Using the Avalara API to Create Invoices in Javascript
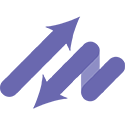
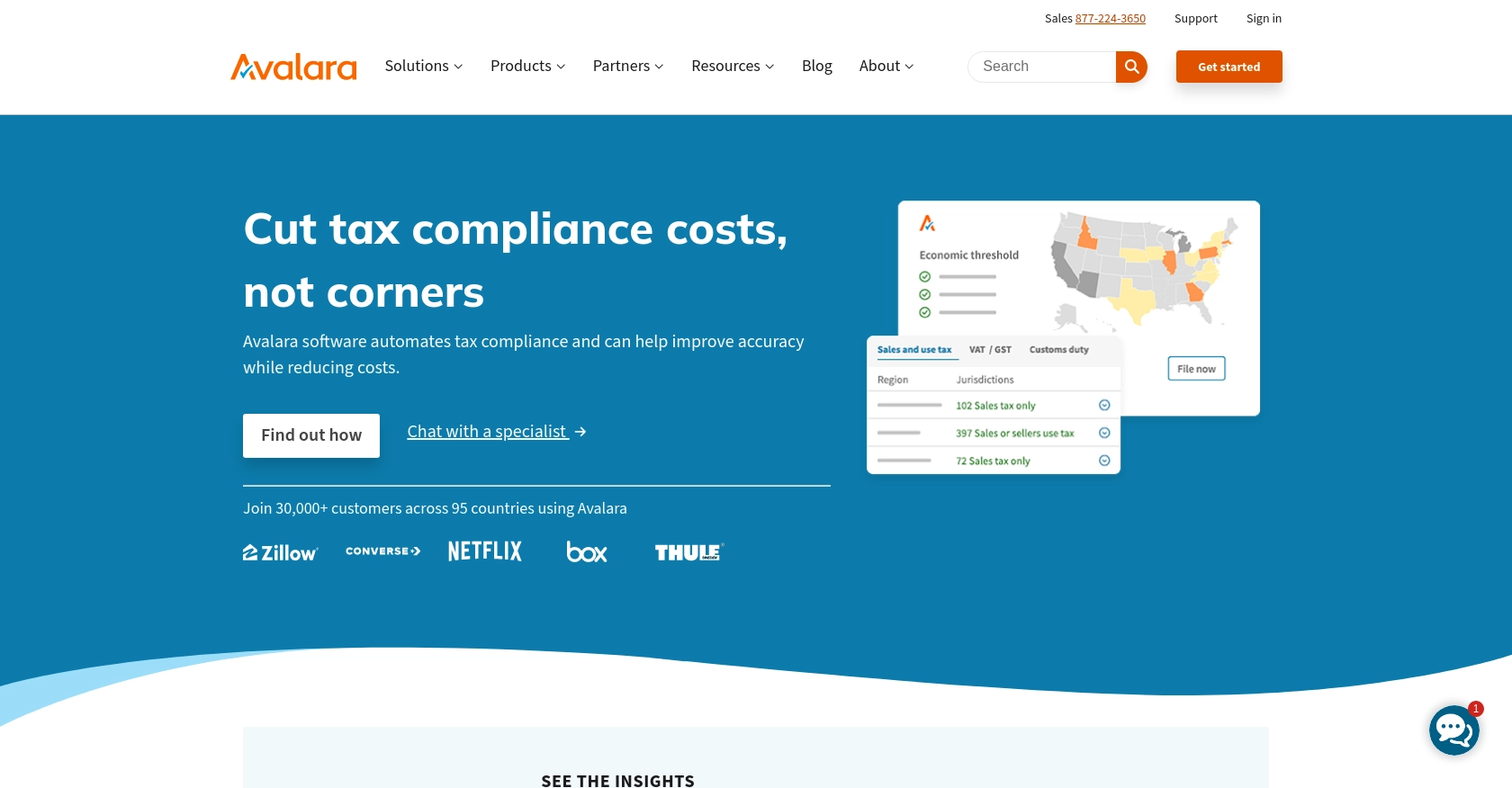
Introduction to Avalara and Its API for Invoice Creation
Avalara is a renowned provider of tax compliance solutions, offering a comprehensive suite of tools to automate tax calculations, returns, and compliance for businesses across various industries. With its robust AvaTax API, Avalara simplifies the complexities of tax management, making it an essential tool for businesses looking to streamline their tax processes.
Developers may want to integrate with Avalara's API to automate the creation of invoices, ensuring accurate tax calculations and compliance with local tax regulations. For example, a developer could use the Avalara API to generate invoices directly from an e-commerce platform, automatically applying the correct tax rates based on the customer's location and the nature of the transaction.
This article will guide you through using JavaScript to interact with the Avalara API, focusing on creating invoices efficiently and accurately. By leveraging Avalara's capabilities, developers can enhance their applications with seamless tax compliance features.
Setting Up Your Avalara Sandbox Account for API Integration
Before diving into the integration process with Avalara's API, it's crucial to set up a sandbox account. This allows developers to test API calls without affecting live data, ensuring a smooth and error-free integration.
Creating an Avalara Sandbox Account
To begin, you'll need to create a sandbox account on Avalara's platform. Follow these steps:
- Visit the Avalara Developer Portal and sign up for a developer account.
- Once registered, navigate to the sandbox environment section and create a new sandbox account.
- Fill in the necessary details and submit your request. You will receive an email confirmation with your sandbox credentials.
Generating API Credentials for Avalara Integration
After setting up your sandbox account, you'll need to generate API credentials to authenticate your requests:
- Log in to your Avalara sandbox account.
- Go to the "Settings" section and select "API Credentials."
- Click on "Generate License Key" to create a new license key. Remember, this key is essential for API authentication.
- Note down your account ID and the newly generated license key. These will be used to authenticate your API calls.
Understanding Avalara's Basic Authentication
Avalara uses Basic HTTP Authentication for its API. Here's how to set it up:
- Combine your account ID and license key in the format:
accountid:licensekey
. - Encode this string using Base64 encoding.
- Use the encoded string as the value for the "Authorization" header in your API requests, prefixed with "Basic".
For more details on authentication, refer to the Avalara Authentication Documentation.
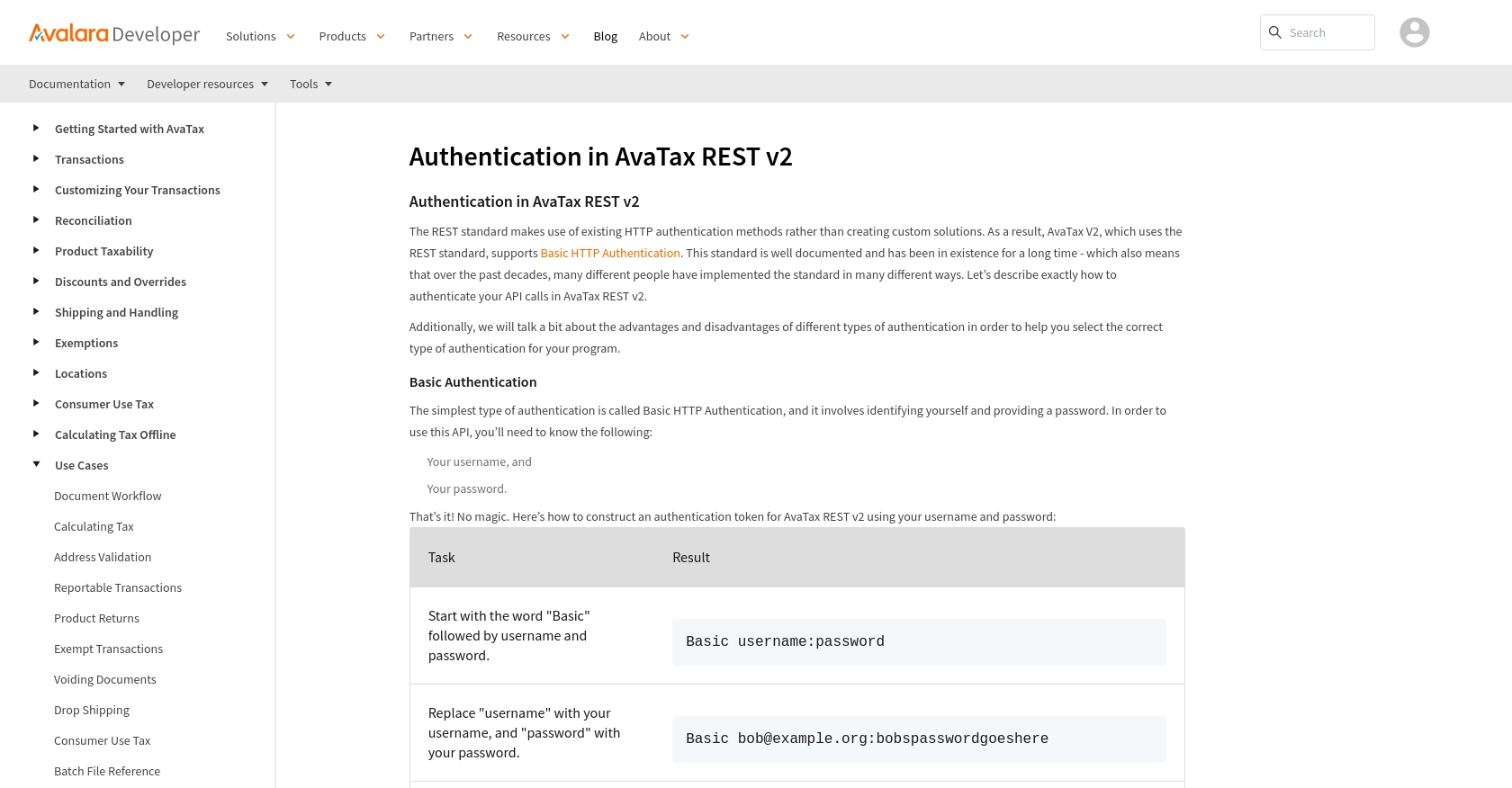
sbb-itb-96038d7
Making API Calls to Create Invoices with Avalara Using JavaScript
To effectively create invoices using the Avalara API with JavaScript, you'll need to set up your development environment and understand the necessary API endpoints and request structures. This section will guide you through the process of making API calls to Avalara's AvaTax service.
Setting Up Your JavaScript Environment for Avalara API Integration
Before making API calls, ensure your JavaScript environment is ready. You'll need Node.js and npm installed on your machine. Follow these steps to set up your environment:
- Install Node.js from the official website if you haven't already.
- Create a new project directory and navigate into it using your terminal.
- Initialize a new Node.js project by running
npm init -y
. - Install the Axios library for making HTTP requests by running
npm install axios
.
Understanding the Avalara CreateTransaction API Endpoint
The Avalara CreateTransaction API endpoint is used to record a new transaction, such as an invoice. This endpoint calculates the appropriate taxes based on the transaction details provided. For more information, refer to the Avalara CreateTransaction API Documentation.
Example Code to Create an Invoice Using Avalara API in JavaScript
Below is an example of how to create an invoice using the Avalara API with JavaScript:
const axios = require('axios');
// Set your Avalara account credentials
const accountId = 'your_account_id';
const licenseKey = 'your_license_key';
// Encode credentials for Basic Authentication
const auth = Buffer.from(`${accountId}:${licenseKey}`).toString('base64');
// Define the API endpoint
const endpoint = 'https://sandbox-rest.avatax.com/api/v2/transactions/create';
// Create the invoice data
const invoiceData = {
type: 'SalesInvoice',
companyCode: 'DEFAULT',
date: '2024-09-06',
customerCode: 'ABC',
purchaseOrderNo: '2024-09-06-001',
addresses: {
singleLocation: {
line1: '2000 Main Street',
city: 'Irvine',
region: 'CA',
country: 'US',
postalCode: '92614'
}
},
lines: [
{
number: '1',
quantity: 1,
amount: 100,
taxCode: 'PS081282',
itemCode: 'Y0001',
description: 'Yarn'
}
],
commit: true,
currencyCode: 'USD',
description: 'Yarn'
};
// Make the API call
axios.post(endpoint, invoiceData, {
headers: {
'Authorization': `Basic ${auth}`,
'Content-Type': 'application/json'
}
})
.then(response => {
console.log('Invoice Created Successfully:', response.data);
})
.catch(error => {
console.error('Error Creating Invoice:', error.response.data);
});
Verifying the Success of Your API Call in Avalara Sandbox
After running the above code, check your Avalara sandbox account to verify that the invoice was created successfully. You should see the transaction details reflected in your sandbox environment.
Handling Errors and Understanding Avalara API Error Codes
When making API calls, it's crucial to handle potential errors. Avalara provides detailed error codes to help diagnose issues. If an error occurs, inspect the response to understand the cause. Common issues include authentication errors or incorrect data formats. For a complete list of error codes, refer to the Avalara Authentication Documentation.
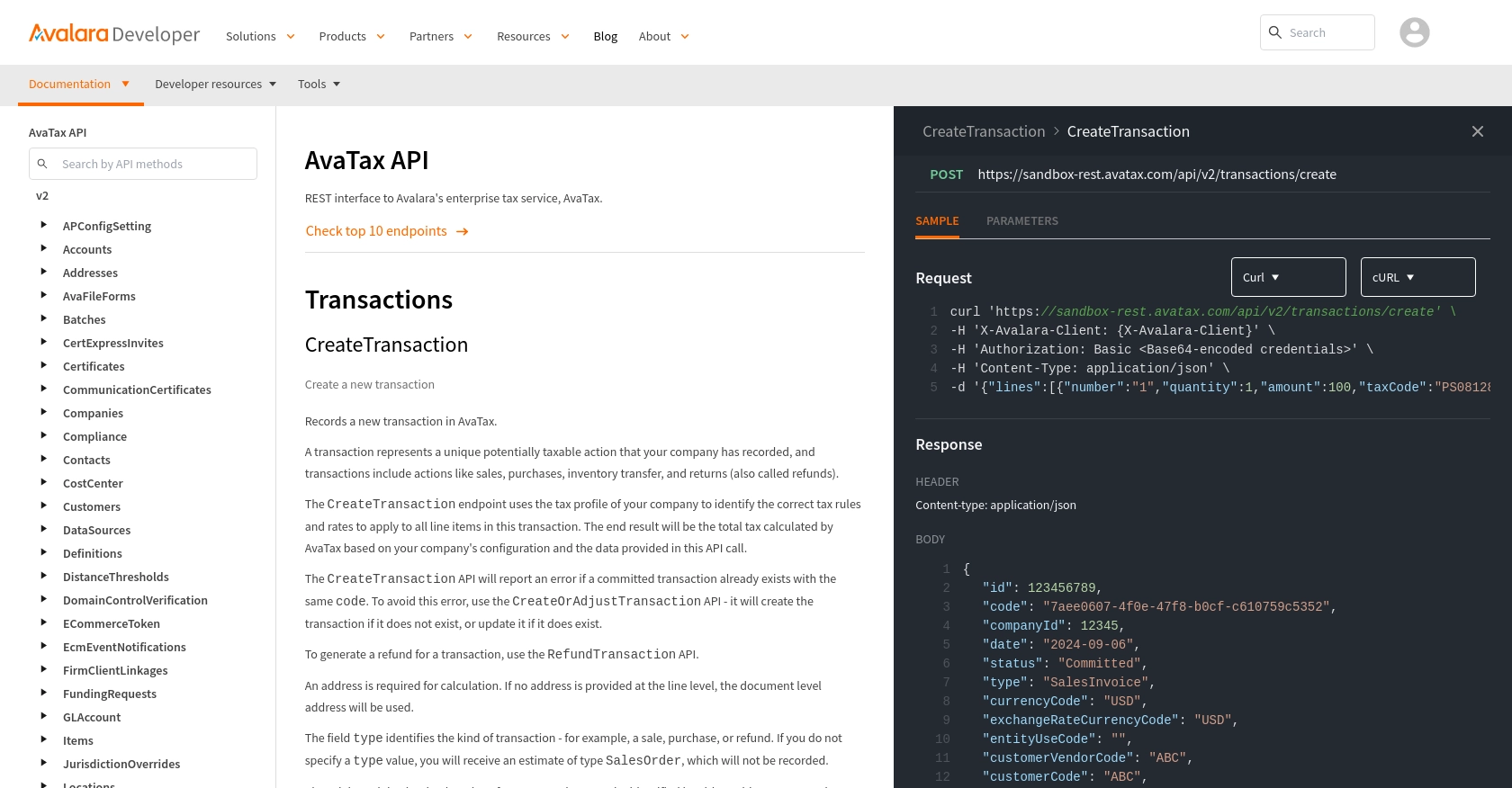
Conclusion and Best Practices for Using Avalara API in JavaScript
Integrating Avalara's API into your JavaScript applications can significantly enhance your ability to manage tax compliance efficiently. By automating invoice creation and tax calculations, you can ensure accuracy and streamline your business processes.
Best Practices for Storing Avalara API Credentials Securely
When handling sensitive information such as API credentials, it's crucial to follow best practices to ensure security:
- Store credentials in environment variables rather than hardcoding them in your source code.
- Use secure storage solutions like AWS Secrets Manager or Azure Key Vault for managing sensitive data.
- Regularly rotate your API keys and credentials to minimize security risks.
Handling Avalara API Rate Limiting and Error Management
Understanding and managing API rate limits is essential for maintaining a smooth integration:
- Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Monitor your API usage to ensure you stay within the allowed limits.
- Refer to Avalara's documentation for detailed error codes and handling strategies.
Transforming and Standardizing Data for Avalara API Integration
To ensure seamless integration, consider the following data management practices:
- Standardize data formats, such as dates and currency codes, to match Avalara's API requirements.
- Validate data before sending it to the API to prevent errors and ensure compliance.
- Use data transformation tools or libraries to automate the process of data standardization.
Enhancing Integration Efficiency with Endgrate
For developers looking to streamline multiple integrations, Endgrate offers a powerful solution. By providing a unified API endpoint, Endgrate simplifies the process of connecting to various platforms, including Avalara. This allows you to focus on your core product while ensuring a seamless integration experience for your customers.
Explore how Endgrate can save you time and resources by visiting Endgrate and discover the benefits of outsourcing your integration needs.
Read More
Ready to get started?