Using the Cloze API to Create Or Update Contacts (with PHP examples)
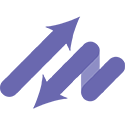
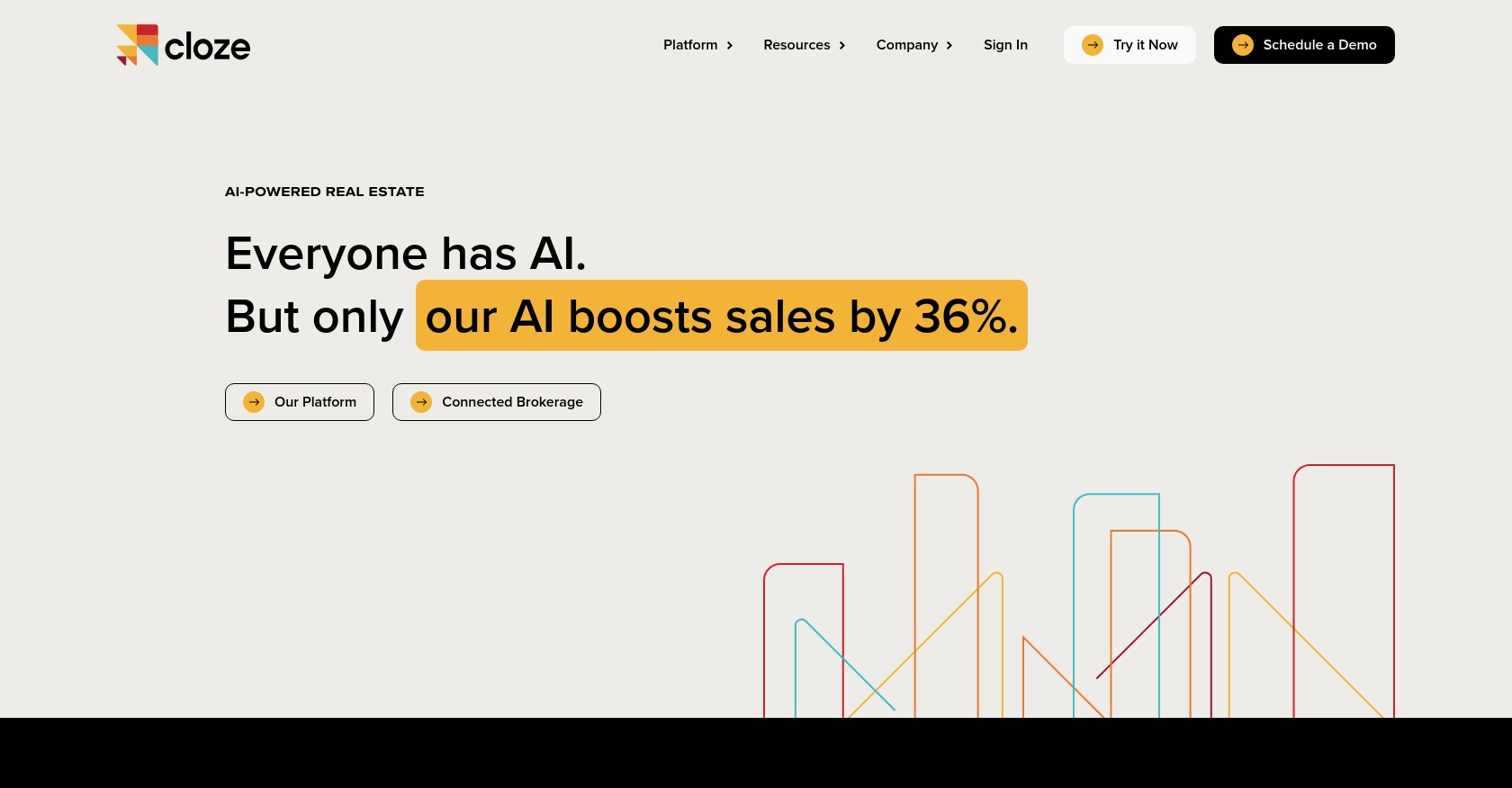
Introduction to Cloze API for Contact Management
Cloze is a powerful relationship management platform that helps businesses manage their contacts, projects, and communications seamlessly. It offers a comprehensive suite of tools designed to enhance productivity and streamline workflows, making it an ideal choice for businesses looking to optimize their relationship management processes.
Integrating with the Cloze API allows developers to automate and enhance contact management tasks, such as creating or updating contact information. For example, a developer might use the Cloze API to automatically update contact details from an external CRM system, ensuring that all contact information is current and accurate across platforms.
This article will guide you through using PHP to interact with the Cloze API for creating or updating contacts, providing detailed steps and code examples to help you integrate Cloze's capabilities into your applications effectively.
Setting Up Your Cloze API Test or Sandbox Account
Before you can start integrating with the Cloze API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Follow these steps to create and configure your Cloze account for API access.
Step 1: Sign Up for a Cloze Account
If you don't already have a Cloze account, visit the Cloze website and sign up for a free trial or demo account. This will provide you with access to the platform's features and allow you to test API interactions.
Step 2: Generate an API Key for Authentication
Once your account is set up, you'll need to generate an API key to authenticate your requests. Follow these steps:
- Log in to your Cloze account.
- Navigate to the settings section, typically found in the top navigation bar.
- Look for the API settings or developer section.
- Follow the instructions to create a new API key. You'll need to provide a name for the key and specify any necessary permissions.
- Once generated, copy the API key and store it securely. You'll use this key in your API requests.
For more detailed instructions, refer to the Cloze API documentation.
Step 3: Configure OAuth for Public Integrations
If you're planning to build a public integration intended for use by Cloze users outside your organization, you'll need to configure OAuth authentication. This involves:
- Contacting Cloze support at support@cloze.com to get started with OAuth setup.
- Following the provided instructions to register your application and obtain the necessary client ID and client secret.
- Implementing the OAuth flow in your application to allow users to authorize access to their Cloze data.
For initial development and testing, you can use the API key method before transitioning to OAuth for production environments.
Step 4: Test Your API Setup
With your API key or OAuth credentials ready, you can now test your setup by making a simple API call. Use the following PHP code snippet to verify your authentication:
Replace your_api_key_here
and your_email@domain.com
with your actual API key and email. Running this script should return your Cloze profile information, confirming that your setup is correct.
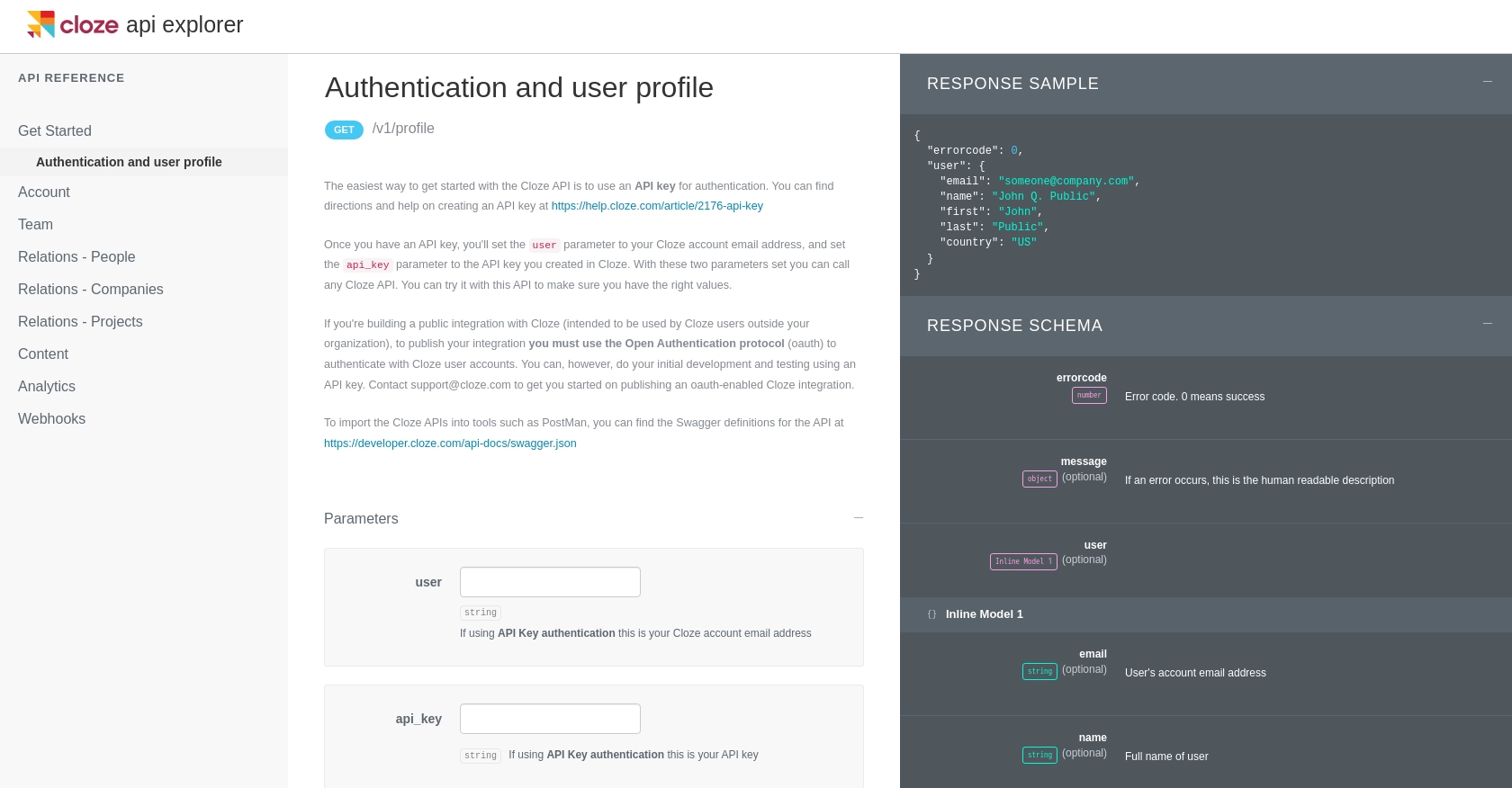
sbb-itb-96038d7
Making API Calls to Cloze for Contact Management Using PHP
In this section, we'll explore how to interact with the Cloze API using PHP to create or update contacts. This involves setting up your PHP environment, writing the necessary code, and handling responses and errors effectively.
Setting Up Your PHP Environment for Cloze API Integration
Before making API calls, ensure your PHP environment is ready. You'll need:
- PHP 7.4 or later
- cURL extension enabled
To verify cURL is enabled, run php -m
in your terminal and check for 'curl' in the output.
Installing Required PHP Dependencies
For this tutorial, we'll use PHP's built-in cURL functions. Ensure your PHP installation includes cURL support. If not, you may need to install or enable it in your PHP configuration.
Creating a New Contact in Cloze Using PHP
To create a new contact, use the following PHP code:
'John Doe',
'emails' => [
['value' => 'johndoe@example.com', 'work' => true]
],
'stage' => 'lead',
'segment' => 'customer'
];
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, 'https://api.cloze.com/v1/people/create');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($contactData));
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Bearer ' . $apiKey,
'Content-Type: application/json'
]);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
?>
Replace your_api_key_here
and your_email@domain.com
with your actual API key and email. This script sends a POST request to create a new contact with the specified details.
Updating an Existing Contact in Cloze Using PHP
To update an existing contact, modify the contact data and use the following PHP code:
'John Doe',
'emails' => [
['value' => 'johndoe@example.com', 'work' => true]
],
'stage' => 'current',
'segment' => 'partner'
];
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, 'https://api.cloze.com/v1/people/update');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($contactData));
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Bearer ' . $apiKey,
'Content-Type: application/json'
]);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
?>
This script updates the contact's stage and segment. Ensure the contact's unique identifier (e.g., email) is included in the data.
Handling API Responses and Errors
After executing an API call, check the response to ensure the request was successful. Cloze API responses include an errorcode
field, where 0 indicates success. Handle errors by checking this field and implementing appropriate error handling logic.
Example error handling:
By following these steps, you can effectively manage contacts in Cloze using PHP, ensuring seamless integration and data consistency across your applications.
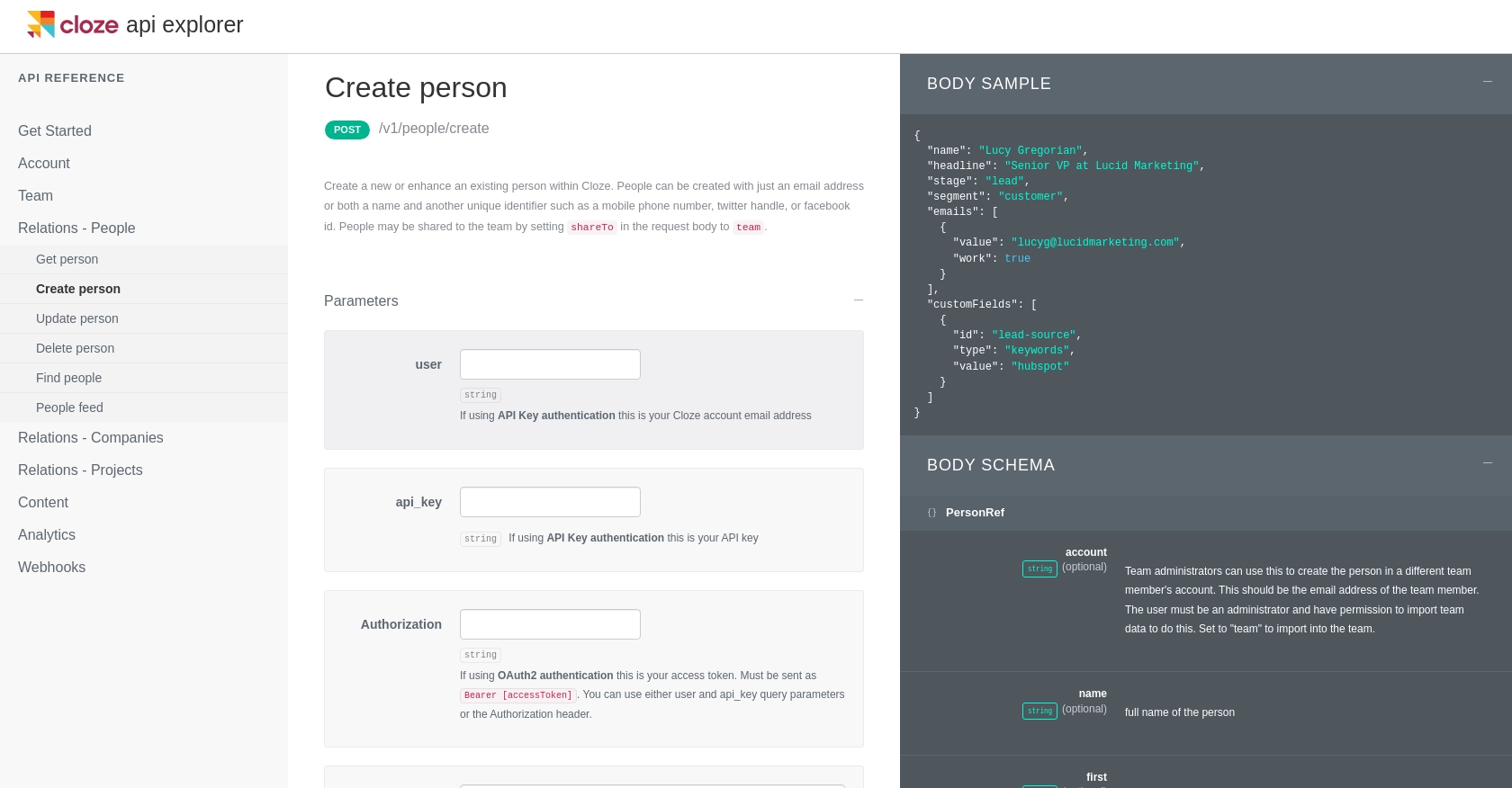
Conclusion and Best Practices for Using the Cloze API with PHP
Integrating the Cloze API into your applications using PHP can significantly enhance your contact management processes. By automating tasks such as creating and updating contacts, you can ensure data consistency and improve workflow efficiency across platforms.
Best Practices for Secure and Efficient API Integration
- Secure API Key Storage: Always store your API keys securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of API rate limits to avoid throttling. Implement exponential backoff strategies for retrying requests.
- Data Standardization: Ensure that data fields are standardized across systems to maintain consistency and accuracy.
- Error Handling: Implement robust error handling to manage API response errors and ensure smooth operation.
Streamlining Integration with Endgrate
For developers looking to simplify their integration processes, Endgrate offers a unified API solution that connects to multiple platforms, including Cloze. By using Endgrate, you can:
- Save time and resources by outsourcing integration tasks and focusing on core product development.
- Build once for each use case, avoiding repetitive work for different integrations.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website and discover the benefits of a unified API approach.
Read More
Ready to get started?