Using the Google Analytics API to Get Analytics By Page (with Python examples)
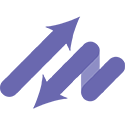
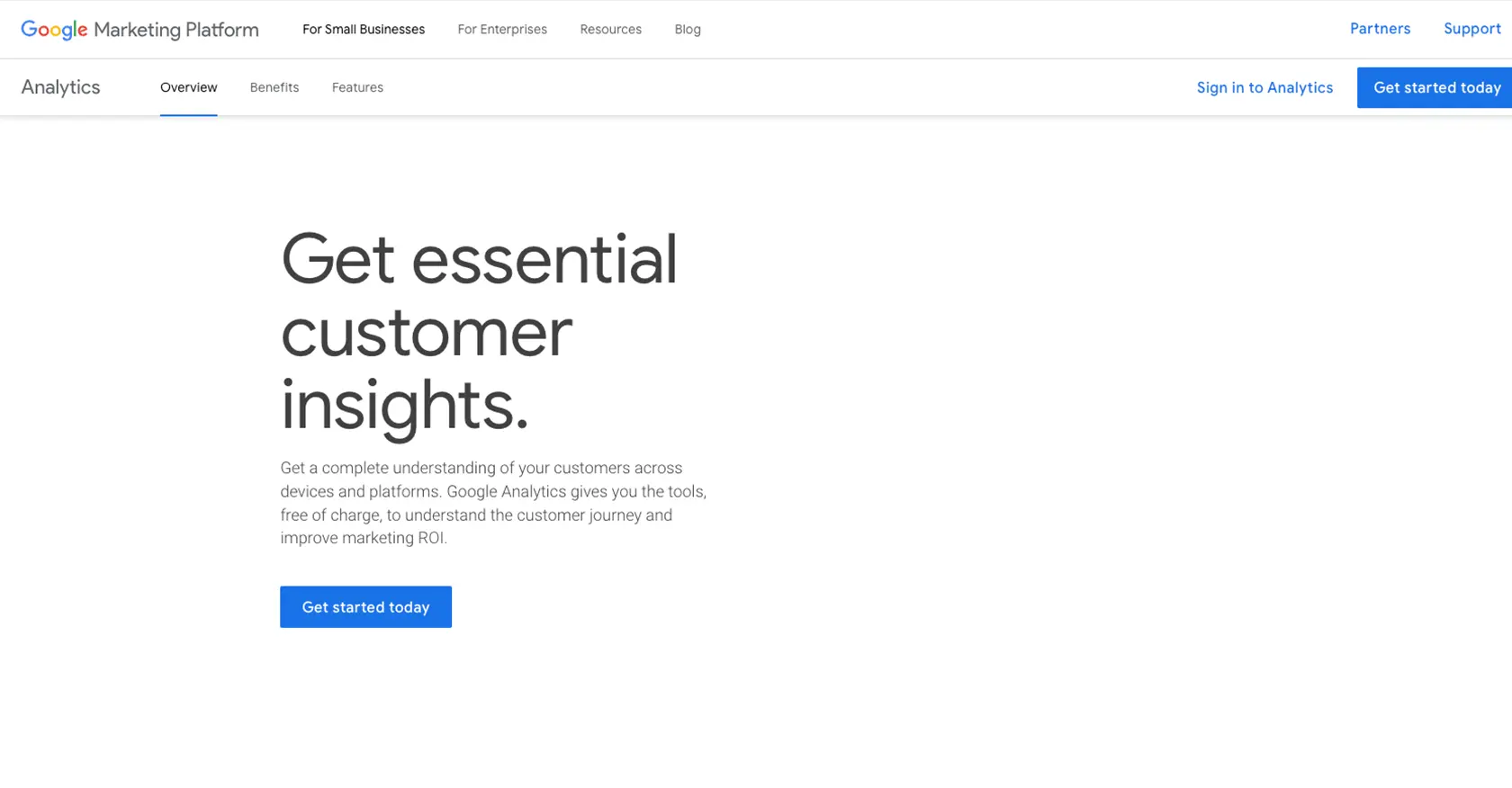
Introduction to Google Analytics API
Google Analytics is a powerful tool that provides insights into website traffic and user behavior. It allows businesses to track and analyze data from their websites and apps, helping them make informed decisions to improve user experience and drive growth.
For developers, integrating with the Google Analytics API offers the ability to programmatically access and manipulate analytics data. This can be particularly useful for automating reporting tasks, building custom dashboards, or integrating analytics data with other business applications.
One common use case is retrieving analytics by page to understand which pages are performing well and which need improvement. By using the Google Analytics API with Python, developers can efficiently gather and analyze this data, enabling data-driven decisions to enhance website performance.
Setting Up Your Google Analytics API Test Account
Before you can start using the Google Analytics API, you need to set up a Google Cloud project and configure OAuth 2.0 authentication. This process involves creating a test account that allows you to access and interact with the API securely.
Create a Google Cloud Project for Google Analytics API
To begin, you'll need a Google Cloud project. Follow these steps to create one:
- Go to the Google Cloud Console.
- Click on the Menu icon and select IAM & Admin > Create a Project.
- Enter a descriptive name for your project and click Create.
Enable Google Analytics API in Your Project
Once your project is created, you need to enable the Google Analytics API:
- In the Google Cloud Console, navigate to APIs & Services > Library.
- Search for "Google Analytics API" and click on it.
- Click Enable to activate the API for your project.
Configure OAuth Consent Screen for Google Analytics API
Next, configure the OAuth consent screen to define how your app will request access to user data:
- In the Google Cloud Console, go to APIs & Services > OAuth consent screen.
- Select the user type and fill out the required fields, such as app name and support email.
- Click Save and Continue.
Create OAuth Credentials for Google Analytics API
To authenticate requests, you'll need to create OAuth credentials:
- Navigate to APIs & Services > Credentials.
- Click Create Credentials and select OAuth client ID.
- Choose the application type that suits your needs (e.g., Web application) and fill in the necessary details.
- Click Create to generate your client ID and client secret.
Make sure to securely store your client ID and client secret, as you'll need them to authenticate your API requests.
For more detailed instructions, refer to the official documentation on creating a Google Cloud project and configuring OAuth consent.
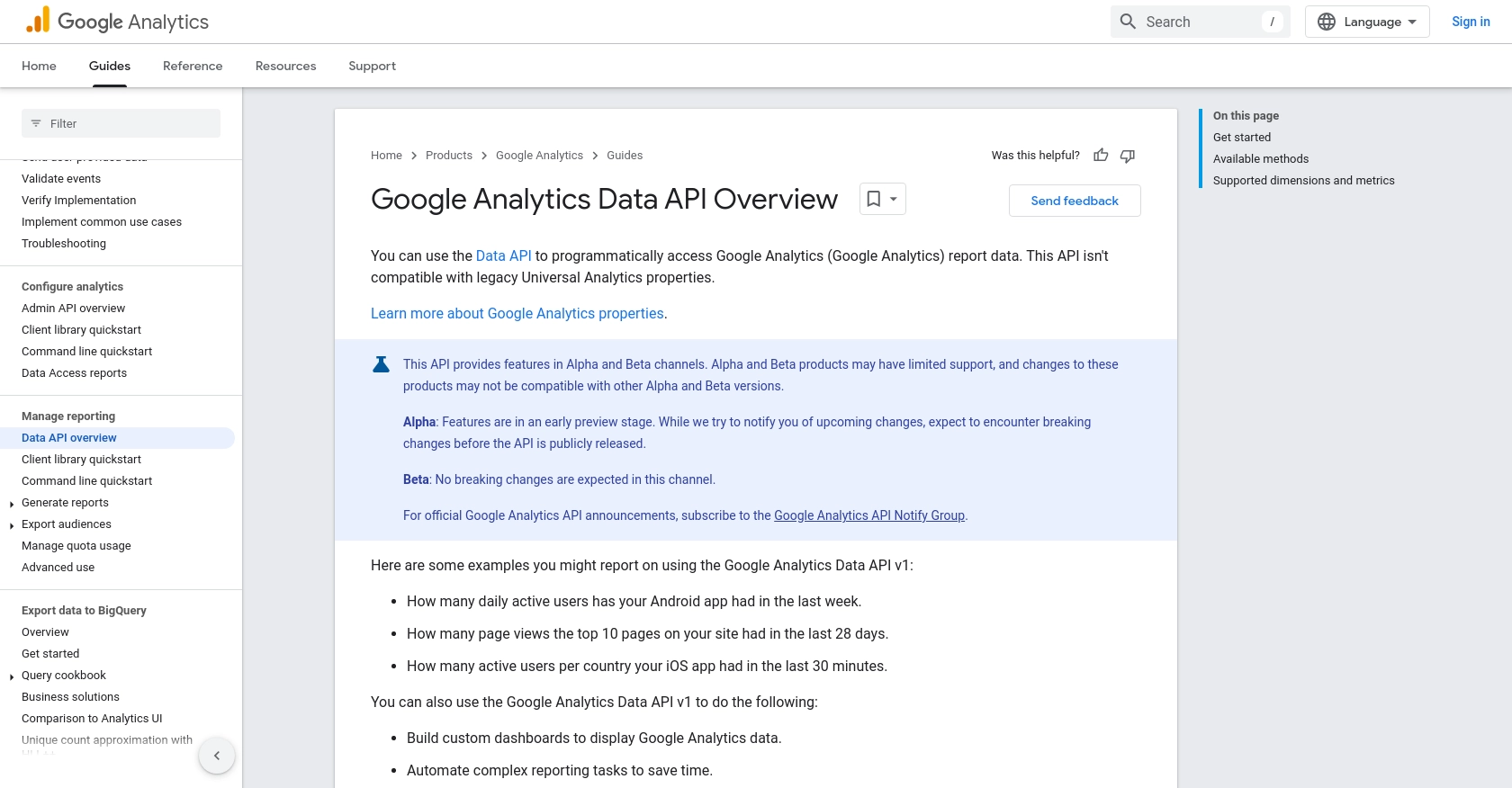
sbb-itb-96038d7
Making API Calls to Google Analytics Using Python
To interact with the Google Analytics API using Python, you'll need to set up your development environment and write code to make API requests. This section will guide you through the necessary steps to retrieve analytics data by page using Python.
Setting Up Your Python Environment for Google Analytics API
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need to install the Google Analytics Data API client library.
- Install the Google Analytics Data API client library using pip:
pip install google-analytics-data
Writing Python Code to Retrieve Analytics Data by Page
With your environment set up, you can now write a Python script to retrieve analytics data by page. The following example demonstrates how to use the Google Analytics API to get page views for specific pages.
from google.analytics.data_v1beta import BetaAnalyticsDataClient from google.analytics.data_v1beta.types import ( DateRange, Dimension, Metric, RunReportRequest, ) def run_report(property_id="YOUR-GA4-PROPERTY-ID"): """Runs a report to get page views by page title.""" client = BetaAnalyticsDataClient() request = RunReportRequest( property=f"properties/{property_id}", dimensions=[Dimension(name="pageTitle")], metrics=[Metric(name="pageviews")], date_ranges=[DateRange(start_date="2023-09-01", end_date="2023-09-15")], ) response = client.run_report(request) print_run_report_response(response) def print_run_report_response(response): """Prints the results of a runReport call.""" print(f"{response.row_count} rows received") for dimension_header in response.dimension_headers: print(f"Dimension header name: {dimension_header.name}") for metric_header in response.metric_headers: print(f"Metric header name: {metric_header.name} ({metric_header.type_})") print("Report result:") for row in response.rows: for dimension_value in row.dimension_values: print(f"Page Title: {dimension_value.value}") for metric_value in row.metric_values: print(f"Page Views: {metric_value.value}") # Replace 'YOUR-GA4-PROPERTY-ID' with your actual Google Analytics 4 property ID run_report("YOUR-GA4-PROPERTY-ID")
Replace YOUR-GA4-PROPERTY-ID
with your actual Google Analytics 4 property ID. This script retrieves page views for each page title within the specified date range.
Verifying API Call Success and Handling Errors
After running your script, verify the results by checking the console output. If the API call is successful, you should see the page titles and their corresponding page views. If there are errors, the API will return error codes that you can handle in your code.
Common error codes include:
- 400 Bad Request: The request was invalid or cannot be served. Check your request parameters.
- 401 Unauthorized: Authentication failed. Ensure your OAuth credentials are correct.
- 403 Forbidden: You do not have permission to access the requested resource.
For more detailed error handling, refer to the Google Analytics API documentation.
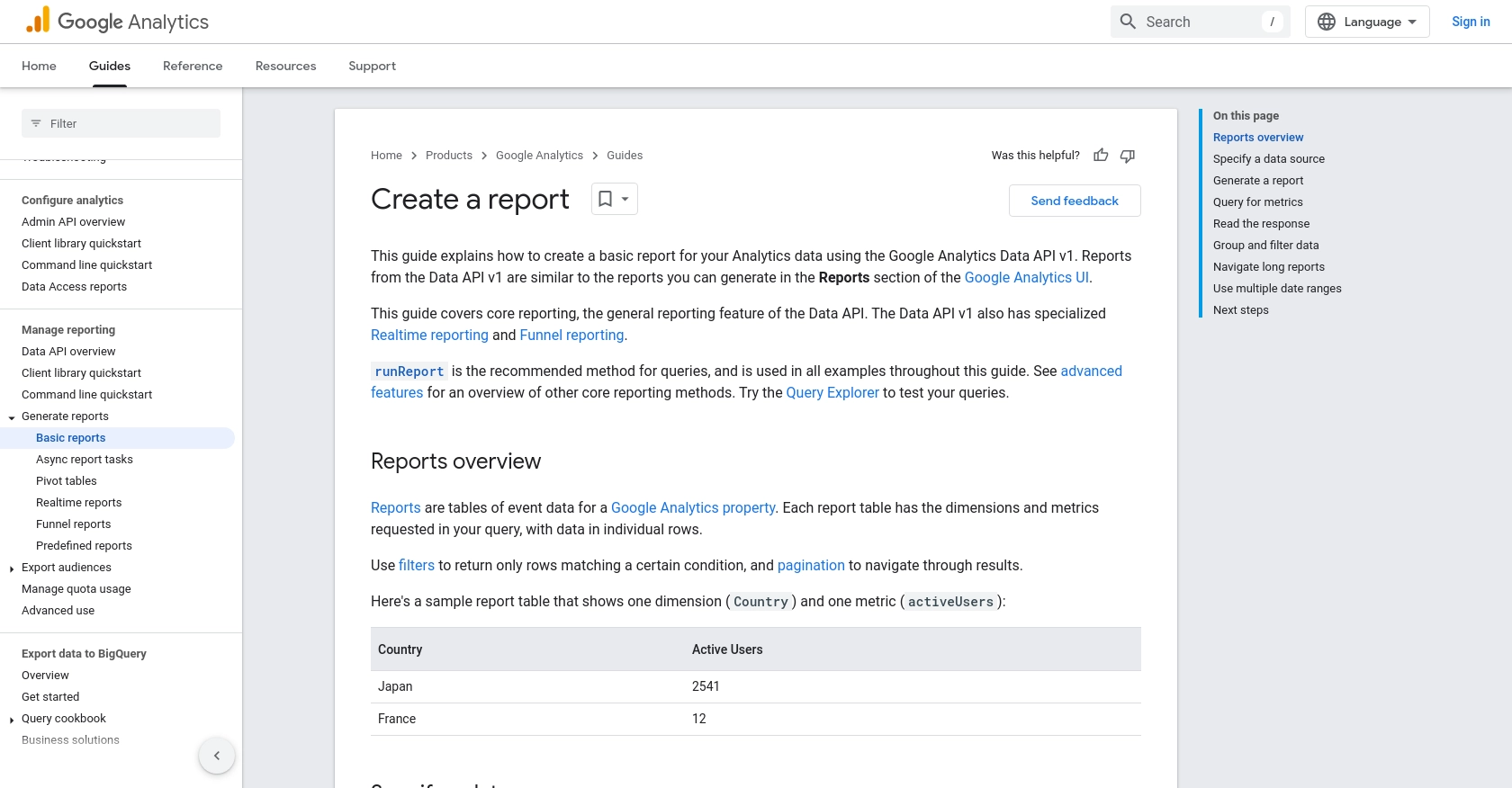
Best Practices for Using Google Analytics API with Python
When working with the Google Analytics API, it's essential to follow best practices to ensure efficient and secure integration. Here are some recommendations:
- Securely Store Credentials: Always store your OAuth client ID and client secret securely. Avoid hardcoding them in your source code. Consider using environment variables or a secure vault.
- Handle Rate Limiting: Google Analytics API has rate limits. Ensure your application handles these limits gracefully by implementing retry logic with exponential backoff. For more details, refer to the API documentation.
- Optimize Data Requests: Minimize the amount of data requested by specifying only the necessary dimensions and metrics. This reduces the load on the API and speeds up response times.
- Transform and Standardize Data: Consider transforming and standardizing the data fields to match your application's requirements. This can simplify data processing and integration with other systems.
Streamlining Integrations with Endgrate
While integrating Google Analytics API can be a powerful tool for data-driven insights, managing multiple integrations can become complex and time-consuming. This is where Endgrate can help.
Endgrate offers a unified API endpoint that connects to multiple platforms, including Google Analytics. By using Endgrate, you can streamline your integration processes, allowing your development team to focus on core product features rather than managing individual integrations.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Save time and resources by outsourcing integrations and focusing on what truly matters for your business.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/googleanalytics
- https://developers.google.com/analytics/devguides/reporting/data/v1
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/analytics/devguides/reporting/data/v1/basics
Ready to get started?