Using the Affinity API to Get Companies in Javascript
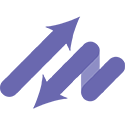
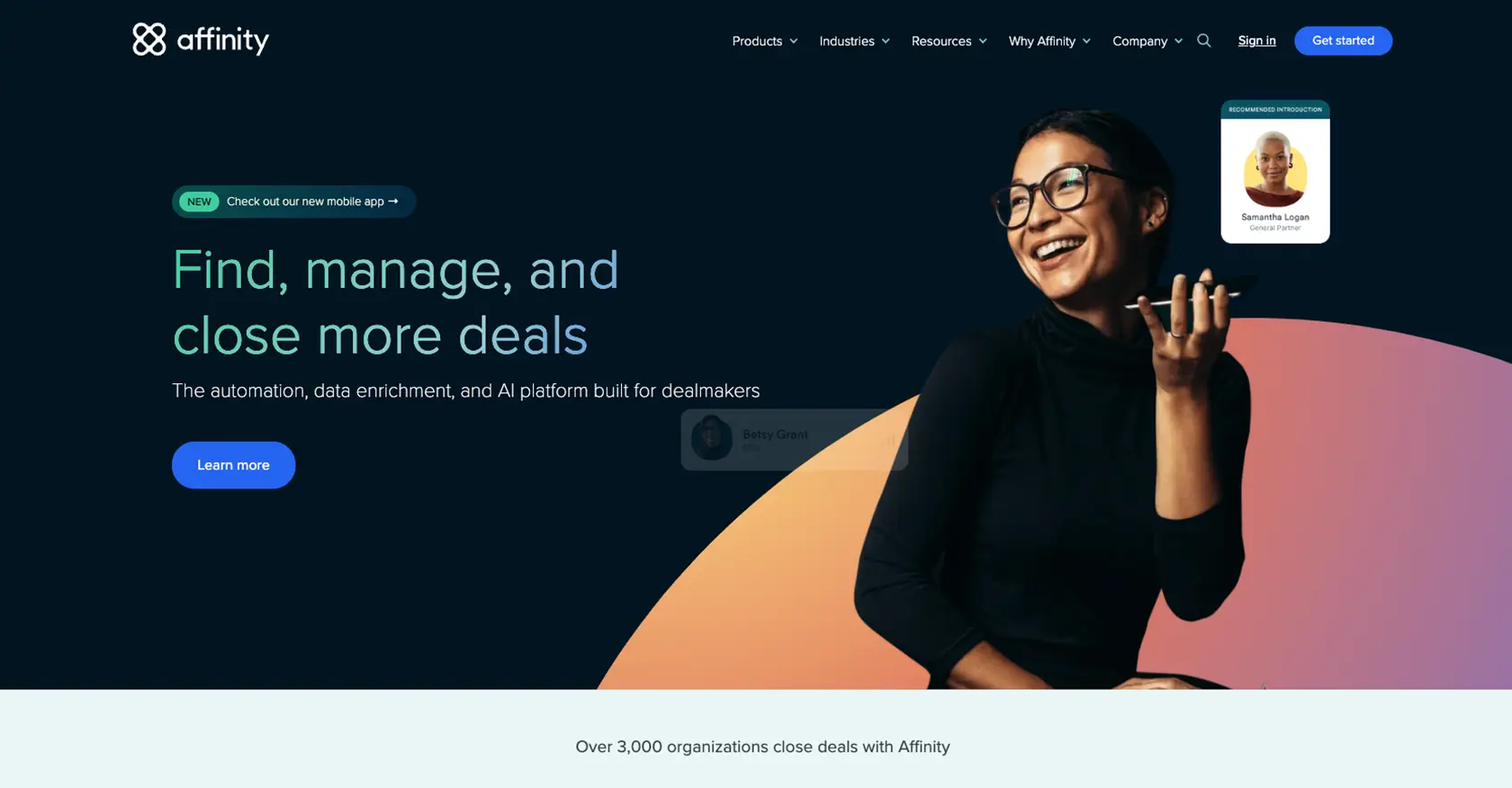
Introduction to Affinity API
Affinity is a powerful relationship intelligence platform designed to help businesses manage and leverage their professional networks. By providing insights into connections and interactions, Affinity enables companies to build stronger relationships and drive growth.
Integrating with the Affinity API allows developers to access and manage data related to people, organizations, and opportunities. For example, you can use the API to retrieve a list of companies your team is connected with, enabling you to analyze and enhance your business relationships.
This article will guide you through using JavaScript to interact with the Affinity API, specifically focusing on retrieving company data. By the end of this tutorial, you'll be equipped to efficiently access and utilize company information within your applications.
Setting Up Your Affinity API Account
Before you can start using the Affinity API to retrieve company data, you'll need to set up an account and obtain the necessary API key. This section will guide you through the process of creating an Affinity account and generating an API key for authentication.
Create an Affinity Account
If you don't already have an Affinity account, follow these steps to create one:
- Visit the Affinity website and click on the "Sign Up" button.
- Fill in the required information, such as your name, email address, and password.
- Follow the on-screen instructions to complete the registration process.
Generate an API Key for Affinity
Once your account is set up, you need to generate an API key to authenticate your requests to the Affinity API. Follow these steps:
- Log in to your Affinity account.
- Navigate to the "Settings" panel, accessible from the left sidebar.
- Locate the "API Keys" section and click on "Generate New API Key."
- Copy the generated API key and store it securely, as you'll need it for API requests.
Authenticate Using the Affinity API Key
The Affinity API uses HTTP Basic Auth for authentication. You will need to include your API key in the request header as the password. No username is required. Here's an example of how to authenticate using JavaScript:
// Example of making an authenticated request to the Affinity API
const apiKey = 'Your_Affinity_API_Key';
const endpoint = 'https://api.affinity.co/organizations';
fetch(endpoint, {
method: 'GET',
headers: {
'Authorization': `Basic ${btoa(':' + apiKey)}`,
'Content-Type': 'application/json'
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Replace Your_Affinity_API_Key
with the API key you generated earlier. This code snippet demonstrates how to set up the request headers for authentication and make a GET request to the Affinity API.
With your Affinity account set up and API key generated, you're now ready to start making API calls to retrieve company data.
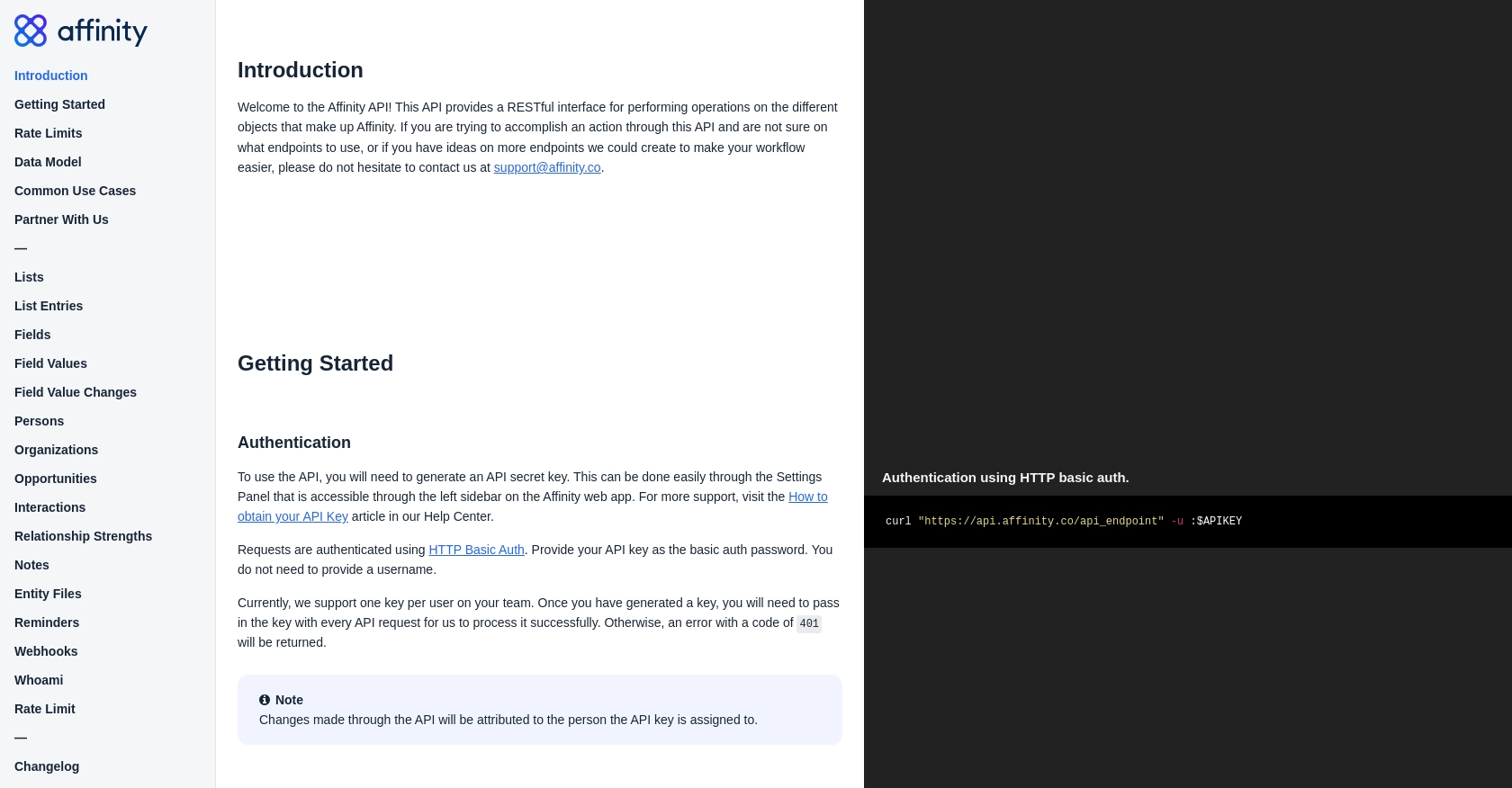
sbb-itb-96038d7
Executing API Calls to Retrieve Company Data Using Affinity API in JavaScript
To effectively interact with the Affinity API and retrieve company data, you'll need to understand how to make API calls using JavaScript. This section will guide you through setting up your environment, writing the necessary code, and handling responses and errors.
Setting Up Your JavaScript Environment for Affinity API
Before making API calls, ensure you have a suitable JavaScript environment. You can use Node.js or a browser-based environment. Make sure you have the fetch
API available, which is standard in modern browsers and can be used in Node.js with packages like node-fetch
.
Writing JavaScript Code to Call Affinity API
Below is a step-by-step guide to writing JavaScript code that interacts with the Affinity API to retrieve company data:
// Define your API key and endpoint
const apiKey = 'Your_Affinity_API_Key';
const endpoint = 'https://api.affinity.co/organizations';
// Function to fetch company data
async function fetchCompanies() {
try {
const response = await fetch(endpoint, {
method: 'GET',
headers: {
'Authorization': `Basic ${btoa(':' + apiKey)}`,
'Content-Type': 'application/json'
}
});
if (!response.ok) {
throw new Error(`Error: ${response.status} - ${response.statusText}`);
}
const data = await response.json();
console.log('Company Data:', data);
} catch (error) {
console.error('Failed to fetch companies:', error);
}
}
// Execute the function
fetchCompanies();
Replace Your_Affinity_API_Key
with your actual API key. This code snippet demonstrates how to set up the request headers for authentication, make a GET request, and handle the response.
Handling API Responses and Errors
When making API calls, it's crucial to handle responses and potential errors effectively:
- Success: If the request is successful, the response will contain the company data in JSON format. You can parse and use this data within your application.
- Errors: If the request fails, handle errors gracefully by checking the response status and displaying appropriate error messages. Common error codes include 401 (Unauthorized) and 429 (Too Many Requests).
Refer to the Affinity API documentation for more details on error codes and handling.
Verifying API Call Success in Affinity Sandbox
After executing your API call, verify the success by checking the data in your Affinity sandbox account. Ensure that the retrieved company data matches the expected results.
By following these steps, you can efficiently retrieve and utilize company data from the Affinity API within your JavaScript applications.
Conclusion and Best Practices for Using Affinity API in JavaScript
Integrating with the Affinity API using JavaScript provides a powerful way to access and manage company data, enhancing your ability to build and maintain professional relationships. By following the steps outlined in this guide, you can efficiently retrieve and utilize company information within your applications.
Best Practices for Secure and Efficient API Integration
- Secure API Key Storage: Always store your API keys securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limits: Be mindful of the Affinity API rate limits. Implement error handling for status code 429 (Too Many Requests) and consider implementing exponential backoff strategies to manage retries.
- Data Standardization: Ensure that the data retrieved from the API is standardized and transformed as needed to fit your application's data model. This will help maintain consistency and improve data quality.
Leverage Endgrate for Streamlined Integration Solutions
While integrating with the Affinity API can be straightforward, managing multiple integrations can become complex. Consider using Endgrate to simplify your integration processes. Endgrate offers a unified API endpoint that connects to various platforms, allowing you to focus on your core product while outsourcing integration tasks.
By leveraging Endgrate, you can save time and resources, providing an intuitive integration experience for your customers. Visit Endgrate to learn more about how it can benefit your business.
Read More
Ready to get started?