How to Get Invoices with the Sap Business One API in Javascript
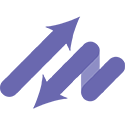
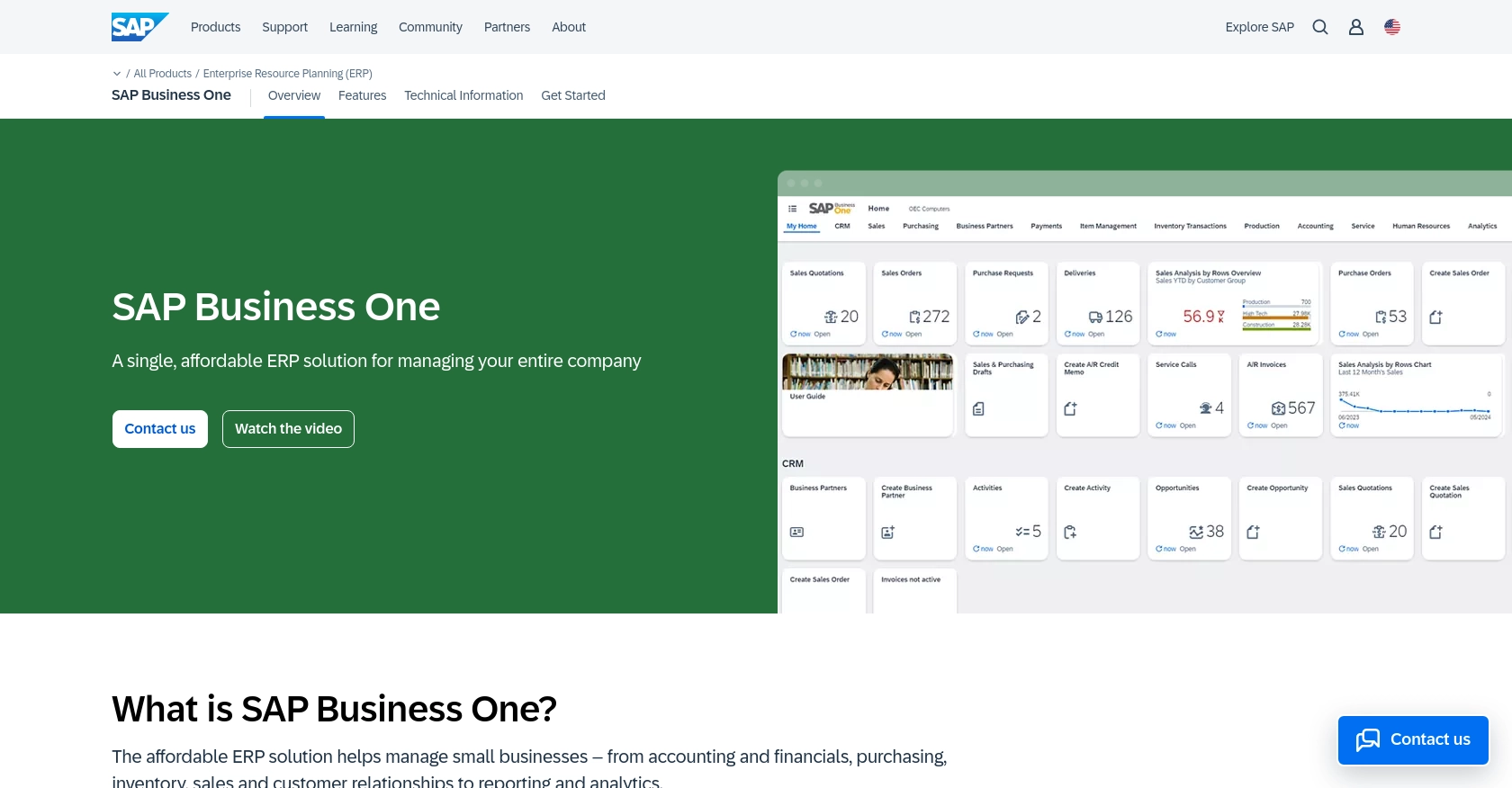
Introduction to SAP Business One
SAP Business One is a comprehensive enterprise resource planning (ERP) solution tailored for small and medium-sized businesses. It offers a wide range of functionalities, including financial management, sales, customer relationship management, and inventory control, all designed to streamline business operations.
Integrating with the SAP Business One API allows developers to access and manage critical business data programmatically. For example, retrieving invoices using the SAP Business One API in JavaScript can help automate financial reporting and analysis, enabling businesses to maintain accurate and up-to-date financial records effortlessly.
Setting Up Your SAP Business One Test/Sandbox Account
Before you can start interacting with the SAP Business One API, you need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data.
Creating a SAP Business One Sandbox Account
To begin, you need access to a SAP Business One sandbox environment. If your organization already uses SAP Business One, you can request access to a sandbox instance from your system administrator. If not, you may need to contact SAP directly or work with a SAP partner to set up a trial or demo environment.
Configuring API Access in SAP Business One
Once you have access to the sandbox, follow these steps to configure API access:
- Log in to the SAP Business One Service Layer using your sandbox credentials.
- Navigate to the Administration section and select Setup.
- Under General, find the Service Layer Configuration settings.
- Enable API access by configuring the necessary permissions and roles for your user account.
Generating API Credentials for SAP Business One
To authenticate API requests, you need to generate API credentials:
- In the Service Layer, navigate to the API Management section.
- Create a new API client by providing a name and description.
- Generate a client ID and client secret. Make sure to store these securely, as they will be used for authentication.
Authenticating with Custom Authentication
SAP Business One uses a custom authentication method. To authenticate, you will need to use the client ID and client secret obtained in the previous step. Refer to the SAP Business One Service Layer documentation for detailed instructions on implementing custom authentication.
With your sandbox account and API credentials set up, you're ready to start making API calls to retrieve invoices using JavaScript.
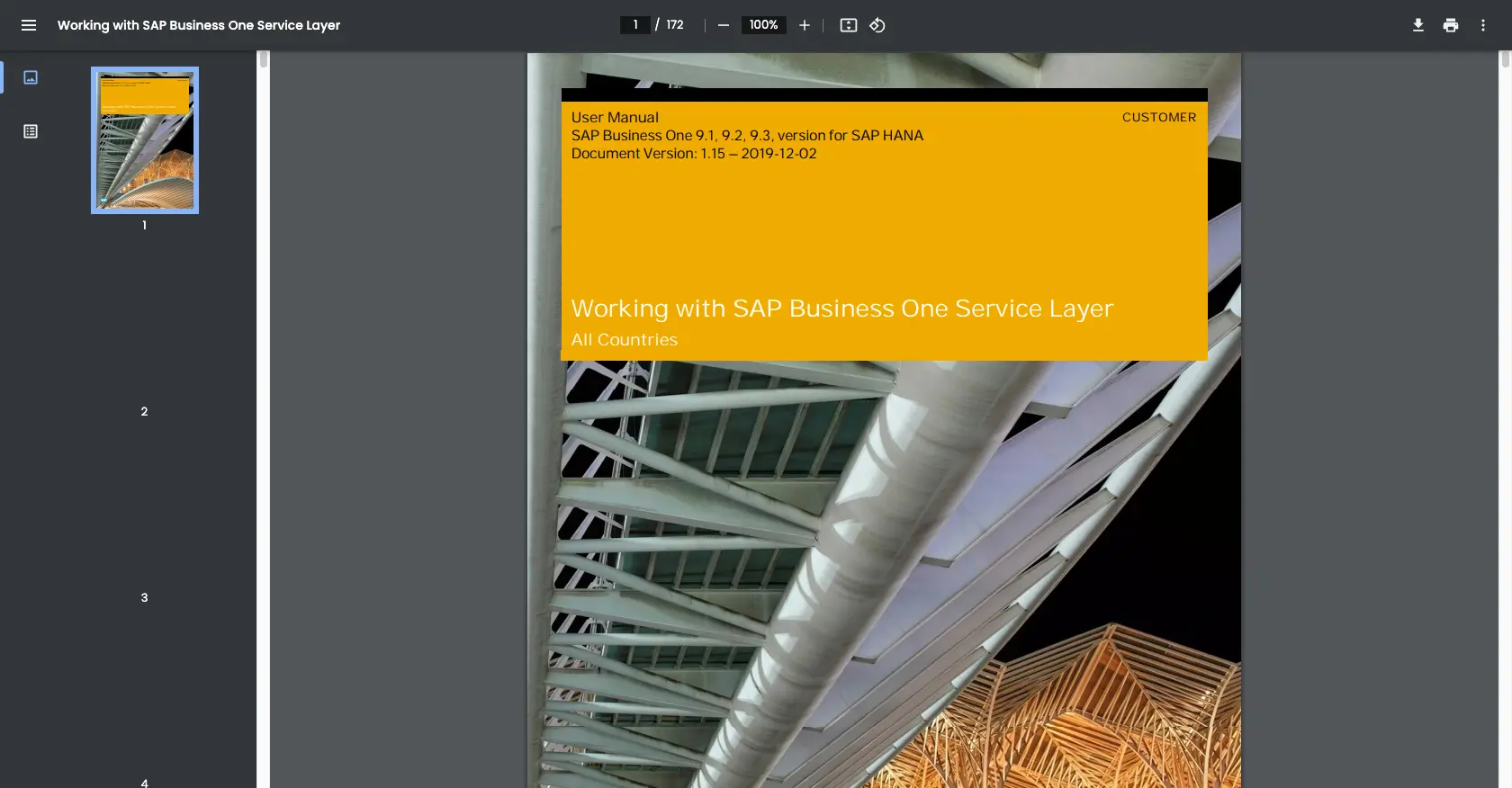
sbb-itb-96038d7
Making API Calls to Retrieve Invoices with SAP Business One in JavaScript
To interact with the SAP Business One API and retrieve invoices using JavaScript, you need to ensure that your development environment is properly set up. This section will guide you through the necessary steps, including setting up JavaScript, installing dependencies, and writing the code to make API calls.
Setting Up Your JavaScript Environment for SAP Business One API
Before you begin coding, make sure you have Node.js installed on your machine. Node.js provides the runtime environment for executing JavaScript code outside of a browser.
- Download and install Node.js from the official website.
- Verify the installation by running the following command in your terminal:
node -v
This command should return the version number of Node.js installed on your system.
Installing Required Dependencies for SAP Business One API Integration
To make HTTP requests to the SAP Business One API, you will need to install the Axios library, a popular promise-based HTTP client for JavaScript.
- Navigate to your project directory in the terminal.
- Run the following command to initialize a new Node.js project:
npm init -y
This command creates a package.json
file with default settings.
- Install Axios by running:
npm install axios
Writing JavaScript Code to Retrieve Invoices from SAP Business One
With your environment set up, you can now write the JavaScript code to make API calls to SAP Business One and retrieve invoices.
const axios = require('axios');
// Define the API endpoint and credentials
const endpoint = 'https://your-sap-business-one-instance.com/b1s/v1/Invoices';
const clientId = 'Your_Client_ID';
const clientSecret = 'Your_Client_Secret';
// Function to authenticate and retrieve invoices
async function getInvoices() {
try {
// Authenticate with SAP Business One
const authResponse = await axios.post('https://your-sap-business-one-instance.com/b1s/v1/Login', {
CompanyDB: 'Your_Company_DB',
UserName: clientId,
Password: clientSecret
});
// Extract the session ID from the authentication response
const sessionId = authResponse.data.SessionId;
// Set up headers with the session ID
const headers = {
'Cookie': `B1SESSION=${sessionId}`
};
// Make the API call to retrieve invoices
const response = await axios.get(endpoint, { headers });
// Log the retrieved invoices
console.log(response.data);
} catch (error) {
console.error('Error retrieving invoices:', error);
}
}
// Call the function to get invoices
getInvoices();
Replace Your_Client_ID
, Your_Client_Secret
, and Your_Company_DB
with your actual SAP Business One credentials and database name.
Verifying Successful API Calls and Handling Errors
After running the script, you should see the retrieved invoices logged in the console. To verify the success of the API call, check your SAP Business One sandbox environment to ensure the data matches the output.
In case of errors, the script will log the error details. Common issues may include incorrect credentials or network problems. Refer to the SAP Business One API Reference for detailed error codes and troubleshooting tips.
Best Practices for Using the SAP Business One API in JavaScript
When working with the SAP Business One API, it's essential to follow best practices to ensure efficient and secure integration. Here are some recommendations:
- Securely Store Credentials: Always store your client ID, client secret, and other sensitive information securely. Consider using environment variables or a secure vault to manage these credentials.
- Handle Rate Limiting: Be aware of any rate limits imposed by the SAP Business One API. Implement logic to handle rate limit responses gracefully, such as retrying requests after a delay.
- Standardize Data Fields: Ensure that data retrieved from the API is transformed and standardized to fit your application's data model. This will help maintain consistency across your systems.
- Error Handling: Implement robust error handling to manage unexpected API responses. Log errors for troubleshooting and provide meaningful feedback to users when issues occur.
Streamline Your Integrations with Endgrate
Building and maintaining integrations can be time-consuming and complex. With Endgrate, you can simplify this process by leveraging a unified API endpoint that connects to multiple platforms, including SAP Business One.
Endgrate allows you to focus on your core product by outsourcing integrations, saving both time and resources. With its intuitive integration experience, you can build once for each use case and easily extend support to other platforms.
Explore how Endgrate can enhance your integration capabilities and streamline your development process by visiting Endgrate today.
Read More
Ready to get started?