Using the Sage 100 API to Get Vendors in Javascript
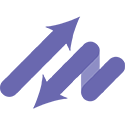
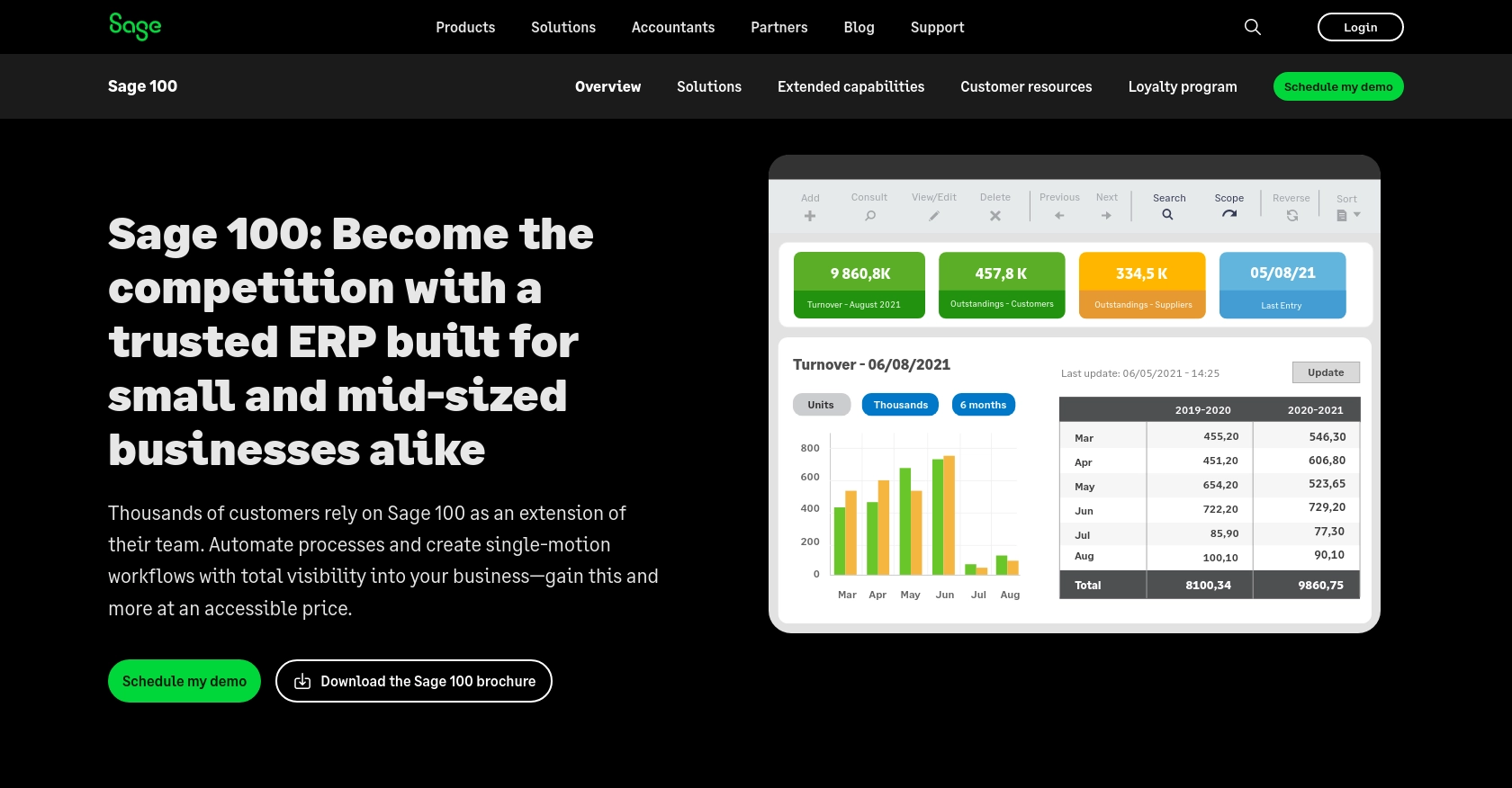
Introduction to Sage 100 API Integration
Sage 100 is a robust enterprise resource planning (ERP) solution tailored for small to medium-sized businesses. It offers comprehensive tools for managing accounting, inventory, and business operations, making it a popular choice for companies seeking to streamline their processes.
Developers may want to integrate with the Sage 100 API to access and manage vendor data efficiently. For example, by retrieving vendor information, a developer can automate the process of updating vendor records in a separate system, ensuring data consistency across platforms.
Setting Up a Sage 100 Test/Sandbox Account for API Integration
Before you can start interacting with the Sage 100 API to retrieve vendor data, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Configuring the Sage 100 ODBC Driver
To begin, ensure that the Sage 100 ODBC driver is installed and properly configured on your system. This is crucial for establishing a connection to the Sage 100 database. Follow these steps:
- Access the ODBC Data Source Administrator on your system.
- Create a Data Source Name (DSN) that connects to the Sage 100 ERP system using the correct server, database, and authentication settings.
For detailed instructions, refer to the Sage 100 ODBC Driver Configuration Guide.
Creating a Sage 100 Sandbox Account
To test API interactions, you may need access to a Sage 100 sandbox environment. Contact your Sage 100 administrator or representative to request access to a sandbox account, which will provide a safe environment for testing API calls.
Setting Up Authentication for Sage 100 API
Sage 100 uses a custom authentication method. Once your DSN is configured, you can establish a connection in your application using the following steps:
- Use the
System.Data.Odbc
namespace in your application. - Create an
OdbcConnection
object with the DSN name in the connection string. - Open the connection using
OdbcConnection.Open()
.
Ensure you handle exceptions using try-catch blocks to manage connection errors and timeouts.
Testing the ODBC Connection
After setting up the DSN, test the connection to ensure it's working correctly:
- Open the ODBC Data Source Administrator.
- Select the DSN you created and test the connection.
If the connection is successful, you are ready to proceed with making API calls to retrieve vendor data.
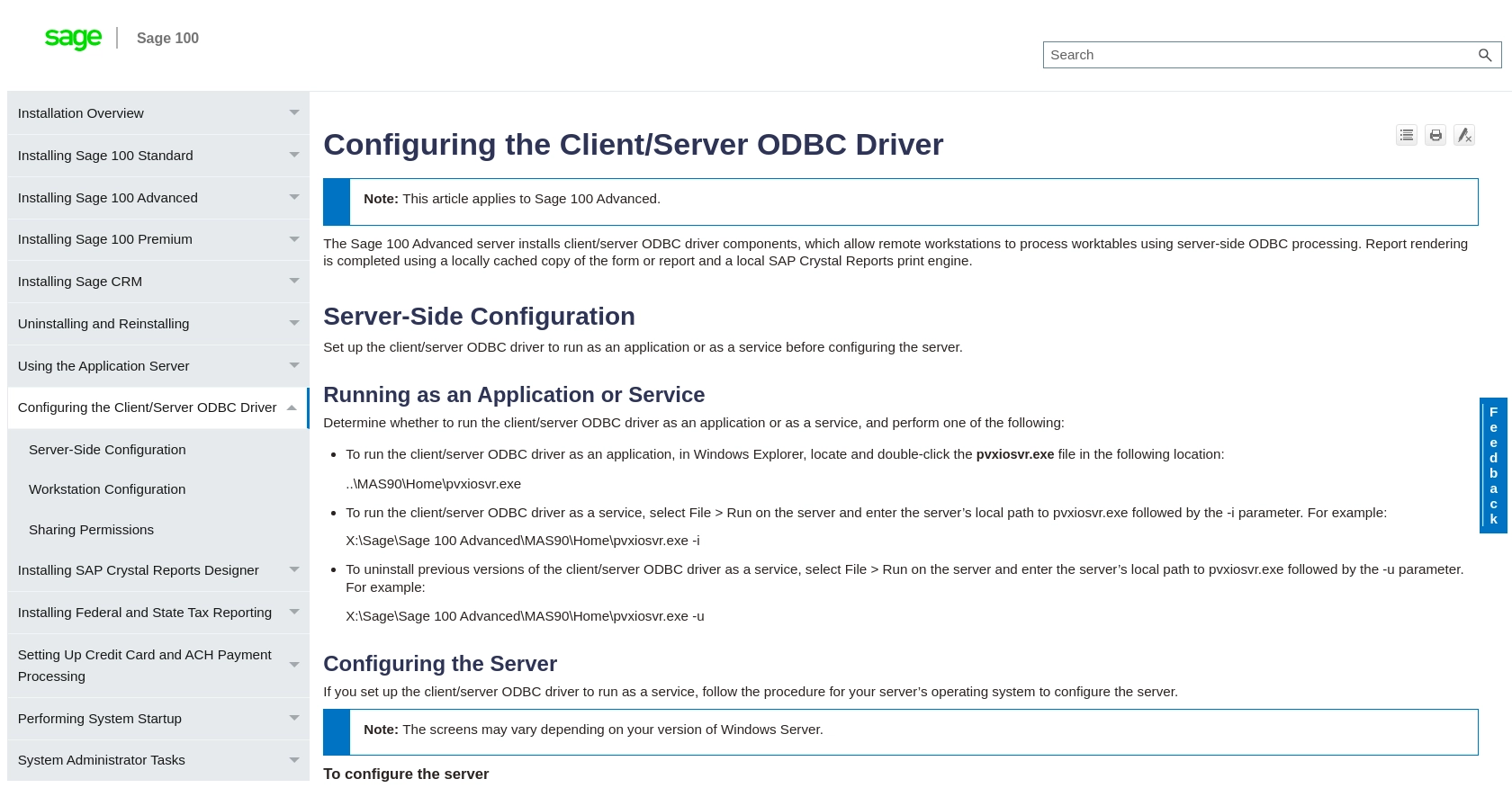
sbb-itb-96038d7
Making API Calls to Retrieve Vendor Data from Sage 100 Using JavaScript
To interact with the Sage 100 API and retrieve vendor data, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment and executing the necessary API calls.
Setting Up Your JavaScript Environment for Sage 100 API
Before making API calls, ensure you have Node.js installed on your system. Node.js provides a runtime environment for executing JavaScript code outside of a browser. You can download and install it from the official Node.js website.
Once Node.js is installed, you can use npm (Node Package Manager) to install any required packages. For this tutorial, we'll use the axios
library to handle HTTP requests. Install it by running the following command in your terminal:
npm install axios
Writing JavaScript Code to Fetch Vendor Data from Sage 100
With your environment set up, you can now write the JavaScript code to fetch vendor data from Sage 100. Create a new file named getVendors.js
and add the following code:
const axios = require('axios'); const odbc = require('odbc'); async function fetchVendors() { try { // Establish ODBC connection const connection = await odbc.connect('DSN=Your_DSN_Name'); // Define SQL query to retrieve vendor data const query = 'SELECT * FROM AP_Vendor'; // Execute the query const result = await connection.query(query); // Log the vendor data console.log(result); // Close the connection await connection.close(); } catch (error) { console.error('Error fetching vendor data:', error); } } fetchVendors();
Replace Your_DSN_Name
with the name of your configured Data Source Name (DSN).
Executing the JavaScript Code to Retrieve Vendor Data
Run the JavaScript file using Node.js by executing the following command in your terminal:
node getVendors.js
If successful, the vendor data from Sage 100 will be printed to the console.
Handling Errors and Validating API Requests
It's crucial to handle potential errors when making API calls. The code above includes a try-catch
block to catch and log any errors that occur during the process. Ensure you have proper error handling to manage connection issues or query failures.
To verify that the request succeeded, check the output in your console. The data should match the vendor records in your Sage 100 sandbox environment.
For more detailed information on the Sage 100 API, refer to the Sage 100 API Documentation.
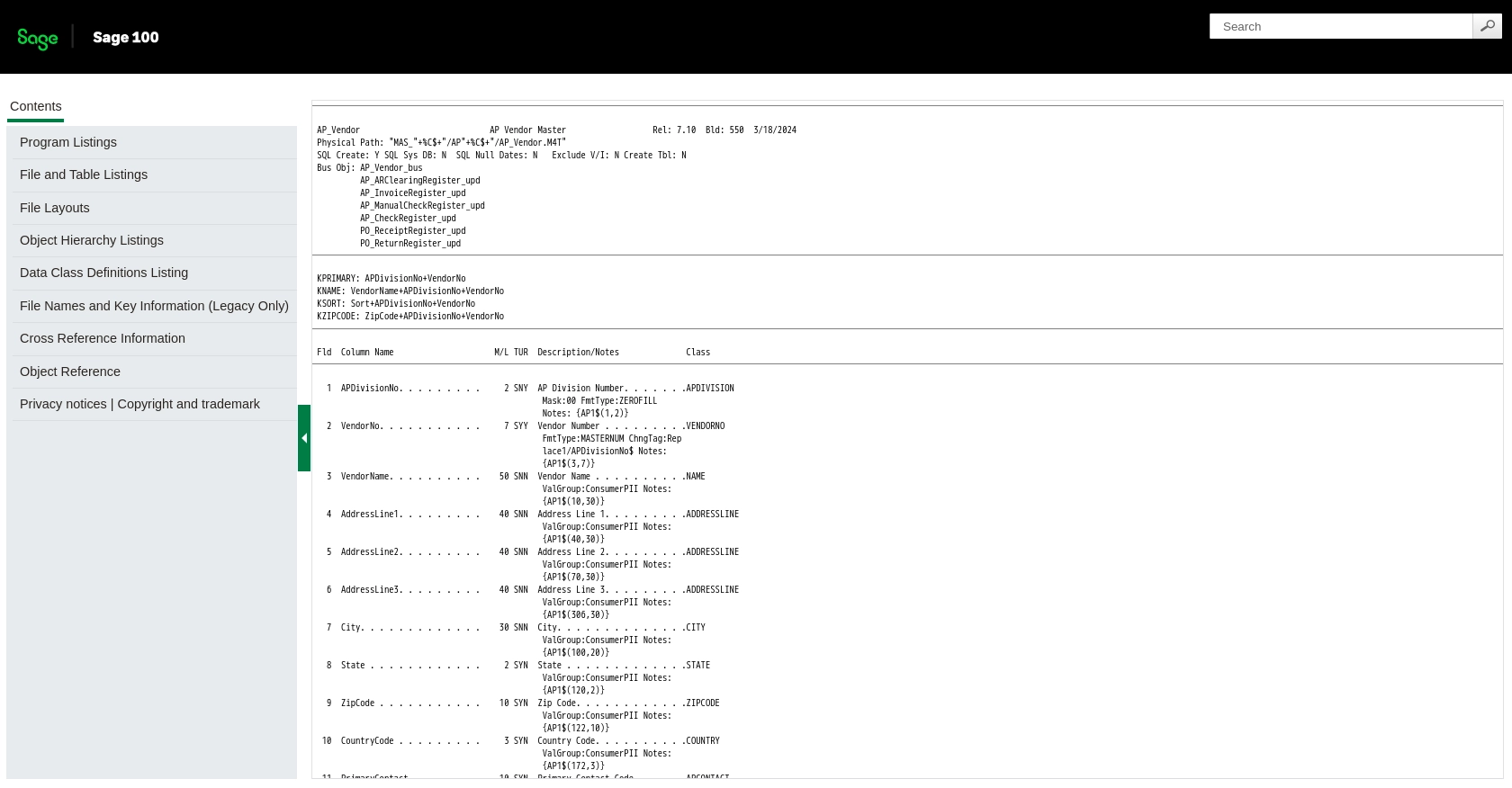
Conclusion and Best Practices for Sage 100 API Integration Using JavaScript
Integrating with the Sage 100 API to retrieve vendor data using JavaScript can significantly enhance your business operations by automating data management and ensuring consistency across platforms. By following the steps outlined in this guide, you can efficiently set up your environment, establish a connection, and execute API calls to access valuable vendor information.
Best Practices for Secure and Efficient Sage 100 API Usage
- Securely Store Credentials: Always store your DSN and any sensitive information securely, using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the Sage 100 API. Implement retry logic and exponential backoff strategies to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that the vendor data retrieved is transformed and standardized to match the format required by your application or system.
- Error Handling: Implement comprehensive error handling to manage potential connection issues, query failures, and other exceptions that may arise during API interactions.
Streamlining Integrations with Endgrate
While integrating with Sage 100 can provide immense value, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including Sage 100. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development while providing an intuitive integration experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover the benefits of outsourcing integrations to streamline your business operations.
Read More
Ready to get started?