How to Get Accounts with the Front API in Python
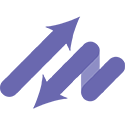
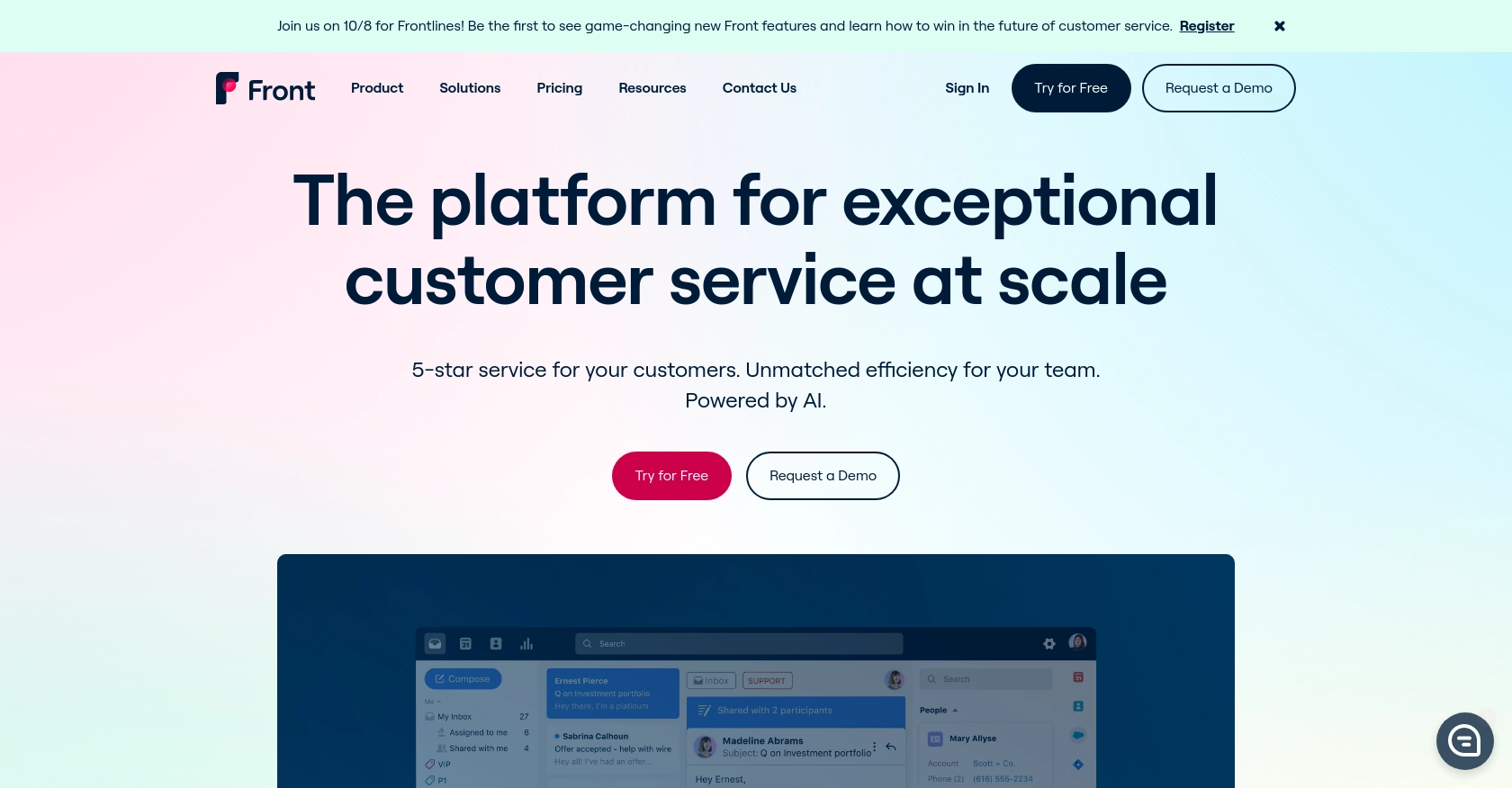
Introduction to Front API Integration
Front is a powerful communication platform that centralizes emails, messages, and other communication channels into a single collaborative workspace. It is designed to enhance team productivity by streamlining communication and providing a unified interface for managing customer interactions.
Integrating with Front's API allows developers to automate and enhance their workflows by accessing and managing various resources within Front. For example, a developer might want to retrieve account information to synchronize customer data between Front and an external CRM system, ensuring that all team members have access to the most up-to-date information.
Setting Up a Test or Sandbox Account for Front API Integration
Before you can start interacting with the Front API, you'll need to set up a test or sandbox account. This environment allows you to experiment with API calls without affecting live data, making it ideal for development and testing purposes.
Creating a Front Developer Account
If you don't already have a Front account, you can create a developer account by signing up on the Front website. This account will provide you with access to a free developer environment, which includes all the tools you need to begin building with Front's APIs.
- Visit the Front Developer Portal and sign up for a developer account.
- Follow the instructions to complete the registration process.
- Once your account is created, you'll have access to the developer dashboard.
Generating an API Token for Authentication
Front uses API tokens for authentication, allowing you to securely access their API. Here's how to generate an API token:
- Log in to your Front developer account.
- Navigate to the API tokens section in the dashboard.
- Click on "Create API Token" and provide a name for your token.
- Select the appropriate scopes for your token, such as "Shared Resources," to ensure you have the necessary permissions.
- Click "Generate" to create your token. Make sure to copy and store it securely, as you'll need it for API requests.
With your developer account and API token set up, you're ready to start making API calls to Front. In the next section, we'll explore how to use Python to interact with the Front API and retrieve account information.
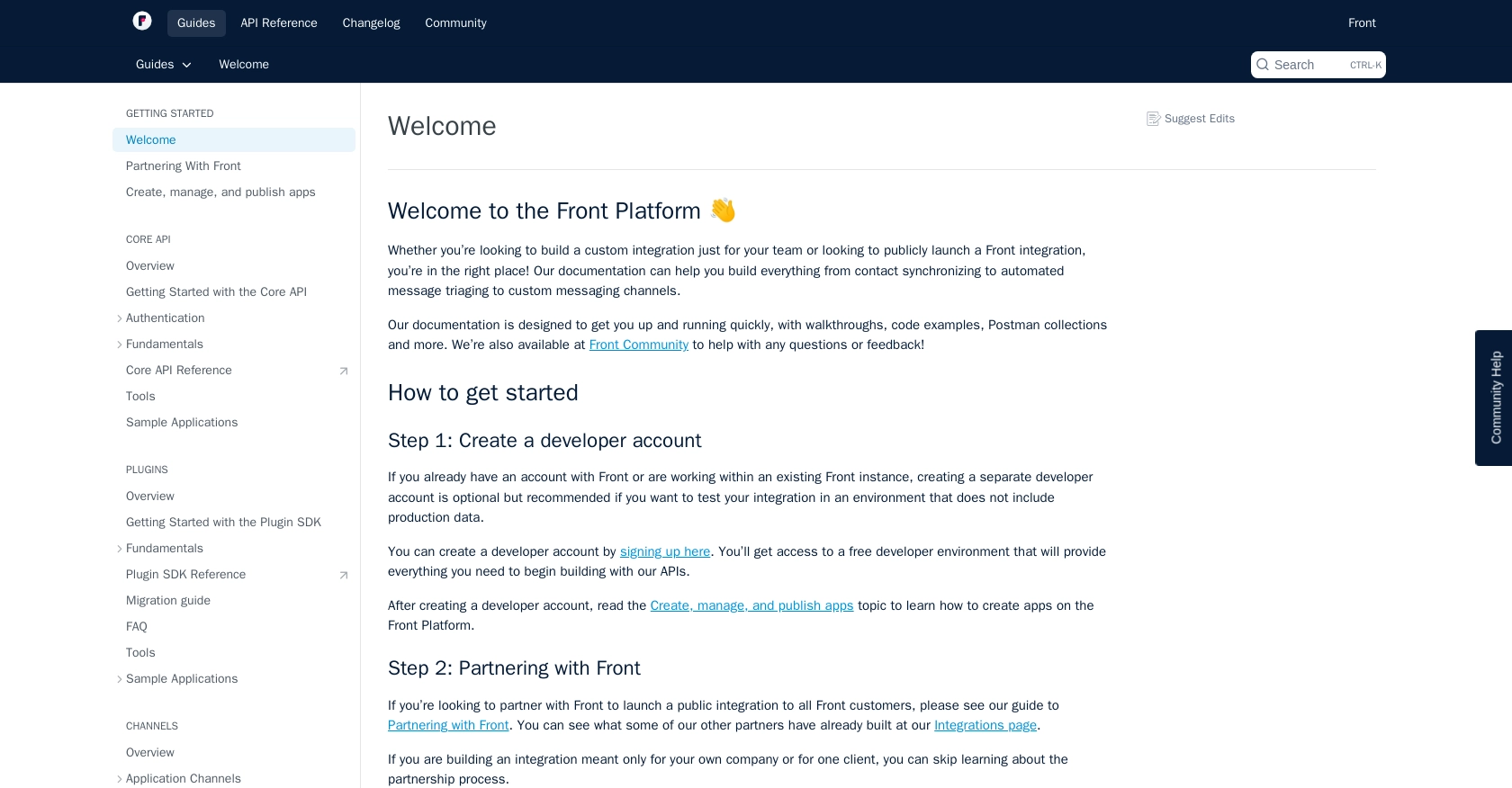
sbb-itb-96038d7
Using Python to Make API Calls to Front for Account Retrieval
Python is a versatile programming language that is widely used for web development, data analysis, and automation tasks. In this section, we'll walk through the steps to interact with the Front API using Python to retrieve account information. We'll cover the necessary setup, code implementation, and how to handle responses and errors.
Prerequisites for Front API Integration with Python
Before proceeding, ensure you have the following installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Once you have these prerequisites, install the requests
library, which will be used to make HTTP requests to the Front API:
pip install requests
Writing Python Code to Retrieve Accounts from Front API
Let's create a Python script to retrieve account information from Front. Follow these steps to set up and execute the API call:
import requests
# Define the API endpoint and headers
url = "https://api2.frontapp.com/accounts"
headers = {
"Authorization": "Bearer YOUR_API_TOKEN",
"Accept": "application/json"
}
# Make a GET request to the API
response = requests.get(url, headers=headers)
# Check if the request was successful
if response.status_code == 200:
accounts = response.json()
for account in accounts["_results"]:
print(f"Account ID: {account['id']}, Name: {account['name']}")
else:
print(f"Failed to retrieve accounts: {response.status_code} - {response.text}")
Replace YOUR_API_TOKEN
with the API token you generated earlier. This script sends a GET request to the Front API to list accounts and prints the account ID and name for each account retrieved.
Verifying Successful API Requests and Handling Errors
After running the script, you should see a list of accounts printed in the console. If the request fails, the script will output the status code and error message. Common error codes include:
- 401 Unauthorized: Check if your API token is correct and has the necessary permissions.
- 429 Too Many Requests: You have exceeded the rate limit. Refer to the Front API rate limiting documentation for more details.
To verify the accounts retrieved, you can cross-check the data in your Front developer dashboard.
Handling Pagination in Front API Responses
The Front API may return paginated results. To handle pagination, check for a _pagination
object in the response and use the next
URL to fetch additional pages:
while response.status_code == 200:
data = response.json()
for account in data["_results"]:
print(f"Account ID: {account['id']}, Name: {account['name']}")
next_page = data["_pagination"].get("next")
if not next_page:
break
response = requests.get(next_page, headers=headers)
This loop continues to fetch and print accounts until there are no more pages left.
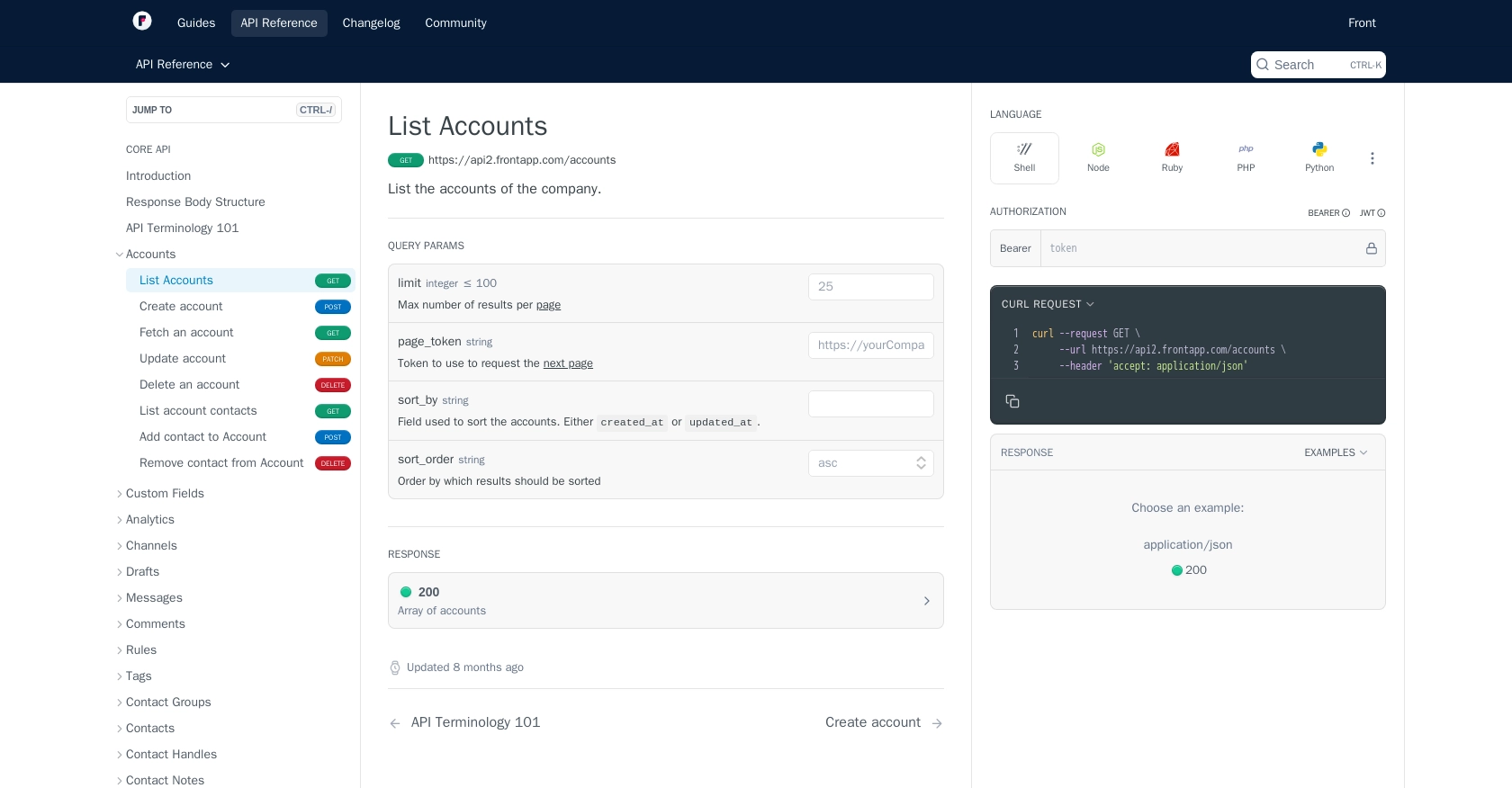
Conclusion and Best Practices for Front API Integration
Integrating with the Front API using Python allows developers to efficiently manage and synchronize account data, enhancing workflow automation and ensuring data consistency across platforms. By following the steps outlined in this guide, you can successfully retrieve account information and handle API responses, including pagination and error management.
Best Practices for Secure and Efficient Front API Usage
- Securely Store API Tokens: Always store your API tokens securely and avoid hardcoding them in your scripts. Consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of Front's rate limits, which start at 50 requests per minute. Implement logic to handle 429 errors by respecting the
Retry-After
header. For more details, refer to the Front API rate limiting documentation. - Implement Pagination: Ensure your application can handle paginated responses by checking for the
_pagination
object and using thenext
URL to fetch additional data. - Data Transformation: Consider transforming and standardizing data fields to maintain consistency across different systems.
Streamline Your Integration Process with Endgrate
While building integrations with the Front API can be rewarding, it can also be time-consuming and complex, especially when managing multiple integrations. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Front.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can transform your integration strategy by visiting Endgrate.
Read More
Ready to get started?