Using the Intercom API to Get Contacts in Python
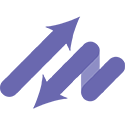
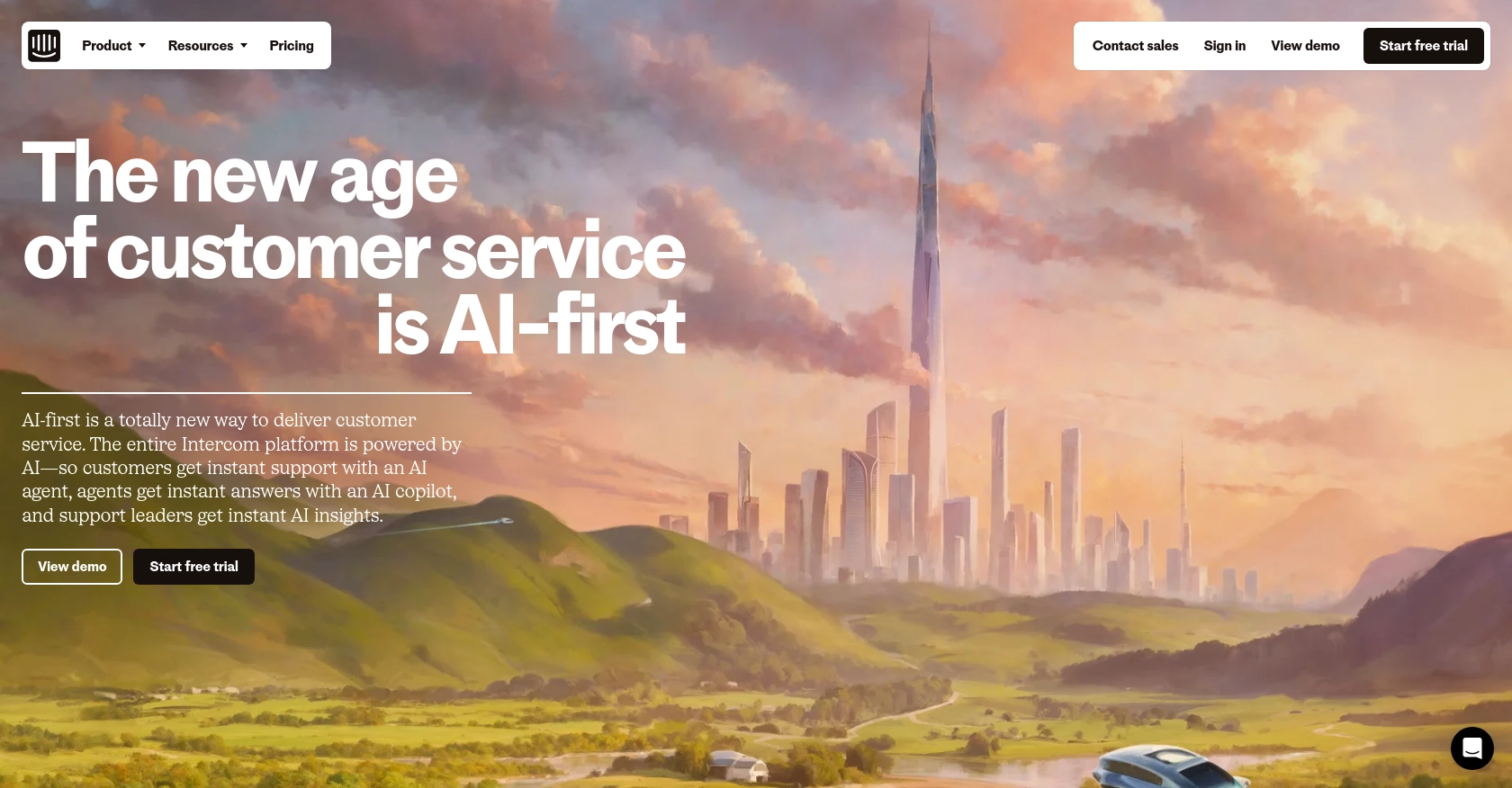
Introduction to Intercom API
Intercom is a powerful customer communication platform that enables businesses to engage with their users through messaging, email, and more. It offers a suite of tools designed to enhance customer support, marketing, and sales efforts, making it a popular choice for companies looking to improve their customer interactions.
Integrating with Intercom's API allows developers to access and manage customer data efficiently. For example, you can use the Intercom API to retrieve contact information, enabling personalized communication and targeted marketing campaigns. This integration can streamline processes and enhance the overall customer experience.
Setting Up Your Intercom Developer Account
Before you can start using the Intercom API to manage contacts, you'll need to set up a developer account. This involves creating a development workspace and configuring OAuth for authentication. Follow these steps to get started:
Create an Intercom Developer Workspace
- Visit the Intercom Developer Hub and sign up for a free developer account.
- Once registered, create a new development workspace. This workspace will allow you to test your integration without affecting live data.
- Navigate to the Developer Hub to access your workspace settings and manage your apps.
Configure OAuth for Intercom API Access
Intercom uses OAuth for secure authentication. Follow these steps to configure OAuth for your application:
- In your Developer Hub, go to the Authentication section and enable the Use OAuth option.
- Provide the necessary information, including redirect URLs. Ensure these URLs use HTTPS as required by Intercom.
- Select the permissions your app needs. For accessing contacts, ensure you have the necessary scopes checked, such as reading and writing user data.
- Save your settings to generate your Client ID and Client Secret. These credentials will be used to authenticate API requests.
Generate an Access Token
To interact with the Intercom API, you'll need an access token. Here's how to obtain it:
- Direct your users to the following URL to authorize your app:
https://app.intercom.com/oauth?client_id=YOUR_CLIENT_ID&state=YOUR_STATE
. - Once authorized, users will be redirected to your specified redirect URL with a code parameter.
- Exchange this code for an access token by making a POST request to
https://api.intercom.io/auth/eagle/token
with the following parameters:import requests url = "https://api.intercom.io/auth/eagle/token" data = { "code": "AUTHORIZATION_CODE", "client_id": "YOUR_CLIENT_ID", "client_secret": "YOUR_CLIENT_SECRET" } response = requests.post(url, data=data) access_token = response.json().get("access_token") print(access_token)
- Store the access token securely, as it will be used for all subsequent API requests.
With your Intercom developer account set up and OAuth configured, you're ready to start making API calls to manage contacts.
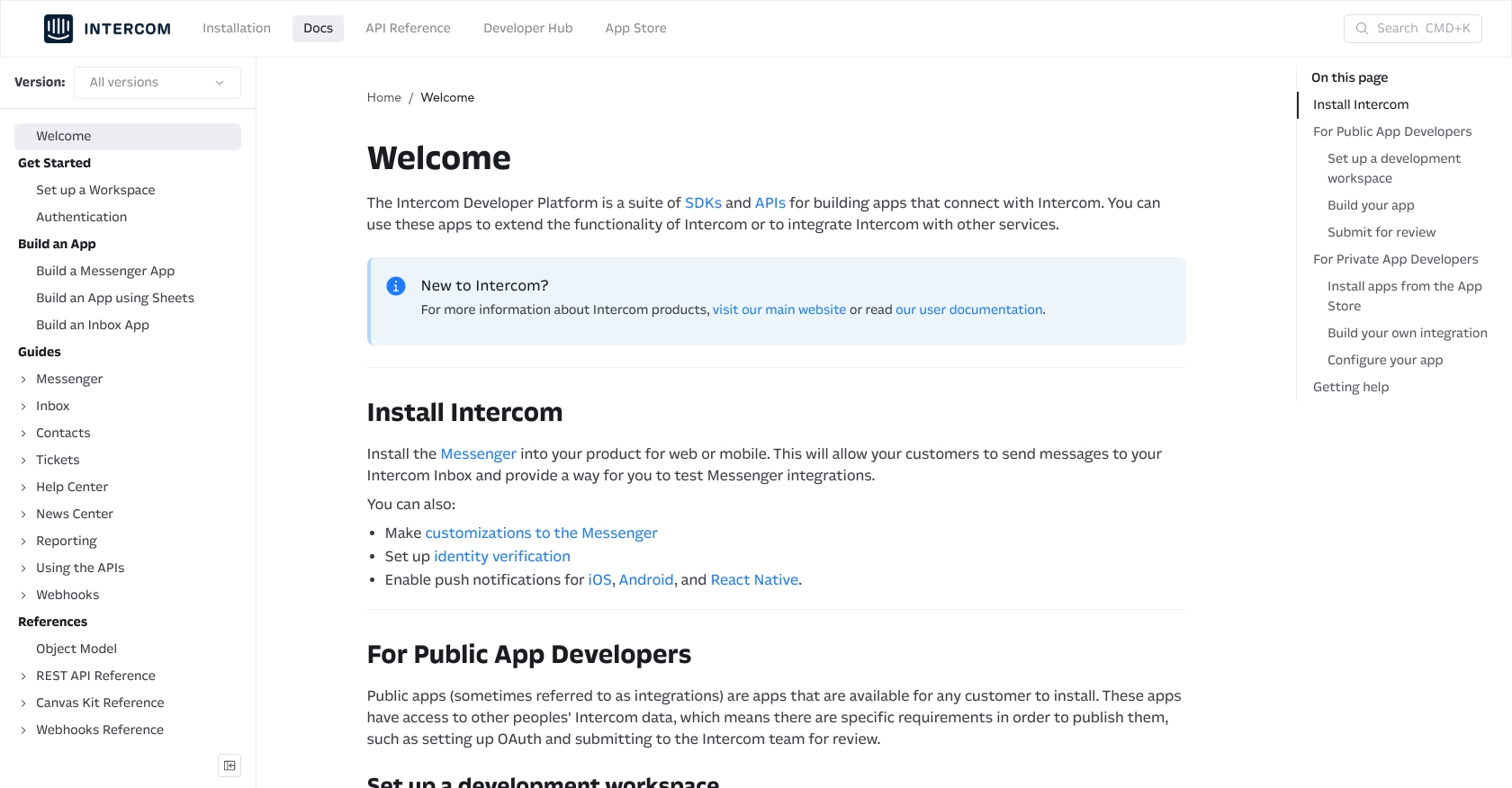
sbb-itb-96038d7
Making API Calls to Retrieve Contacts Using Intercom API in Python
To interact with the Intercom API and retrieve contacts, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses and errors.
Setting Up Your Python Environment for Intercom API Integration
Before making API calls, ensure you have Python 3.11.1 installed on your machine. You'll also need the requests
library to handle HTTP requests. Install it using the following command:
pip install requests
Writing Python Code to Fetch Contacts from Intercom
With your environment ready, you can now write the code to fetch contacts from Intercom. Create a file named get_intercom_contacts.py
and add the following code:
import requests
# Define the API endpoint and headers
url = "https://api.intercom.io/contacts"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Intercom-Version": "2.11"
}
# Make a GET request to the API
response = requests.get(url, headers=headers)
# Check if the request was successful
if response.status_code == 200:
contacts = response.json().get("data", [])
for contact in contacts:
print(contact)
else:
print(f"Failed to retrieve contacts: {response.status_code}")
Replace YOUR_ACCESS_TOKEN
with the access token you obtained earlier. This script sends a GET request to the Intercom API to retrieve a list of contacts. If successful, it prints each contact's information.
Verifying API Call Success and Handling Errors
After running the script, you should see the contacts listed in your console. To verify the request's success, check the status code. A status code of 200 indicates success, while other codes may indicate errors. Refer to the Intercom API documentation for detailed error code information.
Handling Common Errors and Error Codes
When working with the Intercom API, you may encounter common errors such as:
- 401 Unauthorized: Ensure your access token is valid and has the necessary permissions.
- 404 Not Found: Verify the endpoint URL and resource identifiers.
- 429 Too Many Requests: Implement rate limiting to avoid exceeding the API's request limits.
For more information on error handling, consult the Intercom API documentation.
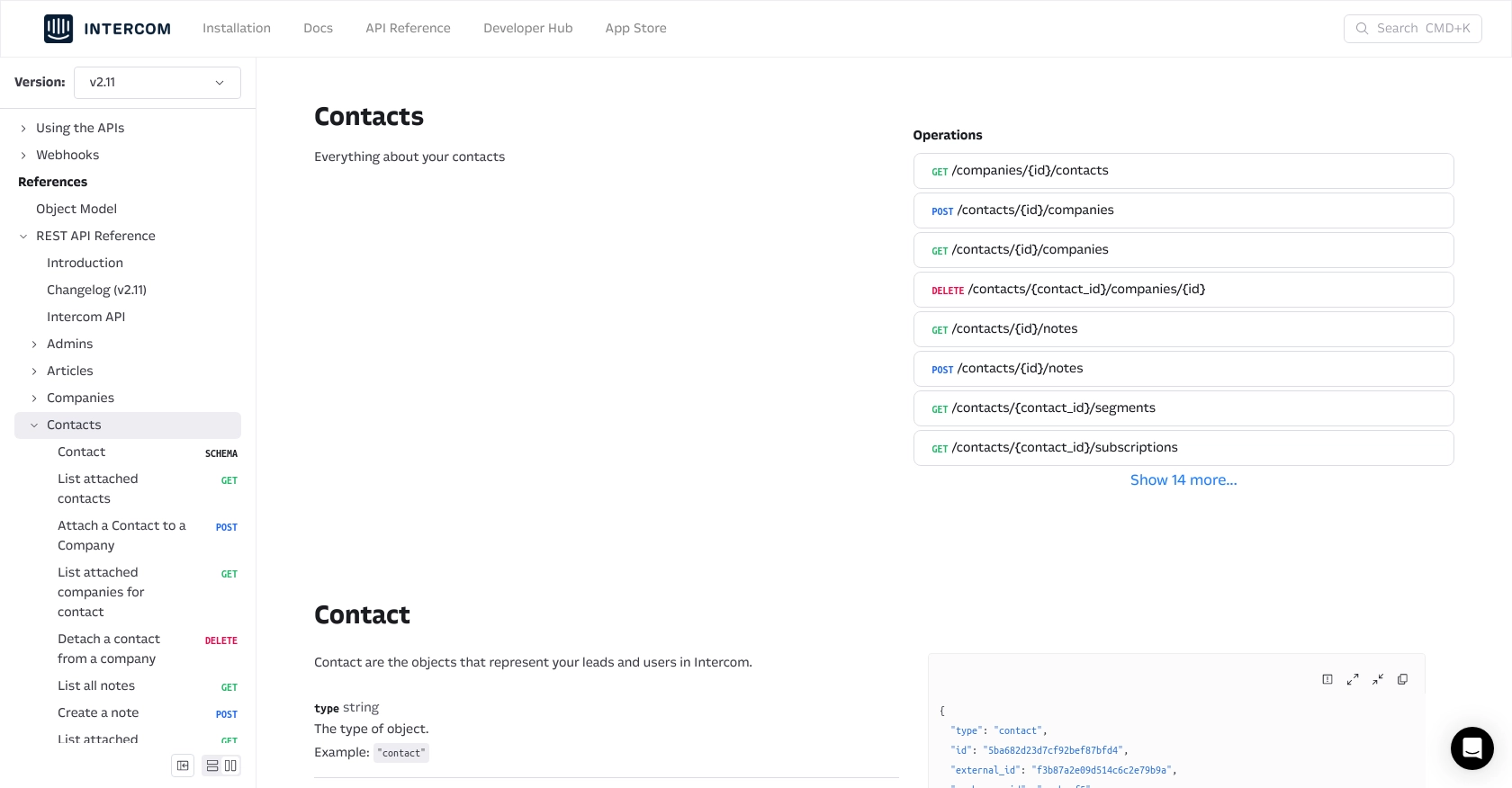
Conclusion and Best Practices for Using Intercom API in Python
Integrating with the Intercom API using Python can significantly enhance your ability to manage customer interactions and data. By following the steps outlined in this guide, you can efficiently retrieve contact information and leverage it for personalized communication and marketing strategies.
Best Practices for Secure and Efficient Intercom API Integration
- Securely Store Access Tokens: Ensure that your access tokens are stored securely and not exposed in your codebase. Consider using environment variables or secure vaults.
- Implement Rate Limiting: Be mindful of Intercom's rate limits to avoid 429 errors. Implement retry logic and backoff strategies to handle rate limiting gracefully.
- Standardize Data Fields: Consistently format and validate data fields to ensure compatibility with your application and Intercom's API requirements.
- Monitor API Usage: Regularly monitor your API usage and logs to identify any anomalies or potential issues in your integration.
Enhancing Your Integration Strategy with Endgrate
While building integrations with the Intercom API can be rewarding, it can also be time-consuming and complex. Endgrate offers a streamlined solution to manage multiple integrations efficiently. By using Endgrate, you can:
- Save time and resources by outsourcing integration management, allowing you to focus on your core product development.
- Build once for each use case and apply it across various platforms, reducing redundancy and effort.
- Provide an intuitive integration experience for your customers, enhancing their satisfaction and engagement.
Explore how Endgrate can simplify your integration processes by visiting Endgrate's website.
Read More
Ready to get started?