Using the Pipedrive API to Create or Update Organizations in PHP
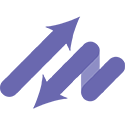
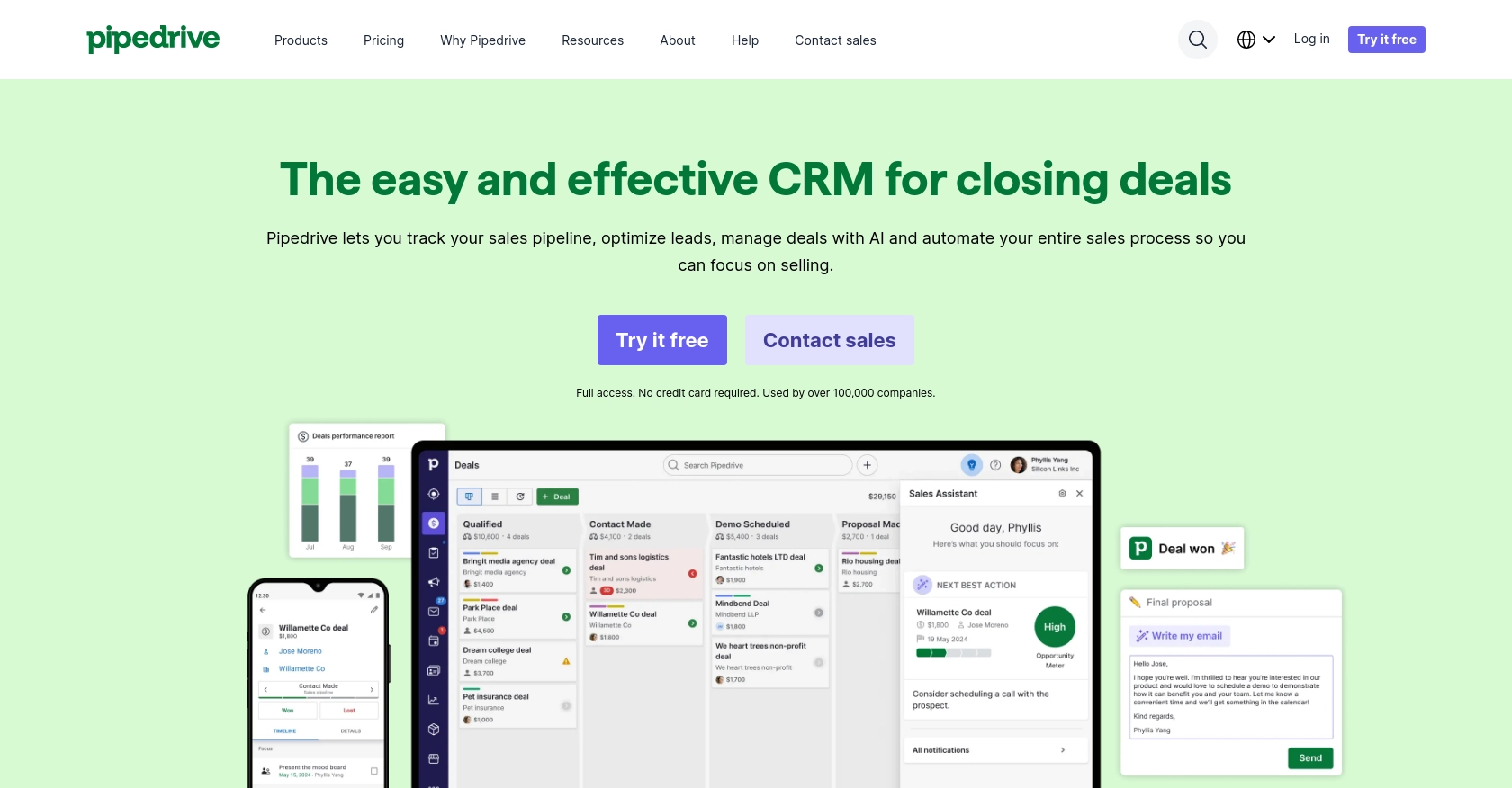
Introduction to Pipedrive CRM
Pipedrive is a powerful sales CRM platform designed to help businesses manage their sales processes more effectively. Known for its intuitive interface and robust features, Pipedrive allows sales teams to track leads, manage customer relationships, and optimize sales workflows.
Developers often seek to integrate with Pipedrive's API to automate and enhance sales operations. For example, using the Pipedrive API, a developer can create or update organization records directly from a custom application, ensuring that sales data is always up-to-date and accessible.
This article will guide you through using PHP to interact with the Pipedrive API, focusing on creating or updating organizations. By following this tutorial, you'll learn how to streamline your sales data management and improve your team's efficiency.
Setting Up Your Pipedrive Developer Sandbox Account
Before you can start integrating with the Pipedrive API, you need to set up a developer sandbox account. This account provides a risk-free environment to test and develop your applications without affecting live data.
Steps to Create a Pipedrive Developer Sandbox Account
- Visit the Pipedrive Developer Sandbox page.
- Fill out the form to request access to a sandbox account. This account is similar to a regular Pipedrive company account but is limited to five seats.
- Once your sandbox account is set up, you can import sample data to familiarize yourself with Pipedrive's features. Go to “...” (More) > Import data > From a spreadsheet in the Pipedrive web app to import template spreadsheets.
Creating a Pipedrive App for OAuth Authentication
To interact with the Pipedrive API, you'll need to create an app that uses OAuth 2.0 for authentication. Follow these steps to create your app:
- Log in to your Pipedrive sandbox account and navigate to the Developer Hub.
- Register your app by providing necessary details such as the app name and description.
- Obtain your Client ID and Client Secret, which are essential for implementing the OAuth flow.
- Ensure your app has the proper scopes and permissions to access the necessary data. Choose scopes that are essential for your integration needs.
With your sandbox account and app set up, you're ready to start making API calls to Pipedrive. In the next section, we'll guide you through creating or updating organizations using PHP.
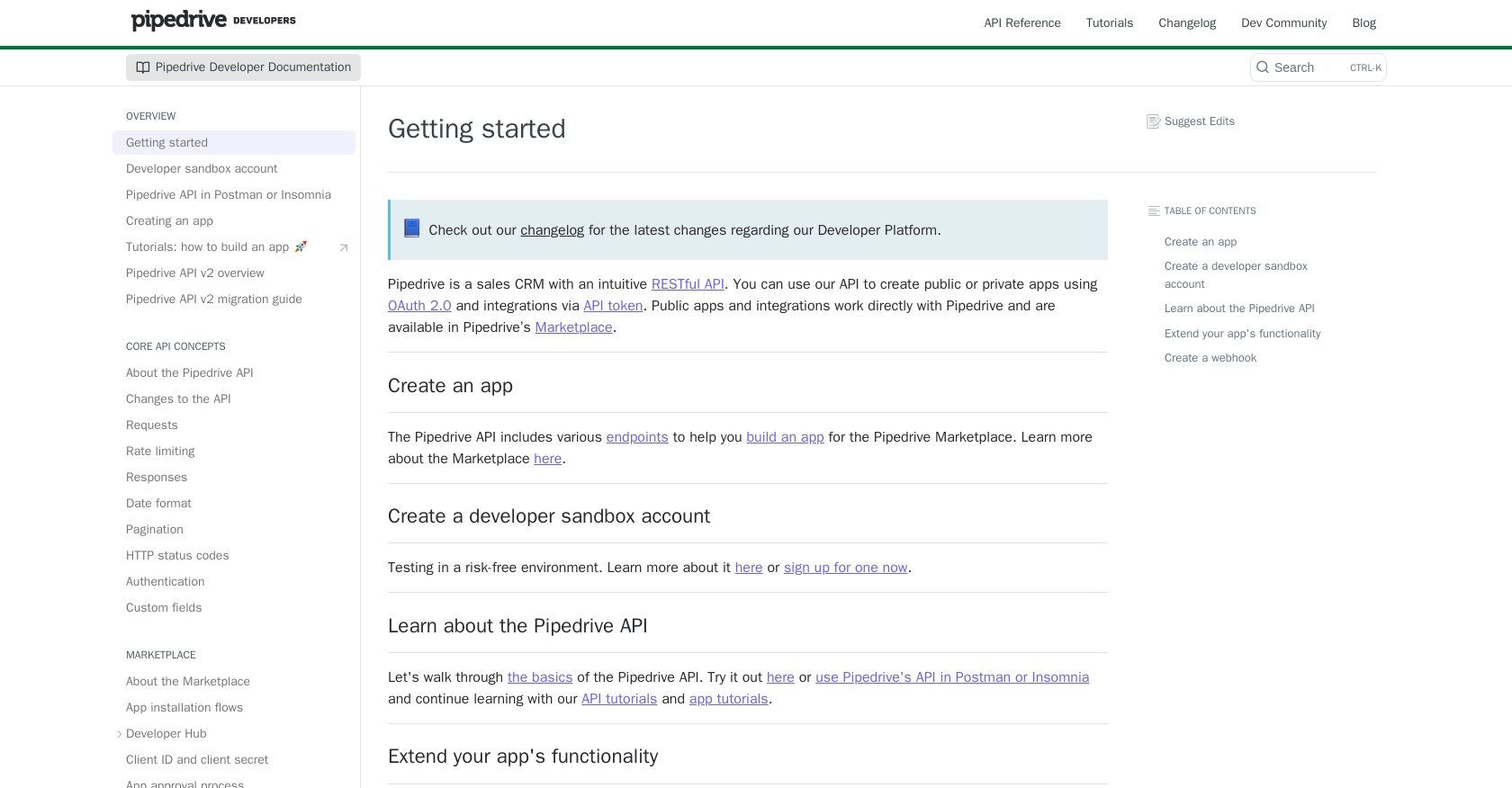
sbb-itb-96038d7
Making API Calls to Pipedrive for Creating or Updating Organizations Using PHP
To interact with the Pipedrive API for creating or updating organizations, you'll need to use PHP. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses.
Setting Up Your PHP Environment for Pipedrive API Integration
Before making API calls, ensure you have the following prerequisites:
- PHP 7.4 or higher installed on your machine.
- Composer for managing dependencies.
- The
guzzlehttp/guzzle
library for making HTTP requests. Install it using Composer:
composer require guzzlehttp/guzzle
Creating or Updating Organizations with Pipedrive API in PHP
With your environment set up, you can now write the PHP code to create or update organizations in Pipedrive.
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token'; // Replace with your actual access token
// Define the organization data
$organizationData = [
'name' => 'New Organization Name',
'owner_id' => 123456 // Optional: Replace with actual owner ID
];
// Make the API request to create an organization
$response = $client->request('POST', 'https://api.pipedrive.com/v1/organizations', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json'
],
'json' => $organizationData
]);
// Check the response status
if ($response->getStatusCode() == 201) {
echo "Organization created successfully.";
} else {
echo "Failed to create organization.";
}
Replace Your_Access_Token
with the access token obtained during the OAuth process. The above code snippet demonstrates how to create a new organization. To update an existing organization, use the PUT
method and provide the organization ID in the URL.
Handling API Responses and Errors
After making the API call, it's crucial to handle the response properly. Check the status code to verify if the request was successful. Pipedrive uses standard HTTP status codes:
- 200 OK: Request fulfilled successfully.
- 201 Created: New resource created successfully.
- 400 Bad Request: Request not understood by the server.
- 401 Unauthorized: Invalid API token or access token.
- 429 Too Many Requests: Rate limit exceeded.
For more detailed error handling, refer to the Pipedrive API documentation on HTTP status codes.
Verifying API Call Success in Pipedrive Sandbox
To confirm that your API call was successful, log in to your Pipedrive sandbox account and check the organizations list. Newly created or updated organizations should appear there. This verification step ensures that your integration is functioning as expected.
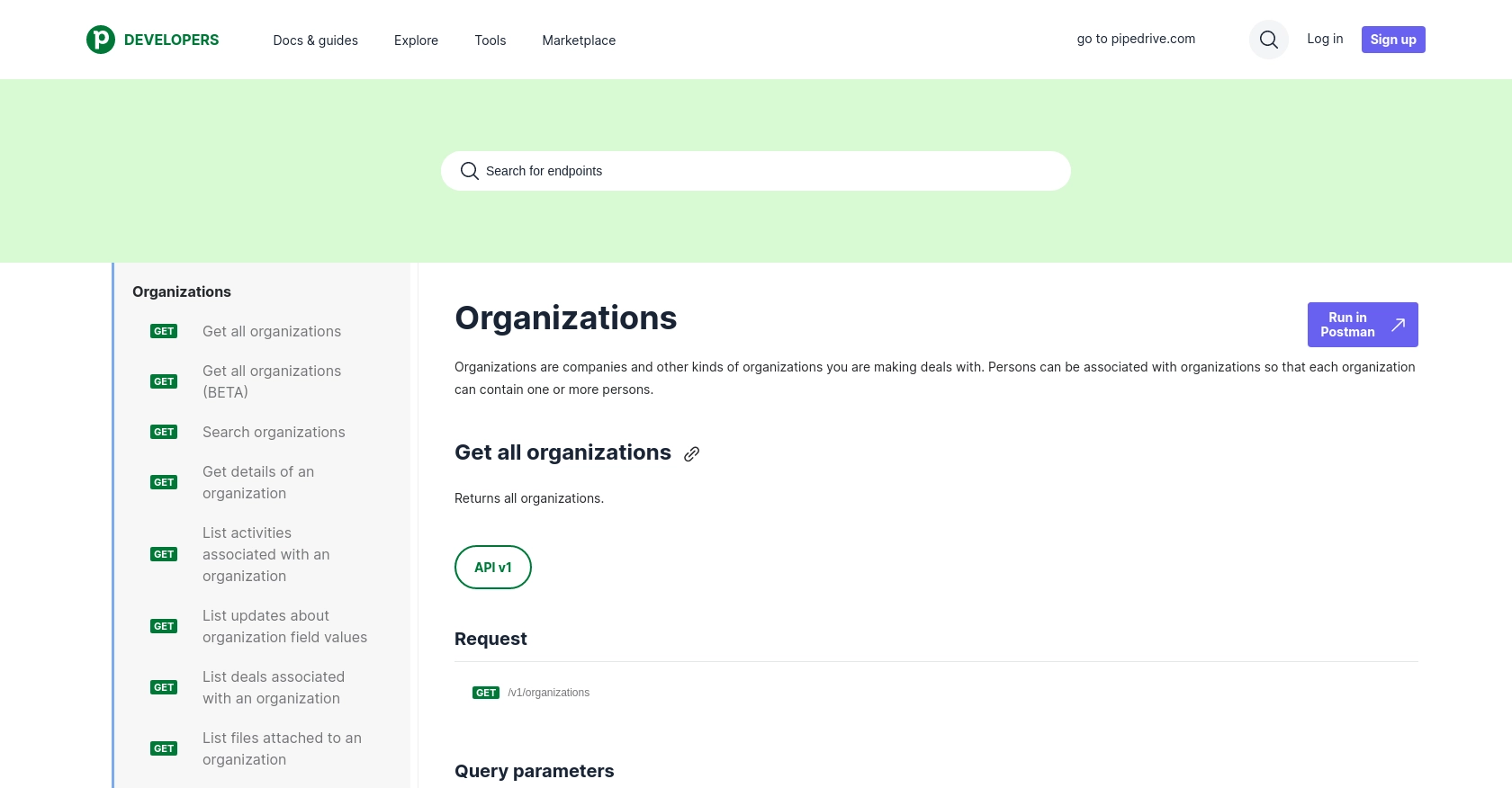
Conclusion and Best Practices for Pipedrive API Integration in PHP
Integrating with the Pipedrive API using PHP allows developers to automate and enhance sales operations efficiently. By following the steps outlined in this guide, you can create or update organization records seamlessly, ensuring your sales data remains current and accessible.
Best Practices for Secure and Efficient Pipedrive API Usage
- Securely Store Credentials: Always store your OAuth credentials, such as the client ID and secret, securely. Avoid hardcoding them in your source code.
- Handle Rate Limiting: Pipedrive's API has rate limits based on your plan. For OAuth apps, the limit is 80 requests per 2 seconds per access token. Implement logic to handle HTTP 429 errors gracefully and consider using webhooks to reduce unnecessary API calls. For more details, refer to the Pipedrive API rate limiting documentation.
- Data Transformation: Standardize and transform data fields as needed to ensure consistency across your applications and Pipedrive.
Streamline Your Integration Process with Endgrate
While building integrations with the Pipedrive API can be rewarding, it can also be time-consuming. Endgrate offers a unified API endpoint that simplifies the integration process, allowing you to focus on your core product. With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Visit Endgrate to learn more about how you can save time and resources by outsourcing your integrations.
By adhering to these best practices and utilizing tools like Endgrate, you can ensure a robust and efficient integration with Pipedrive, enhancing your sales operations and driving business success.
Read More
- https://endgrate.com/provider/pipedrive
- https://pipedrive.readme.io/docs/getting-started
- https://pipedrive.readme.io/docs/developer-sandbox-account
- https://pipedrive.readme.io/docs/marketplace-creating-a-proper-app
- https://pipedrive.readme.io/docs/core-api-concepts-rate-limiting
- https://pipedrive.readme.io/docs/core-api-concepts-pagination
- https://pipedrive.readme.io/docs/core-api-concepts-http-status-codes
- https://pipedrive.readme.io/docs/core-api-concepts-custom-fields
- https://developers.pipedrive.com/docs/api/v1/Organizations
- https://developers.pipedrive.com/docs/api/v1/OrganizationFields
- https://developers.pipedrive.com/docs/api/v1/OrganizationRelationships
Ready to get started?