PATCH',
CURLOPT_POSTFIELDS => json_encode($data)
];
$ch = curl_init();
curl_setopt_array($ch, $options);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
?>
Replace PROSPECT_ID
with the ID of the prospect you wish to update. This script updates the prospect's first and last name.
Handling API Responses and Errors
After making API calls, it's crucial to handle responses and potential errors. The Outreach API returns JSON responses, which you can decode and process in PHP:
<?php
$responseData = json_decode($response, true);
if (isset($responseData['errors'])) {
echo 'Error: ' . $responseData['errors'][0]['detail'];
} else {
echo 'Success: Prospect data updated.';
}
?>
This code checks for errors in the response and prints an appropriate message. For more information on error handling, refer to the Outreach API Documentation.
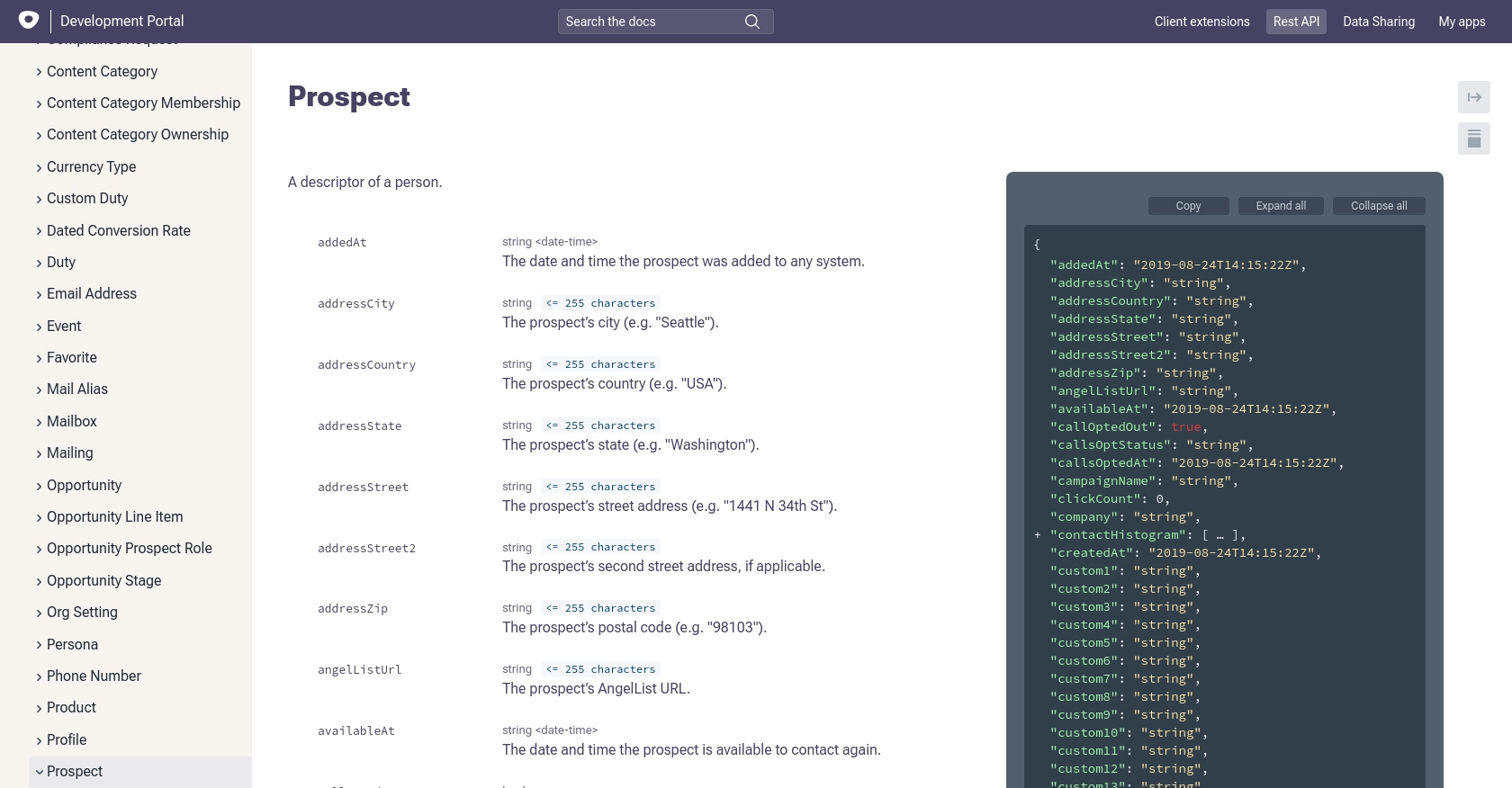
Best Practices for Using Outreach API in PHP
When integrating with the Outreach API, it's essential to follow best practices to ensure security, efficiency, and maintainability. Here are some recommendations:
- Securely Store Credentials: Always store your OAuth tokens securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: The Outreach API has a rate limit of 10,000 requests per hour. Implement logic to handle 429 errors gracefully and retry requests after the specified time. Monitor the
X-RateLimit-Remaining
header to manage your request quota effectively. - Use Refresh Tokens: Access tokens expire after two hours. Use the refresh token to obtain new access tokens without requiring user reauthorization. Always use the latest refresh token provided in the response.
- Validate API Responses: Always check the API response for errors and handle them appropriately. Use the error messages to debug and improve your integration.
- Standardize Data Fields: Ensure that data fields are consistent across different systems. This helps in maintaining data integrity and simplifies data processing.
Enhance Your Integration with Endgrate
Building and maintaining integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Outreach. With Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers, enhancing their satisfaction and engagement.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate today.
Read More
Ready to get started?