How to Create or Update Vendors with the Sage Accounting API in PHP
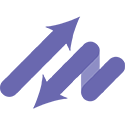
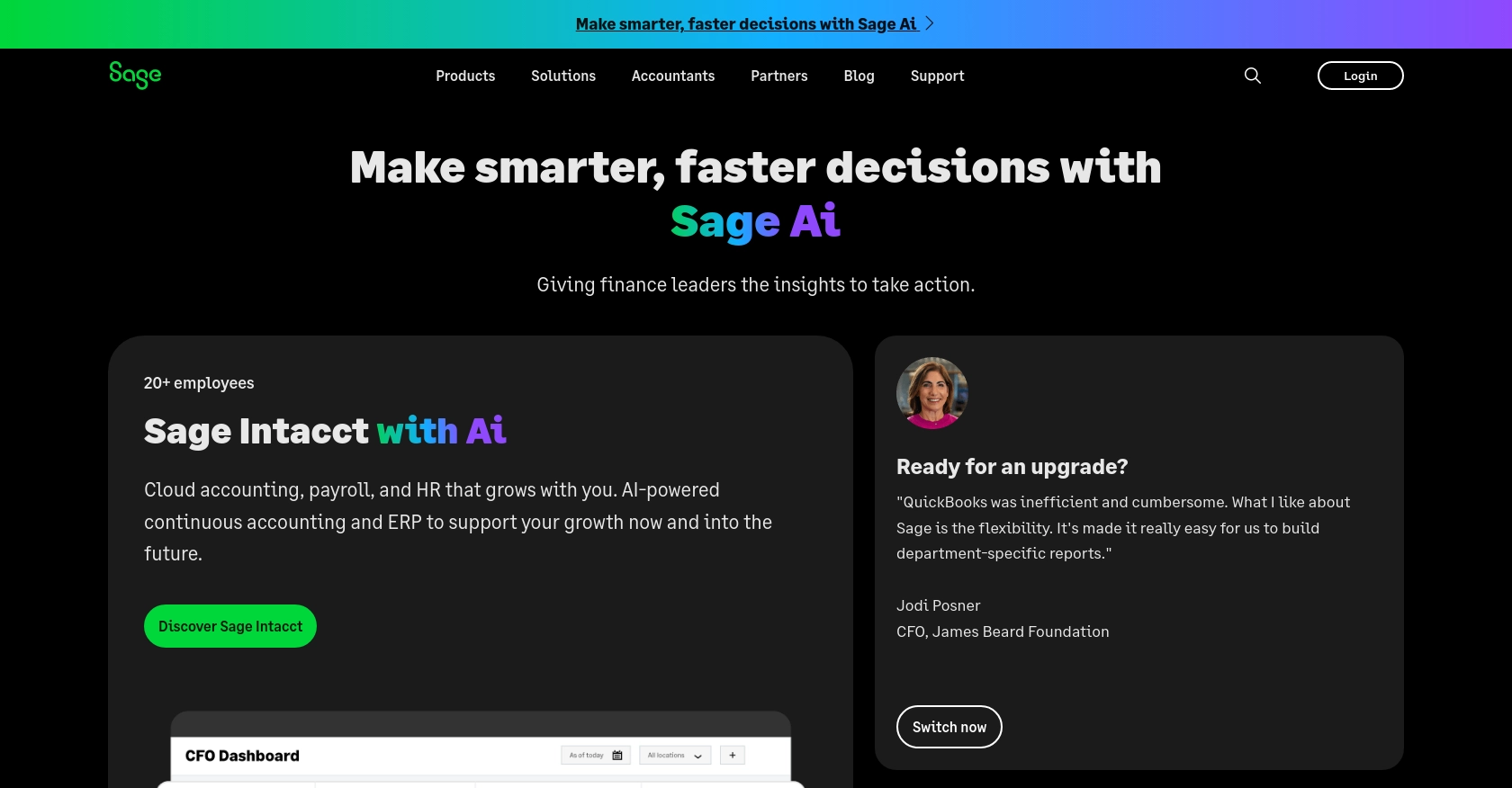
Introduction to Sage Accounting API for Vendor Management
Sage Accounting is a robust cloud-based accounting solution tailored for small and medium-sized businesses. It offers a comprehensive suite of tools for managing financial transactions, invoicing, and vendor relationships. By leveraging the Sage Accounting API, developers can streamline and automate accounting processes, enhancing efficiency and accuracy.
Integrating with the Sage Accounting API allows developers to create or update vendor information programmatically. This capability is particularly useful for businesses that need to manage a large number of vendors or frequently update vendor details. For example, a developer might use the API to automatically update vendor contact information from an external CRM system, ensuring that all records are current and accurate.
Setting Up a Sage Accounting Test or Sandbox Account
Before you can begin integrating with the Sage Accounting API, you'll need to set up a test or sandbox account. This environment allows you to safely develop and test your application without affecting live data.
Step 1: Sign Up for a Sage Developer Account
To start, you'll need a Sage Developer account. This account enables you to register and manage your applications, obtain client credentials, and specify key details such as callback URLs.
- Visit the Sage Developer Portal and sign up using your GitHub account or an email address.
- Follow the guide on creating an app to get your Client ID and Client Secret.
Step 2: Create a Trial Business Account
Next, you'll need to set up a trial business account for development purposes. Sage allows you to create trial businesses for all supported regions and subscription tiers.
- Navigate to the Sage Business Cloud Accounting page.
- Select the appropriate region and subscription tier to create your trial account.
- Use email services that support aliasing to manage multiple environments from the same email account.
Step 3: Configure OAuth Authentication
Sage Accounting API uses OAuth for authentication. You'll need to create an app within your Sage Developer account to obtain the necessary credentials.
- Log in to your Sage Developer account and click on "Create App."
- Enter a name and Callback URL for your app. Optionally, provide an alternative email address and homepage URL.
- Save your app to receive your Client ID and Client Secret.
Step 4: Test Your Setup with Postman
To ensure everything is set up correctly, use a tool like Postman to test your API requests.
- Sign up for a free Postman account and download the Postman app.
- Use your Client ID and Client Secret to authenticate and make requests to the Sage Accounting API.
By following these steps, you'll have a fully configured test environment ready for developing and testing your integration with the Sage Accounting API.
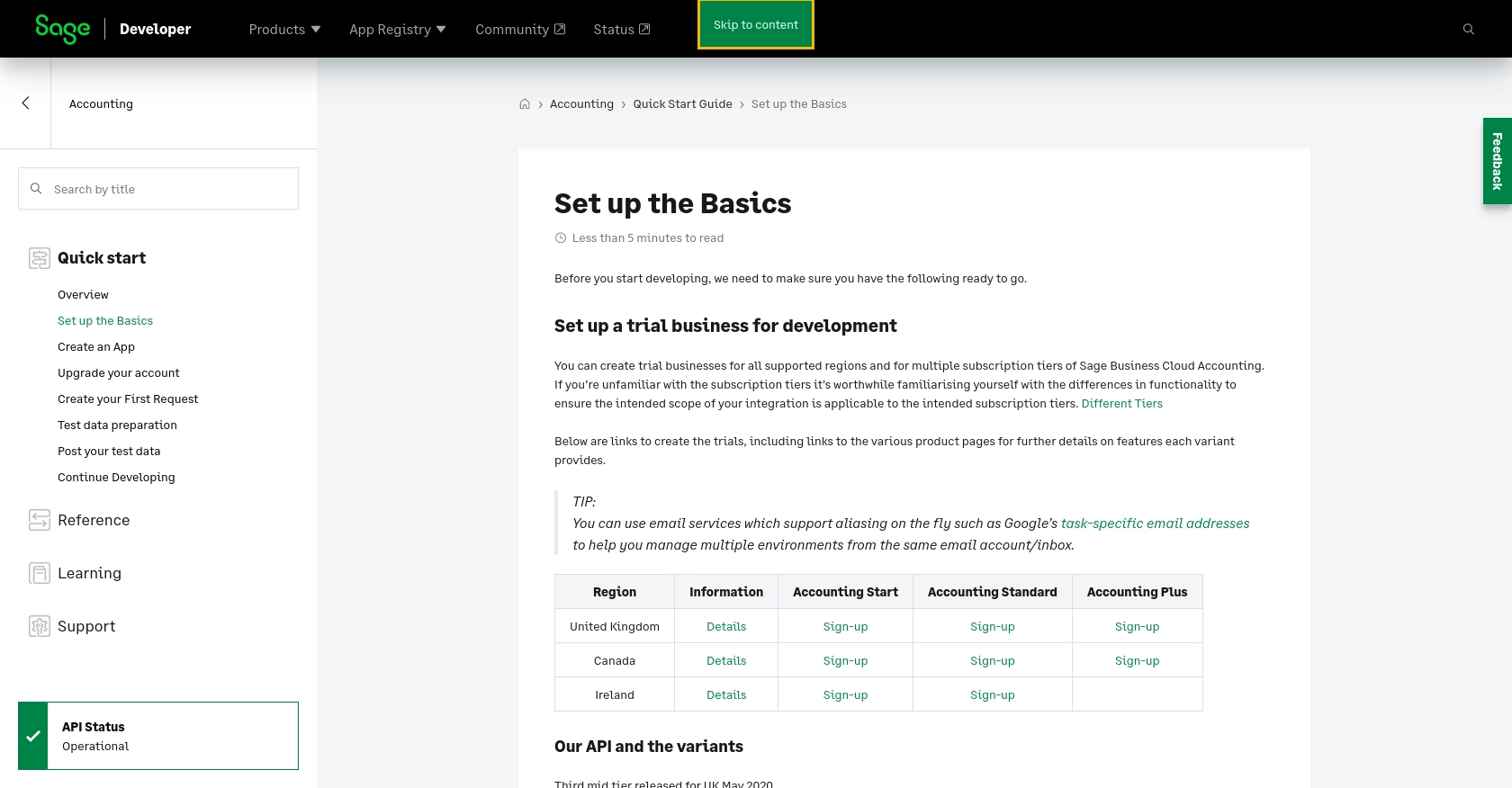
sbb-itb-96038d7
Making API Calls to Create or Update Vendors with Sage Accounting API in PHP
In this section, we'll guide you through the process of making API calls to create or update vendor information using the Sage Accounting API with PHP. This involves setting up your PHP environment, installing necessary dependencies, and executing the API requests.
Setting Up Your PHP Environment for Sage Accounting API
Before making API calls, ensure your PHP environment is properly configured. You'll need PHP version 7.4 or higher and the Composer dependency manager.
- Download and install PHP if it's not already installed on your system.
- Install Composer to manage PHP dependencies.
Installing Required PHP Libraries for API Integration
To interact with the Sage Accounting API, you'll need the Guzzle HTTP client, which simplifies HTTP requests in PHP.
composer require guzzlehttp/guzzle
Creating a Vendor with Sage Accounting API in PHP
Let's create a vendor using the Sage Accounting API. The following PHP script demonstrates how to make a POST request to the API to add a new vendor.
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$response = $client->post('https://api.accounting.sage.com/v3.1/contacts', [
'headers' => [
'Authorization' => 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type' => 'application/json',
],
'json' => [
'contact' => [
'name' => 'New Vendor',
'contact_type_ids' => ['VENDOR_TYPE_ID'],
'reference' => 'Vendor123',
'default_purchase_ledger_account_id' => 'LEDGER_ACCOUNT_ID',
'tax_number' => '123456789',
'main_address' => [
'address_line_1' => '123 Main St',
'city' => 'Anytown',
'postal_code' => '12345',
'country_id' => 'COUNTRY_ID',
],
],
],
]);
echo $response->getBody();
Replace YOUR_ACCESS_TOKEN
, VENDOR_TYPE_ID
, LEDGER_ACCOUNT_ID
, and COUNTRY_ID
with your actual values. This script sends a POST request to create a new vendor with the specified details.
Updating a Vendor with Sage Accounting API in PHP
To update an existing vendor, you'll need to make a PUT request. Here's how you can update vendor information:
$response = $client->put('https://api.accounting.sage.com/v3.1/contacts/{vendor_id}', [
'headers' => [
'Authorization' => 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type' => 'application/json',
],
'json' => [
'contact' => [
'name' => 'Updated Vendor Name',
'tax_number' => '987654321',
],
],
]);
echo $response->getBody();
Replace {vendor_id}
with the ID of the vendor you wish to update. This script updates the vendor's name and tax number.
Handling API Responses and Errors
After making API calls, it's crucial to handle responses and potential errors. Check the response status code to ensure the request was successful.
if ($response->getStatusCode() == 200 || $response->getStatusCode() == 201) {
echo "Vendor operation successful.";
} else {
echo "Failed to perform vendor operation. Error: " . $response->getBody();
}
By following these steps, you can effectively create or update vendors using the Sage Accounting API in PHP. For more details, refer to the Sage API documentation.
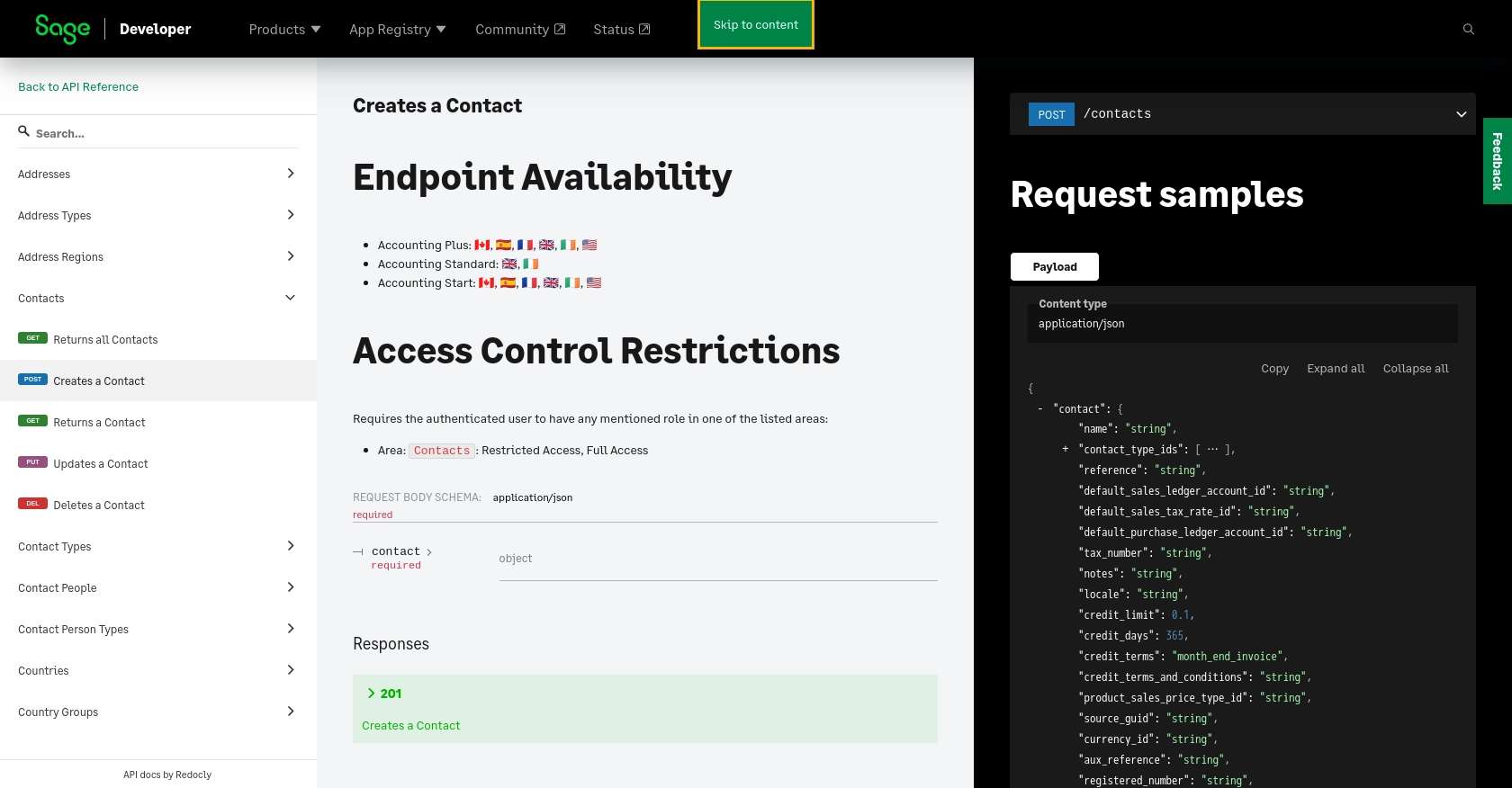
Best Practices for Using Sage Accounting API in PHP
When integrating with the Sage Accounting API, it's essential to follow best practices to ensure security, efficiency, and maintainability. Here are some key recommendations:
Securely Store User Credentials
- Always store sensitive information like Client ID, Client Secret, and access tokens securely. Use environment variables or secure vaults to manage these credentials.
- Regularly rotate your access tokens and secrets to minimize security risks.
Handle API Rate Limiting
Sage Accounting API may impose rate limits on the number of requests you can make. To handle rate limiting effectively:
- Implement exponential backoff strategies to retry requests when you hit rate limits.
- Monitor your API usage and adjust your application's request patterns to stay within limits.
Transform and Standardize Data Fields
Ensure that data fields are consistent and standardized across your application:
- Map external data fields to Sage Accounting's expected formats and values.
- Validate data before sending it to the API to prevent errors and data inconsistencies.
Streamline Your Integration Process with Endgrate
Building and maintaining integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Sage Accounting. With Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive and seamless integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/sage
- https://developer.sage.com/accounting/quick-start/set-up-the-basics/
- https://developer.sage.com/accounting/quick-start/create-an-app/
- https://developer.sage.com/accounting/quick-start/upgrade-your-account/
- https://developer.sage.com/accounting/reference/contacts/#tag/Contacts/operation/postContacts
Ready to get started?