Using the Zoho Books API to Get Accounts (with Javascript examples)
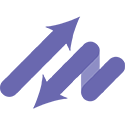
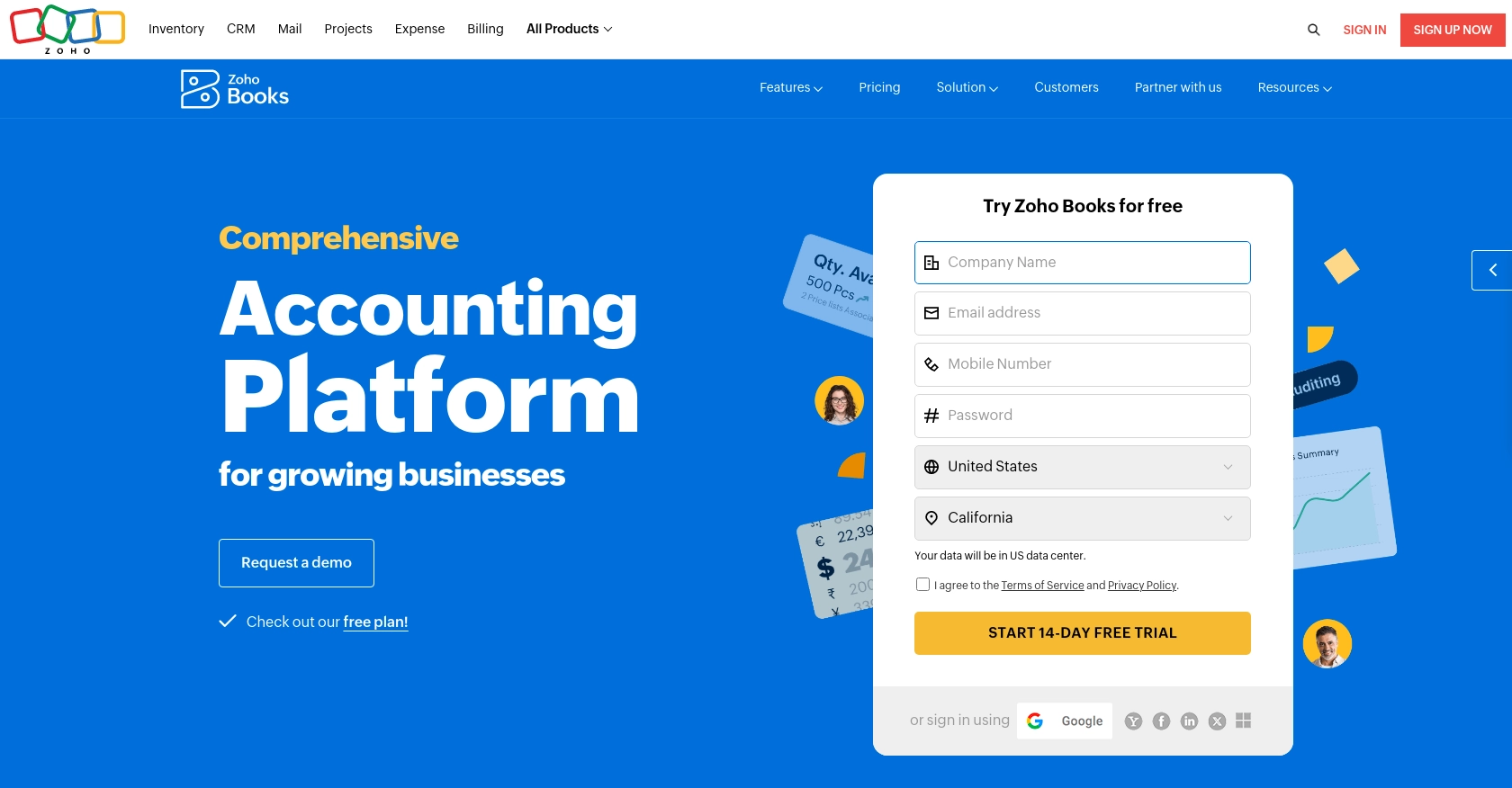
Introduction to Zoho Books API Integration
Zoho Books is a comprehensive online accounting software designed to manage your finances, automate business workflows, and help you work collectively across departments. It offers a wide range of features including invoicing, expense tracking, and inventory management, making it a popular choice for businesses of all sizes.
Integrating with the Zoho Books API allows developers to access and manage financial data programmatically, enabling automation and customization of accounting tasks. For example, a developer might use the Zoho Books API to retrieve account details and generate financial reports, streamlining the accounting process and reducing manual effort.
This article will guide you through using JavaScript to interact with the Zoho Books API, specifically focusing on retrieving account information. By the end of this tutorial, you'll be equipped to efficiently access and manage accounts within Zoho Books using JavaScript.
Setting Up Your Zoho Books Test/Sandbox Account for API Integration
Before you can start interacting with the Zoho Books API using JavaScript, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data. Zoho Books provides a free trial that you can use for this purpose.
Creating a Zoho Books Account
To begin, you'll need to create a Zoho Books account if you don't already have one. Follow these steps:
- Visit the Zoho Books signup page.
- Fill in the required information, such as your email address and password, to create your account.
- Once your account is created, you'll be logged into the Zoho Books dashboard.
Setting Up OAuth for Zoho Books API Access
Zoho Books uses OAuth 2.0 for authentication, which ensures secure access to the API. Follow these steps to set up OAuth:
- Go to the Zoho Developer Console and log in with your Zoho account credentials.
- Click on "Add Client ID" to register a new application.
- Provide the necessary details, such as the client name and redirect URI, and submit the form.
- Upon successful registration, you'll receive a Client ID and Client Secret. Keep these credentials secure as they are essential for API access.
Generating OAuth Tokens for Zoho Books API
With your Client ID and Client Secret, you can now generate OAuth tokens to authenticate API requests:
- Redirect users to the following URL to obtain a grant token:
- After the user consents, Zoho will redirect to your specified URI with a code parameter.
- Exchange this code for an access token by making a POST request:
- Store the access token securely, as it will be used in the Authorization header for API requests.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.fullaccess.all&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI&access_type=offline
https://accounts.zoho.com/oauth/v2/token?code=YOUR_CODE&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
With your Zoho Books account and OAuth setup complete, you're ready to start making API calls to retrieve account information using JavaScript.
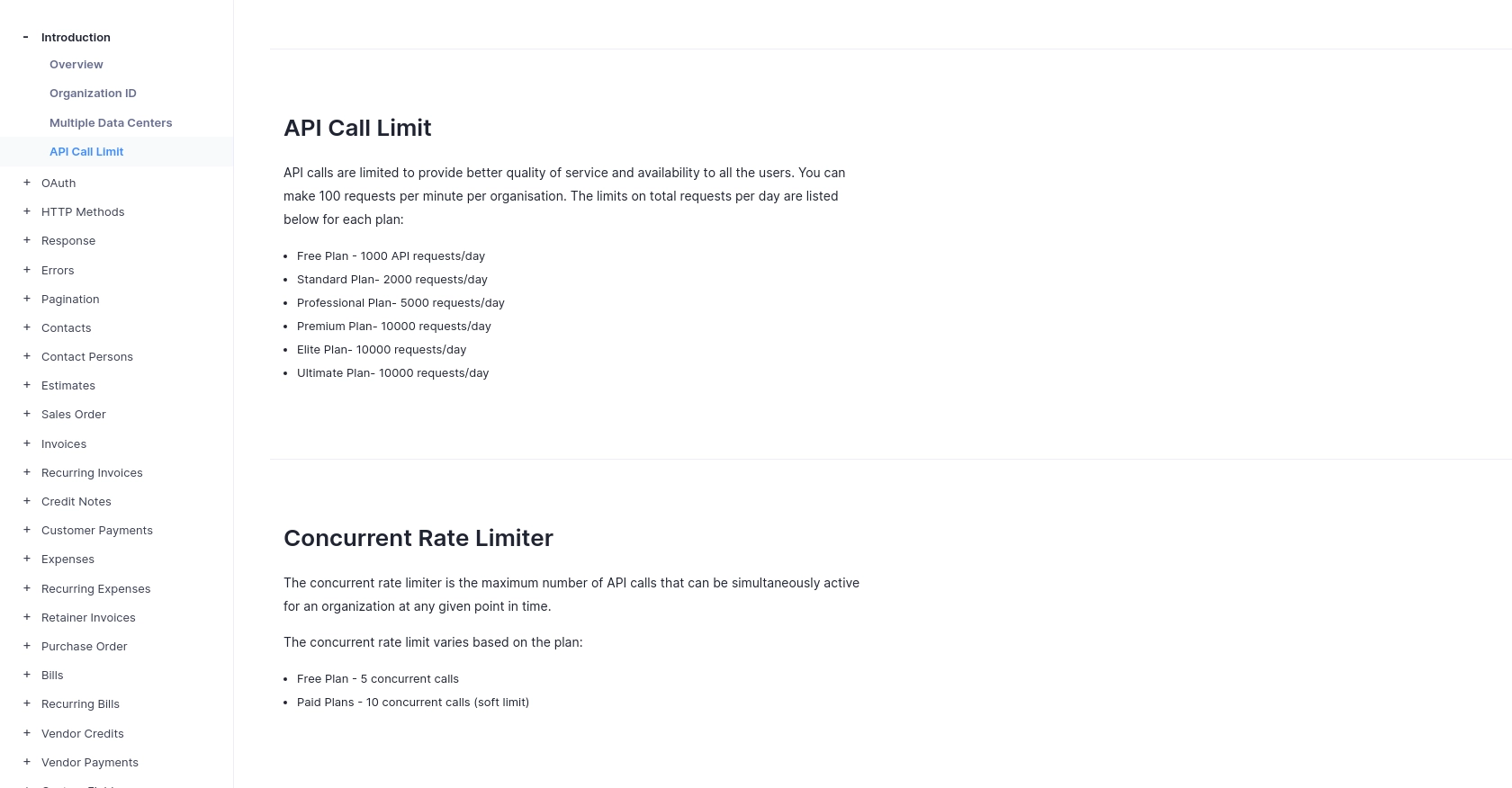
sbb-itb-96038d7
Making API Calls to Retrieve Account Information from Zoho Books Using JavaScript
With your Zoho Books account and OAuth setup complete, you can now proceed to make API calls to retrieve account information. This section will guide you through the process of using JavaScript to interact with the Zoho Books API, specifically focusing on fetching account details.
JavaScript Prerequisites for Zoho Books API Integration
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A text editor or IDE for writing JavaScript code.
- Basic understanding of JavaScript and asynchronous programming.
Installing Required Dependencies for Zoho Books API Calls
To make HTTP requests in JavaScript, you can use the node-fetch
package. Install it using the following command:
npm install node-fetch
Example Code to Fetch Accounts from Zoho Books API
Below is an example of how to retrieve account information from Zoho Books using JavaScript:
const fetch = require('node-fetch');
async function getAccounts() {
const url = 'https://www.zohoapis.com/books/v3/chartofaccounts?organization_id=YOUR_ORGANIZATION_ID';
const options = {
method: 'GET',
headers: {
'Authorization': 'Zoho-oauthtoken YOUR_ACCESS_TOKEN'
}
};
try {
const response = await fetch(url, options);
if (response.ok) {
const data = await response.json();
console.log('Accounts:', data.chartofaccounts);
} else {
console.error('Error:', response.status, response.statusText);
}
} catch (error) {
console.error('Fetch Error:', error);
}
}
getAccounts();
Replace YOUR_ORGANIZATION_ID
and YOUR_ACCESS_TOKEN
with your actual organization ID and access token obtained during the OAuth setup.
Understanding the API Response and Error Handling
The API response will include a list of accounts, each with details such as account name, type, and status. You can verify the success of your request by checking the response code:
- 200 OK: The request was successful, and the accounts data is returned.
- 401 Unauthorized: The access token is invalid or expired. Ensure your token is correct and hasn't expired.
- 429 Rate Limit Exceeded: You've exceeded the API call limit. Refer to the rate limit guidelines in the Zoho Books API documentation.
For more detailed error codes, refer to the Zoho Books API error documentation.
Verifying API Call Success in Zoho Books Dashboard
To verify the success of your API call, log in to your Zoho Books account and navigate to the Chart of Accounts section. Ensure that the accounts listed match the data retrieved through the API call.
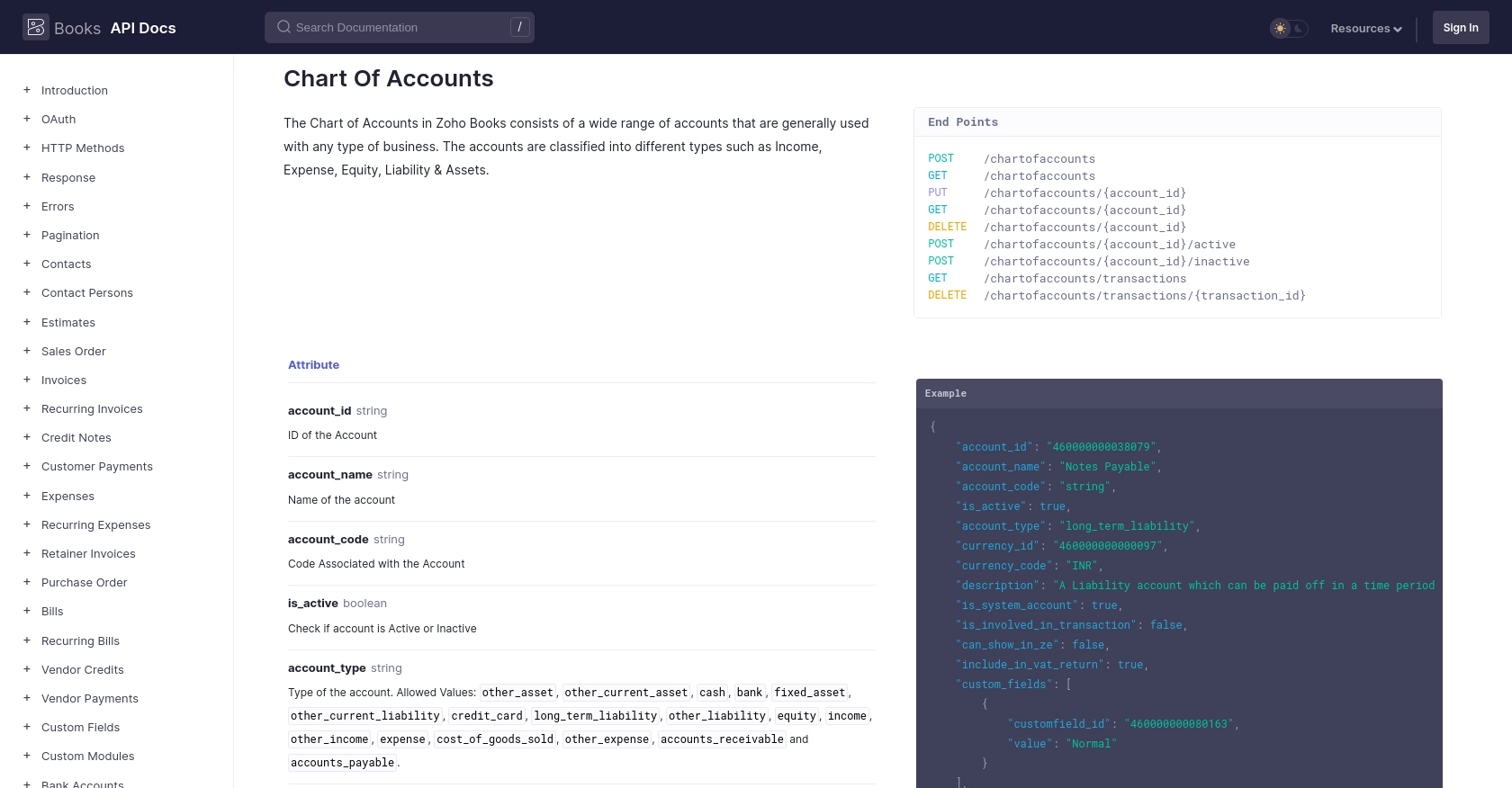
Conclusion and Best Practices for Zoho Books API Integration Using JavaScript
Integrating with the Zoho Books API using JavaScript offers a powerful way to automate and customize your accounting processes. By following the steps outlined in this guide, you can efficiently retrieve and manage account information, streamlining your financial operations.
Best Practices for Secure and Efficient Zoho Books API Usage
- Securely Store Credentials: Always store your OAuth tokens and client credentials securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limiting: Zoho Books API enforces rate limits, allowing 100 requests per minute per organization. Implement retry logic and exponential backoff strategies to handle rate limit errors gracefully. For more details, refer to the API call limit documentation.
- Data Standardization: Ensure that data retrieved from Zoho Books is standardized and transformed as needed for your application. This helps maintain consistency across different systems.
- Error Handling: Implement comprehensive error handling to manage different HTTP status codes. Refer to the error documentation for detailed guidance.
Enhancing Your Integration Experience with Endgrate
While integrating with Zoho Books API can be straightforward, managing multiple integrations across different platforms can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Zoho Books.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product while outsourcing integration tasks to Endgrate.
- Build Once, Use Everywhere: Develop a single integration solution that works across multiple platforms.
- Offer a Seamless Experience: Provide your customers with an intuitive and efficient integration experience.
Explore how Endgrate can enhance your integration capabilities by visiting Endgrate.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/chart-of-accounts/#overview
Ready to get started?