Using the Asana API to Get Tasks in Python
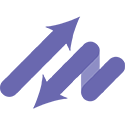
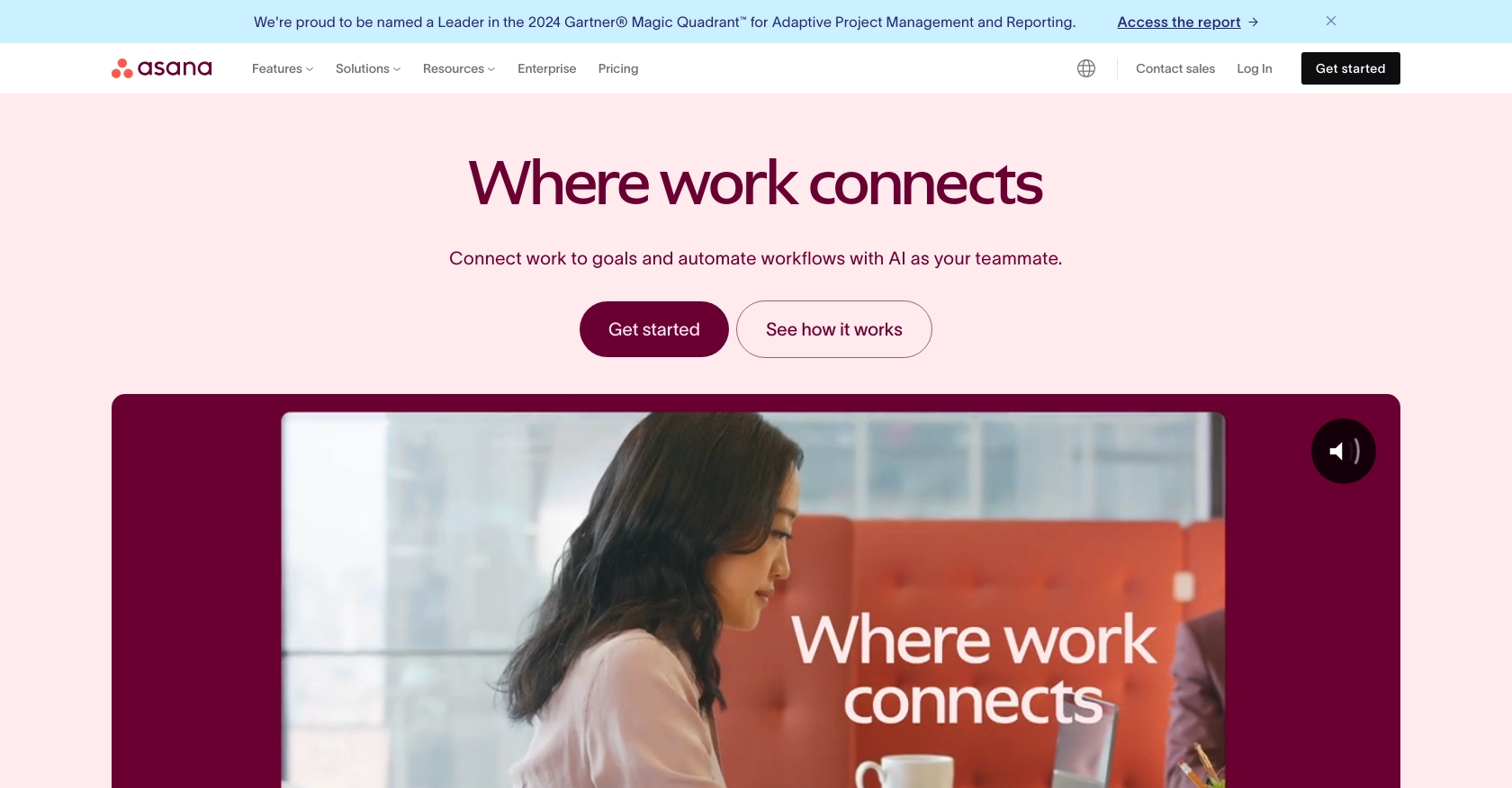
Introduction to Asana API Integration
Asana is a powerful project management tool that helps teams organize, track, and manage their work. With its intuitive interface and robust features, Asana is widely used by businesses to enhance productivity and collaboration across various projects and tasks.
Integrating with the Asana API allows developers to automate and streamline task management processes. For example, a developer might use the Asana API to retrieve tasks and automatically update them in a custom dashboard, enabling real-time tracking and reporting of project progress.
Setting Up Your Asana Developer Account for API Integration
Before you can start interacting with the Asana API, you'll need to set up a developer account and create an application for testing purposes. This setup allows you to authenticate your API requests and access Asana's features programmatically.
Creating an Asana Developer Account
If you don't already have an Asana account, you can sign up for a free account on the Asana website. Once your account is created, log in to access the Asana dashboard.
Accessing the Asana Developer Console
To begin working with the Asana API, navigate to the Asana Developer Console. Here, you can manage your applications and generate the necessary credentials for API access.
Creating an Asana App for OAuth Authentication
- In the Developer Console, click on Create New App.
- Fill in the required details, such as the app name and redirect URL. The redirect URL is crucial for OAuth authentication.
- Once your app is created, you'll be provided with a Client ID and Client Secret. Keep these credentials secure as they are essential for authenticating your API requests.
Generating an OAuth Access Token
Asana uses OAuth 2.0 for authentication, which allows users to authorize your app to access their data without sharing their credentials. Follow these steps to generate an access token:
- Direct users to Asana's authorization URL, including your app's Client ID and redirect URL.
- Upon user approval, Asana will redirect to your specified URL with an authorization code.
- Exchange this authorization code for an access token by making a POST request to Asana's token endpoint, using your Client ID and Client Secret.
For detailed instructions, refer to the Asana OAuth documentation.
Testing Your Asana API Setup
With your access token, you can now make API calls to Asana. It's a good practice to test your setup by retrieving some basic data, such as a list of tasks, to ensure everything is working correctly.
For more information on making API calls, visit the Asana API documentation.
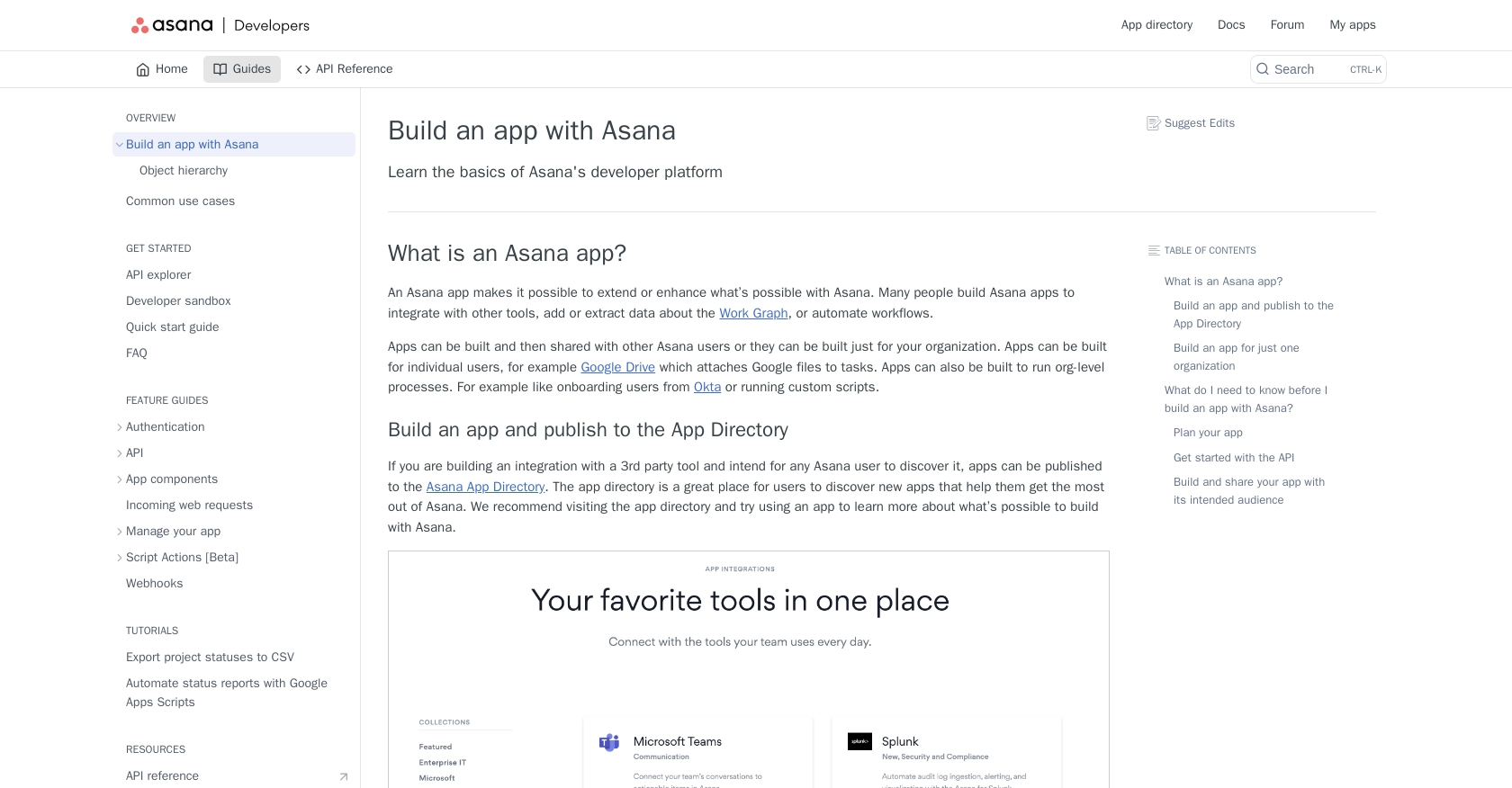
sbb-itb-96038d7
Making API Calls to Retrieve Asana Tasks Using Python
To interact with the Asana API and retrieve tasks, you'll need to use Python, a versatile programming language known for its simplicity and readability. This section will guide you through the process of setting up your environment, writing the necessary code, and executing API calls to fetch tasks from Asana.
Setting Up Your Python Environment for Asana API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python from the official website if you haven't already.
- Use pip to install the
requests
library by running the following command in your terminal:
pip install requests
Writing Python Code to Fetch Tasks from Asana
With your environment set up, you can now write a Python script to retrieve tasks from Asana. Create a file named get_asana_tasks.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://app.asana.com/api/1.0/tasks"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Parse the JSON data from the response
data = response.json()
# Loop through the tasks and print their information
for task in data["data"]:
print(task["name"], "-", task["gid"])
Replace YOUR_ACCESS_TOKEN
with the OAuth access token you obtained earlier. This script sends a GET request to Asana's API endpoint to fetch tasks and prints their names and IDs.
Executing the Python Script to Retrieve Asana Tasks
Run the script from your terminal using the following command:
python get_asana_tasks.py
If successful, you should see a list of task names and their corresponding IDs printed in the terminal. This confirms that your API call to Asana was successful.
Handling Errors and Verifying API Call Success
It's crucial to handle potential errors when making API calls. You can check the response status code to ensure the request was successful:
if response.status_code == 200:
print("Tasks retrieved successfully.")
else:
print("Failed to retrieve tasks. Status code:", response.status_code)
Refer to the Asana API documentation for more details on error codes and handling.
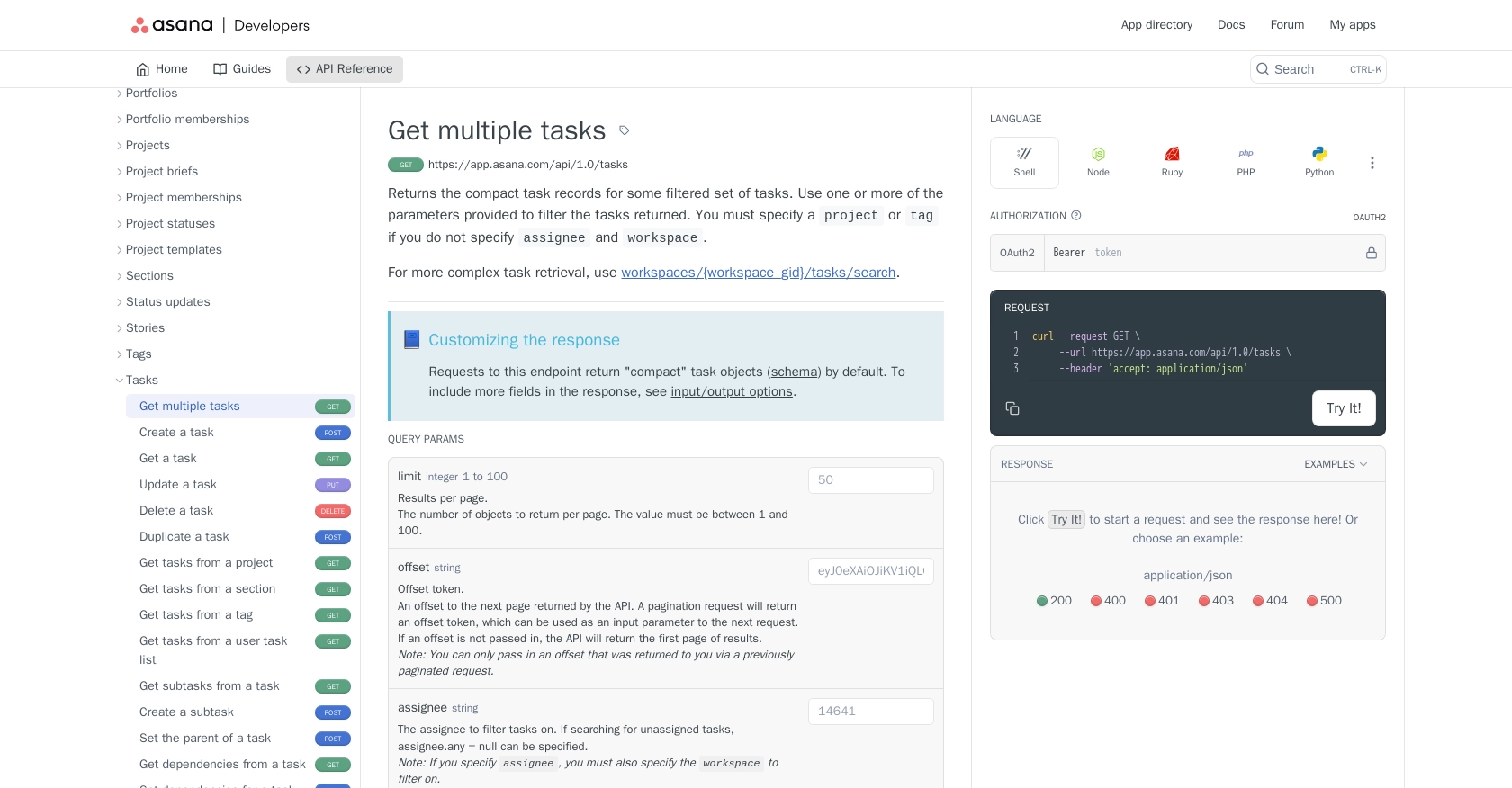
Conclusion and Best Practices for Asana API Integration
Integrating with the Asana API using Python provides a powerful way to automate and enhance your project management workflows. By retrieving tasks programmatically, you can streamline processes, improve productivity, and maintain real-time visibility into project progress.
Best Practices for Secure and Efficient Asana API Usage
- Securely Store Credentials: Always store your OAuth tokens and client secrets securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Asana's API has rate limits to ensure fair usage. Monitor the response headers for rate limit information and implement retry logic with exponential backoff to handle rate limit errors gracefully. For more details, refer to the Asana API documentation.
- Standardize Data Fields: When retrieving tasks, ensure that the data fields are standardized to match your application's requirements. This will facilitate seamless integration and data consistency across platforms.
Enhance Your Integration Strategy with Endgrate
While building custom integrations with Asana can be rewarding, it can also be time-consuming and complex. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to multiple platforms, including Asana. This allows you to focus on your core product while outsourcing the intricacies of integration development.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Explore how Endgrate can simplify your integration strategy by visiting Endgrate.
Read More
Ready to get started?