How to Get Companies with the OnePageCRM API in Python
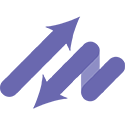
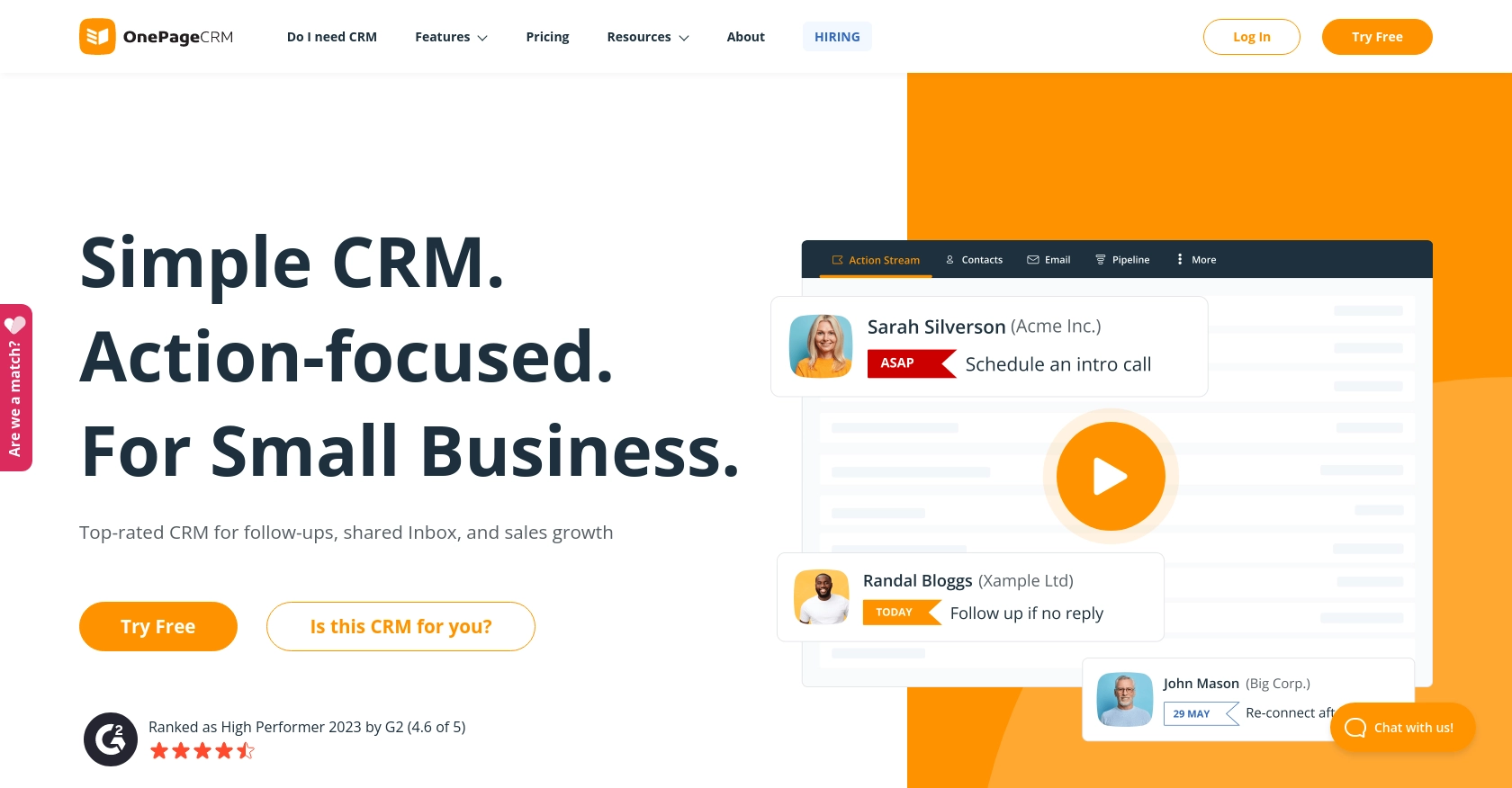
Introduction to OnePageCRM
OnePageCRM is a dynamic customer relationship management platform designed to simplify sales processes for small and medium-sized businesses. Its intuitive interface and robust features help sales teams manage contacts, track deals, and automate workflows efficiently.
Integrating with OnePageCRM's API allows developers to access and manage company data seamlessly. For example, you might want to retrieve a list of companies to analyze sales trends or integrate with other business applications. This article will guide you through using Python to interact with the OnePageCRM API to fetch company information.
Setting Up Your OnePageCRM Test Account
Before you can start interacting with the OnePageCRM API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Creating a OnePageCRM Account
If you don't already have a OnePageCRM account, you can sign up for a free trial on their website. This trial will give you access to the necessary features to test API interactions.
- Visit the OnePageCRM website and click on the "Free Trial" button.
- Fill out the registration form with your details and submit it.
- Once registered, log in to your OnePageCRM account.
Generating API Credentials for OnePageCRM
OnePageCRM uses a custom authentication method to secure API access. Follow these steps to generate the necessary credentials:
- Navigate to the "Settings" section in your OnePageCRM dashboard.
- Locate the "API & Integrations" tab and click on it.
- Here, you will find options to create an API key. Follow the prompts to generate your API key.
- Make sure to securely store the API key, as you will need it to authenticate your API requests.
Configuring Your Development Environment
With your OnePageCRM account and API key ready, you can now set up your development environment to start making API calls:
- Ensure you have Python 3.11.1 installed on your machine.
- Install the necessary Python packages using pip:
pip install requests
With these steps completed, you're ready to begin interacting with the OnePageCRM API using Python.
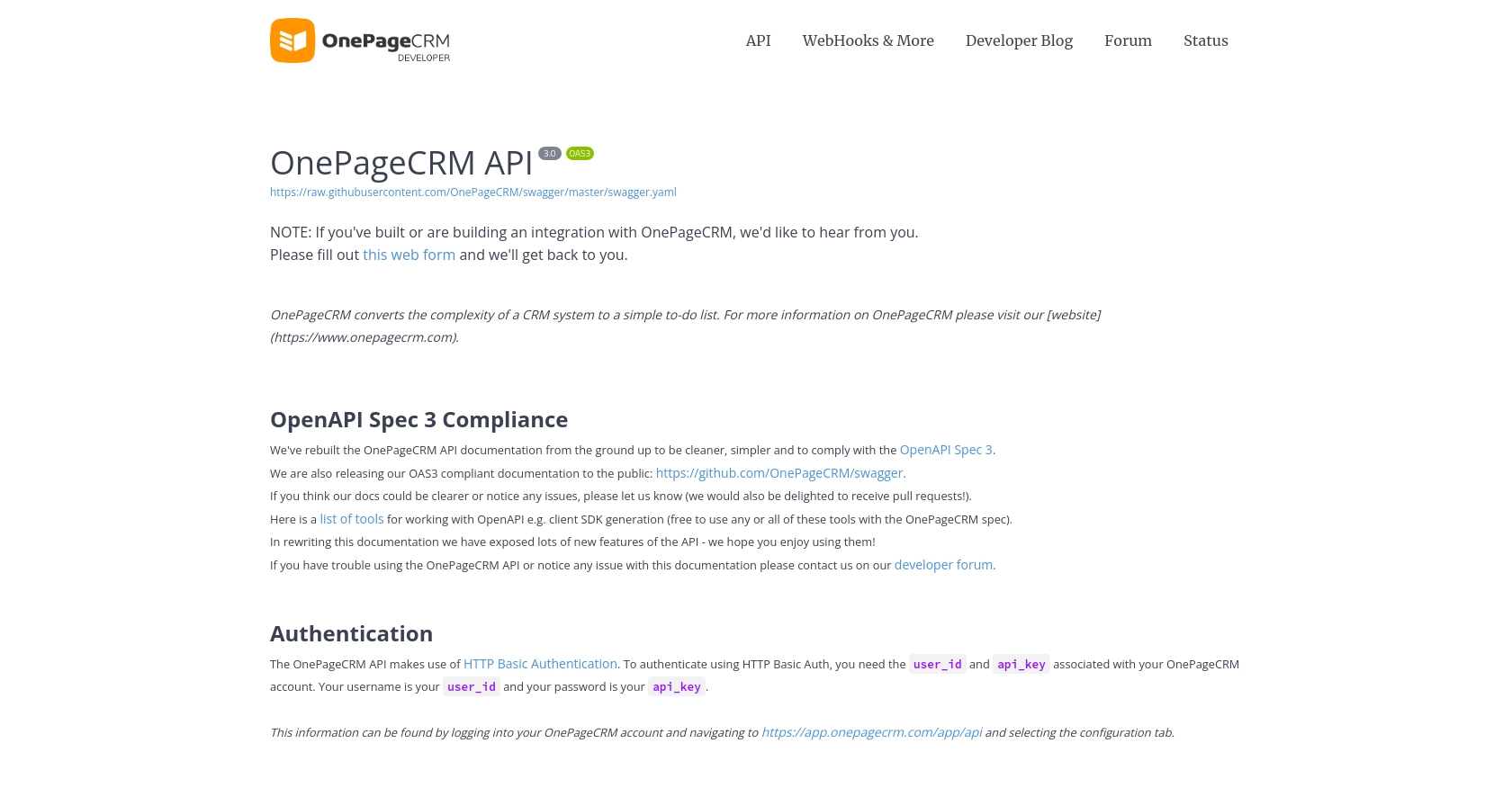
sbb-itb-96038d7
Making API Calls to Retrieve Companies from OnePageCRM Using Python
With your OnePageCRM account set up and API key in hand, you can now proceed to make API calls to retrieve company data. This section will guide you through the process of using Python to interact with the OnePageCRM API and fetch a list of companies.
Setting Up Your Python Environment for OnePageCRM API
Before making API calls, ensure your Python environment is correctly configured:
- Verify that Python 3.11.1 is installed on your system.
- Install the
requests
library, which is essential for making HTTP requests:
pip install requests
Writing Python Code to Fetch Companies from OnePageCRM
Now, let's write the Python code to retrieve a list of companies from OnePageCRM:
import requests
# Define the API endpoint and headers
base_url = "https://app.onepagecrm.com/api/v3/"
endpoint = "companies"
url = f"{base_url}{endpoint}"
headers = {
"Authorization": "Bearer Your_API_Key",
"Content-Type": "application/json"
}
# Make a GET request to the API
response = requests.get(url, headers=headers)
# Check if the request was successful
if response.status_code == 200:
companies = response.json()
for company in companies['data']:
print(company['name'])
else:
print(f"Failed to retrieve companies: {response.status_code} - {response.text}")
Replace Your_API_Key
with the API key you generated earlier.
Understanding the API Response and Handling Errors
Upon executing the code, the API will return a JSON response containing the list of companies. The code iterates through the data and prints each company's name. If the request fails, the error message and status code will be displayed.
Common response codes include:
- 200 OK: The request was successful, and the companies' data is returned.
- 400 Bad Request: The request was malformed.
- 401 Unauthorized: Authentication failed, possibly due to an invalid API key.
- 403 Forbidden: The request is not allowed.
- 404 Not Found: The endpoint could not be found.
- 500 Internal Server Error: An error occurred on the server.
For more details on error codes, refer to the OnePageCRM API documentation.
Verifying API Call Success in OnePageCRM
To confirm the successful retrieval of company data, you can cross-check the results in your OnePageCRM dashboard. The companies listed in the API response should match those in your OnePageCRM account.
Conclusion and Best Practices for Using OnePageCRM API with Python
Integrating with the OnePageCRM API using Python can significantly enhance your ability to manage and analyze company data efficiently. By following the steps outlined in this guide, you can seamlessly retrieve company information and integrate it with other business applications.
Best Practices for Secure and Efficient API Integration
- Securely Store API Credentials: Always store your API key securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement exponential backoff strategies to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that the data retrieved from OnePageCRM is standardized and transformed as needed to fit your application's requirements.
- Monitor API Usage: Regularly monitor your API usage and error logs to identify and resolve issues promptly.
Streamline Your Integration Process with Endgrate
While integrating with OnePageCRM's API is straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including OnePageCRM.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. With a single API endpoint, you can connect to multiple services, providing an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined integration process.
Read More
Ready to get started?