How to Create or Update Contacts with the OnePageCRM API in Python
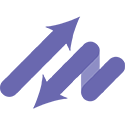
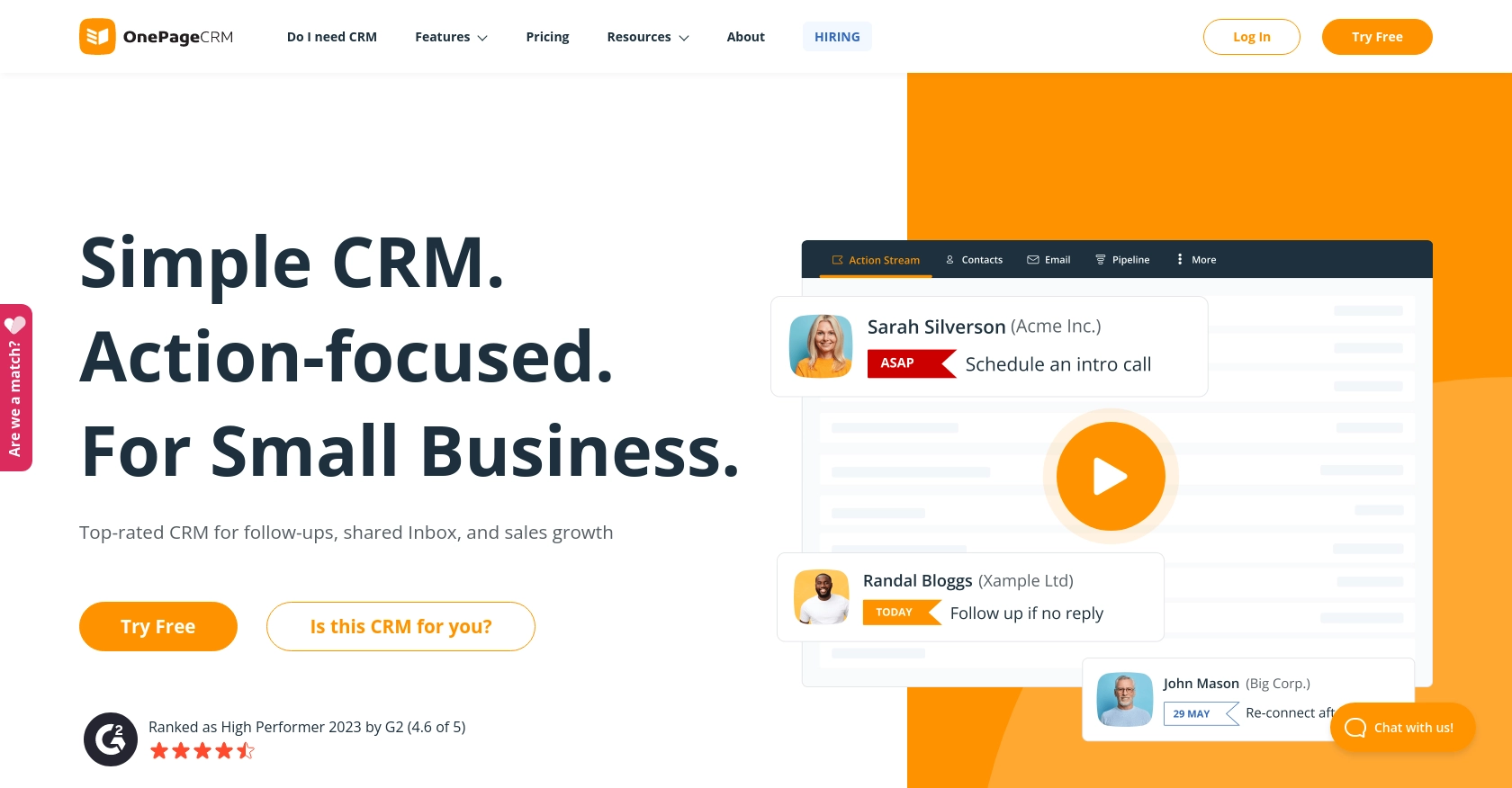
Introduction to OnePageCRM API Integration
OnePageCRM is a dynamic CRM platform designed to simplify sales processes for businesses by focusing on actionable tasks and streamlined workflows. It offers a user-friendly interface that helps sales teams manage contacts, track deals, and enhance productivity.
Integrating with the OnePageCRM API allows developers to automate and enhance customer relationship management tasks. For example, you can create or update contacts directly from your application, ensuring that your CRM data is always current and accurate. This integration can be particularly useful for syncing contact information from various sources, reducing manual data entry, and improving data consistency across platforms.
Setting Up Your OnePageCRM Test Account
Before you can start integrating with the OnePageCRM API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Sign Up for a OnePageCRM Account
- Visit the OnePageCRM website and sign up for a free trial account if you don't already have one.
- Follow the on-screen instructions to complete the registration process.
- Once registered, log in to your OnePageCRM account to access the dashboard.
Generate API Credentials for OnePageCRM
To interact with the OnePageCRM API, you'll need to generate API credentials. These credentials will include a client ID and client secret, which are necessary for authenticating your API requests.
- Navigate to the API settings in your OnePageCRM account.
- Create a new API application by providing the required details, such as the application name and description.
- Once the application is created, note down the client ID and client secret. These will be used in your API calls.
Understanding OnePageCRM Custom Authentication
OnePageCRM uses a custom authentication method for API access. Ensure you have the following details ready:
- Base URL:
https://app.onepagecrm.com/api/v3/
- Authentication Details: Use the client ID and client secret generated earlier to authenticate your requests.
For more detailed information, refer to the OnePageCRM API documentation.
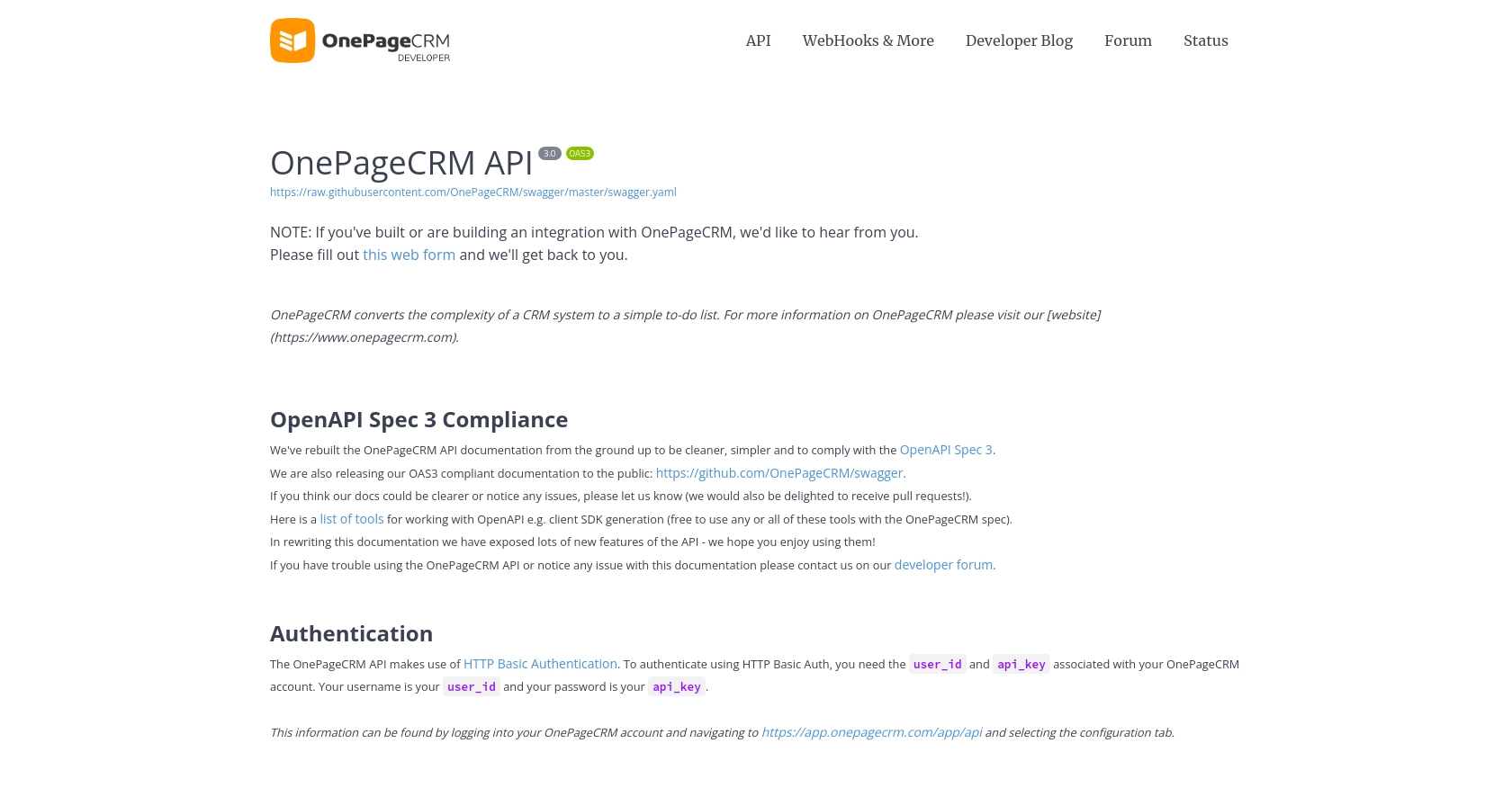
sbb-itb-96038d7
Making API Calls to Create or Update Contacts with OnePageCRM in Python
To interact with the OnePageCRM API and manage contacts, you'll need to make HTTP requests using Python. This section will guide you through the process of setting up your environment, writing the code, and handling responses effectively.
Setting Up Your Python Environment for OnePageCRM API Integration
Before you begin coding, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Next, install the requests
library, which will be used to make HTTP requests to the OnePageCRM API:
pip install requests
Creating Contacts Using the OnePageCRM API in Python
To create a new contact in OnePageCRM, you'll use the POST method. Below is a sample Python script to achieve this:
import requests
import json
# Set the API endpoint
url = "https://app.onepagecrm.com/api/v3/contacts"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Token"
}
# Define the contact data
contact_data = {
"title": "Mr",
"first_name": "Joe",
"last_name": "Bloggs",
"job_title": "Engineer",
"company_name": "Morgan's Forensic Lab",
"emails": [{"type": "work", "value": "joe.bloggs@foo.bar"}],
"phones": [{"type": "work", "value": "(912) 644-1770"}],
"address_list": [{
"address": "Unit 5, Business Innovation Centre",
"city": "Upper Newcastle",
"state": "Galway",
"zip_code": "H91 Y0T0",
"country_code": "IE",
"type": "delivery"
}]
}
# Make the POST request to create a contact
response = requests.post(url, headers=headers, data=json.dumps(contact_data))
# Check the response status
if response.status_code == 201:
print("Contact created successfully.")
else:
print(f"Failed to create contact: {response.status_code} - {response.text}")
Replace Your_Token
with your actual API token. This script sets up the necessary headers and contact data, then sends a POST request to the OnePageCRM API. If successful, it will confirm the creation of the contact.
Updating Contacts Using the OnePageCRM API in Python
To update an existing contact, you'll use the PUT method. Here's how you can do it:
# Set the API endpoint for updating a contact
contact_id = "5aba31ea9007ba0f570c92d4" # Replace with the actual contact ID
url = f"https://app.onepagecrm.com/api/v3/contacts/{contact_id}"
# Define the updated contact data
updated_contact_data = {
"job_title": "Senior Engineer",
"emails": [{"type": "work", "value": "joe.bloggs@updated.foo.bar"}]
}
# Make the PUT request to update the contact
response = requests.put(url, headers=headers, data=json.dumps(updated_contact_data))
# Check the response status
if response.status_code == 200:
print("Contact updated successfully.")
else:
print(f"Failed to update contact: {response.status_code} - {response.text}")
Ensure you replace contact_id
with the ID of the contact you wish to update. This script modifies the job title and email of the specified contact.
Handling API Responses and Errors
When making API calls, it's crucial to handle potential errors. The OnePageCRM API may return various error codes, such as:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed.
- 403 Forbidden: You do not have permission to access the resource.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on the server.
Always check the response status code and handle errors appropriately to ensure robust integration. For more details, refer to the OnePageCRM API documentation.
Best Practices for OnePageCRM API Integration
When integrating with the OnePageCRM API, it's essential to follow best practices to ensure a smooth and efficient process. Here are some key considerations:
- Securely Store Credentials: Always store your API credentials securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid being throttled. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Data Transformation and Standardization: Ensure that data fields are transformed and standardized to match OnePageCRM's requirements. This will help maintain data consistency across platforms.
- Robust Error Handling: Implement comprehensive error handling to manage different response codes effectively. This will help you identify and resolve issues quickly.
Streamline Your Integrations with Endgrate
Building and maintaining integrations can be time-consuming and complex. With Endgrate, you can simplify this process by leveraging a unified API endpoint that connects to multiple platforms, including OnePageCRM.
Endgrate allows you to focus on your core product by outsourcing integrations, saving time and resources. With its intuitive integration experience, you can build once for each use case and easily scale your integrations across different platforms.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?