Using the Teamwork CRM API to Create or Update Companies (with PHP examples)
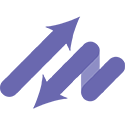
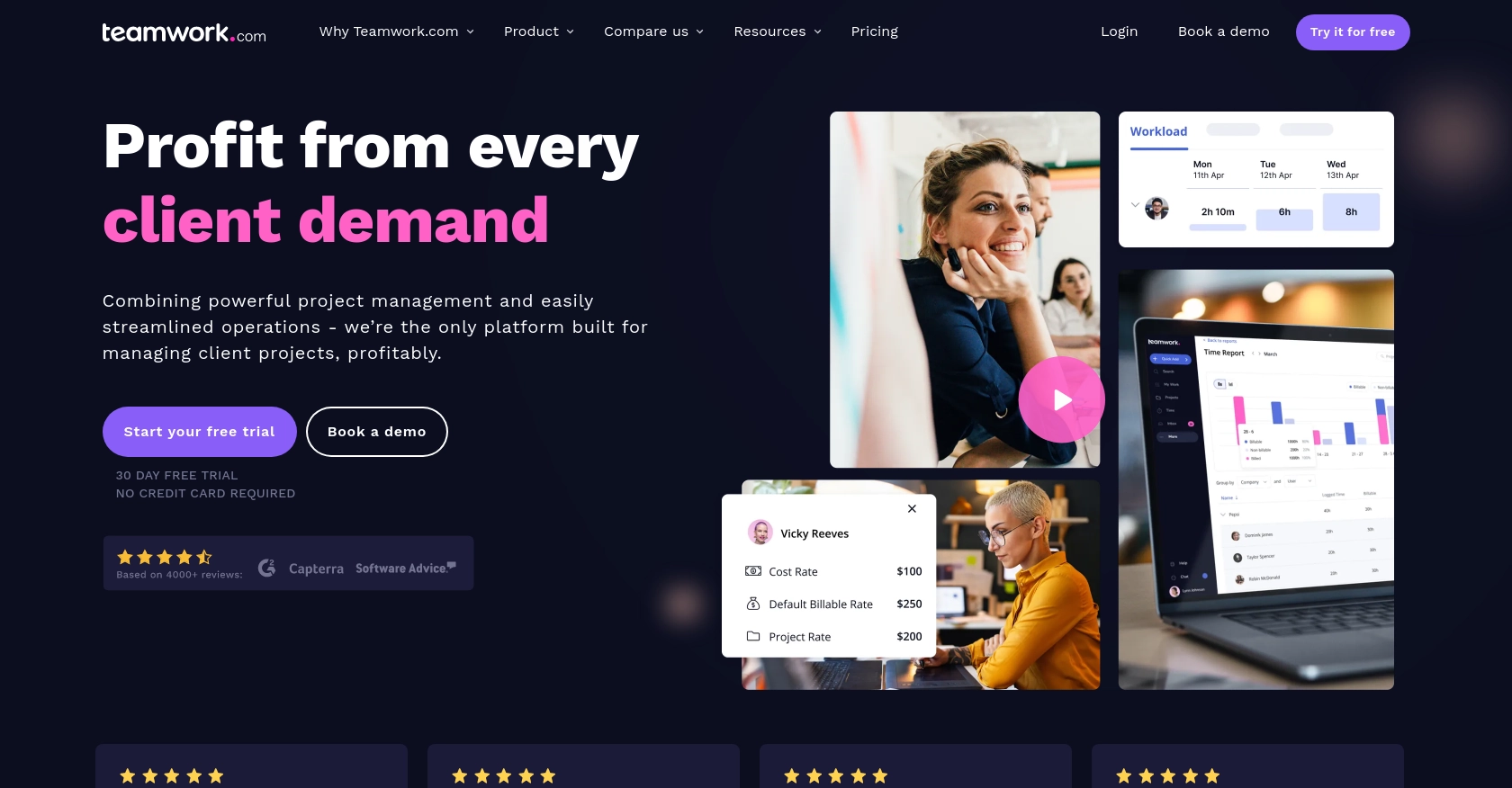
Introduction to Teamwork CRM API Integration
Teamwork CRM is a powerful customer relationship management tool designed to help businesses manage their sales processes more efficiently. With features that support lead tracking, sales forecasting, and customer communication, Teamwork CRM is an essential platform for businesses looking to enhance their customer interactions and drive sales growth.
Integrating with the Teamwork CRM API allows developers to automate and streamline various CRM tasks, such as creating or updating company records. For example, a developer might use the API to automatically update company details in Teamwork CRM whenever there is a change in an external database, ensuring that all customer information is up-to-date and accurate.
Setting Up Your Teamwork CRM Test Account
Before you can start integrating with the Teamwork CRM API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Teamwork CRM Account
If you don't already have a Teamwork CRM account, you can sign up for a free trial on the Teamwork CRM website. Follow the instructions to create your account. Once your account is set up, log in to access the CRM dashboard.
Generating API Credentials for Teamwork CRM
To interact with the Teamwork CRM API, you'll need to generate API credentials. Teamwork CRM supports both Basic Authentication and OAuth 2.0 for secure API access.
- Basic Authentication: Navigate to your account settings and locate the API section. Here, you can generate an API key. Use this key in the Authorization header as a base64-encoded string in the format
username:password
. - OAuth 2.0: Register your app in the Teamwork Developer Portal to obtain a client ID and secret. Implement the App Login Flow to acquire a bearer token, which you'll include in the Authorization header for API requests.
For more details on authentication, refer to the Teamwork CRM Authentication Documentation.
Configuring Your Teamwork CRM Sandbox Environment
Once your account and API credentials are ready, you can configure your sandbox environment. This involves setting up test data and ensuring that your API calls are directed to the sandbox rather than the production environment.
Ensure that your API requests are correctly authenticated and that you have the necessary permissions to create or update company records within the sandbox. This setup will allow you to test your integration thoroughly before deploying it to a live environment.
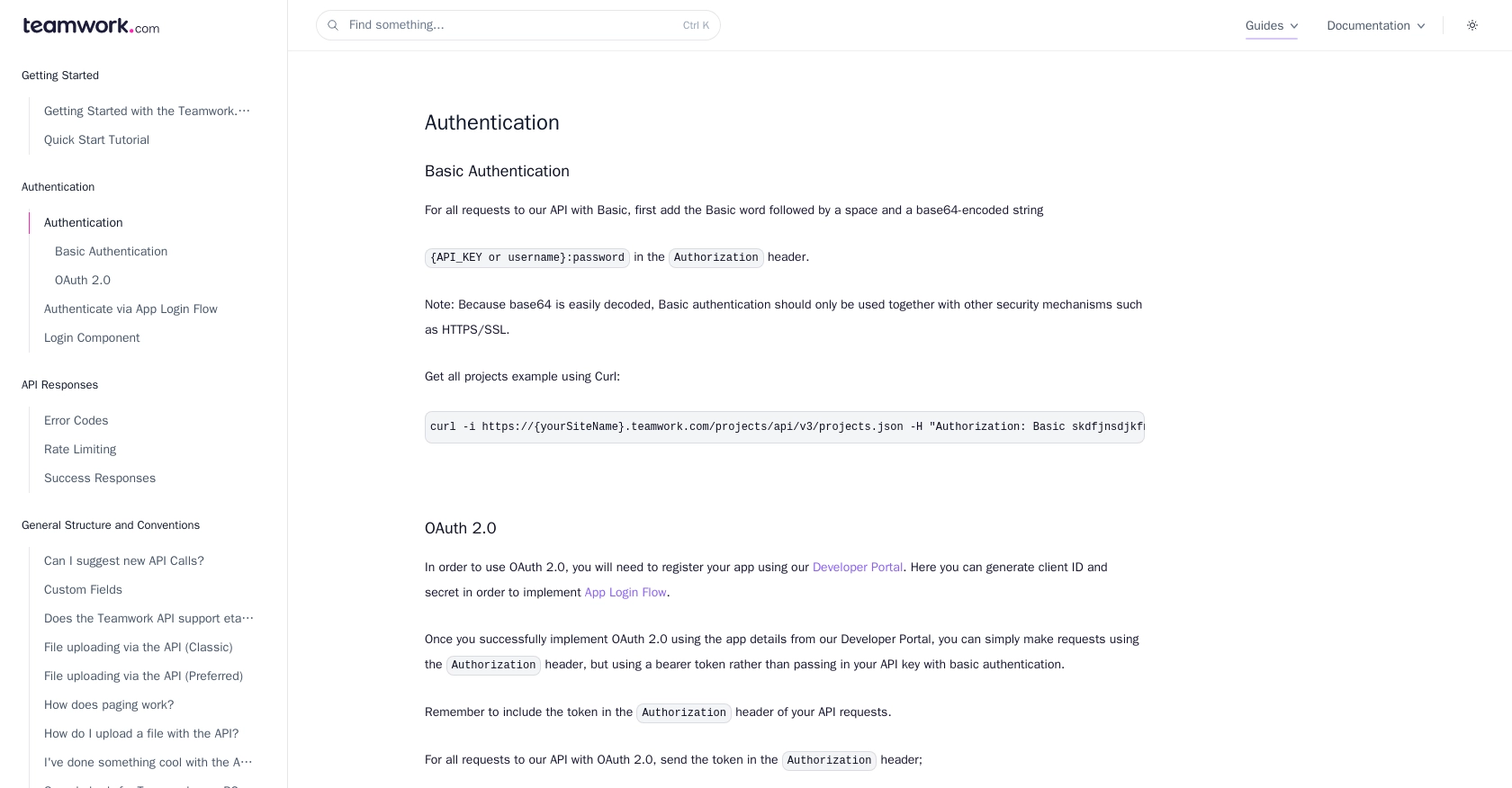
sbb-itb-96038d7
Making API Calls to Teamwork CRM with PHP
To interact with the Teamwork CRM API using PHP, you'll need to set up your development environment and write code to create or update company records. This section will guide you through the process, including setting up PHP, installing necessary dependencies, and writing the code to make API calls.
Setting Up Your PHP Environment for Teamwork CRM API Integration
Before you start coding, ensure that you have PHP installed on your machine. You can download the latest version of PHP from the official PHP website. Additionally, you'll need Composer, a dependency manager for PHP, which you can install by following the instructions on the Composer website.
Installing Required PHP Libraries
To make HTTP requests to the Teamwork CRM API, you'll need the Guzzle HTTP client. Install it using Composer with the following command:
composer require guzzlehttp/guzzle
Creating or Updating Companies in Teamwork CRM Using PHP
With your environment set up, you can now write PHP code to create or update company records in Teamwork CRM. Below is an example script demonstrating how to perform these actions using the API:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiKey = 'your_api_key';
$baseUri = 'https://yourSiteName.teamwork.com/crm/v2/companies';
$headers = [
'Authorization' => 'Basic ' . base64_encode($apiKey . ':'),
'Content-Type' => 'application/json'
];
// Data for creating or updating a company
$companyData = [
'name' => 'Example Company',
'address' => '123 Example Street',
'phone' => '123-456-7890'
];
try {
$response = $client->post($baseUri, [
'headers' => $headers,
'json' => $companyData
]);
if ($response->getStatusCode() == 201) {
echo "Company created successfully.";
} else {
echo "Company updated successfully.";
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace your_api_key
and yourSiteName
with your actual API key and site name. This script uses Guzzle to send a POST request to the Teamwork CRM API, creating or updating a company record with the specified data.
Verifying API Call Success in Teamwork CRM
After running the script, you can verify the success of the API call by checking the Teamwork CRM dashboard. The new or updated company should appear in your sandbox environment. If the API call fails, ensure that your API key is correct and that you have the necessary permissions.
Handling Errors and Understanding Teamwork CRM API Error Codes
When making API calls, it's crucial to handle potential errors. The Teamwork CRM API may return various error codes, such as:
- 401 Unauthorized: Check your API key and ensure it's correctly set up.
- 422 Unprocessable Entity: Ensure the JSON/XML is correctly formatted.
- 429 Too Many Requests: You have exceeded the rate limit. Wait and try again later.
For more details on error codes, refer to the Teamwork CRM Error Codes Documentation.
Best Practices for Teamwork CRM API Integration
When integrating with the Teamwork CRM API, it's essential to follow best practices to ensure a secure and efficient implementation. Here are some key considerations:
- Secure Storage of API Credentials: Always store your API keys and OAuth tokens securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handling Rate Limits: Be mindful of the API's rate limits. Teamwork CRM allows up to 150 requests per minute for most plans. Implement logic to handle HTTP 429 errors and retry requests after the rate limit resets. For more details, refer to the Teamwork CRM Rate Limiting Documentation.
- Data Transformation and Standardization: Ensure that data sent to and received from the API is properly formatted and standardized. This helps maintain consistency across different systems and improves data quality.
Leveraging Endgrate for Seamless Teamwork CRM Integrations
Integrating multiple platforms can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Teamwork CRM. By using Endgrate, you can:
- Save time and resources by outsourcing integration tasks and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations, reducing redundancy and maintenance efforts.
- Offer an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can streamline your integration processes by visiting Endgrate's website and discover the benefits of a unified API solution.
Read More
Ready to get started?