Using the Outreach API to Create or Update Accounts in PHP
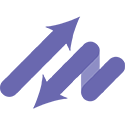
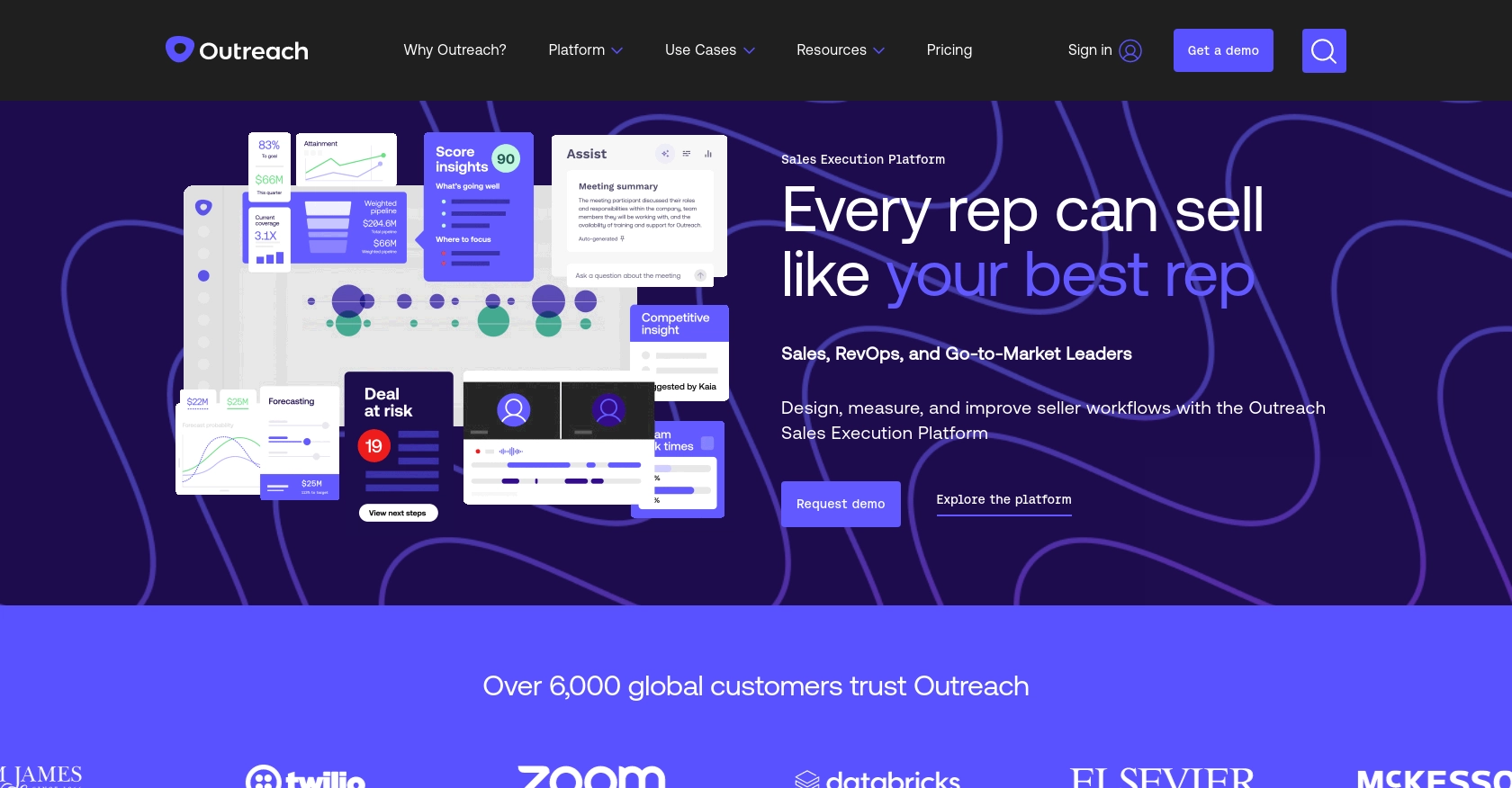
Introduction to Outreach API for Account Management
Outreach is a powerful sales engagement platform that enables businesses to streamline their sales processes and improve productivity. It offers a comprehensive suite of tools designed to enhance communication, automate workflows, and provide valuable insights into sales activities.
For developers, integrating with the Outreach API can significantly enhance the ability to manage account data efficiently. By leveraging the API, developers can automate the creation and updating of account information, ensuring that sales teams have access to the most current data. This can be particularly useful in scenarios where account details need to be synchronized across multiple systems or when integrating Outreach with other business applications.
In this article, we will explore how to use PHP to interact with the Outreach API, focusing on creating and updating account records. This integration can help developers automate account management tasks, allowing sales teams to focus on engaging with prospects and closing deals.
Setting Up Your Outreach Test/Sandbox Account for API Integration
Before diving into the integration process, it's essential to set up a test or sandbox account with Outreach. This environment allows developers to experiment with API calls without affecting live data, ensuring a smooth development process.
Creating an Outreach Sandbox Account
If you don't already have an Outreach account, you can sign up for a free trial or request access to a sandbox environment through the Outreach website. This will provide you with the necessary credentials to begin testing API interactions.
- Visit the Outreach website and navigate to the sign-up page.
- Follow the instructions to create your account, ensuring you select the option for a sandbox or trial environment if available.
- Once your account is set up, log in to access the Outreach dashboard.
Configuring OAuth for API Access
Outreach uses OAuth 2.0 for authentication, which requires setting up an application within your sandbox account. This process will provide you with the client ID and client secret needed for API authorization.
- Log in to your Outreach account and navigate to the "API Access" section under settings.
- Create a new application by providing the necessary details such as application name and description.
- Specify one or more redirect URIs that Outreach will use to send authorization codes after user consent.
- Select the OAuth scopes required for your application, ensuring you include permissions for account management.
- Save the application settings to generate your client ID and client secret. Note that the client secret will only be displayed once, so store it securely.
Obtaining an Access Token
With your application configured, you can now obtain an access token to authenticate API requests. Follow these steps to complete the OAuth flow:
- Redirect users to the following URL for authorization, replacing placeholders with your client ID and redirect URI:
- After user consent, Outreach will redirect to your specified URI with an authorization code.
- Exchange this code for an access token by making a POST request to the token endpoint:
- Store the access token securely, as it will be used to authenticate your API requests.
https://api.outreach.io/oauth/authorize?client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&response_type=code&scope=YOUR_SCOPES
curl https://api.outreach.io/oauth/token \
-X POST \
-d client_id=YOUR_CLIENT_ID \
-d client_secret=YOUR_CLIENT_SECRET \
-d redirect_uri=YOUR_REDIRECT_URI \
-d grant_type=authorization_code \
-d code=AUTHORIZATION_CODE
With your sandbox account and OAuth credentials set up, you're ready to start integrating with the Outreach API using PHP. This setup ensures a secure and efficient development process, allowing you to focus on building robust integrations.
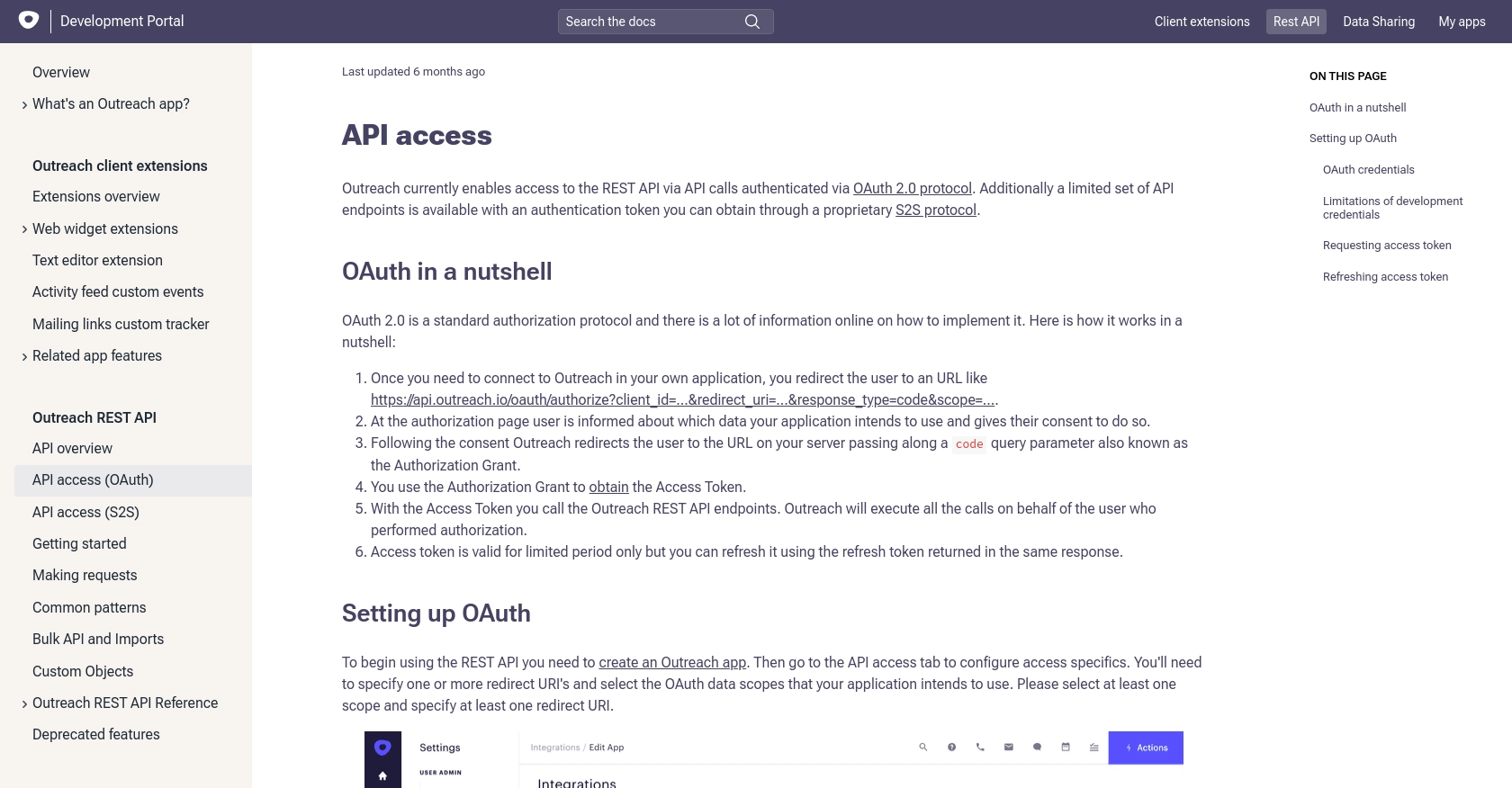
sbb-itb-96038d7
Making API Calls to Create or Update Accounts with Outreach API in PHP
To interact with the Outreach API for creating or updating accounts, you'll need to use PHP to make HTTP requests. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code to make API calls, and handling responses.
Setting Up Your PHP Environment for Outreach API Integration
Before you begin coding, ensure that your PHP environment is correctly set up. You'll need PHP version 7.4 or later and the cURL extension enabled to make HTTP requests.
- Verify your PHP version by running
php -v
in your terminal. - Ensure the cURL extension is enabled by checking your
php.ini
file or runningphp -m
to list installed modules.
Installing Required PHP Dependencies
To simplify HTTP requests, you can use the Guzzle library. Install it using Composer:
composer require guzzlehttp/guzzle
Writing PHP Code to Create an Account with Outreach API
With your environment set up, you can now write the PHP code to create an account in Outreach. Here's a step-by-step guide:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$response = $client->post('https://api.outreach.io/api/v2/accounts', [
'headers' => [
'Authorization' => 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type' => 'application/vnd.api+json',
],
'json' => [
'data' => [
'type' => 'account',
'attributes' => [
'name' => 'New Account Name',
'domain' => 'example.com',
'industry' => 'Technology',
],
],
],
]);
if ($response->getStatusCode() == 201) {
echo "Account created successfully.";
} else {
echo "Failed to create account.";
}
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth flow. This code sends a POST request to the Outreach API to create a new account with specified attributes.
Handling API Responses and Errors
It's crucial to handle API responses and potential errors gracefully. The Outreach API returns various status codes that you should check to ensure successful operations.
- 201 Created: The account was successfully created.
- 422 Unprocessable Entity: There was an issue with the data provided. Check the response body for details.
- 429 Too Many Requests: You've exceeded the rate limit of 10,000 requests per hour. Wait for the reset period before retrying.
Always log errors and handle exceptions to maintain a robust integration.
Updating an Existing Account with Outreach API
To update an existing account, you'll need to send a PATCH request with the account ID and the updated attributes. Here's an example:
$response = $client->patch('https://api.outreach.io/api/v2/accounts/{account_id}', [
'headers' => [
'Authorization' => 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type' => 'application/vnd.api+json',
],
'json' => [
'data' => [
'type' => 'account',
'id' => '{account_id}',
'attributes' => [
'name' => 'Updated Account Name',
'industry' => 'Updated Industry',
],
],
],
]);
if ($response->getStatusCode() == 200) {
echo "Account updated successfully.";
} else {
echo "Failed to update account.";
}
Replace {account_id}
with the actual ID of the account you wish to update. This code demonstrates how to modify account details using the Outreach API.
By following these steps, you can efficiently create and update accounts in Outreach using PHP, streamlining your sales processes and ensuring data consistency across platforms.
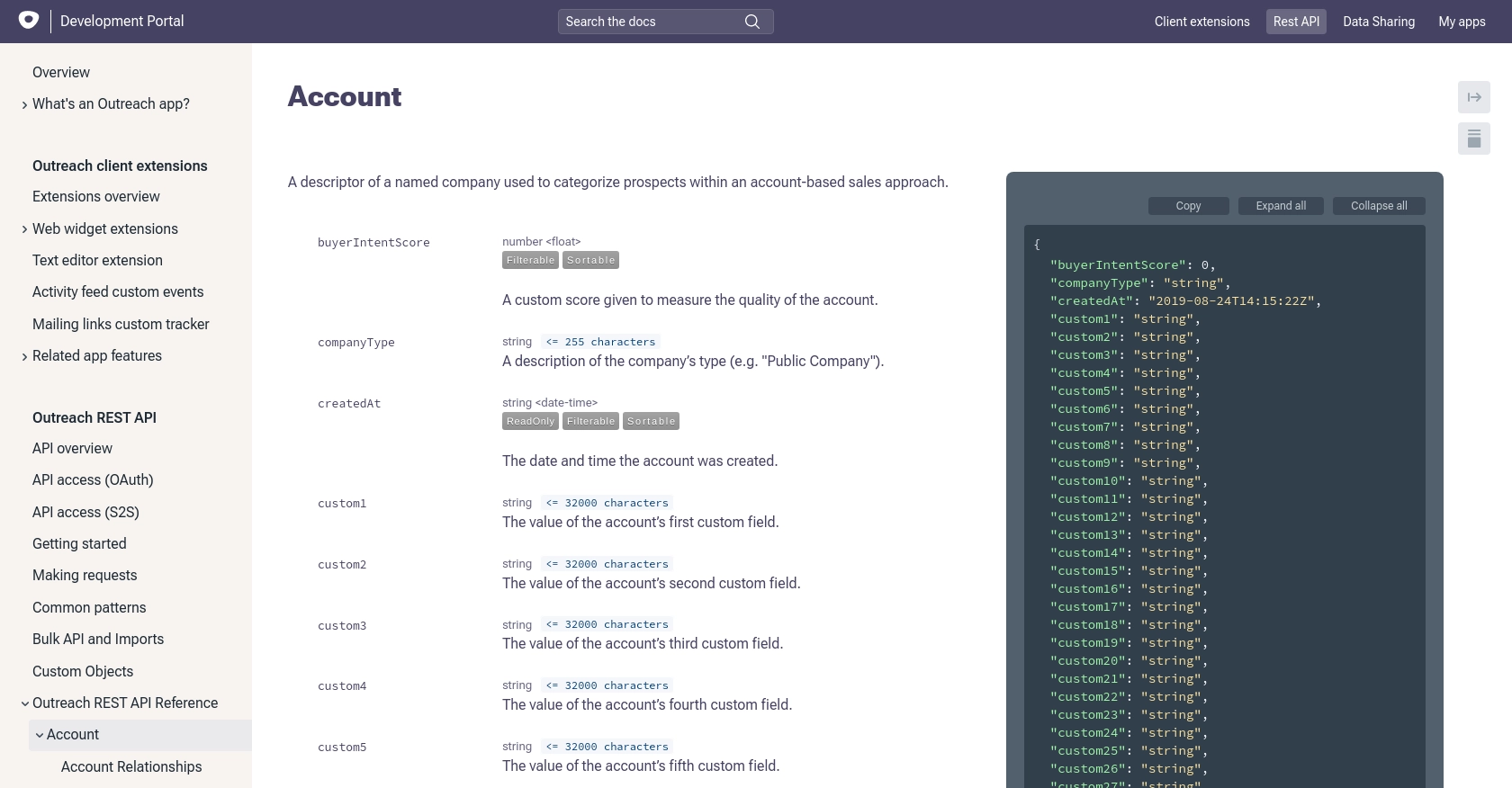
Conclusion and Best Practices for Using Outreach API with PHP
Integrating with the Outreach API using PHP provides a powerful way to automate account management tasks, ensuring that your sales team has access to the most up-to-date information. By following the steps outlined in this guide, you can efficiently create and update accounts, streamlining your sales processes and enhancing productivity.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth credentials, including client secrets and access tokens, securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handle Rate Limiting: Be mindful of the Outreach API's rate limit of 10,000 requests per hour. Implement logic to handle 429 status codes and retry requests after the reset period.
- Use Refresh Tokens: Access tokens are short-lived, so ensure you use refresh tokens to obtain new access tokens as needed. This will help maintain a seamless integration experience.
- Log and Monitor API Calls: Implement logging for all API interactions to help diagnose issues and monitor usage patterns. This can assist in troubleshooting and optimizing your integration.
- Validate API Responses: Always check API responses for success or error codes and handle them appropriately. This ensures robust error handling and improves the reliability of your integration.
Enhance Your Integration Strategy with Endgrate
While integrating with the Outreach API directly offers many benefits, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Outreach.
By leveraging Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an easy, intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration strategy by visiting Endgrate today.
Read More
Ready to get started?