Using the Zoho Books API to Get Vendors in Python
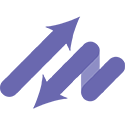
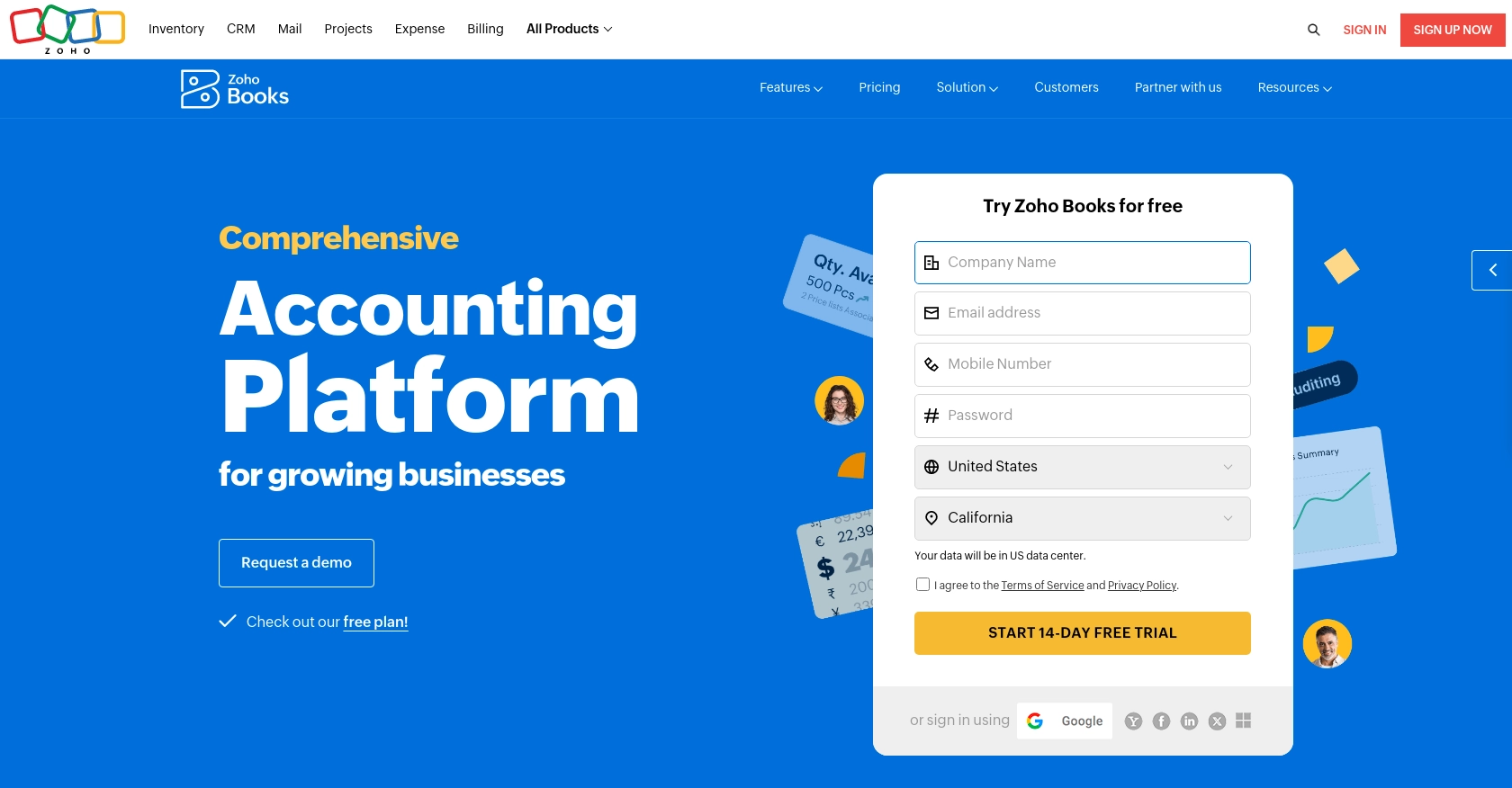
Introduction to Zoho Books API Integration
Zoho Books is a comprehensive cloud-based accounting software designed to streamline financial operations for businesses of all sizes. It offers a wide range of features including invoicing, expense tracking, and financial reporting, making it a popular choice for businesses seeking efficient financial management solutions.
Integrating with the Zoho Books API allows developers to automate and enhance various accounting processes. For example, accessing vendor information through the API can help businesses manage supplier relationships more effectively. By retrieving vendor data, developers can automate tasks such as updating vendor records or generating reports, thereby improving operational efficiency.
This article will guide you through using Python to interact with the Zoho Books API to retrieve vendor information. You'll learn how to set up your environment, authenticate with Zoho Books, and execute API calls to access vendor data.
Setting Up Your Zoho Books Test/Sandbox Account
Before you can start interacting with the Zoho Books API using Python, you'll need to set up a Zoho Books account. This section will guide you through creating a test or sandbox account, which is essential for development and testing purposes.
Creating a Zoho Books Account
If you don't already have a Zoho Books account, you can sign up for a free trial on the Zoho Books website. This will give you access to the necessary features to test API interactions.
- Visit the Zoho Books website.
- Click on the "Free Trial" button and follow the registration process.
- Once registered, log in to your Zoho Books account.
Registering a New Client for OAuth Authentication
Zoho Books uses OAuth 2.0 for authentication. To access the API, you'll need to register your application to obtain a Client ID and Client Secret.
- Go to the Zoho Developer Console.
- Click on "Add Client ID" and fill in the required details.
- After registration, you'll receive your Client ID and Client Secret. Keep these credentials secure.
Generating an OAuth Access Token
With your Client ID and Client Secret, you can generate an OAuth access token to authenticate API requests.
- Redirect to the authorization URL with the necessary parameters:
- After user consent, you'll receive a code in the redirect URI. Use this code to request an access token:
- Store the access token securely for use in API requests.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.contacts.READ,ZohoBooks.contacts.CREATE&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI&access_type=offline
https://accounts.zoho.com/oauth/v2/token?code=YOUR_CODE&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
For more detailed information on OAuth setup, refer to the Zoho Books OAuth documentation.
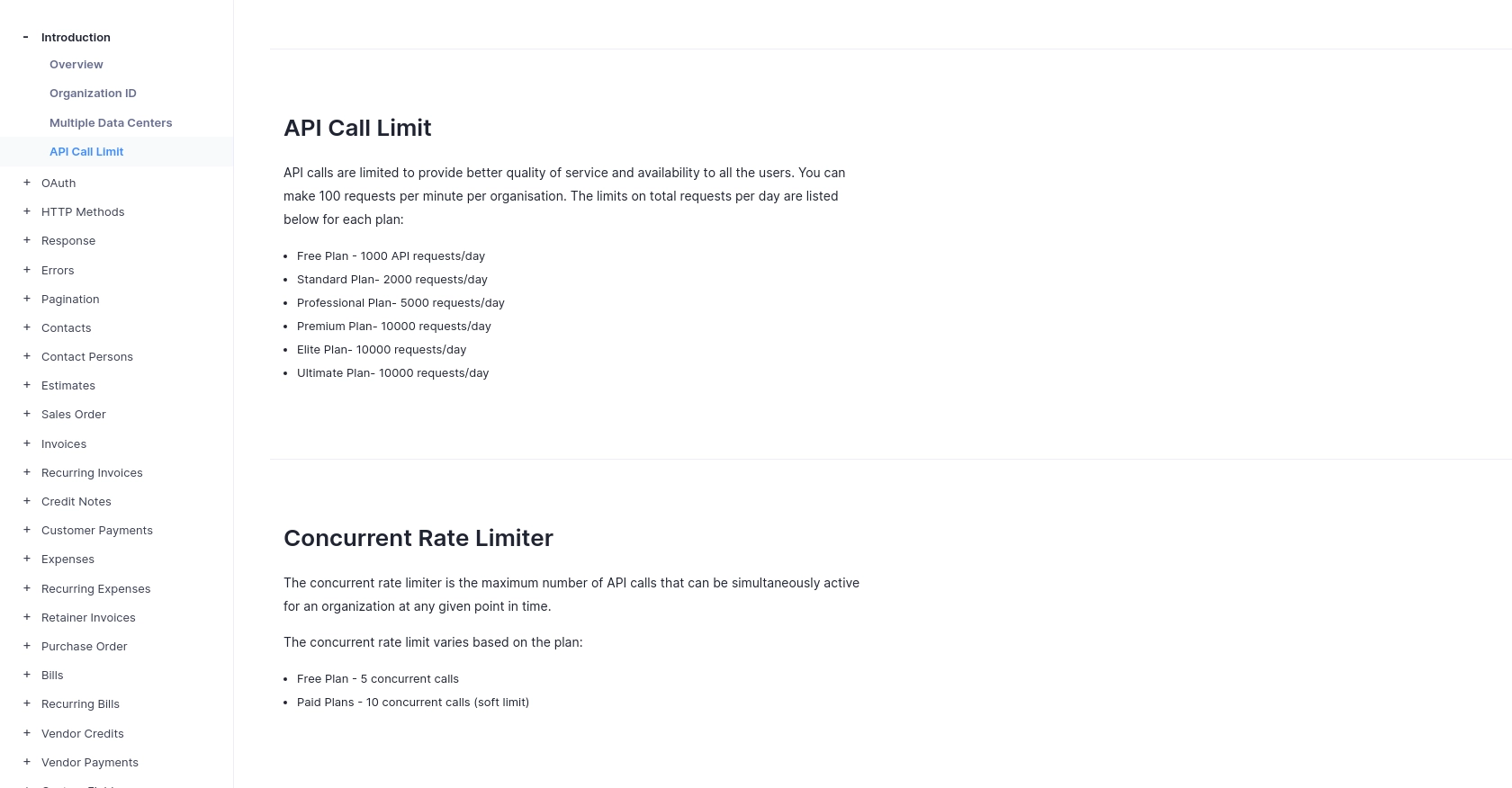
sbb-itb-96038d7
Executing API Calls to Retrieve Vendor Information from Zoho Books Using Python
Now that you have set up your Zoho Books account and obtained the necessary OAuth credentials, it's time to make API calls to retrieve vendor information. This section will guide you through the process of setting up your Python environment, installing necessary dependencies, and executing API calls to interact with the Zoho Books API.
Setting Up Your Python Environment for Zoho Books API Integration
To begin, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. You will also need the requests
library to handle HTTP requests.
- Verify your Python installation by running
python3 --version
in your terminal. - Install the
requests
library using pip:
pip3 install requests
Making API Calls to Retrieve Vendor Data from Zoho Books
With your environment ready, you can now write a Python script to interact with the Zoho Books API and retrieve vendor information. The following code demonstrates how to make a GET request to the Zoho Books API to fetch vendor data.
import requests
# Define the API endpoint and headers
endpoint = "https://www.zohoapis.com/books/v3/contacts"
headers = {
"Authorization": "Zoho-oauthtoken YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
# Set the parameters to filter by contact type 'vendors'
params = {
"organization_id": "YOUR_ORGANIZATION_ID",
"contact_type": "vendors"
}
# Make the GET request to the API
response = requests.get(endpoint, headers=headers, params=params)
# Check if the request was successful
if response.status_code == 200:
vendors = response.json().get("contacts", [])
for vendor in vendors:
print(f"Vendor Name: {vendor['contact_name']}")
else:
print(f"Failed to retrieve vendors: {response.status_code} - {response.text}")
Replace YOUR_ACCESS_TOKEN
and YOUR_ORGANIZATION_ID
with your actual access token and organization ID. The script filters contacts by setting the contact_type
parameter to 'vendors'.
Verifying API Call Success and Handling Errors
After executing the script, you should see a list of vendor names printed in the console. If the request fails, the script will output the error code and message. Common HTTP status codes include:
- 200: OK - The request was successful.
- 400: Bad Request - The request was invalid.
- 401: Unauthorized - Invalid authentication token.
- 429: Rate Limit Exceeded - Too many requests.
For more detailed error information, refer to the Zoho Books Error Documentation.
By following these steps, you can efficiently retrieve vendor information from Zoho Books using Python, enabling you to automate and streamline your accounting processes.
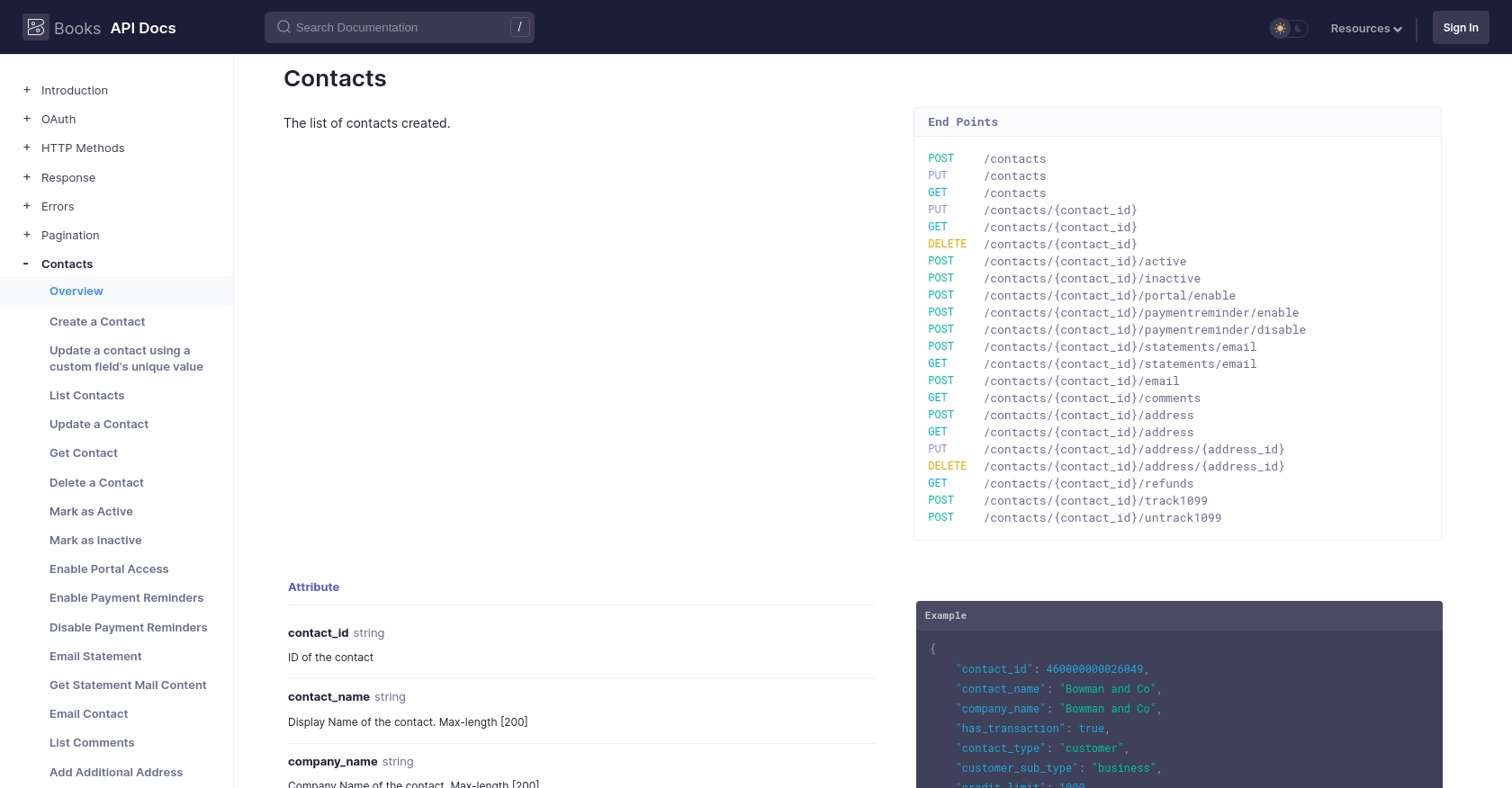
Conclusion: Best Practices for Zoho Books API Integration
Integrating with the Zoho Books API using Python provides a powerful way to automate and enhance your financial management processes. By following the steps outlined in this guide, you can efficiently retrieve vendor information and streamline your accounting tasks.
Securely Storing Zoho Books API Credentials
Always ensure that your OAuth credentials, such as the Client ID, Client Secret, and access tokens, are stored securely. Avoid hardcoding them in your scripts. Instead, use environment variables or secure vaults to manage sensitive information.
Handling Zoho Books API Rate Limits
Zoho Books imposes rate limits to ensure fair usage of its API. You can make up to 100 requests per minute per organization. Exceeding this limit will result in a 429 status code. Implementing retry logic and exponential backoff can help manage rate limits effectively. For more details, refer to the Zoho Books API Call Limit Documentation.
Data Transformation and Standardization
When working with vendor data, consider transforming and standardizing fields to match your application's requirements. This ensures consistency and accuracy across your systems.
Leverage Endgrate for Seamless Integration
For developers looking to simplify their integration processes, Endgrate offers a unified API solution that connects to multiple platforms, including Zoho Books. By using Endgrate, you can save time and resources, allowing you to focus on your core product development. Explore how Endgrate can enhance your integration experience by visiting Endgrate.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/contacts/#overview
Ready to get started?