Using the Xero API to Get Vendors (with Javascript examples)
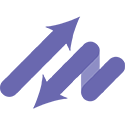
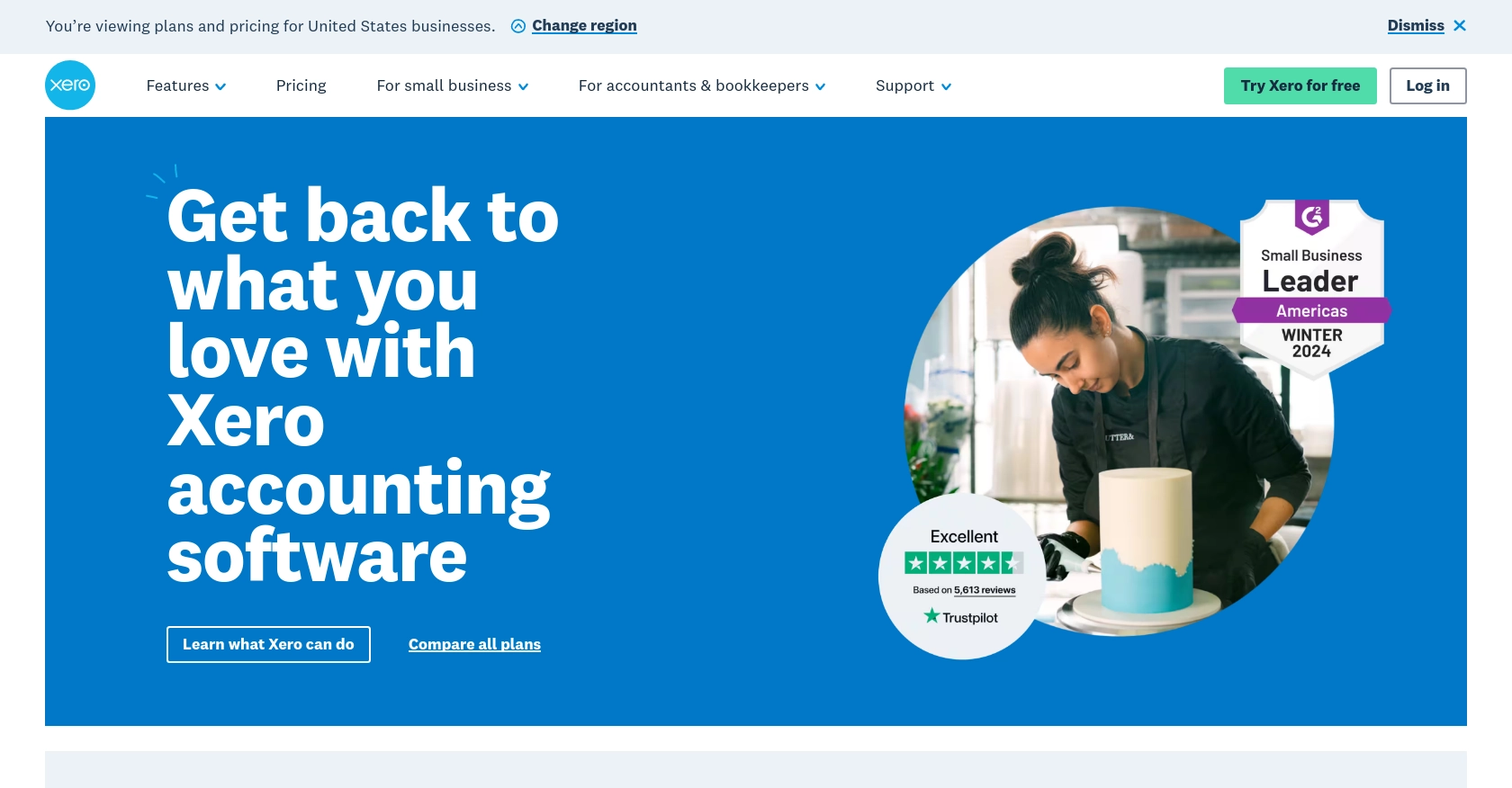
Introduction to Xero API Integration
Xero is a powerful cloud-based accounting software platform designed to help small and medium-sized businesses manage their finances efficiently. With features such as invoicing, payroll, and expense tracking, Xero provides a comprehensive solution for financial management.
Integrating with Xero's API allows developers to automate and streamline accounting processes, such as managing vendor information. For example, a developer might use the Xero API to retrieve a list of vendors, enabling seamless synchronization of supplier data with other business systems.
This article will guide you through using JavaScript to interact with the Xero API, specifically focusing on retrieving vendor information. By following this tutorial, you'll learn how to efficiently access and manage vendor data within the Xero platform.
Setting Up Your Xero Developer Account and Sandbox Environment
Before you can start interacting with the Xero API, you'll need to set up a developer account and create a sandbox environment. This will allow you to test your API calls without affecting live data. Follow these steps to get started:
Create a Xero Developer Account
- Visit the Xero Developer Portal and sign up for a developer account if you haven't already.
- Once registered, log in to your developer account to access the dashboard.
Create a Xero App for OAuth 2.0 Authentication
Xero uses OAuth 2.0 for authentication, which requires you to create an app to obtain the necessary credentials.
- In the Xero Developer Dashboard, navigate to the "My Apps" section and click on "New App."
- Fill in the required details such as the app name, company or organization, and a redirect URI (this can be a placeholder for testing).
- Once your app is created, you'll receive a client ID and client secret. Keep these credentials secure as they are essential for authenticating API requests.
Configure OAuth 2.0 Scopes and Permissions
To access vendor information, you'll need to set the appropriate scopes for your app:
- In your app settings, navigate to the "Scopes" section.
- Select the necessary scopes for accessing contacts, such as
accounting.contacts.read
. - Save your changes to ensure your app has the correct permissions.
Connect to a Xero Organization
To test your API calls, you'll need to connect your app to a Xero organization:
- Use the OAuth 2.0 authorization flow to connect your app to a Xero organization. This involves directing users to Xero's authorization URL and handling the callback to exchange the authorization code for an access token.
- Refer to the Xero OAuth 2.0 Authorization Flow Guide for detailed steps.
With your Xero developer account, app, and sandbox environment set up, you're ready to start making API calls to retrieve vendor information using JavaScript.
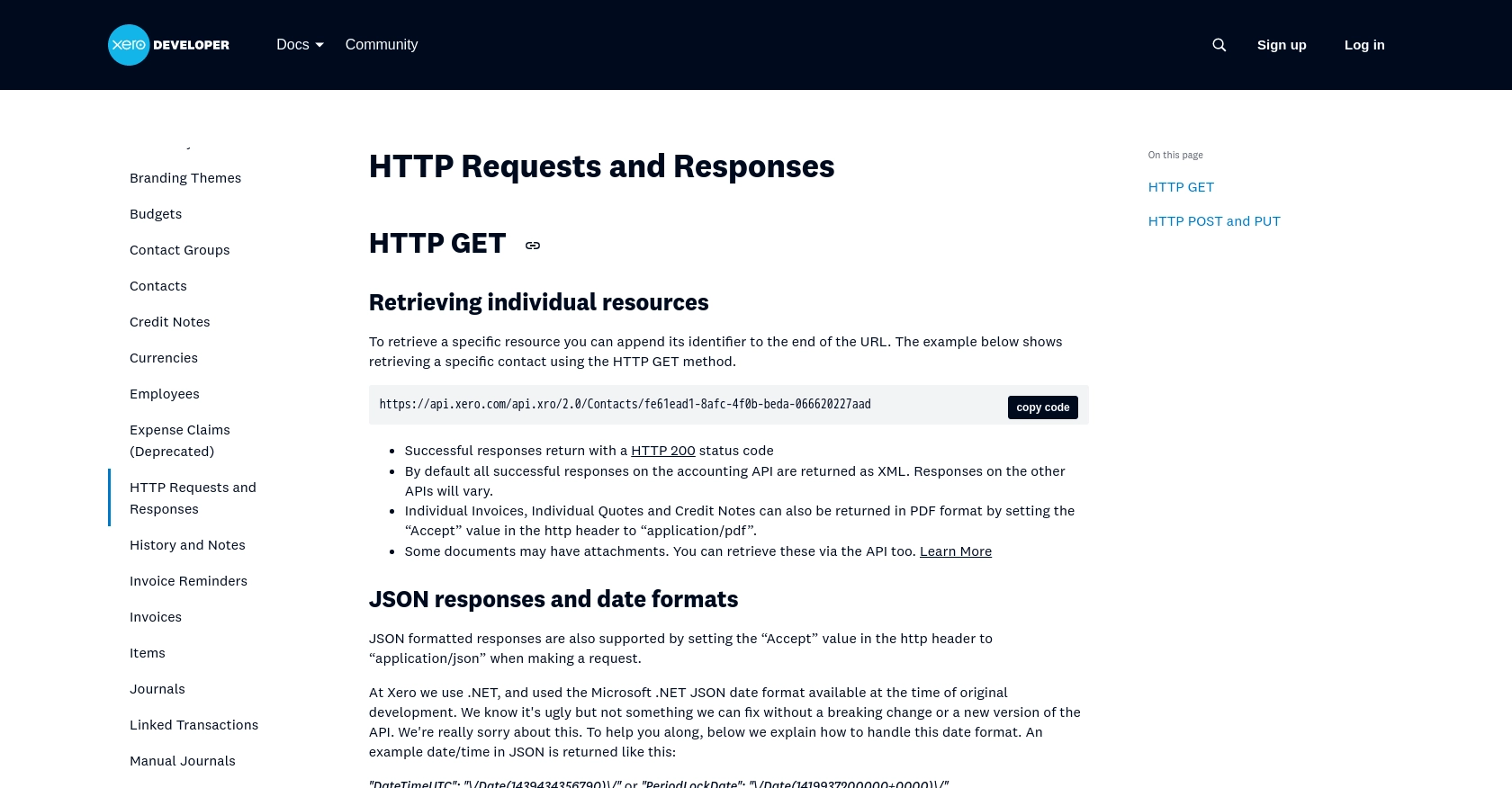
sbb-itb-96038d7
Making API Calls to Retrieve Vendors from Xero Using JavaScript
With your Xero developer account and app set up, you can now proceed to make API calls to retrieve vendor information. This section will guide you through the process of using JavaScript to interact with the Xero API, focusing on retrieving vendors by filtering contacts.
Prerequisites for Xero API Integration with JavaScript
Before diving into the code, ensure you have the following prerequisites in place:
- Node.js installed on your machine.
- A package manager like npm or yarn.
- Familiarity with JavaScript and asynchronous programming.
Installing Required Dependencies
To interact with the Xero API, you'll need to install the Axios library for making HTTP requests. Run the following command in your terminal:
npm install axios
Writing JavaScript Code to Retrieve Vendors from Xero
Create a new JavaScript file named getVendors.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.xero.com/api.xro/2.0/Contacts';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
// Function to get vendors
async function getVendors() {
try {
const response = await axios.get(endpoint, {
headers: headers,
params: {
'IsSupplier': true
}
});
const vendors = response.data.Contacts;
vendors.forEach(vendor => {
console.log(`Vendor Name: ${vendor.Name}`);
});
} catch (error) {
console.error('Error fetching vendors:', error.response.data);
}
}
// Call the function
getVendors();
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth 2.0 authorization process.
Running the JavaScript Code
Execute the script using Node.js by running the following command in your terminal:
node getVendors.js
If successful, the script will output the names of the vendors retrieved from your Xero sandbox environment.
Handling Errors and Verifying API Requests
When making API calls, it's crucial to handle potential errors gracefully. The example code includes a try-catch
block to catch and log any errors that occur during the request. Common error codes include:
- 401 Unauthorized: Check your access token and ensure it hasn't expired.
- 403 Forbidden: Verify that your app has the correct scopes and permissions.
- 429 Too Many Requests: Xero's API rate limit is 60 requests per minute. Implement retry logic if you encounter this error.
For more information on error codes, refer to the Xero API documentation.
By following these steps, you can efficiently retrieve vendor information from Xero using JavaScript, enabling seamless integration with your business systems.
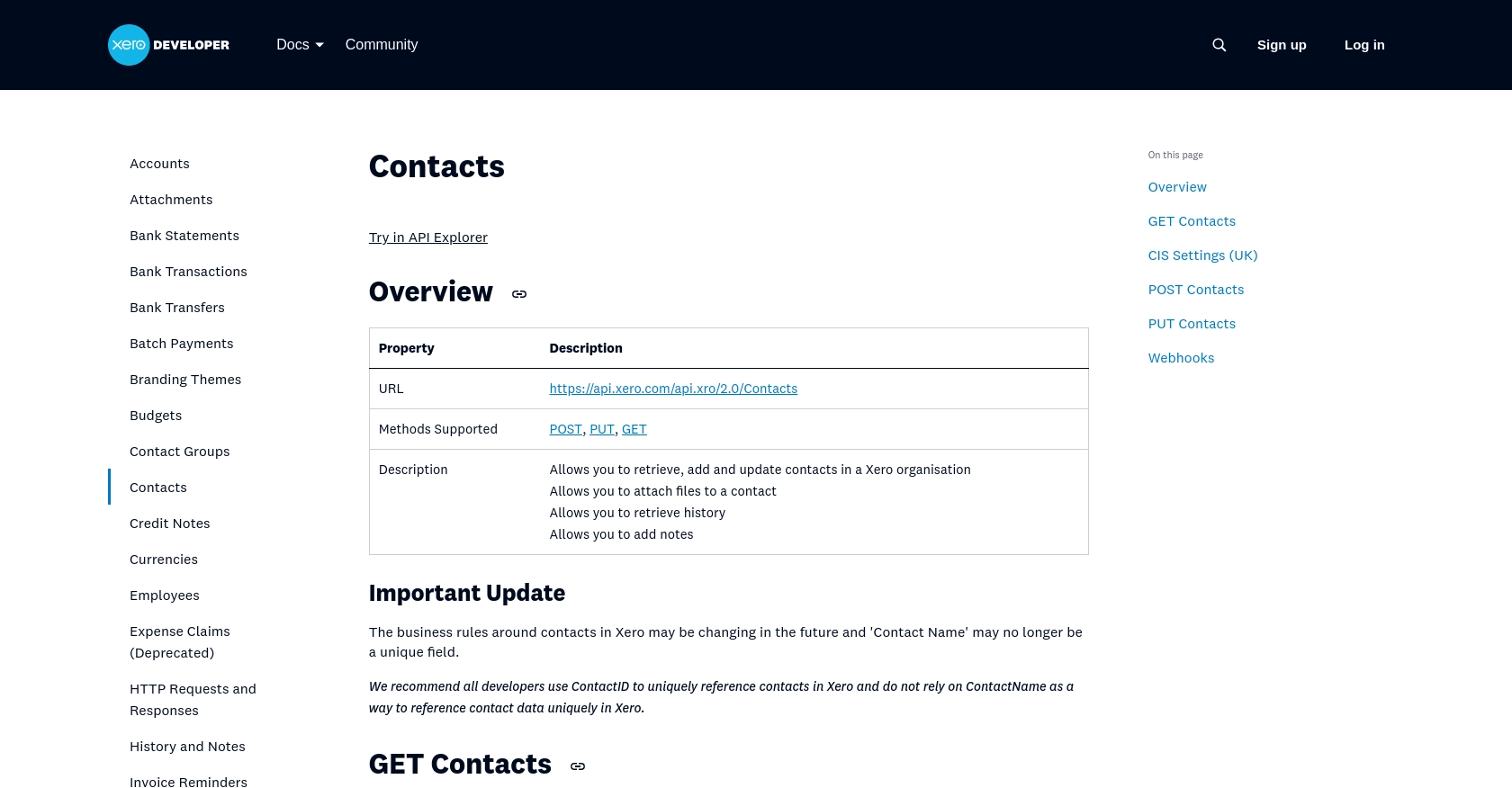
Conclusion and Best Practices for Integrating Xero API with JavaScript
Integrating with the Xero API using JavaScript provides a powerful way to automate and streamline your accounting processes. By retrieving vendor information, you can ensure that your business systems are synchronized and up-to-date, enhancing efficiency and accuracy.
Best Practices for Secure and Efficient Xero API Integration
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault service to manage sensitive information.
- Handle Rate Limiting: Xero's API rate limit is 60 requests per minute. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from Xero is transformed and standardized to match your business systems' requirements, facilitating seamless integration.
Streamline Your Integrations with Endgrate
While integrating with Xero's API can be highly beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product.
With Endgrate, you can build once for each use case instead of multiple times for different integrations, saving time and resources. Take advantage of an easy, intuitive integration experience for your customers and streamline your development efforts.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/contacts
Ready to get started?