Using the Salesflare API to Get Contacts in PHP
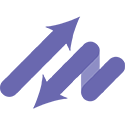
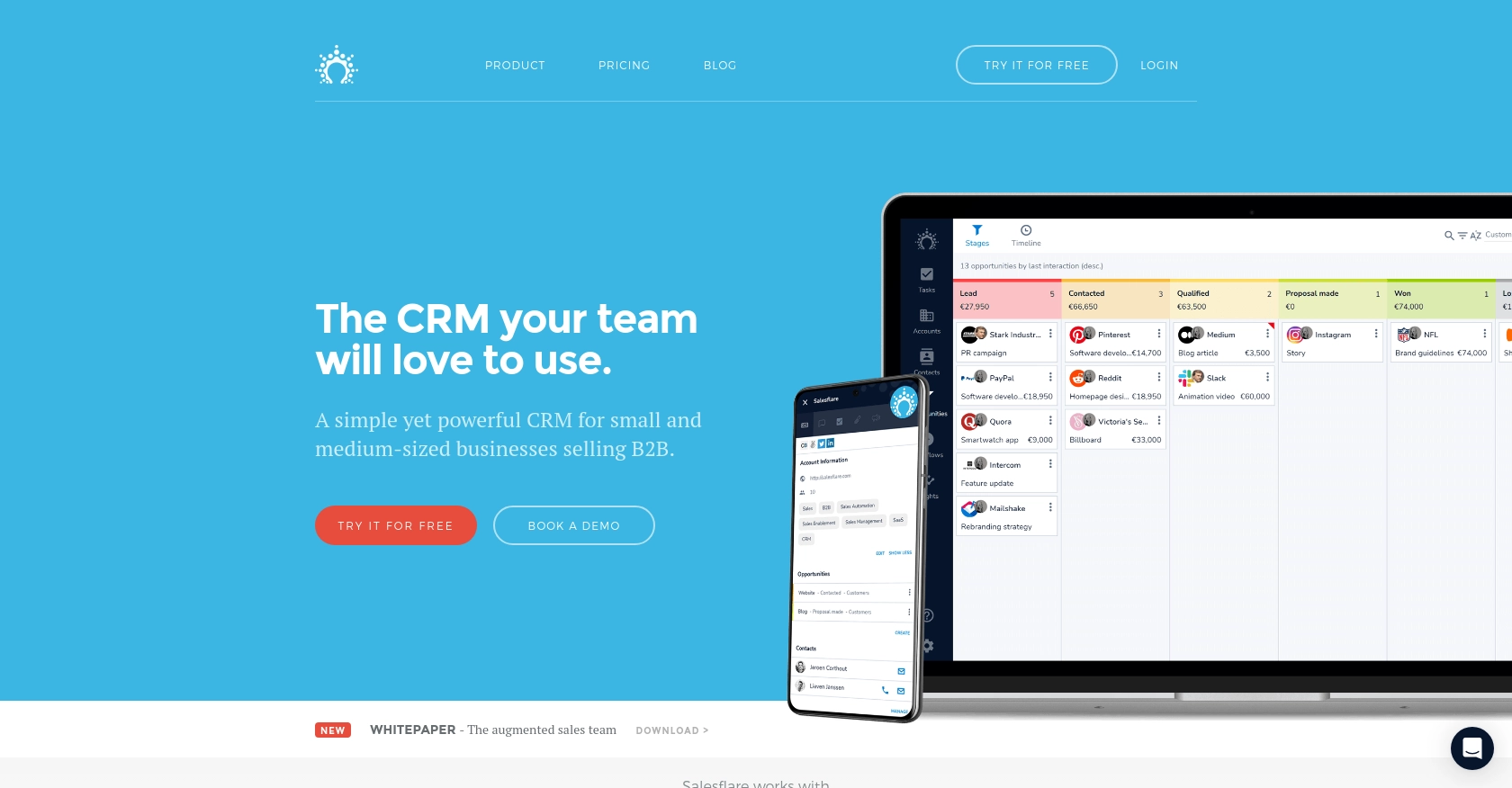
Introduction to Salesflare API Integration
Salesflare is a powerful CRM platform designed to simplify the sales process for small and medium-sized businesses. It offers a range of features that help sales teams manage leads, track customer interactions, and automate repetitive tasks, all within an intuitive interface.
Integrating with Salesflare's API allows developers to access and manipulate customer data programmatically, enhancing the efficiency of sales operations. For example, a developer might use the Salesflare API to retrieve contact information and integrate it with other business tools, enabling seamless data synchronization and improved customer insights.
Setting Up Your Salesflare Test Account
Before you can start using the Salesflare API to retrieve contacts, you'll need to set up a test account. Salesflare offers a straightforward process to get started, allowing developers to explore its features and functionalities.
Creating a Salesflare Account
If you don't already have a Salesflare account, you can sign up for a free trial on the Salesflare website. This trial will give you access to the platform's features, enabling you to test API interactions without any initial cost.
- Visit the Salesflare website.
- Click on the "Try for Free" button.
- Follow the on-screen instructions to create your account.
Generating Your Salesflare API Key
Salesflare uses API key-based authentication to secure API requests. Follow these steps to generate your API key:
- Log in to your Salesflare account.
- Navigate to the "Settings" section from the dashboard.
- Find the "API Keys" tab and click on it.
- Click on "Generate New API Key" and provide a name for your key.
- Copy the generated API key and store it securely, as you'll need it to authenticate your API requests.
For more detailed information, refer to the Salesflare API documentation.
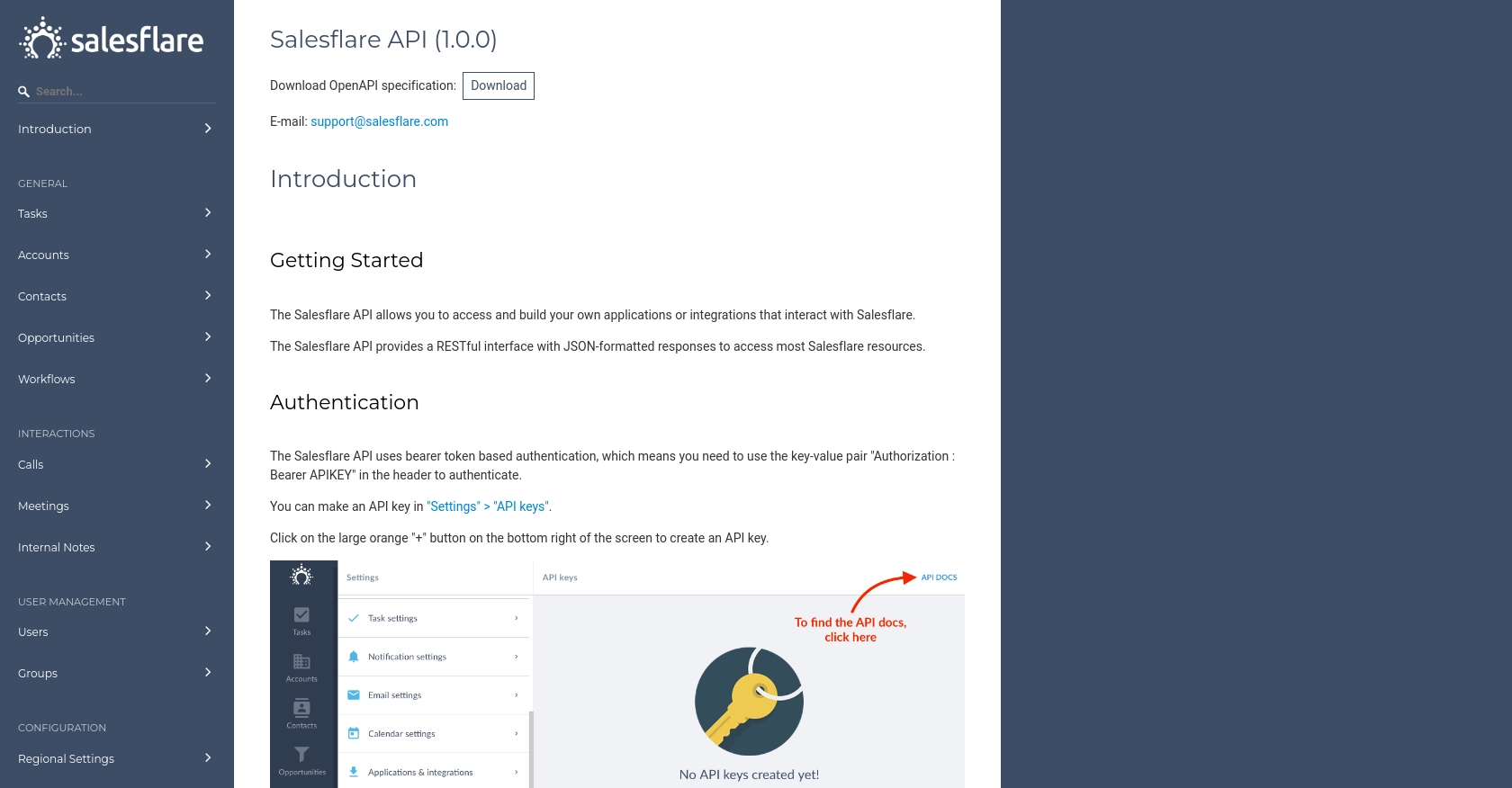
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Salesflare Using PHP
To interact with the Salesflare API and retrieve contact information, you'll need to use PHP, a popular server-side scripting language known for its ease of use and flexibility. This section will guide you through setting up your PHP environment, making the API call, and handling the response.
Setting Up Your PHP Environment for Salesflare API Integration
Before making API calls, ensure your development environment is ready. You'll need:
- PHP 7.4 or later
- Composer, the PHP package manager
Begin by installing the Guzzle HTTP client, which simplifies making HTTP requests in PHP. Run the following command in your terminal:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Contacts from Salesflare
With your environment set up, you can now write the PHP code to interact with the Salesflare API. Create a file named get_salesflare_contacts.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiKey = 'Your_Salesflare_API_Key'; // Replace with your actual API key
try {
$response = $client->request('GET', 'https://api.salesflare.com/v1/contacts', [
'headers' => [
'Authorization' => 'Bearer ' . $apiKey,
'Accept' => 'application/json',
]
]);
$contacts = json_decode($response->getBody(), true);
foreach ($contacts as $contact) {
echo 'Name: ' . $contact['name'] . '<br>';
echo 'Email: ' . $contact['email'] . '<br><br>';
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace Your_Salesflare_API_Key
with the API key you generated earlier. This script uses Guzzle to send a GET request to the Salesflare API endpoint for contacts. It then decodes the JSON response and prints each contact's name and email.
Verifying the API Request and Handling Errors
After running the script, you should see a list of contacts displayed. If the request is successful, the contacts will match those in your Salesflare account. If there are errors, ensure your API key is correct and check for any network issues.
Common error codes include:
- 401 Unauthorized: Check your API key and authentication headers.
- 404 Not Found: Verify the endpoint URL.
- 500 Internal Server Error: Try again later or contact Salesflare support.
For more details, refer to the Salesflare API documentation.
Conclusion and Best Practices for Using Salesflare API with PHP
Integrating with the Salesflare API using PHP can significantly enhance your ability to manage customer data efficiently. By following the steps outlined in this guide, you can seamlessly retrieve contact information and integrate it with other business tools, streamlining your sales operations.
Best Practices for Secure and Efficient API Integration
- Secure Storage of API Keys: Always store your API keys securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the Salesflare API to avoid service disruptions. Implement retry logic with exponential backoff if necessary.
- Data Transformation and Standardization: Ensure that the data retrieved from Salesflare is transformed and standardized to fit your application's requirements, maintaining consistency across your systems.
Enhance Your Integration Strategy with Endgrate
While integrating with individual APIs like Salesflare can be beneficial, managing multiple integrations can become complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Salesflare. This allows you to focus on your core product while outsourcing the intricacies of integration management.
Explore how Endgrate can streamline your integration efforts and provide an intuitive experience for your customers by visiting Endgrate. Save time and resources by building once for each use case, rather than multiple times for different integrations.
Read More
Ready to get started?