How to Get Balance Transactions with the Stripe API in Python
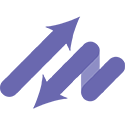
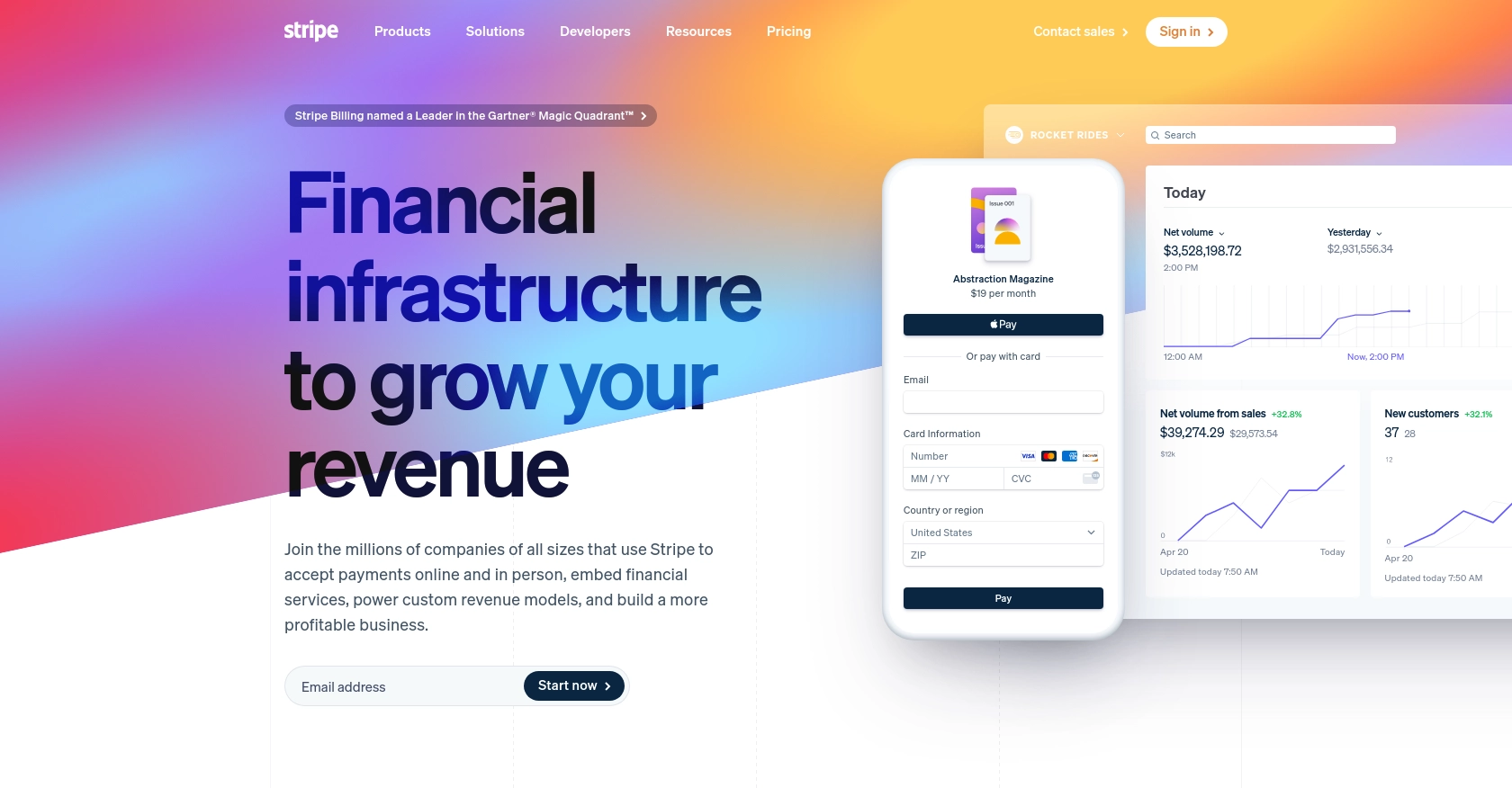
Introduction to Stripe API for Balance Transactions
Stripe is a powerful payment processing platform that enables businesses to accept payments online with ease. Known for its robust API and extensive documentation, Stripe provides developers with the tools needed to integrate payment solutions seamlessly into their applications.
For developers working with financial data, accessing balance transactions through the Stripe API is crucial. This allows for detailed tracking of funds moving in and out of a Stripe account, which is essential for financial reporting and reconciliation.
For example, a developer might want to retrieve balance transaction data to generate financial reports or to ensure that all transactions are accounted for in their accounting system. By integrating with the Stripe API, developers can automate these processes, saving time and reducing the potential for human error.
Setting Up Your Stripe Test Account for Balance Transactions
Before you can start retrieving balance transactions using the Stripe API, you need to set up a test account. Stripe offers a test mode that allows developers to simulate transactions without affecting live data. This is essential for testing and development purposes.
Creating a Stripe Account
If you don't already have a Stripe account, you can sign up for free on the Stripe website. Once your account is created, you can access the Stripe Dashboard, where you can manage your API keys and other account settings.
Accessing Stripe API Keys
Stripe uses API keys to authenticate requests. You can find your test API keys in the Stripe Dashboard under the "Developers" section. Make sure to use the test mode secret key, which typically starts with sk_test_
.
- Navigate to the "Developers" section in the Stripe Dashboard.
- Select "API keys" from the menu.
- Locate your test mode secret key and copy it for use in your API requests.
Configuring Your Environment for API Requests
To interact with the Stripe API using Python, you'll need to set up your development environment. Ensure you have Python installed, along with the necessary libraries.
# Install the Stripe Python library
pip install stripe
Once installed, you can use the library to authenticate your requests using your test API key.
Verifying Your Setup
After setting up your test account and configuring your environment, it's a good practice to verify that everything is working correctly. You can do this by making a simple API call to retrieve your account balance.
import stripe
# Set your secret key
stripe.api_key = "sk_test_your_test_key"
# Retrieve balance
balance = stripe.Balance.retrieve()
print(balance)
If the setup is correct, you should see a response containing your Stripe account's balance information. This confirms that your API key is valid and your environment is correctly configured.
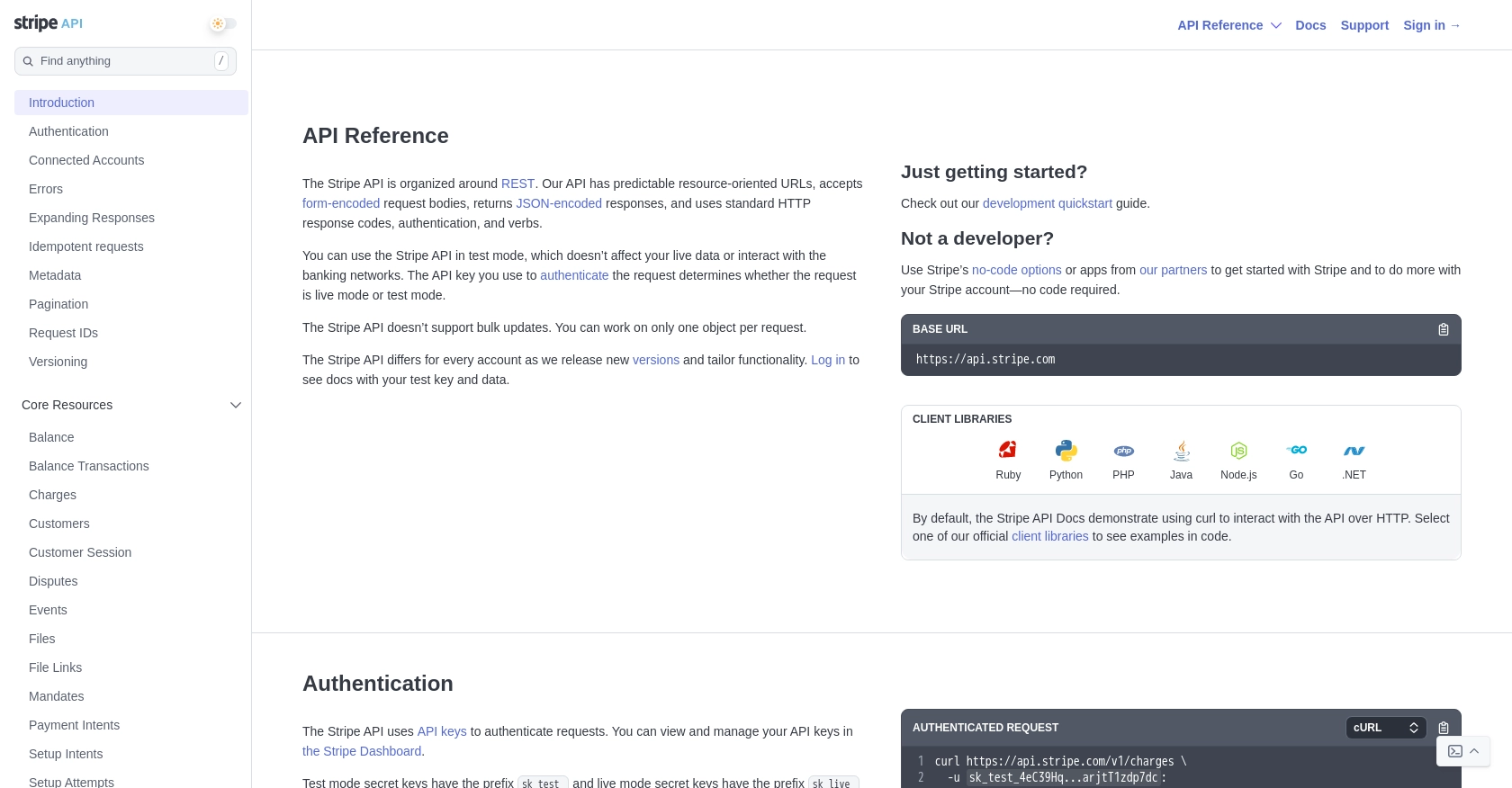
sbb-itb-96038d7
Making API Calls to Retrieve Stripe Balance Transactions Using Python
To effectively interact with the Stripe API and retrieve balance transactions, you'll need to follow a series of steps to ensure your Python environment is properly configured and your API requests are correctly formatted.
Setting Up Python Environment for Stripe API Integration
Before making API calls, ensure you have Python installed on your system. This tutorial uses Python 3.11.1. Additionally, you'll need the Stripe Python library, which can be installed using pip:
pip install stripe
This library simplifies the process of making API requests to Stripe, handling authentication and request formatting for you.
Writing Python Code to Retrieve Balance Transactions from Stripe
Once your environment is set up, you can write a Python script to retrieve balance transactions. Create a file named get_balance_transactions.py
and add the following code:
import stripe
# Set your secret API key
stripe.api_key = "sk_test_your_test_key"
# Retrieve balance transactions
balance_transactions = stripe.BalanceTransaction.list(limit=10)
# Print the balance transactions
for transaction in balance_transactions.auto_paging_iter():
print(transaction)
Replace sk_test_your_test_key
with your actual test API key. This script retrieves a list of balance transactions and prints each transaction's details.
Understanding and Verifying API Response from Stripe
After running your script, you should see a list of balance transactions printed in the console. Each transaction object contains details such as the amount, currency, and type of transaction. This confirms that your API call was successful and your environment is correctly configured.
Handling Errors and Stripe API Response Codes
When making API calls, it's important to handle potential errors. Stripe uses standard HTTP response codes to indicate success or failure:
- 2xx: Success
- 4xx: Client error (e.g., invalid parameters)
- 5xx: Server error (rare)
Here's an example of how to handle errors in your script:
try:
balance_transactions = stripe.BalanceTransaction.list(limit=10)
for transaction in balance_transactions.auto_paging_iter():
print(transaction)
except stripe.error.StripeError as e:
print(f"An error occurred: {e.user_message}")
This code catches any Stripe-related errors and prints a user-friendly message.
For more detailed information on handling errors, refer to the Stripe API documentation.
Conclusion and Best Practices for Using Stripe API with Python
Integrating with the Stripe API to retrieve balance transactions using Python offers developers a powerful way to manage financial data efficiently. By automating the retrieval and processing of transaction data, businesses can enhance their financial reporting and reconciliation processes.
Best Practices for Securely Storing Stripe API Credentials
When working with API keys, it's crucial to keep them secure. Avoid sharing your secret keys in publicly accessible areas such as GitHub or client-side code. Consider using environment variables or secure vaults to store your API keys safely.
Handling Stripe API Rate Limits
Stripe imposes rate limits on API requests to ensure fair usage. If you exceed these limits, you may receive a 429 Too Many Requests
error. Implementing exponential backoff strategies can help manage retries and prevent hitting these limits.
Transforming and Standardizing Stripe Data
When processing balance transactions, consider transforming and standardizing data fields to match your internal systems. This ensures consistency and simplifies integration with other financial tools and databases.
Leverage Endgrate for Streamlined Integration Management
For developers looking to simplify integration processes, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers. Visit Endgrate to learn more about how it can enhance your integration strategy.
Read More
Ready to get started?