How to Send Messages with the SMTP API in Python
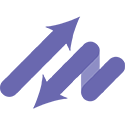
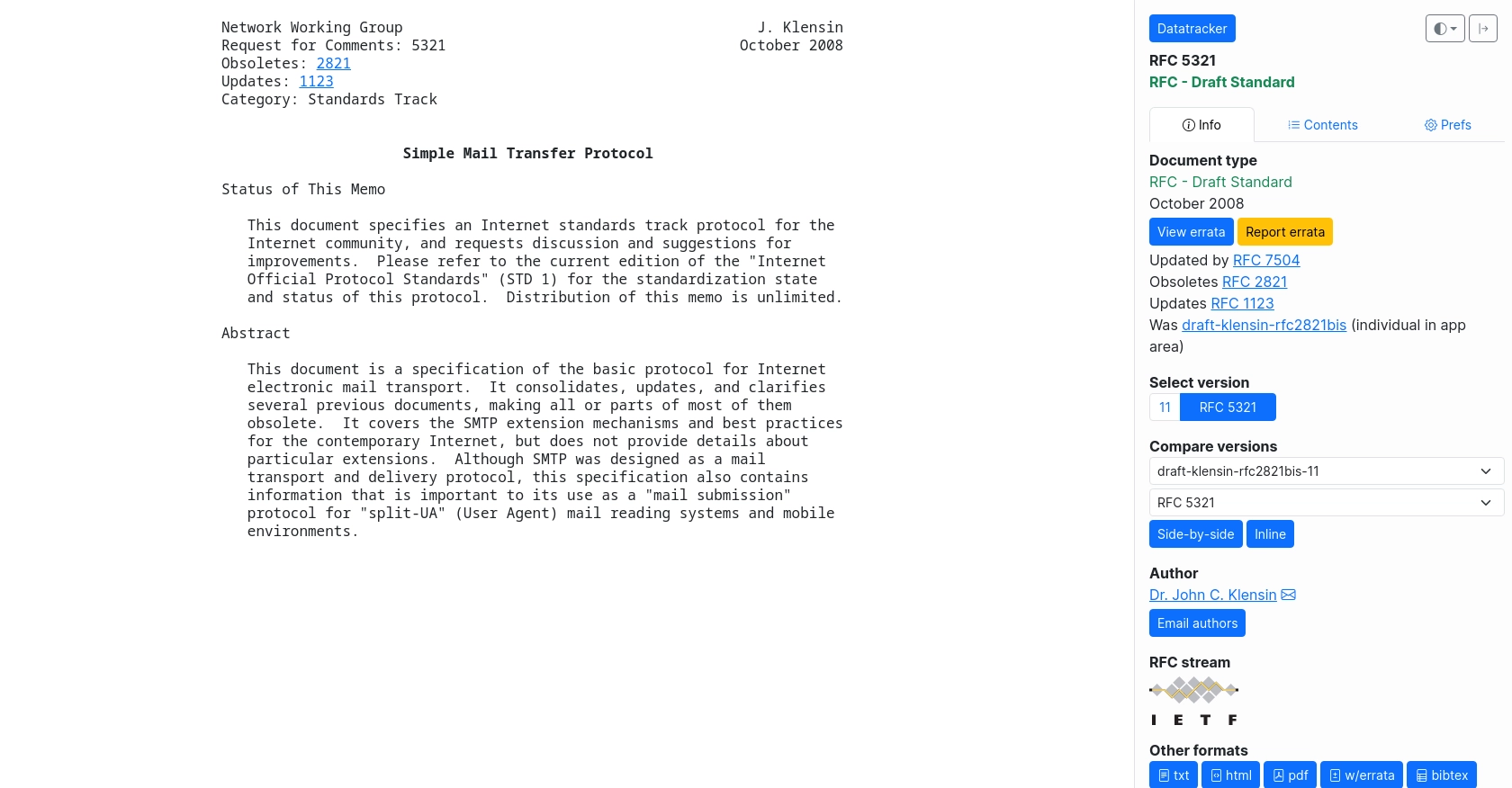
Introduction to SMTP and Its Messaging Capabilities
SMTP, or Simple Mail Transfer Protocol, is a widely used protocol for sending emails across the Internet. It serves as the backbone for email communication, enabling the transmission of messages from a client to a server or between servers. SMTP is essential for developers looking to integrate email functionalities into their applications, providing a reliable and efficient way to send messages programmatically.
Developers may want to connect with SMTP to automate email notifications, send transactional emails, or manage bulk email campaigns. For example, a developer could use the SMTP API to send automated order confirmations to customers after a purchase, enhancing the customer experience and streamlining business operations.
Setting Up Your SMTP Test Environment
Before you can start sending messages using the SMTP API in Python, it's essential to set up a test environment. This allows you to safely test your email functionalities without affecting your production environment.
Create a Test Email Account
To begin, you'll need a test email account. You can use any email provider that supports SMTP, such as Gmail, Outlook, or Yahoo. For this tutorial, we'll use Gmail as an example:
- Sign up for a Gmail account if you don't already have one.
- Enable "Less secure app access" in your Google account settings to allow SMTP connections.
Configure SMTP Settings
Once you have your test email account ready, you'll need to configure the SMTP settings. Here are the typical settings for Gmail:
- SMTP Server: smtp.gmail.com
- Port: 587 (for TLS) or 465 (for SSL)
- Username: Your Gmail email address
- Password: Your Gmail password
Set Up Python Environment
Ensure you have Python installed on your machine. You'll also need the smtplib
library, which is included in Python's standard library, so no additional installation is required.
Generate and Store Credentials Securely
It's crucial to handle your email credentials securely. Consider using environment variables or a configuration file to store your SMTP username and password. This practice helps prevent accidental exposure of sensitive information in your codebase.
With your test environment set up, you're ready to start sending messages using the SMTP API in Python. In the next section, we'll walk through the process of making an actual API call to send an email.
How to Make an SMTP API Call to Send Emails Using Python
Now that your test environment is ready, it's time to dive into sending emails using the SMTP API in Python. This section will guide you through the process, ensuring you understand each step involved in making a successful API call.
Understanding Python's smtplib for SMTP Communication
Python's smtplib
is a built-in library that simplifies the process of sending emails using the SMTP protocol. It provides a straightforward way to connect to an SMTP server, authenticate, and send messages. Since it's part of Python's standard library, you don't need to install any additional packages.
Writing the Python Code to Send an Email via SMTP
Let's create a Python script to send an email using the SMTP API. Follow the steps below to write and execute the code:
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
# SMTP server configuration
smtp_server = "smtp.gmail.com"
port = 587 # For TLS
username = "your_email@gmail.com"
password = "your_password"
# Email content
sender_email = "your_email@gmail.com"
receiver_email = "recipient@example.com"
subject = "Test Email from Python"
body = "This is a test email sent from a Python script using the SMTP API."
# Create a multipart message
message = MIMEMultipart()
message["From"] = sender_email
message["To"] = receiver_email
message["Subject"] = subject
# Attach the email body
message.attach(MIMEText(body, "plain"))
# Connect to the SMTP server and send the email
try:
server = smtplib.SMTP(smtp_server, port)
server.starttls() # Secure the connection
server.login(username, password)
server.sendmail(sender_email, receiver_email, message.as_string())
print("Email sent successfully!")
except Exception as e:
print(f"Error: {e}")
finally:
server.quit()
Executing the Python Script and Verifying the Email
Save the above code in a file named send_email.py
and run it using the following command in your terminal:
python send_email.py
If the script runs successfully, you should see "Email sent successfully!" in the terminal. Check the recipient's inbox to verify the email was received.
Handling Errors and Troubleshooting SMTP Issues
While sending emails, you might encounter errors. Here are some common issues and how to address them:
- Authentication Error: Ensure your username and password are correct and that "Less secure app access" is enabled in your email account settings.
- Connection Error: Verify that the SMTP server and port are correctly configured.
- Firewall/Network Issues: Check if your network allows outbound connections on the specified SMTP port.
By following these steps, you can effectively send emails using the SMTP API in Python, enhancing your application's communication capabilities.
Conclusion and Best Practices for Sending Emails with SMTP in Python
Integrating SMTP into your Python applications allows you to automate and enhance your email communication capabilities. By following the steps outlined in this guide, you can efficiently send emails using Python's smtplib
library, ensuring your messages reach their intended recipients.
Best Practices for Secure and Efficient SMTP Email Sending
- Secure Credential Storage: Always store your SMTP credentials securely, using environment variables or configuration files to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of your email provider's rate limits to avoid service disruptions. Implement logic to manage and retry requests when limits are reached.
- Data Standardization: Ensure that email content and recipient data are standardized to maintain consistency and avoid errors during transmission.
Enhance Your Integration Experience with Endgrate
While sending emails with SMTP in Python is straightforward, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint for various platforms, including SMTP. By leveraging Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an easy and intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration processes and enhance your application's capabilities by visiting Endgrate.
Read More
Ready to get started?