Using the Commerce7 API to Create or Update Customers in Python
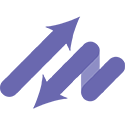
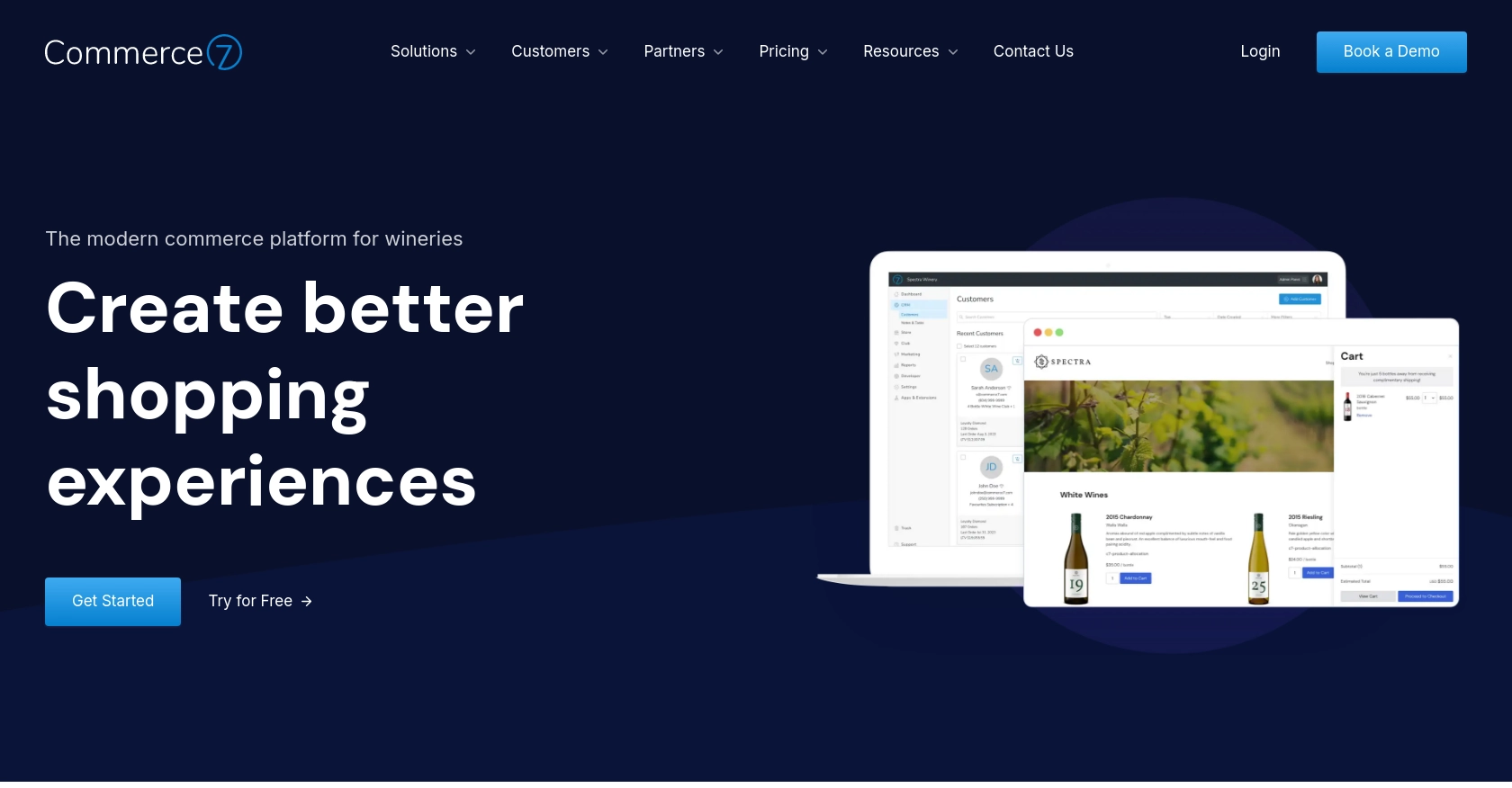
Introduction to Commerce7 API for Customer Management
Commerce7 is a powerful e-commerce platform tailored for the wine industry, offering a suite of tools to enhance customer engagement and streamline sales processes. With its robust API, developers can integrate Commerce7 into their applications to manage customer data efficiently.
Connecting with the Commerce7 API allows developers to automate customer management tasks, such as creating or updating customer profiles. For example, a developer might use the API to sync customer data from an external CRM system to Commerce7, ensuring that customer information is always up-to-date and accurate.
Setting Up a Commerce7 Test/Sandbox Account for API Integration
Before you can start integrating with the Commerce7 API, it's essential to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Create a Commerce7 Developer Account
- Visit the Commerce7 Developer Center and sign up for a developer account.
- Once registered, log in to access the App Development Center.
Generate API Credentials for Authentication
Commerce7 uses a custom authentication method that requires an App ID and App Secret Key. Here's how to generate these credentials:
- In the App Development Center, click on Add App to create a new application.
- Fill in the necessary details and select the API endpoints you need access to.
- Once your app is created, note down the App ID from the URL (e.g.,
demo-app
fromdemo-app.dev-center.platform.commerce7.com
). - Generate an App Secret Key from the app dashboard. Keep this key secure as it will be used for authentication.
Configure Your Sandbox Environment
To ensure your API requests are directed to the correct environment, follow these steps:
- Log into the Commerce7 Admin to obtain the tenant ID. This is the first part of the URL when logged in (e.g.,
spectrawinery
fromhttps://spectrawinery.admin.platform.commerce7.com
). - Ensure your requests include the tenant ID and are directed to the sandbox environment.
Authenticate API Requests
For each API request, use Basic Auth with the following credentials:
# Example of setting up authentication headers
headers = {
"Authorization": "Basic demo-app:App_Secret_Key",
"Content-Type": "application/json",
"tenant": "spectrawinery"
}
Replace demo-app
and App_Secret_Key
with your actual App ID and Secret Key.
With your Commerce7 test account and API credentials set up, you're ready to start making API calls to create or update customer data.
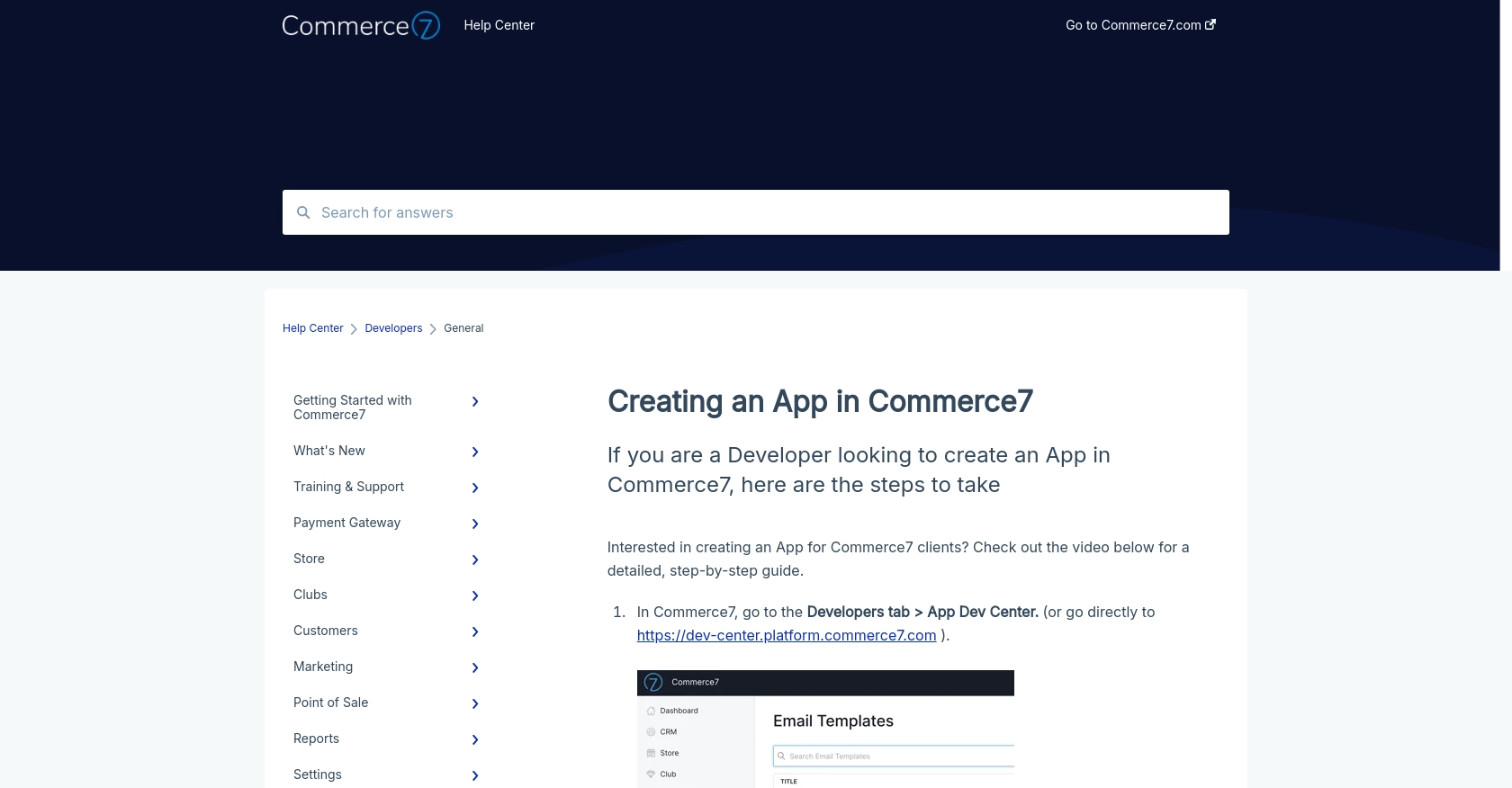
sbb-itb-96038d7
Making API Calls to Create or Update Customers in Commerce7 Using Python
In this section, we'll explore how to interact with the Commerce7 API using Python to create or update customer records. This involves setting up your environment, writing the code, and handling responses effectively.
Setting Up Your Python Environment for Commerce7 API Integration
Before diving into the code, ensure you have the necessary tools and libraries installed:
- Python 3.11.1 or later
- The
requests
library for handling HTTP requests
Install the requests
library using pip:
pip install requests
Creating a Customer in Commerce7 Using Python
To create a new customer, you'll need to make a POST request to the Commerce7 API. Here's a step-by-step guide:
import requests
import base64
# Set up API credentials
app_id = "demo-app"
app_secret_key = "Your_App_Secret_Key"
tenant_id = "spectrawinery"
# Encode credentials for Basic Auth
credentials = f"{app_id}:{app_secret_key}"
encoded_credentials = base64.b64encode(credentials.encode()).decode()
# Set the API endpoint and headers
url = "https://api.commerce7.com/v1/customer"
headers = {
"Authorization": f"Basic {encoded_credentials}",
"Content-Type": "application/json",
"tenant": tenant_id
}
# Define the customer data
customer_data = {
"firstName": "John",
"lastName": "Doe",
"emails": [{"email": "johndoe@example.com"}],
"phones": [{"phone": "+1234567890"}],
"city": "San Francisco",
"stateCode": "CA",
"countryCode": "US"
}
# Make the POST request
response = requests.post(url, json=customer_data, headers=headers)
# Check the response
if response.status_code == 201:
print("Customer created successfully:", response.json())
else:
print("Failed to create customer:", response.status_code, response.text)
Replace Your_App_Secret_Key
with your actual App Secret Key. This script sends a POST request to create a new customer and checks the response for success.
Updating an Existing Customer in Commerce7 Using Python
To update an existing customer, use a PUT request. Here's how you can do it:
# Assume customer_id is the ID of the customer you want to update
customer_id = "a36b6ff1-7190-49a0-895e-fd2c001eb2a6"
# Update endpoint
update_url = f"https://api.commerce7.com/v1/customer/{customer_id}"
# Define the updated data
update_data = {
"city": "Los Angeles",
"stateCode": "CA"
}
# Make the PUT request
update_response = requests.put(update_url, json=update_data, headers=headers)
# Check the response
if update_response.status_code == 200:
print("Customer updated successfully:", update_response.json())
else:
print("Failed to update customer:", update_response.status_code, update_response.text)
This code updates the specified fields for an existing customer. Ensure you have the correct customer_id
for the customer you wish to update.
Handling API Responses and Errors
It's crucial to handle API responses and errors gracefully. Here are some tips:
- Check the status code of the response to determine success or failure.
- Log error messages for debugging purposes.
- Use try-except blocks to catch exceptions during API calls.
For more detailed error handling, refer to the Commerce7 API documentation.
Verifying API Requests in the Commerce7 Sandbox
After making API calls, verify the changes in your Commerce7 sandbox environment. Check if the customer records reflect the updates or creations as expected.
By following these steps, you can efficiently manage customer data in Commerce7 using Python, ensuring a seamless integration experience.
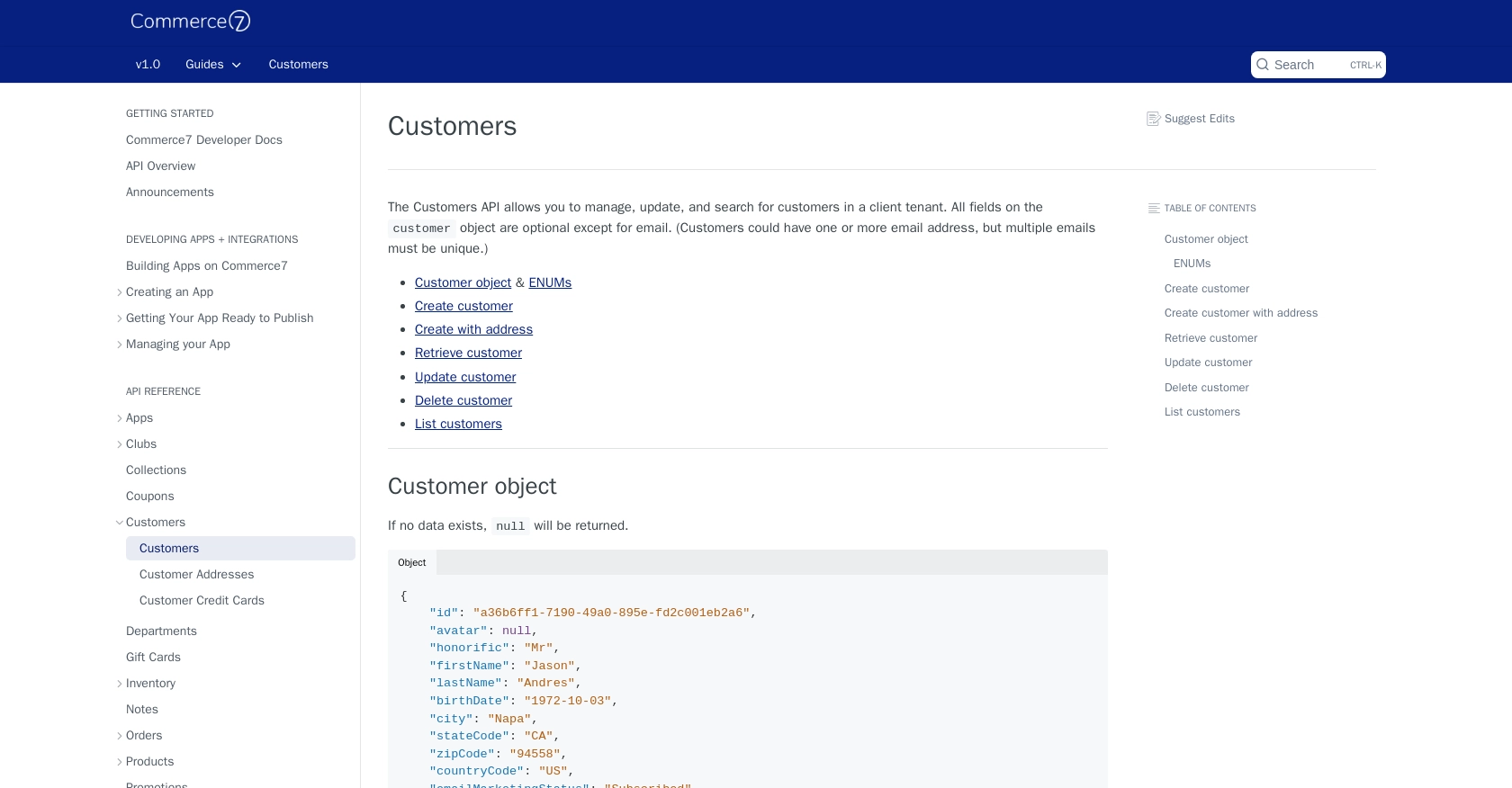
Conclusion and Best Practices for Integrating with Commerce7 API
Integrating with the Commerce7 API to manage customer data offers a streamlined approach to maintaining accurate and up-to-date information within your applications. By following the steps outlined in this guide, you can efficiently create and update customer records using Python, ensuring seamless data synchronization with Commerce7.
Best Practices for Secure and Efficient API Integration with Commerce7
- Secure Storage of Credentials: Always store your App Secret Key securely. Avoid hardcoding it in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Commerce7 enforces a rate limit of 100 requests per minute per tenant. Implement logic to handle rate limits gracefully, such as retry mechanisms or request throttling.
- Data Transformation and Standardization: Ensure that data formats, such as dates and currency, are consistent with Commerce7's requirements to avoid errors during API calls.
- Error Handling: Implement robust error handling to manage API response errors effectively. Log errors for debugging and use try-except blocks to catch exceptions.
Enhance Your Integration Strategy with Endgrate
While integrating with Commerce7 can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate offers a unified API solution that simplifies integration processes, allowing you to focus on your core product development.
With Endgrate, you can:
- Save time and resources by outsourcing integration tasks.
- Build once for each use case, reducing redundancy across different integrations.
- Provide an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate.
Read More
Ready to get started?