Using the Customer.io App API to Get Customers (with PHP examples)
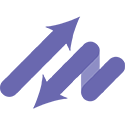
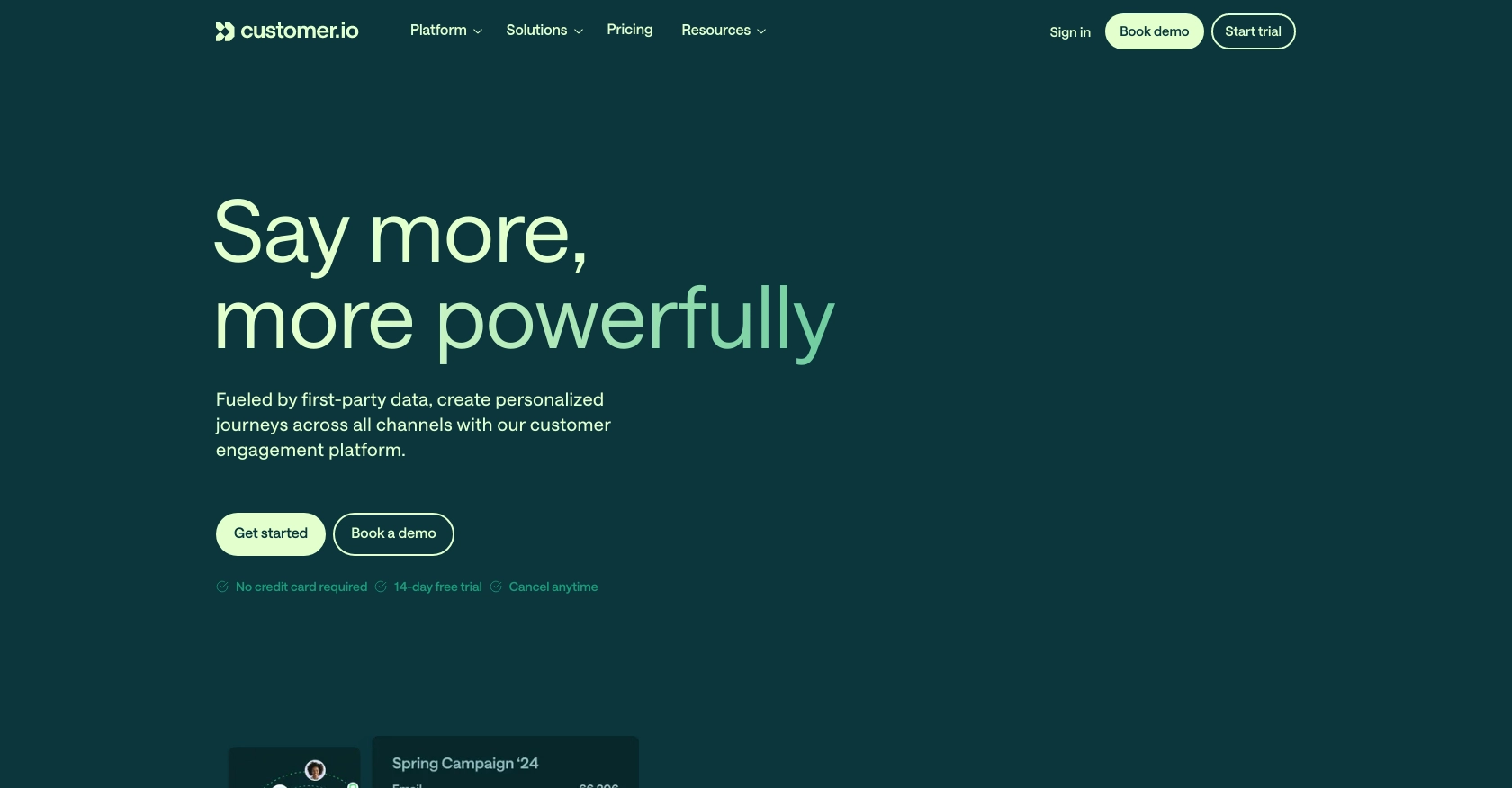
Introduction to Customer.io App API
Customer.io is a powerful marketing automation platform that enables businesses to send targeted messages to their customers. With its robust API, developers can seamlessly integrate Customer.io into their applications, allowing for personalized communication and enhanced customer engagement.
By leveraging the Customer.io App API, developers can access and manage customer data efficiently. This integration is particularly useful for automating marketing workflows, such as sending personalized emails based on customer behavior. For example, you could use the API to retrieve customer information and trigger a series of tailored messages, improving customer retention and satisfaction.
Setting Up Your Customer.io App Test/Sandbox Account
Before you can start using the Customer.io App API, it's essential to set up a test or sandbox account. This environment allows you to experiment with API calls without affecting your live data, ensuring a safe and controlled testing process.
Create a Customer.io Account
If you don't already have a Customer.io account, follow these steps to create one:
- Visit the Customer.io website and click on the "Start Trial" button.
- Fill in the required information, such as your name, email, and password, to create your account.
- Once registered, you'll receive a confirmation email. Follow the instructions in the email to verify your account.
Generate an API Key for Customer.io App
To interact with the Customer.io App API, you'll need to generate an API key. This key will be used to authenticate your requests:
- Log in to your Customer.io account and navigate to the "Account Settings" section.
- Under "API Keys," click on "Create API Key."
- Provide a name for your API key and select the appropriate permissions.
- Click "Generate" to create your API key. Make sure to copy and store it securely, as you'll need it for API requests.
Configure Your Test Environment
With your API key ready, you can now configure your test environment:
- Ensure you have PHP installed on your machine. You can download it from the official PHP website.
- Install the necessary PHP libraries, such as cURL, to make HTTP requests.
- Create a new PHP file where you'll write your API interaction code.
Example PHP Code for Customer.io App API Authentication
Here's a sample PHP code snippet to authenticate with the Customer.io App API using your API key:
<?php
$apiKey = 'your_api_key_here';
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, 'https://api.customer.io/v1/customers');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Bearer ' . $apiKey,
'Content-Type: application/json'
]);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
?>
Replace your_api_key_here
with the API key you generated earlier. This code initializes a cURL session, sets the necessary headers, and makes a GET request to the Customer.io API to retrieve customer data.
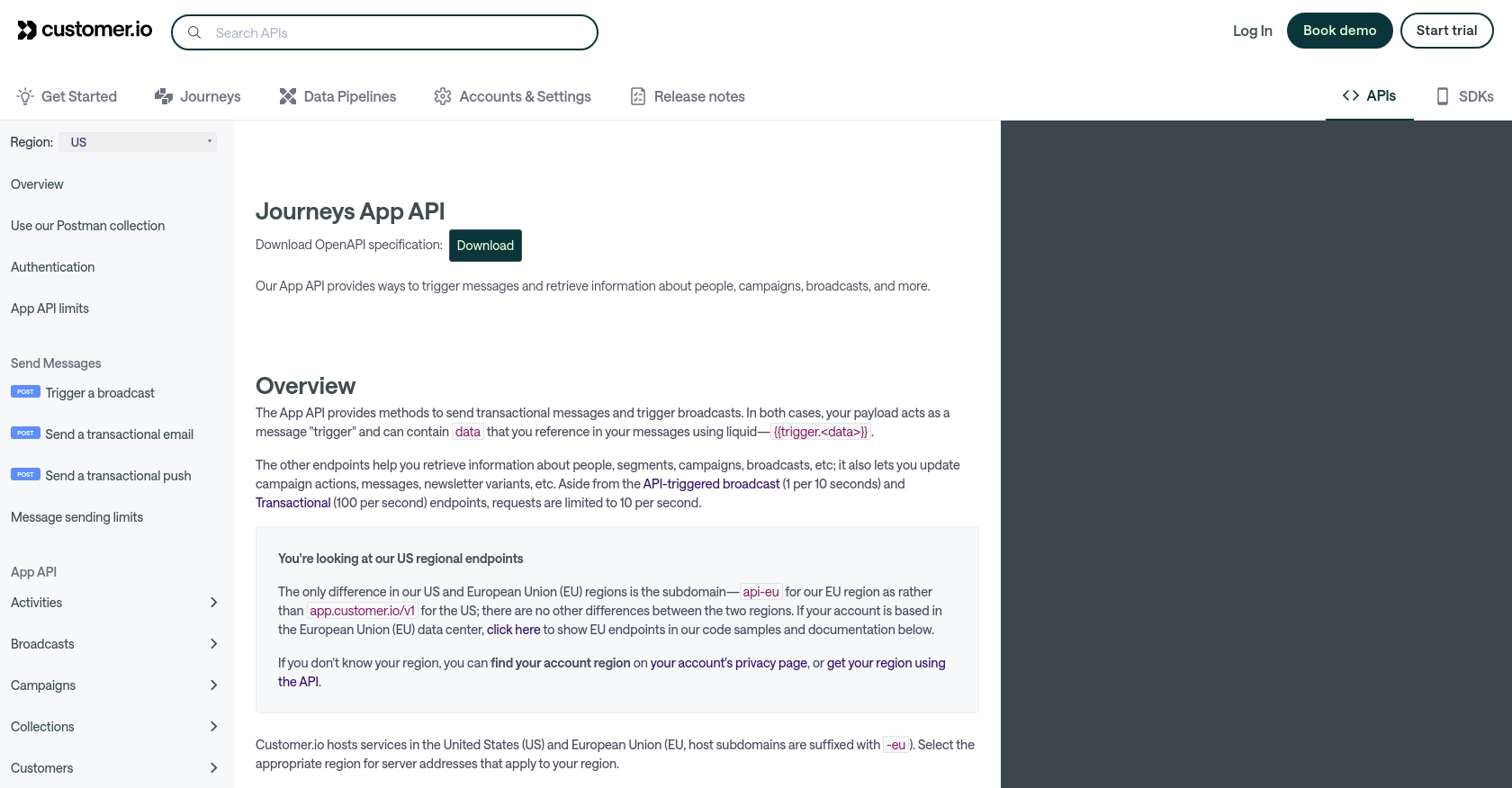
sbb-itb-96038d7
Making API Calls to Retrieve Customers Using Customer.io App API with PHP
To effectively interact with the Customer.io App API and retrieve customer data, you need to ensure your PHP environment is properly set up. This section will guide you through the process of making API calls using PHP, including necessary configurations and example code.
PHP Environment Setup for Customer.io App API
Before making API calls, ensure your PHP environment is ready:
- Ensure PHP is installed on your system. You can download it from the official PHP website.
- Install the cURL library for PHP, which is essential for making HTTP requests. You can do this using a package manager or by enabling it in your PHP configuration.
Example PHP Code to Retrieve Customers from Customer.io App API
Below is a sample PHP script to retrieve customer data from the Customer.io App API:
<?php
$apiKey = 'your_api_key_here';
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, 'https://api.customer.io/v1/customers');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Bearer ' . $apiKey,
'Content-Type: application/json'
]);
$response = curl_exec($ch);
curl_close($ch);
if ($response === false) {
echo 'Error: ' . curl_error($ch);
} else {
$customers = json_decode($response, true);
foreach ($customers['results'] as $customer) {
echo 'Customer ID: ' . $customer['id'] . '<br>';
echo 'Email: ' . $customer['email'] . '<br>';
}
}
?>
Replace your_api_key_here
with your actual API key. This script initializes a cURL session, sets the necessary headers, and sends a GET request to the Customer.io API to fetch customer data. It then processes the response and outputs customer details.
Handling API Response and Errors
It's crucial to handle API responses and potential errors effectively:
- Check if the cURL execution was successful. If not, use
curl_error()
to retrieve the error message. - Parse the JSON response using
json_decode()
and handle the data as needed. - Ensure to handle HTTP status codes and errors returned by the API to manage retries or log issues.
Verifying API Call Success in Customer.io App
After executing the API call, verify the success by checking the returned data:
- Ensure the response contains the expected customer data.
- Log into your Customer.io account and verify that the data matches the API response.
By following these steps, you can efficiently retrieve customer data using the Customer.io App API with PHP, enabling seamless integration and enhanced customer engagement.
Conclusion and Best Practices for Using Customer.io App API with PHP
Integrating the Customer.io App API into your PHP applications can significantly enhance your marketing automation capabilities, allowing for personalized and timely customer interactions. By following the steps outlined in this guide, you can efficiently retrieve and manage customer data, ensuring a seamless integration experience.
Best Practices for Secure and Efficient API Integration
- Secure API Key Management: Always store your API keys securely and avoid hardcoding them directly into your source code. Consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of the API's rate limits, which are set at 10 requests per second. Implement retry logic and backoff strategies to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage API response errors and HTTP status codes effectively, ensuring your application can recover gracefully from failures.
Enhance Your Integration Strategy with Endgrate
While integrating with the Customer.io App API can be highly beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration management, allowing you to focus on your core product development. By leveraging Endgrate, you can streamline your integration processes, reduce development time, and provide a seamless experience for your users.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?