Using the Mixpanel API to Get Annotations (with Python examples)
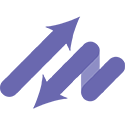
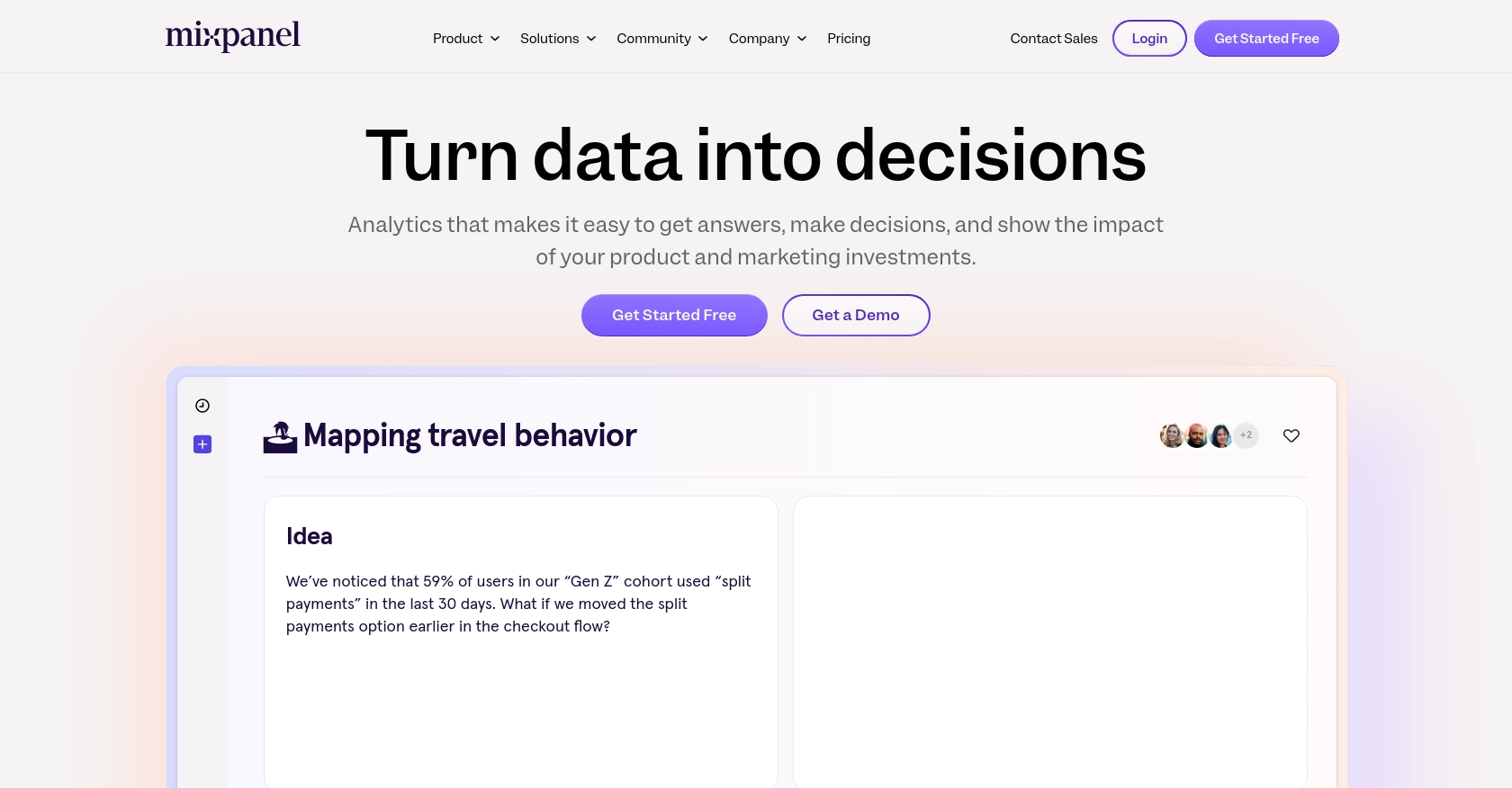
Introduction to Mixpanel API for Annotations
Mixpanel is a powerful analytics platform that helps businesses track user interactions and behaviors across their applications. With its robust set of tools, Mixpanel enables companies to gain insights into user engagement, retention, and conversion rates, allowing for data-driven decision-making.
Developers often integrate with Mixpanel's API to access and manipulate data for enhanced analytics capabilities. One common use case is retrieving annotations, which are notes or comments added to data points to provide context or explanations. By accessing annotations through the Mixpanel API, developers can automate the process of fetching these insights, which can be crucial for understanding trends and anomalies in user behavior.
Setting Up Your Mixpanel Test Account for API Integration
Before you can start interacting with the Mixpanel API to retrieve annotations, you need to set up a Mixpanel account. This setup will allow you to access the necessary credentials and permissions required for API calls.
Create a Mixpanel Account
If you don't already have a Mixpanel account, you can sign up for a free account on the Mixpanel website. Follow the instructions to create your account and log in to the Mixpanel dashboard.
Generate a Mixpanel Service Account
To authenticate API requests, you'll need to create a service account. A service account in Mixpanel is designed for non-human entities like scripts or backend services. Follow these steps to create one:
- Navigate to your organization settings in the Mixpanel dashboard.
- Go to the Service Accounts tab.
- Click on Create Service Account.
- Select the appropriate role and projects for the service account.
- Save the service account's username and secret in a secure location, as you won't be able to access the secret again after creation.
For more details, refer to the Mixpanel Service Accounts documentation.
Authenticate Using HTTP Basic Auth
Mixpanel's API uses HTTP Basic Auth for authentication. You will need the service account's username and secret to authenticate your requests. Here's an example of how to set up authentication in Python:
import requests
response = requests.get(
'https://mixpanel.com/api/app/me',
auth=('', '')
)
Replace <serviceaccount_username>
and <serviceaccount_secret>
with your actual service account credentials.
Verify Your Setup
Once your service account is set up and authenticated, you can test your configuration by making a simple API call to ensure that your credentials are working correctly. This will confirm that you have the necessary access to proceed with retrieving annotations.
For more information on authentication, visit the Mixpanel Authentication documentation.
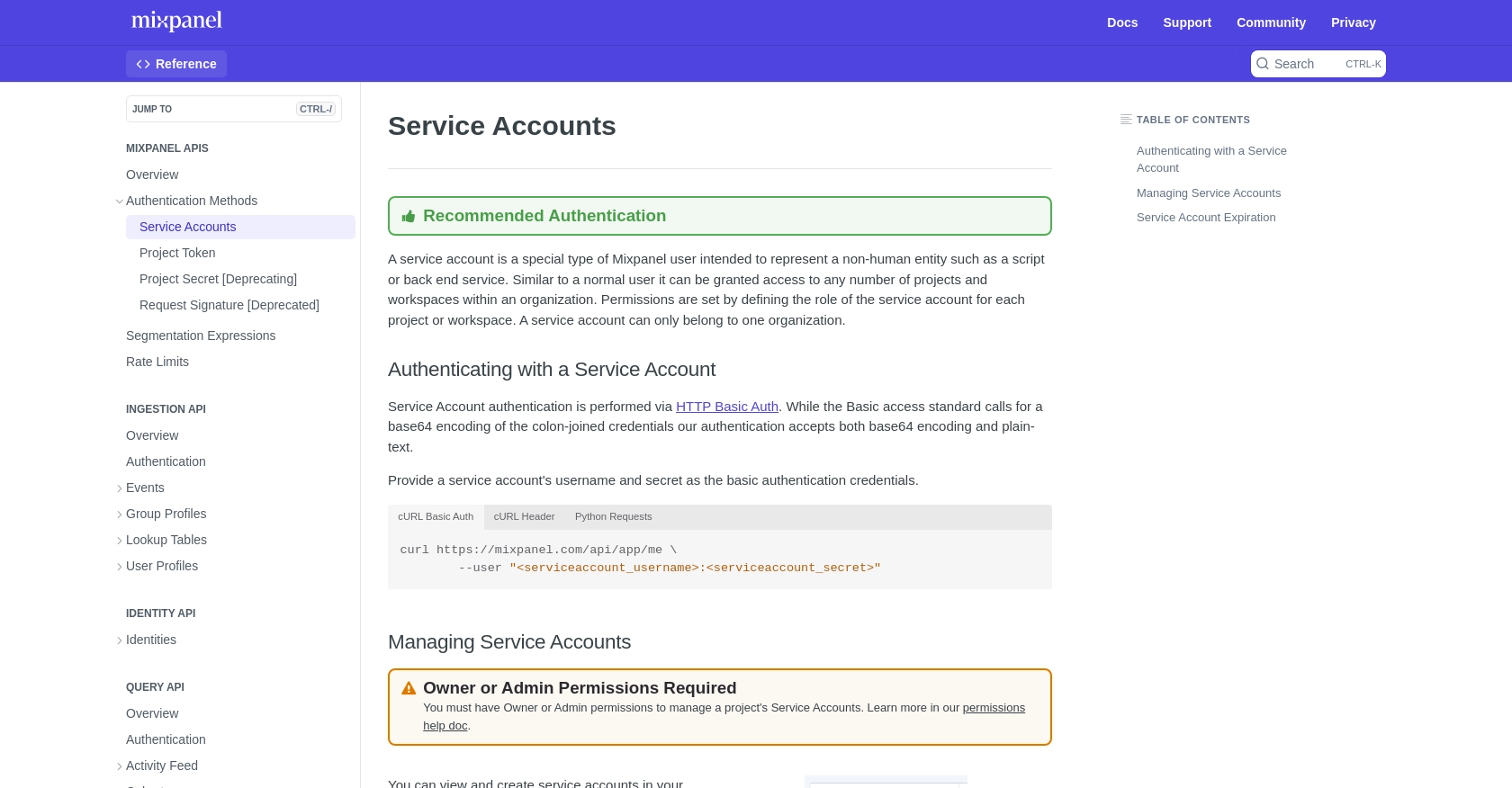
sbb-itb-96038d7
Making API Calls to Retrieve Annotations from Mixpanel Using Python
Now that you have set up your Mixpanel account and authenticated your service account, it's time to make API calls to retrieve annotations. Annotations in Mixpanel provide valuable context to your data, helping you understand trends and anomalies. This section will guide you through the process of making these API calls using Python.
Prerequisites for Python API Integration with Mixpanel
Before proceeding, ensure you have the following installed on your machine:
- Python 3.x
- The Python package installer
pip
Additionally, you will need the requests
library to make HTTP requests. Install it using the following command:
pip install requests
Example Code to Retrieve Annotations from Mixpanel API
To retrieve annotations, you'll need to make a GET request to the Mixpanel API endpoint. Below is a sample Python script to achieve this:
import requests
# Define the API endpoint for retrieving annotations
endpoint = "https://mixpanel.com/api/app/projects/{projectId}/annotations"
# Replace with your actual project ID
project_id = "your_project_id"
# Set up authentication using service account credentials
auth = ('', '')
# Make the GET request to the Mixpanel API
response = requests.get(endpoint.format(projectId=project_id), auth=auth)
# Check if the request was successful
if response.status_code == 200:
annotations = response.json()
print("Annotations retrieved successfully:")
for annotation in annotations:
print(annotation)
else:
print(f"Failed to retrieve annotations. Status code: {response.status_code}")
Replace your_project_id
, <serviceaccount_username>
, and <serviceaccount_secret>
with your actual project ID and service account credentials.
Verifying Successful API Requests and Handling Errors
After running the script, you should see the annotations printed in your console if the request is successful. If you encounter any errors, check the status code returned by the API:
- 200: Success - Annotations retrieved successfully.
- 401: Unauthorized - Check your authentication credentials.
- 429: Too Many Requests - You have hit the rate limit. Consider spreading out your requests.
For more details on rate limits, refer to the Mixpanel Rate Limits documentation.
Testing and Validating API Calls in Mixpanel Sandbox
To ensure your API calls are working as expected, you can verify the annotations in your Mixpanel dashboard. This will confirm that the data retrieved matches the annotations stored in your project.
For further information on the API call, visit the Mixpanel Annotations API documentation.
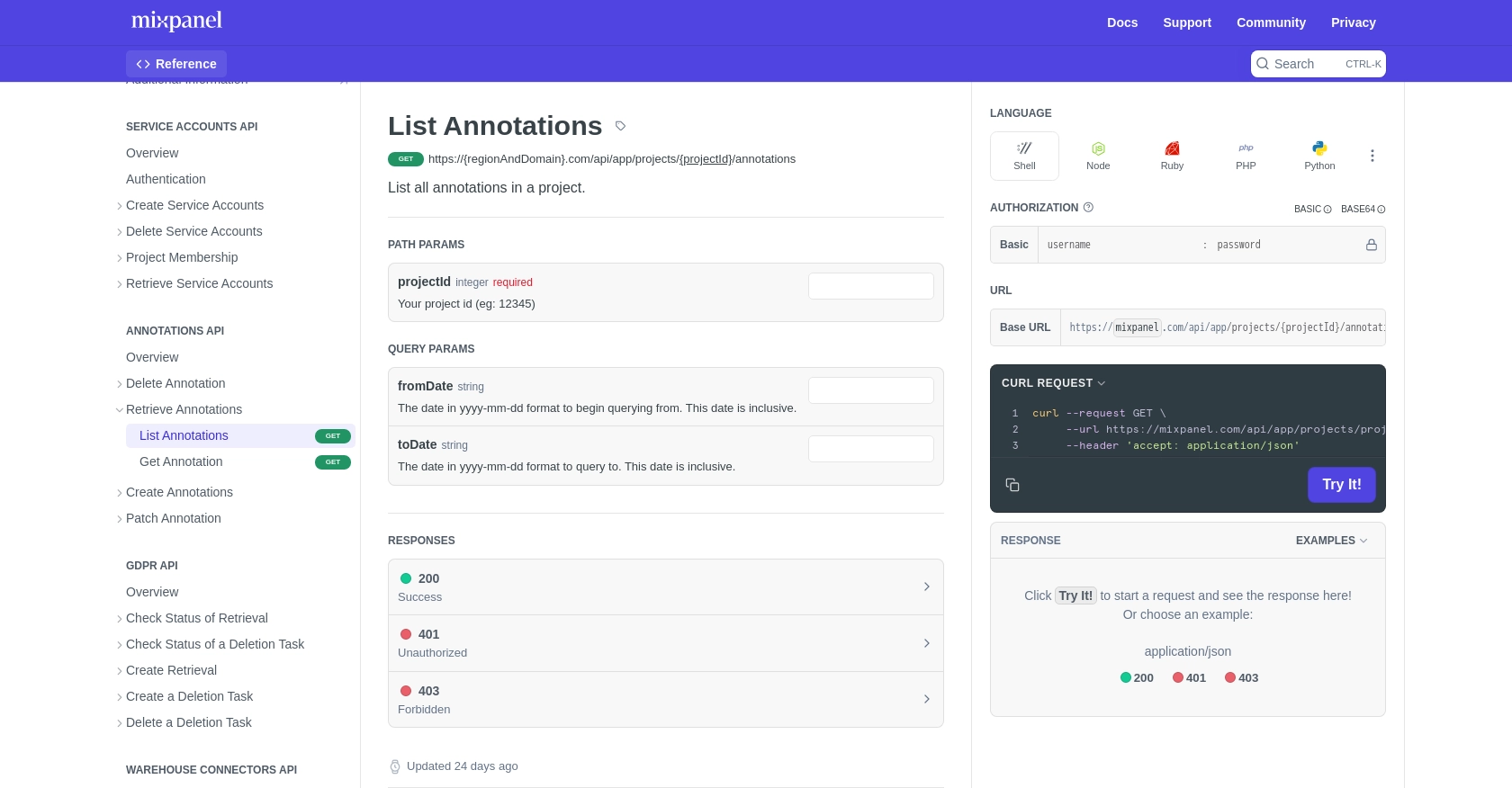
Conclusion and Best Practices for Using Mixpanel API with Python
Integrating with the Mixpanel API to retrieve annotations can significantly enhance your data analysis capabilities by providing context to user interactions. By following the steps outlined in this guide, you can efficiently set up your Mixpanel account, authenticate using service accounts, and make API calls using Python.
Best Practices for Secure and Efficient Mixpanel API Integration
- Secure Storage of Credentials: Always store your service account credentials securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handling Rate Limits: Be mindful of Mixpanel's rate limits. If you encounter a 429 status code, spread out your API requests or consolidate them to avoid hitting the limit. For more details, refer to the Mixpanel Rate Limits documentation.
- Data Standardization: Ensure that the data retrieved from Mixpanel is standardized and transformed as needed to fit your application's requirements.
Streamlining Integrations with Endgrate
Building and maintaining integrations can be time-consuming and complex. With Endgrate, you can simplify this process by leveraging a unified API endpoint that connects to multiple platforms, including Mixpanel. This allows you to focus on your core product while ensuring a seamless integration experience for your customers.
Explore how Endgrate can save you time and resources by visiting Endgrate and discover the benefits of outsourcing your integration needs.
Read More
Ready to get started?