Using the Quickbooks API to Create Or Update Customers in Python
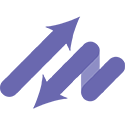
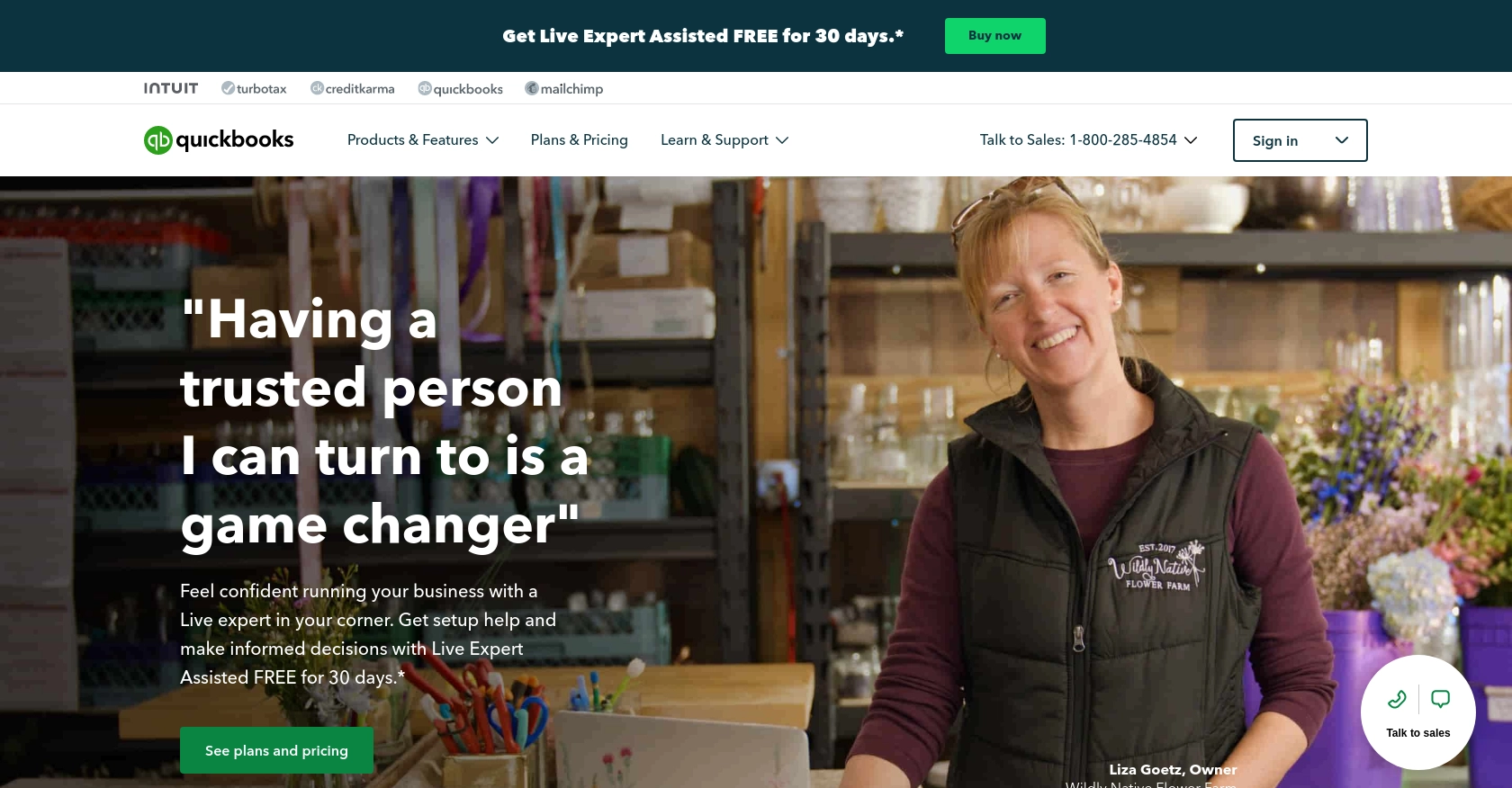
Introduction to QuickBooks API Integration
QuickBooks is a widely-used accounting software that helps businesses manage their finances with ease. It offers a range of features, including invoicing, expense tracking, payroll, and financial reporting, making it a popular choice for small to medium-sized enterprises.
Integrating with the QuickBooks API allows developers to automate and streamline financial processes, such as creating or updating customer records. For example, a developer could use the QuickBooks API to automatically update customer information from an e-commerce platform, ensuring that the accounting records are always up-to-date.
Setting Up Your QuickBooks Sandbox Account for API Integration
Before you can start integrating with the QuickBooks API, you'll need to set up a sandbox account. This allows you to test your application in a safe environment without affecting live data. Follow these steps to create your QuickBooks sandbox account and obtain the necessary credentials for OAuth authentication.
Step 1: Sign Up for a QuickBooks Developer Account
If you don't already have a QuickBooks Developer account, you'll need to create one. Visit the Intuit Developer portal and sign up for a free account. Once registered, log in to access the developer dashboard.
Step 2: Create a New App in QuickBooks
After logging in, navigate to the "My Apps" section and click on "Create an app." Choose "QuickBooks Online and Payments" as the platform. This will allow you to interact with QuickBooks Online APIs.
Step 3: Obtain Client ID and Client Secret
Once your app is created, you'll be directed to the app settings page. Here, you can find your Client ID and Client Secret, which are essential for OAuth authentication. Make sure to copy and securely store these credentials. For more details, refer to the Intuit Developer documentation.
Step 4: Configure App Settings for OAuth
In the app settings, configure the OAuth settings by specifying the redirect URI. This URI is where users will be redirected after they authorize your app. Ensure that this URI matches the one used in your application code. For further guidance, see the app settings documentation.
Step 5: Connect to the QuickBooks Sandbox
With your app configured, you can now connect to the QuickBooks sandbox environment. This allows you to test API calls without affecting real data. Use the sandbox company provided by QuickBooks to simulate real-world scenarios and validate your integration.
By following these steps, you'll be ready to authenticate and interact with the QuickBooks API using OAuth, enabling you to create or update customer records programmatically.
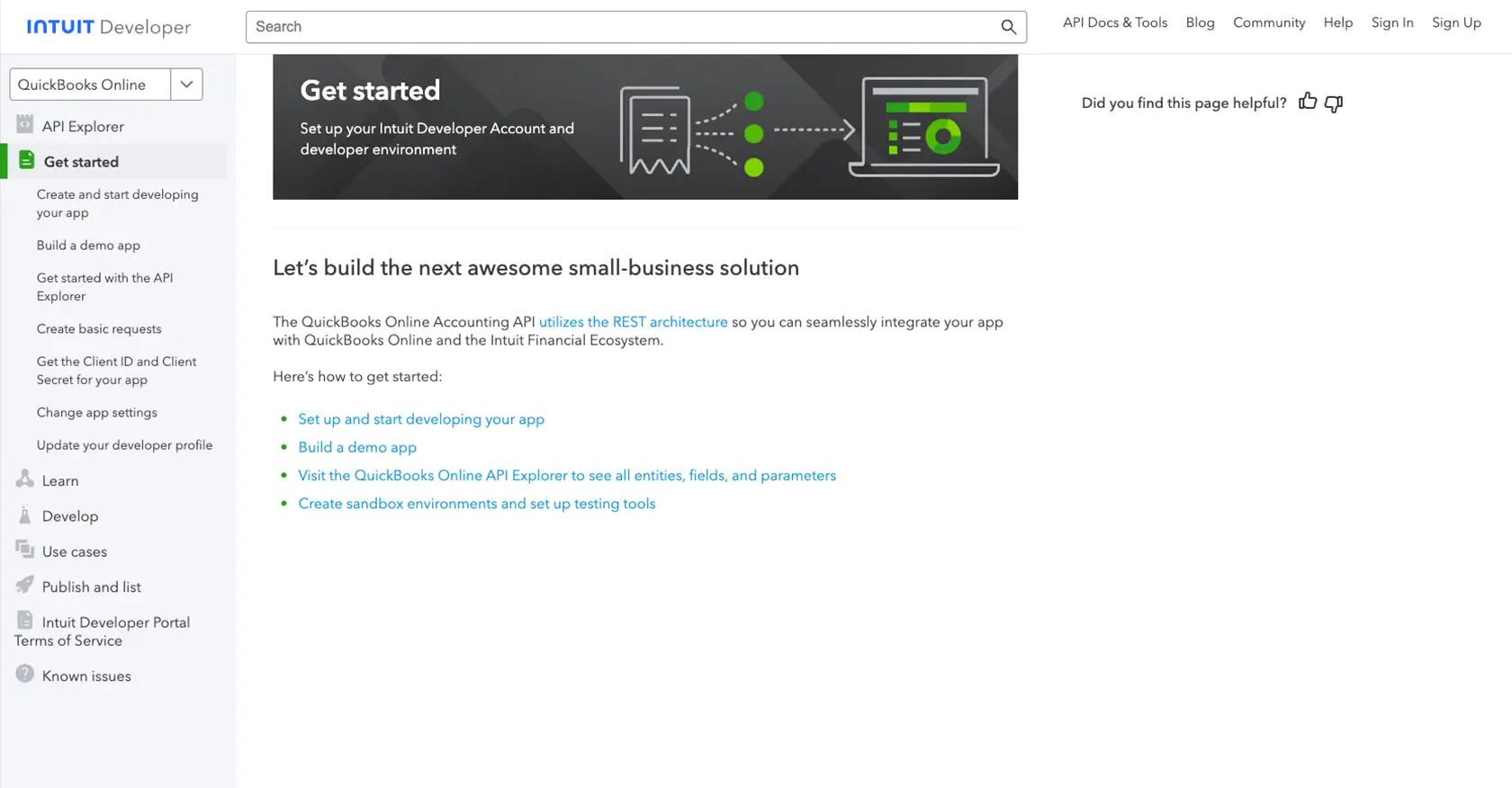
sbb-itb-96038d7
Making API Calls to QuickBooks for Customer Management Using Python
To interact with the QuickBooks API for creating or updating customer records, you'll need to set up your Python environment and write the necessary code to make API calls. This section will guide you through the process, including setting up Python, installing dependencies, and writing the code to interact with the QuickBooks API.
Setting Up Your Python Environment for QuickBooks API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer, pip
Once these are installed, open your terminal or command prompt and install the requests
library, which will be used to make HTTP requests to the QuickBooks API:
pip install requests
Writing Python Code to Create or Update Customers in QuickBooks
Now that your environment is set up, you can write the Python code to create or update customer records in QuickBooks. Follow these steps:
Step 1: Create a Python Script
Create a new Python file named manage_quickbooks_customers.py
and open it in your preferred code editor.
Step 2: Import Required Libraries
Start by importing the necessary libraries:
import requests
import json
Step 3: Define the API Endpoint and Headers
Set up the API endpoint and headers required for authentication. Replace Your_Token
with the OAuth token obtained during the setup process:
# Define the API endpoint for creating or updating customers
url = "https://sandbox-quickbooks.api.intuit.com/v3/company/YOUR_COMPANY_ID/customer"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Token"
}
Step 4: Create or Update Customer Data
Define the customer data you want to create or update. Here's an example of a customer creation request:
# Define the customer data
customer_data = {
"DisplayName": "John Doe",
"PrimaryEmailAddr": {
"Address": "johndoe@example.com"
},
"PrimaryPhone": {
"FreeFormNumber": "(555) 555-5555"
}
}
Step 5: Make the API Call
Use the requests.post
method to send the customer data to QuickBooks:
# Send the request to create or update the customer
response = requests.post(url, headers=headers, json=customer_data)
# Check the response status
if response.status_code == 200:
print("Customer created or updated successfully.")
else:
print("Failed to create or update customer:", response.json())
Verifying the API Call and Handling Errors
After running the script, verify the customer creation or update by checking the QuickBooks sandbox environment. If the request was successful, the customer should appear in the sandbox.
Handle potential errors by checking the response status code and reviewing the error message returned by the API. For more detailed error information, refer to the Intuit Developer documentation.
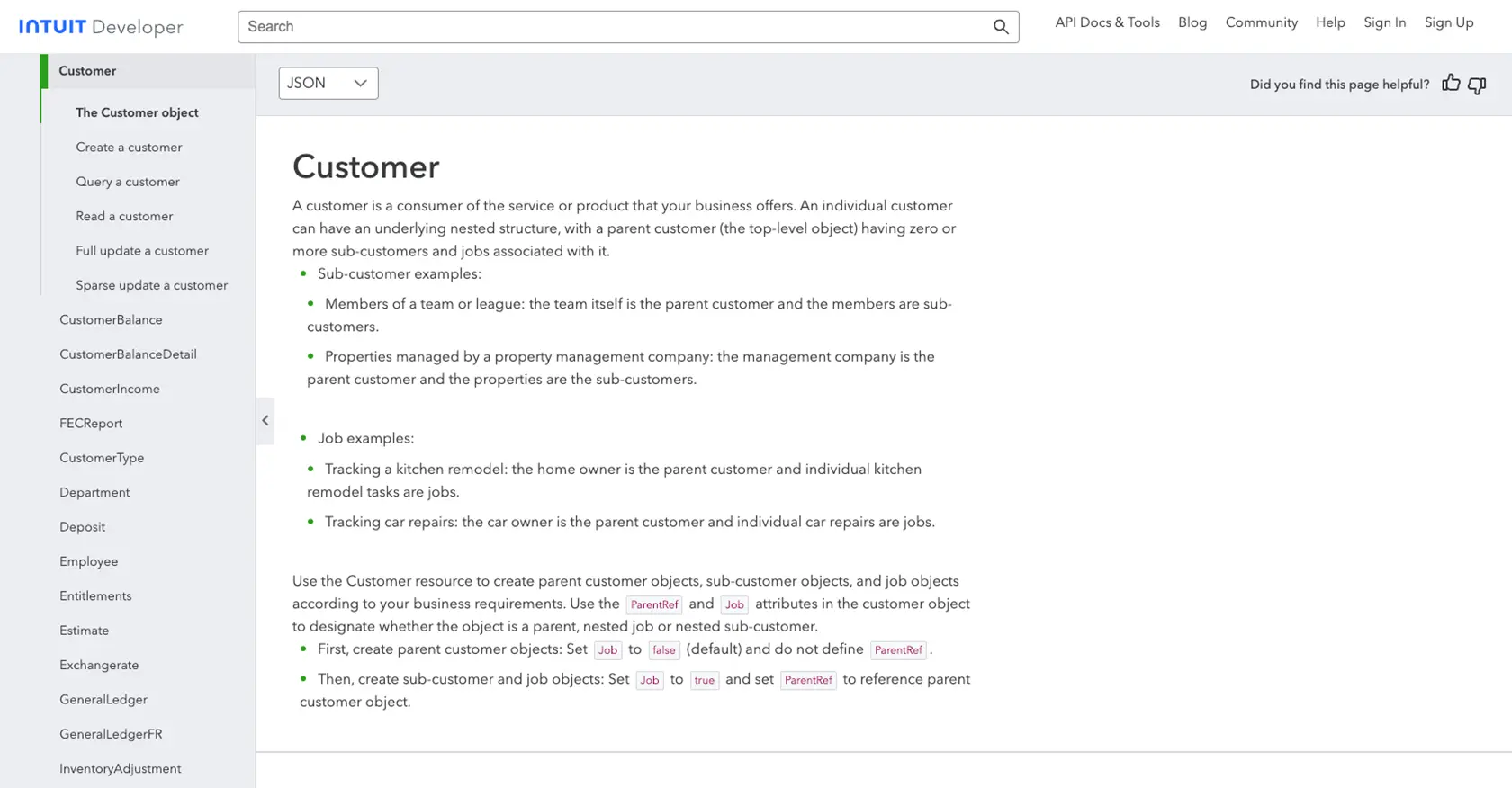
Conclusion and Best Practices for QuickBooks API Integration
Integrating with the QuickBooks API to create or update customer records in Python can significantly enhance your business's financial management processes. By automating these tasks, you ensure that your accounting data remains accurate and up-to-date, reducing manual errors and saving valuable time.
Best Practices for Storing QuickBooks API Credentials
- Always store your OAuth credentials, such as the Client ID and Client Secret, securely. Consider using environment variables or a secure vault to manage sensitive information.
- Regularly rotate your credentials to minimize security risks.
Handling QuickBooks API Rate Limits
QuickBooks API has rate limits that you need to be aware of to avoid service disruptions. Monitor your API usage and implement retry logic with exponential backoff to handle rate limit errors gracefully. For specific rate limit details, refer to the Intuit Developer documentation.
Data Transformation and Standardization
When integrating with QuickBooks, ensure that your data is correctly formatted and standardized. This includes validating email addresses, phone numbers, and other customer details to match QuickBooks' requirements.
Streamlining Integrations with Endgrate
If managing multiple integrations becomes overwhelming, consider using Endgrate to simplify the process. Endgrate provides a unified API endpoint that connects to various platforms, including QuickBooks, allowing you to focus on your core product while outsourcing integration complexities.
With Endgrate, you can build once for each use case instead of multiple times for different integrations, offering an intuitive integration experience for your customers. Visit Endgrate to learn more about how it can benefit your development workflow.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/all-entities/customer
Ready to get started?