Using the Typeform API to Get Questions (with Javascript examples)
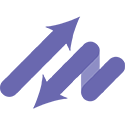
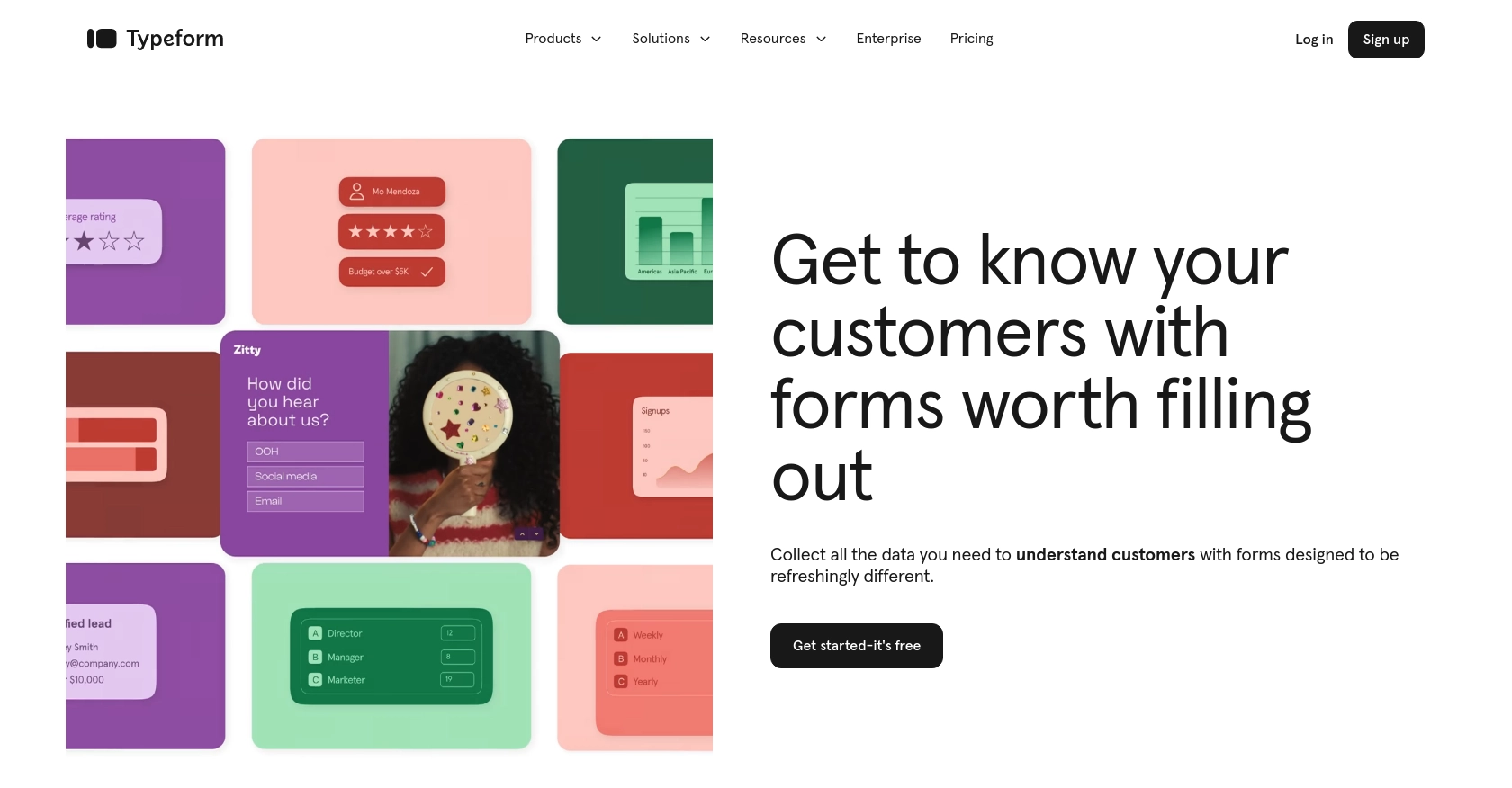
Introduction to Typeform API Integration
Typeform is a versatile online form builder that allows businesses to create engaging and interactive forms, surveys, and quizzes. Its user-friendly interface and customizable design options make it a popular choice for collecting data and feedback from users.
Integrating with Typeform's API enables developers to automate and enhance data collection processes. For example, you can use the Typeform API to retrieve questions from a form and dynamically display them in your application, providing a seamless user experience.
This article will guide you through using JavaScript to interact with the Typeform API, focusing on retrieving questions from a form. By the end of this tutorial, you'll be equipped to efficiently access and manage form data using Typeform's robust API.
Setting Up Your Typeform Developer Account for API Integration
Before you can start interacting with the Typeform API, you need to set up a developer account and create an application. This will allow you to generate the necessary credentials for OAuth 2.0 authentication, which is required to access Typeform's API endpoints.
Creating a Typeform Developer Account
If you don't already have a Typeform account, you can sign up for a free account on the Typeform website. Once your account is created, follow these steps to set up your developer environment:
- Log in to your Typeform account.
- In the upper-left corner, click the icon drop-down menu next to your organization name.
- Under the Organization section, click Developer Apps.
- Click Register a new app.
- Fill in the App Name and App website fields with the appropriate information.
- Enter the Redirect URI(s) for your application. This is where users will be redirected after they log in and grant access.
- Click Register app to complete the process.
Generating OAuth 2.0 Credentials for Typeform API Access
After registering your application, you'll receive the client_id
and client_secret
needed for OAuth 2.0 authentication. These credentials are crucial for generating access tokens to interact with the Typeform API.
- Once your app is registered, the
client_secret
will be displayed only once. Make sure to copy and store it securely. - You can view your
client_id
anytime in the Developer Apps panel. - If you lose your
client_secret
, you can regenerate it by clicking the Regenerate client secret button in the Edit app panel.
For more details on setting up OAuth 2.0, refer to the Typeform Applications Documentation and OAuth 2.0 Scopes Documentation.
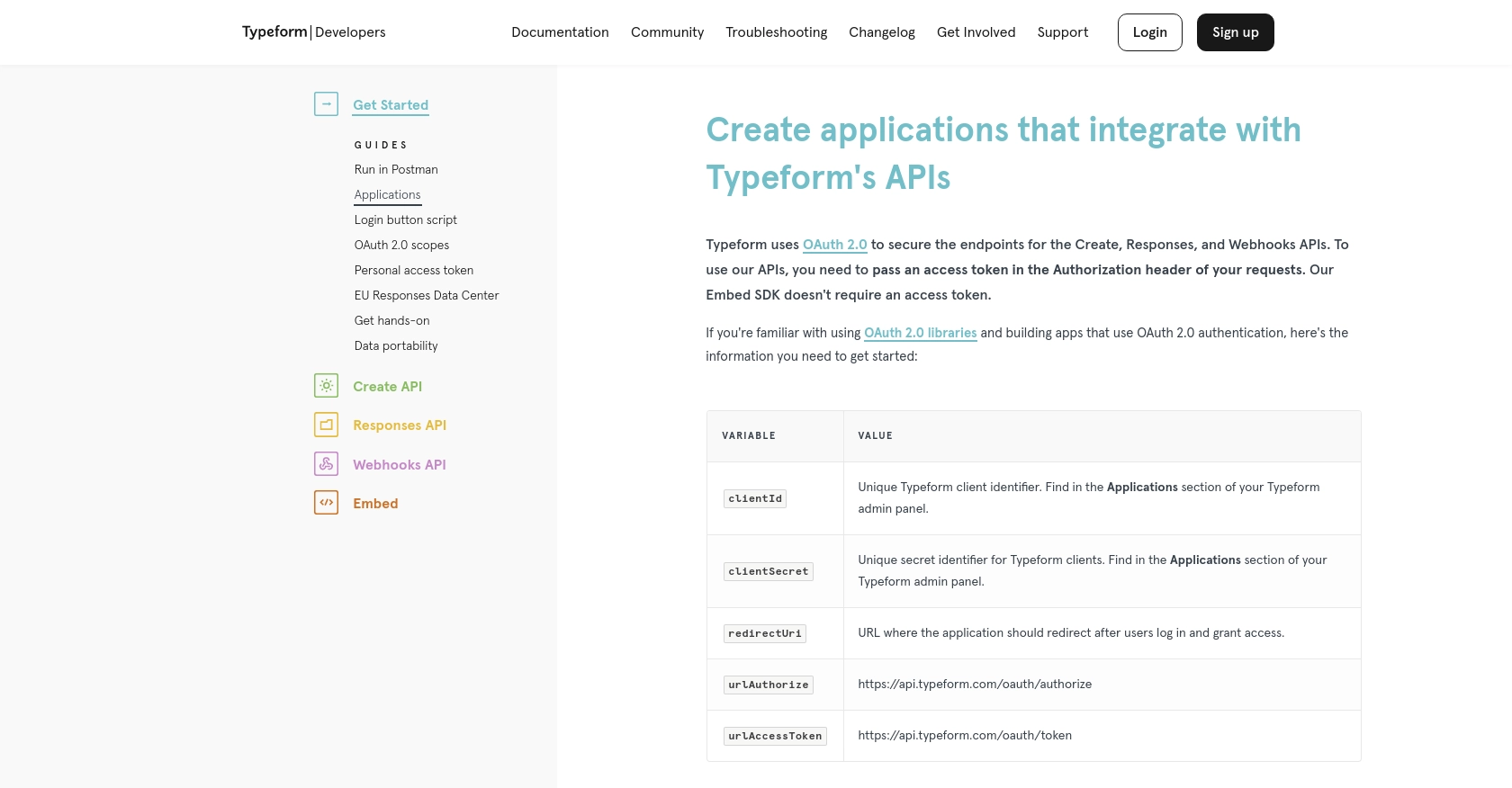
sbb-itb-96038d7
Executing API Calls to Retrieve Typeform Questions Using JavaScript
To interact with the Typeform API and retrieve questions from a form, you'll need to make authenticated API calls using JavaScript. This section will guide you through the process, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for Typeform API Integration
Before making API calls, ensure you have a working JavaScript environment. You can use Node.js or a browser-based setup. For this tutorial, we'll focus on a Node.js environment.
- Ensure Node.js is installed on your machine. You can download it from the official Node.js website.
- Create a new project directory and navigate to it in your terminal.
- Initialize a new Node.js project by running
npm init -y
. - Install the Axios library for making HTTP requests by running
npm install axios
.
Writing JavaScript Code to Retrieve Questions from a Typeform
With your environment set up, you can now write the code to retrieve questions from a Typeform. We'll use Axios to handle the HTTP requests.
const axios = require('axios');
// Replace with your actual access token
const accessToken = 'YOUR_ACCESS_TOKEN';
// Replace with your actual form ID
const formId = 'YOUR_FORM_ID';
// Set the API endpoint
const endpoint = `https://api.typeform.com/forms/${formId}`;
// Configure the request headers
const headers = {
'Authorization': `Bearer ${accessToken}`
};
// Function to get questions from a Typeform
async function getTypeformQuestions() {
try {
const response = await axios.get(endpoint, { headers });
const questions = response.data.fields;
console.log('Questions:', questions);
} catch (error) {
console.error('Error retrieving questions:', error.response ? error.response.data : error.message);
}
}
// Execute the function
getTypeformQuestions();
In this code, replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth 2.0 setup, and YOUR_FORM_ID
with the ID of the form you want to retrieve questions from.
Verifying Successful API Requests and Handling Errors
After running the script, you should see the questions from your Typeform printed in the console. If the request is successful, the response will contain the form's questions, which you can use in your application.
To verify the request's success, check the console output for the list of questions. If there are any issues, the error handling in the code will log the error details, helping you diagnose the problem.
Common errors include invalid access tokens or incorrect form IDs. Ensure your credentials are correct and that the form ID matches the one in your Typeform account. For more detailed error information, refer to the Typeform API Documentation.
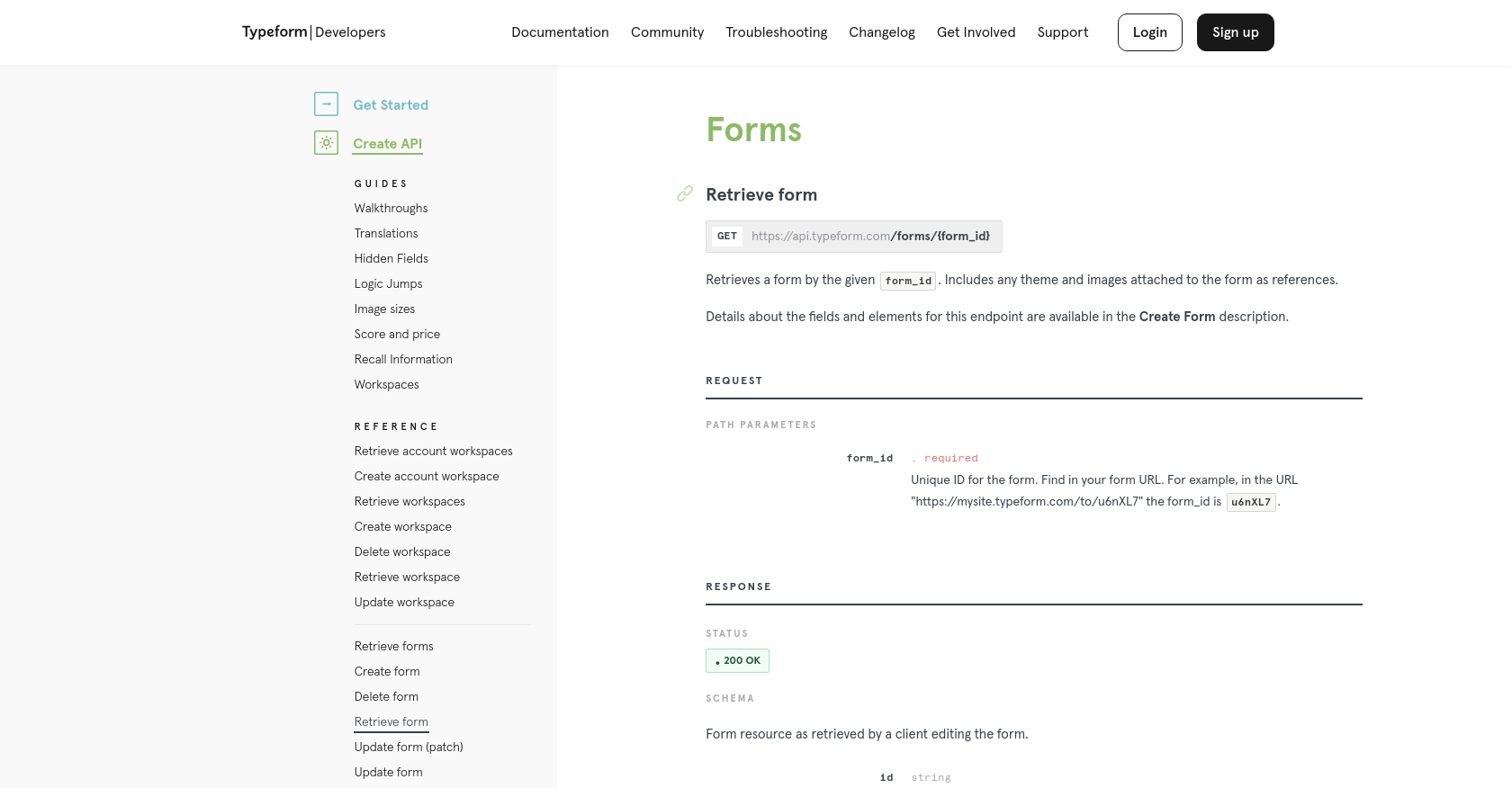
Conclusion and Best Practices for Typeform API Integration
Integrating with the Typeform API using JavaScript offers a powerful way to enhance your application's data collection capabilities. By retrieving questions dynamically, you can create a seamless and interactive user experience that aligns with your application's needs.
Here are some best practices to consider when working with the Typeform API:
- Secure Your Credentials: Always store your
client_id
,client_secret
, and access tokens securely. Avoid hardcoding them in your source code and use environment variables or secure vaults instead. - Handle Rate Limiting: Be aware of Typeform's API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation: Consider transforming and standardizing the data fields you retrieve from Typeform to fit your application's data model. This will ensure consistency and ease of use.
- Error Handling: Implement robust error handling to manage API call failures. Log errors for debugging and provide user-friendly messages to enhance the user experience.
By following these practices, you can ensure a secure and efficient integration with Typeform's API, allowing you to focus on delivering value to your users.
For developers looking to streamline their integration processes further, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers. Learn more at Endgrate.
Read More
Ready to get started?