Using the FreeAgent API to Get Contacts in Javascript
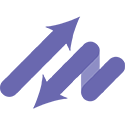
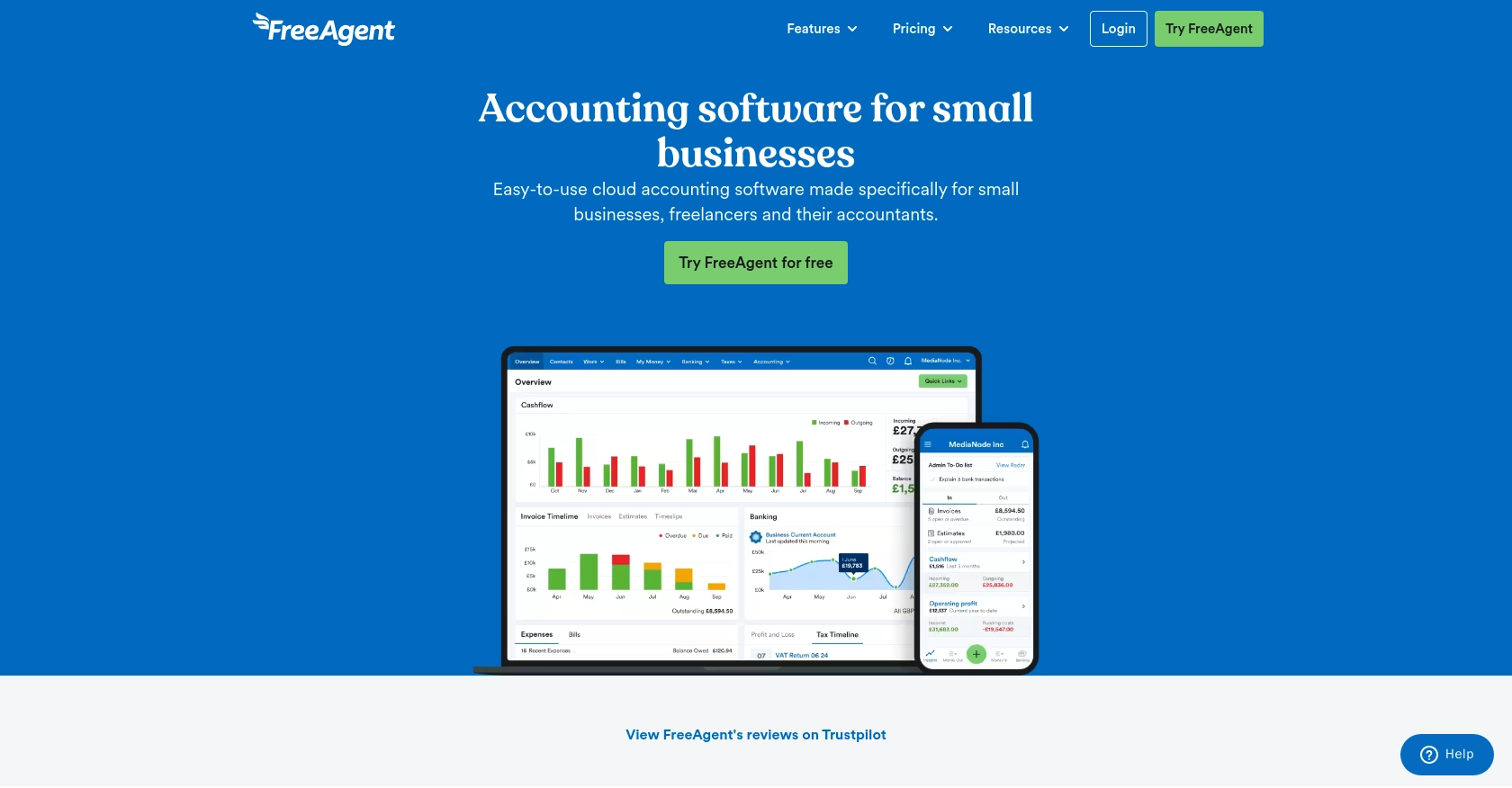
Introduction to FreeAgent API Integration
FreeAgent is a comprehensive accounting software platform tailored for small businesses and freelancers. It offers a range of features including invoicing, expense management, and project tracking, making it an essential tool for managing financial operations efficiently.
Developers may want to integrate with the FreeAgent API to automate and streamline financial data management. For example, accessing contact information through the FreeAgent API can enable developers to synchronize client data across multiple platforms, ensuring consistency and accuracy in financial records.
This article will guide you through using JavaScript to interact with the FreeAgent API, specifically focusing on retrieving contact information. By leveraging this integration, developers can enhance their applications with robust financial data capabilities.
Setting Up Your FreeAgent Sandbox Account for API Integration
Before you can start interacting with the FreeAgent API using JavaScript, you'll need to set up a sandbox account. This allows you to safely test API calls without affecting real data. Follow these steps to create your FreeAgent sandbox account and configure OAuth authentication.
Create a FreeAgent Sandbox Account
- Visit the FreeAgent Sandbox page.
- Sign up for a free temporary account by following the on-screen instructions.
- Complete the setup stages for your company within the sandbox environment to avoid unexpected errors during API usage.
Register Your Application on the FreeAgent Developer Dashboard
- Navigate to the FreeAgent Developer Dashboard.
- Create a new app and take note of the OAuth Client ID and Client Secret provided.
Configure OAuth Authentication Using Google OAuth 2.0 Playground
- Go to the Google OAuth 2.0 Playground.
- Enter the following endpoints:
- Authorization Endpoint:
https://api.sandbox.freeagent.com/v2/approve_app
- Token Endpoint:
https://api.sandbox.freeagent.com/v2/token_endpoint
- Authorization Endpoint:
- Input your OAuth Client ID and Client Secret from the FreeAgent Developer Dashboard.
- Specify a scope name and click on the "Authorize APIs" button.
- Log in to your FreeAgent Sandbox account and approve the app.
- Click "Exchange Authorization Code for Tokens" to generate your Access and Refresh Tokens.
With your sandbox account and OAuth setup complete, you're now ready to start making API calls to FreeAgent using JavaScript.
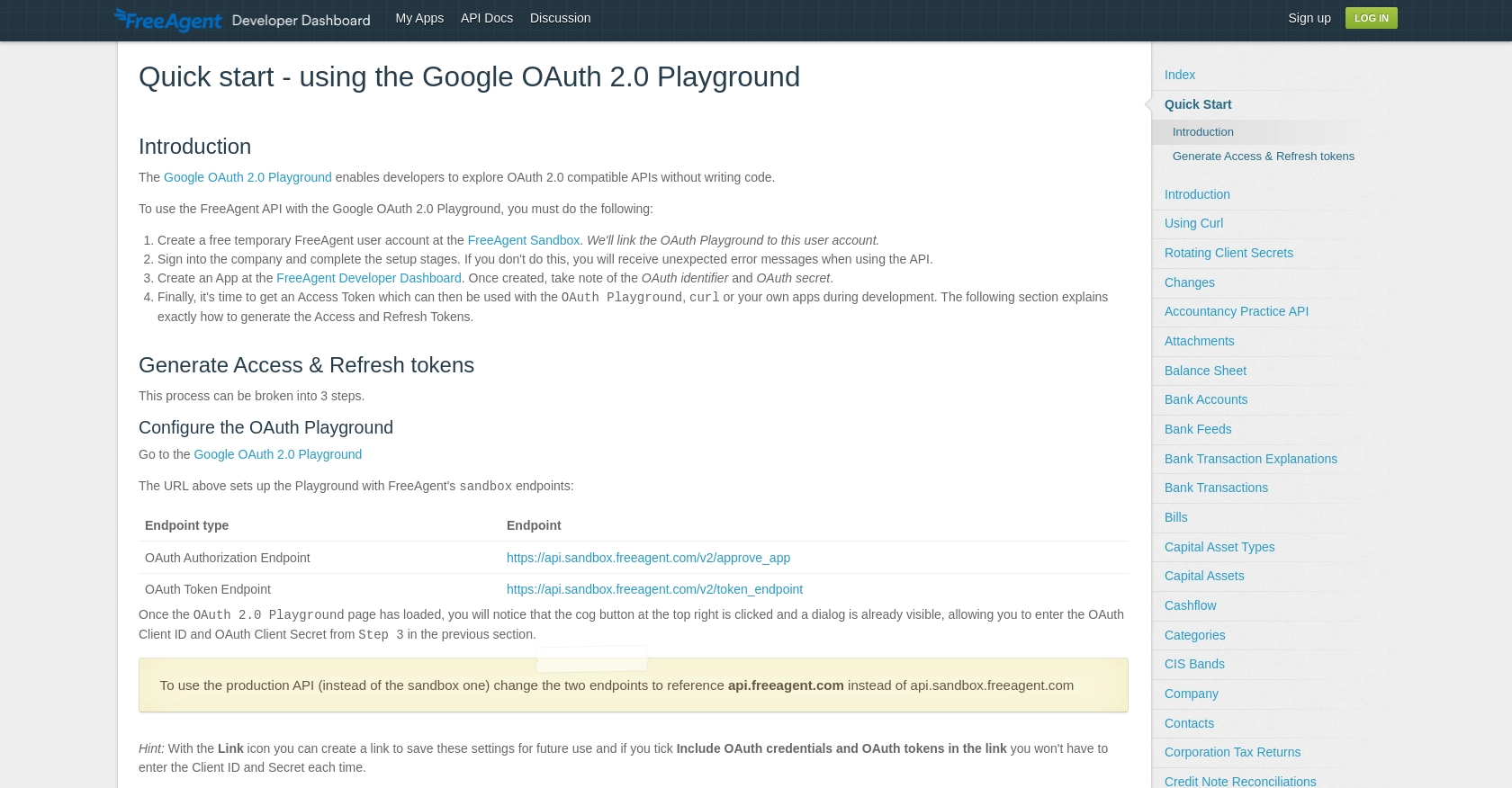
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from FreeAgent Using JavaScript
With your FreeAgent sandbox account and OAuth setup complete, you can now proceed to make API calls to retrieve contact information using JavaScript. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for FreeAgent API Integration
Before making API calls, ensure that your development environment is ready. You'll need Node.js installed on your machine to run JavaScript code outside of a browser. Additionally, you'll use the axios
library to handle HTTP requests.
- Install Node.js from the official website.
- Initialize a new Node.js project by running
npm init -y
in your terminal. - Install the
axios
library by runningnpm install axios
.
Writing JavaScript Code to Fetch Contacts from FreeAgent API
With your environment set up, you can now write the JavaScript code to fetch contacts from the FreeAgent API. The following example demonstrates how to make a GET request to the FreeAgent contacts endpoint.
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.sandbox.freeagent.com/v2/contacts';
const headers = {
'Authorization': 'Bearer Your_Access_Token',
'Accept': 'application/json'
};
// Function to get contacts
async function getContacts() {
try {
const response = await axios.get(endpoint, { headers });
const contacts = response.data.contacts;
console.log('Contacts:', contacts);
} catch (error) {
console.error('Error fetching contacts:', error.response ? error.response.data : error.message);
}
}
// Call the function
getContacts();
Replace Your_Access_Token
with the access token you obtained during the OAuth setup. This script uses axios
to send a GET request to the FreeAgent API, retrieves the contact data, and logs it to the console.
Verifying API Call Success and Handling Errors
After running the script, you should see a list of contacts printed in the console. If the request is successful, the response will include contact details such as first name, last name, and organization name.
In case of errors, the script will catch and log them. Common issues may include invalid tokens or exceeding rate limits. The FreeAgent API enforces a rate limit of 120 requests per minute and 3600 requests per hour. If you exceed these limits, you'll receive a 429 status code. Implement a back-off strategy to handle such errors gracefully.
For more details on error handling and rate limits, refer to the FreeAgent API documentation.
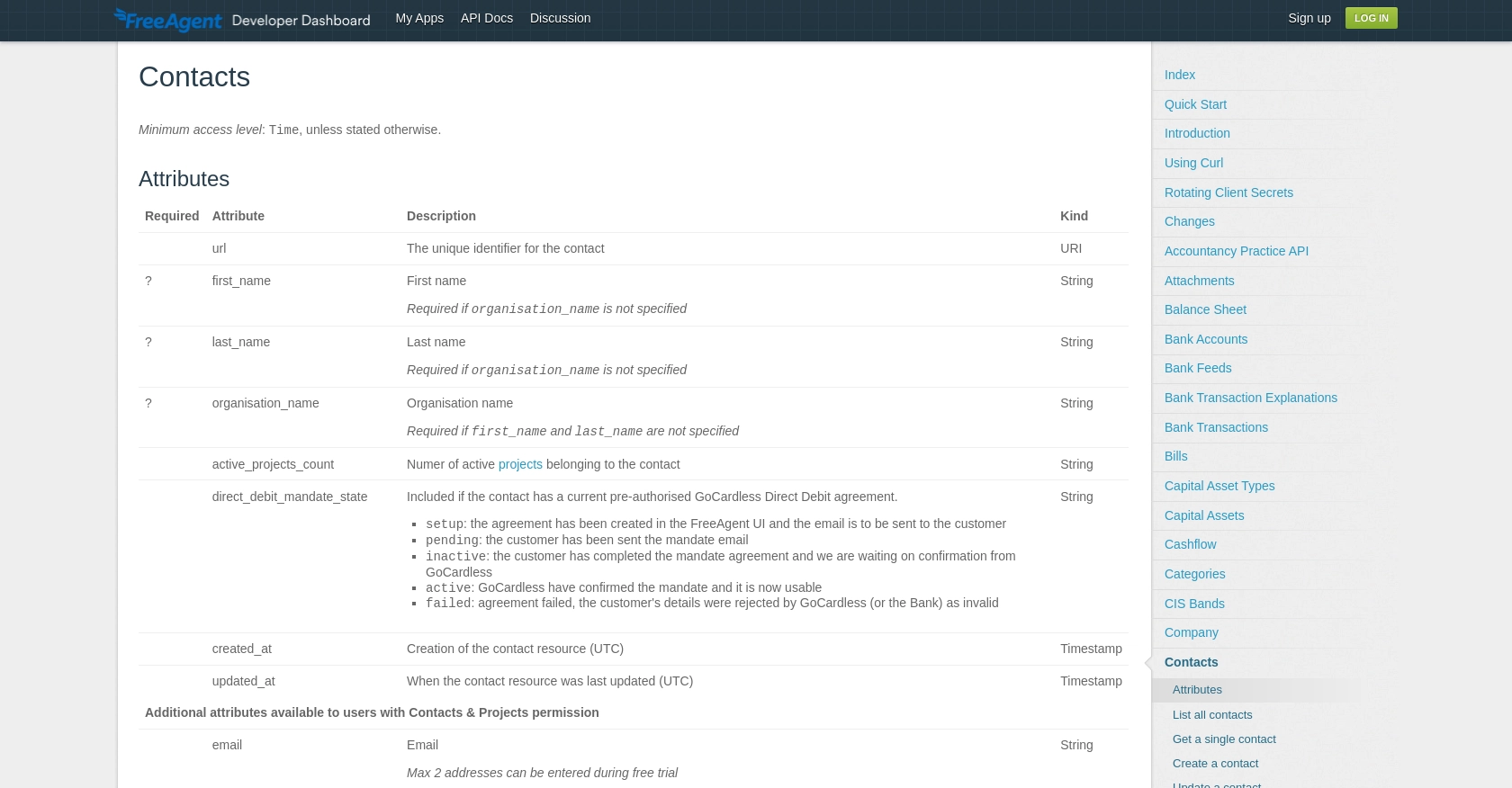
Conclusion: Best Practices for FreeAgent API Integration Using JavaScript
Integrating with the FreeAgent API using JavaScript can significantly enhance your application's ability to manage financial data efficiently. By following the steps outlined in this guide, you can seamlessly retrieve contact information and ensure data consistency across platforms.
Best Practices for Secure and Efficient FreeAgent API Usage
- Secure Storage of Credentials: Always store your OAuth credentials securely. Consider using environment variables or secure vaults to protect sensitive information like access tokens.
- Handle Rate Limiting: Be mindful of FreeAgent's rate limits—120 requests per minute and 3600 per hour. Implement a back-off strategy to handle 429 status codes gracefully, ensuring your application remains responsive.
- Data Standardization: Standardize and transform data fields as needed to maintain consistency across different systems and applications.
Enhancing Integration Capabilities with Endgrate
While integrating with FreeAgent's API provides robust financial data capabilities, managing multiple integrations can be complex. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including FreeAgent. This allows you to build once for each use case and streamline integration management.
By leveraging Endgrate, you can focus on your core product development while ensuring a seamless integration experience for your customers. Explore how Endgrate can save you time and resources by visiting Endgrate's website.
Read More
Ready to get started?