Using the Jira API to Get Users in PHP
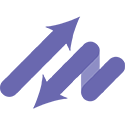
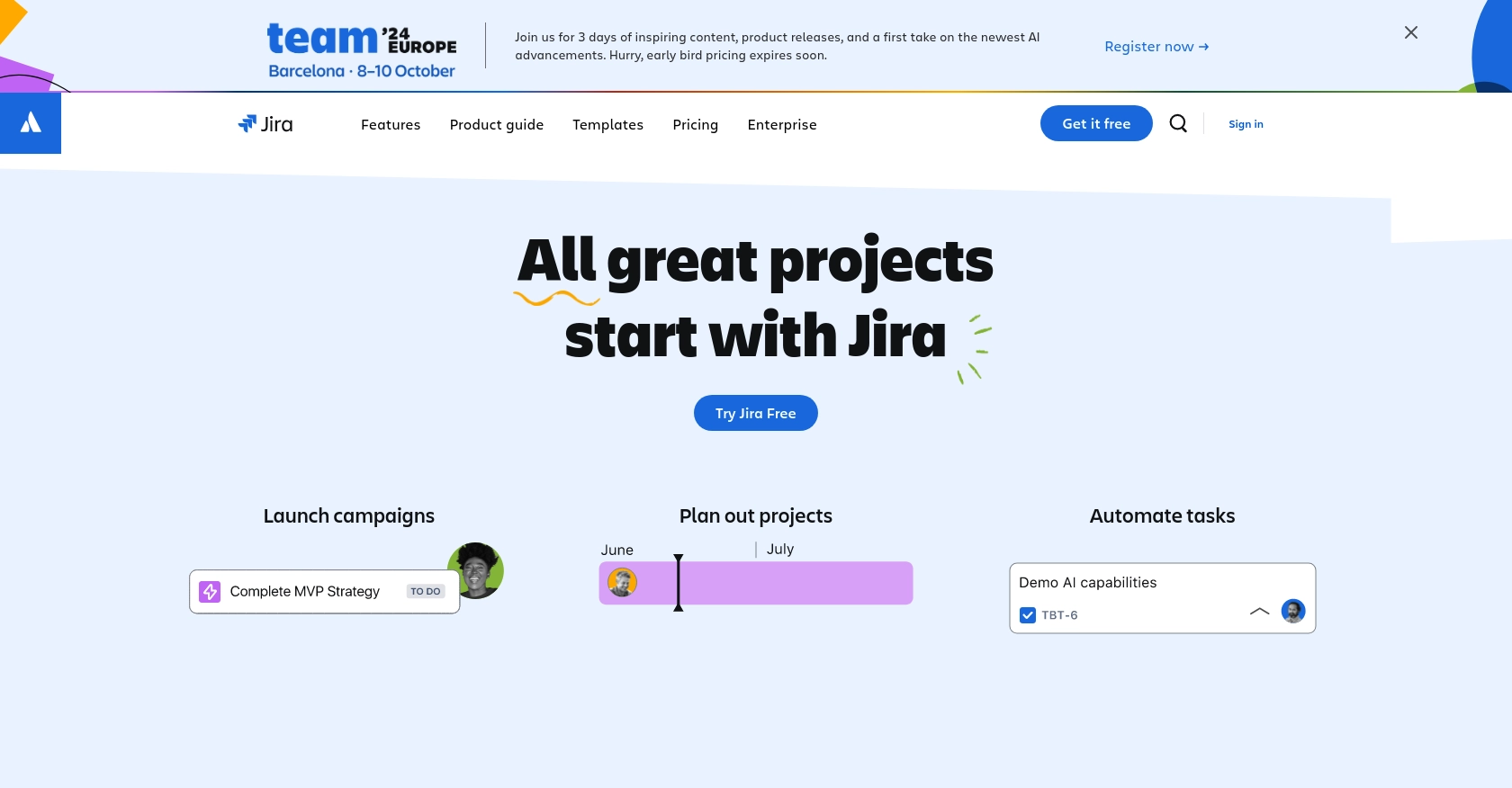
Introduction to Jira API Integration
Jira is a powerful project management tool developed by Atlassian, widely used by software development teams to plan, track, and manage agile software projects. With its robust set of features, Jira helps teams collaborate effectively, ensuring projects are delivered on time and within scope.
Integrating with the Jira API allows developers to automate and streamline various project management tasks. By accessing Jira's extensive API, developers can retrieve user information, manage issues, and enhance workflow automation. For example, a developer might use the Jira API to fetch user details and synchronize them with an internal user management system, ensuring consistency across platforms.
This article will guide you through the process of using PHP to interact with the Jira API, specifically focusing on retrieving user information. By the end of this tutorial, you will be equipped with the knowledge to efficiently integrate Jira's user data into your applications.
Setting Up a Jira Test or Sandbox Account for API Integration
Before you can start interacting with the Jira API using PHP, you need to set up a Jira test or sandbox account. This will allow you to safely experiment with API calls without affecting your production data.
Creating a Jira Account
If you don't already have a Jira account, you can sign up for a free trial on the Jira website. Follow the instructions to create your account. Once your account is set up, you will have access to Jira's features and can begin setting up your API integration.
Setting Up OAuth 2.0 for Jira API Access
Jira uses OAuth 2.0 for secure API access. Follow these steps to set up OAuth 2.0:
- Log in to your Jira account and navigate to the Atlassian Developer Console.
- Click on Create App to start setting up your OAuth 2.0 credentials.
- Fill in the required details for your app, such as the app name and description.
- Under the Authorization section, select OAuth 2.0 (3LO) as the authentication method.
- Specify the necessary scopes for accessing user data. For retrieving users, you will need the
read:jira-user
scope. Refer to the Jira Software scopes documentation for more details. - Once the app is created, you will receive a Client ID and Client Secret. Keep these credentials secure as they will be used to authenticate your API requests.
Generating an Access Token
To interact with the Jira API, you need an access token. Follow these steps to generate one:
- Use the Client ID and Client Secret to request an access token from the Atlassian OAuth token endpoint.
- Include the necessary scopes in your request to ensure you have the required permissions.
- Once you receive the access token, you can use it in your API requests to authenticate and access Jira data.
With your Jira test account and OAuth 2.0 setup complete, you are now ready to start making API calls to retrieve user information using PHP.
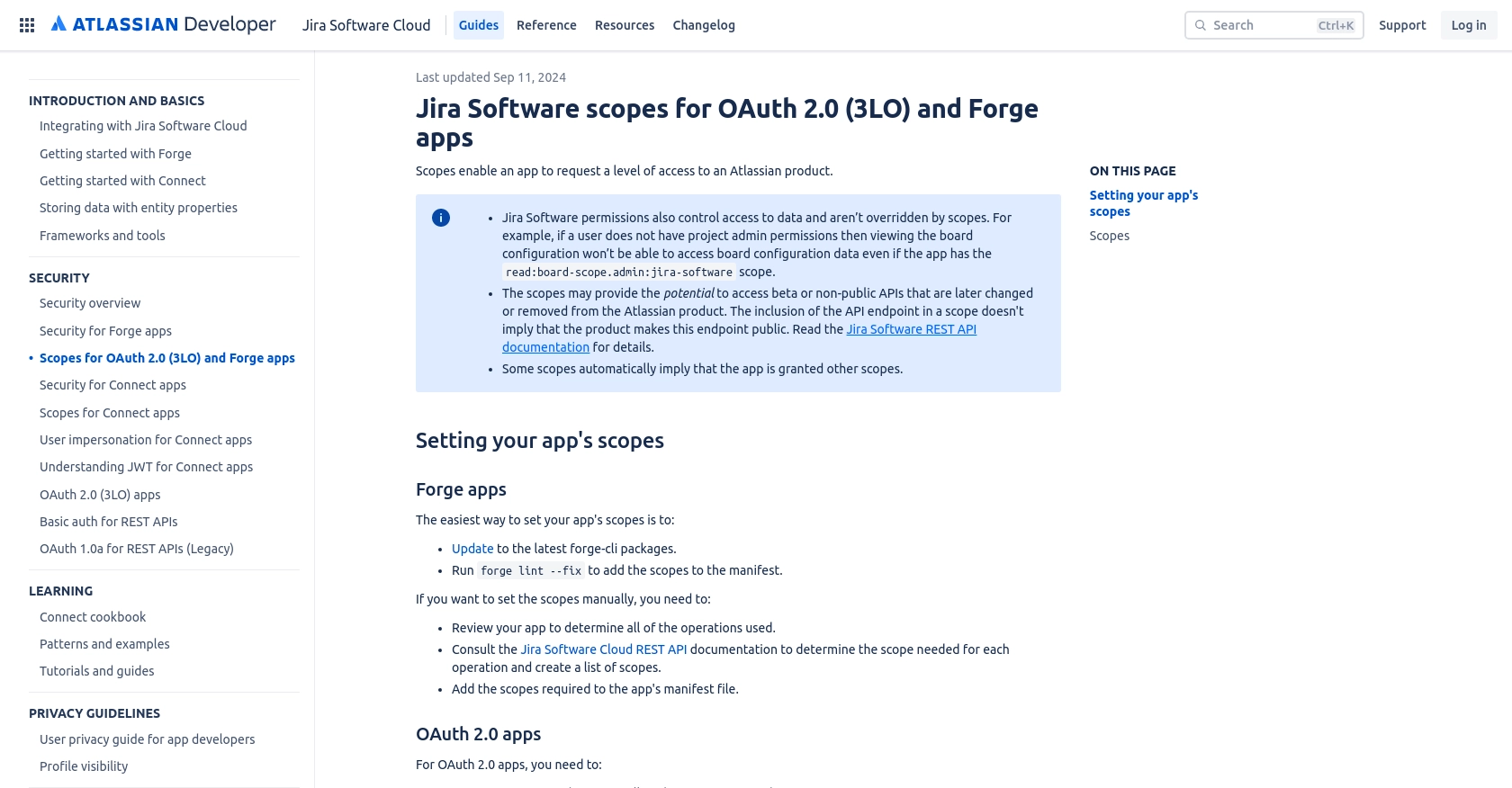
sbb-itb-96038d7
Making API Calls to Retrieve Jira Users Using PHP
Now that you have set up your Jira account and obtained the necessary OAuth 2.0 credentials, you can proceed to make API calls to retrieve user information using PHP. This section will guide you through the process of setting up your PHP environment and executing the API call.
Setting Up Your PHP Environment for Jira API Integration
Before making API requests, ensure that your PHP environment is properly configured. You will need:
- PHP 7.4 or later
- Composer for dependency management
- The
guzzlehttp/guzzle
library for making HTTP requests
Install the Guzzle library using Composer with the following command:
composer require guzzlehttp/guzzle
Executing the API Call to Get Jira Users
With your environment set up, you can now write the PHP code to make the API call. Create a new PHP file, for example, get_jira_users.php
, and add the following code:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token'; // Replace with your actual access token
try {
$response = $client->request('GET', 'https://your-domain.atlassian.net/rest/api/3/users', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Accept' => 'application/json',
],
]);
$users = json_decode($response->getBody(), true);
foreach ($users as $user) {
echo 'User: ' . $user['displayName'] . ' (' . $user['emailAddress'] . ')' . PHP_EOL;
}
} catch (\Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace Your_Access_Token
with the access token you generated earlier. This script uses Guzzle to send a GET request to the Jira API endpoint for retrieving users. It then decodes the JSON response and prints out the display name and email address of each user.
Verifying the API Call and Handling Errors
Run the script from the command line using:
php get_jira_users.php
If successful, you should see a list of users printed in the console. If there are any errors, the script will catch exceptions and display an error message. Common error codes include:
- 401 Unauthorized: Check if your access token is valid and has the necessary scopes.
- 403 Forbidden: Ensure your app has the required permissions to access user data.
- 404 Not Found: Verify the API endpoint URL is correct.
For more details on error codes, refer to the Jira API documentation.
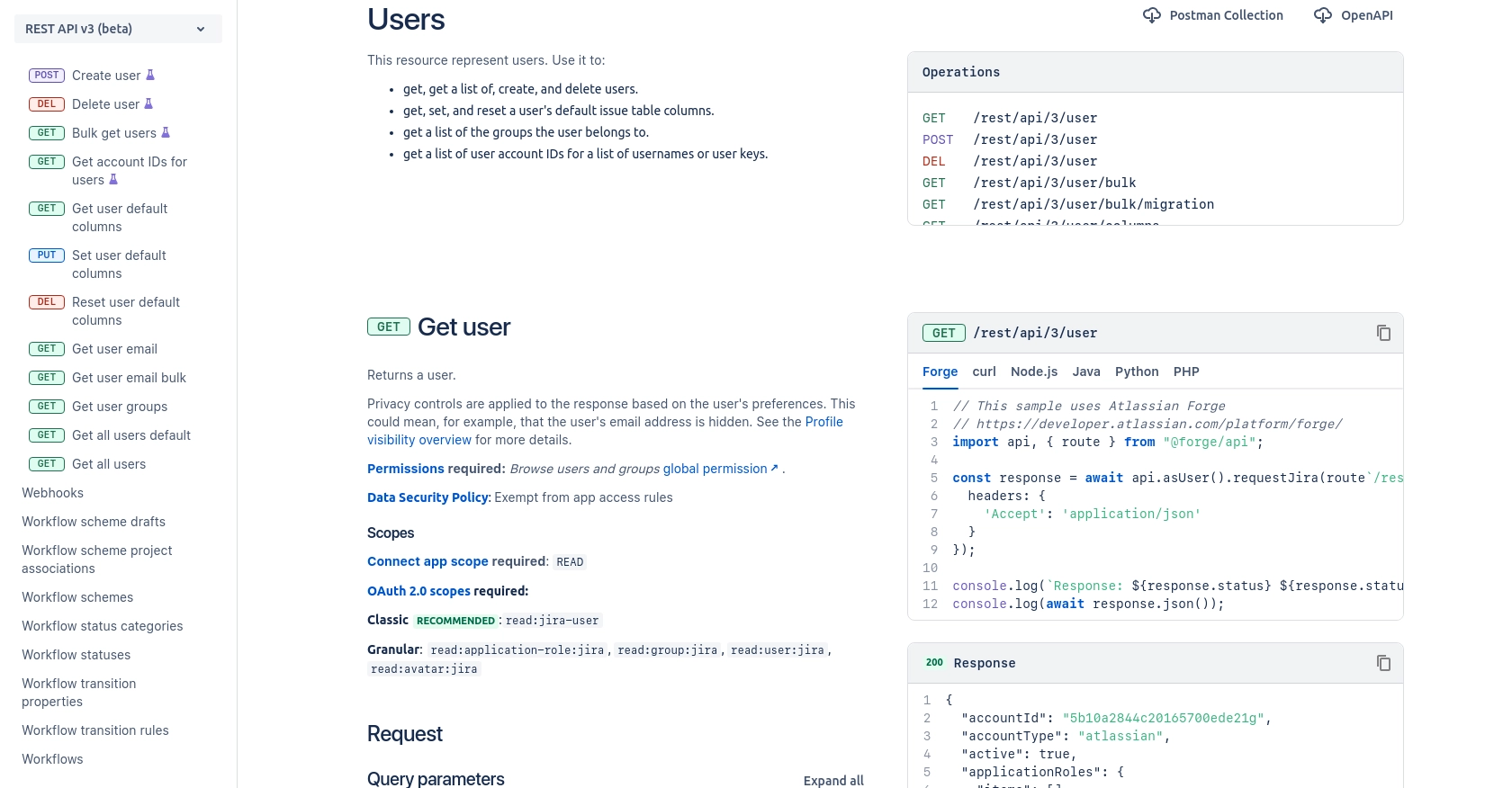
Conclusion and Best Practices for Integrating Jira API with PHP
Integrating with the Jira API using PHP provides a powerful way to automate and enhance your project management workflows. By retrieving user data, you can synchronize information across platforms, ensuring consistency and efficiency in your operations.
Best Practices for Secure and Efficient Jira API Integration
- Secure Storage of Credentials: Always store your Client ID, Client Secret, and access tokens securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Jira API has rate limits to prevent abuse. Ensure your application handles HTTP 429 status codes gracefully and implements retry logic with exponential backoff.
- Data Transformation and Standardization: When integrating data from Jira, ensure that you transform and standardize fields to match your internal data structures for seamless integration.
By following these best practices, you can build robust integrations that leverage Jira's capabilities while maintaining security and efficiency.
Streamline Your Integrations with Endgrate
If managing multiple integrations is becoming a challenge, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers. Visit Endgrate to learn more about how it can simplify your integration processes.
Read More
Ready to get started?