How to Get Organizations with the Insightly API in Python
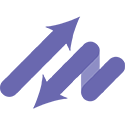
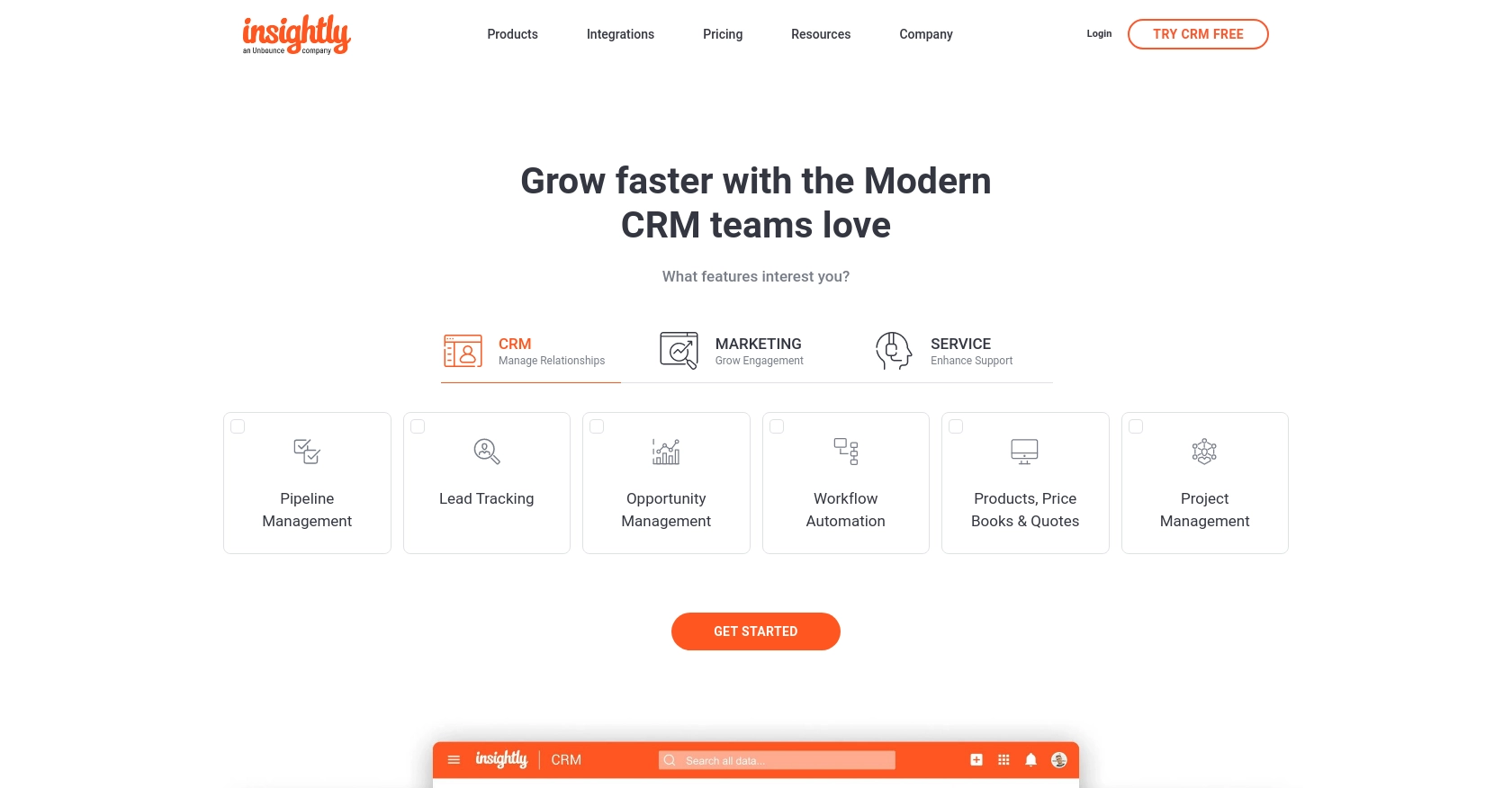
Introduction to Insightly API Integration
Insightly is a powerful CRM platform that helps businesses manage relationships and streamline operations. It offers a suite of tools for project management, contact tracking, and sales automation, making it a popular choice for organizations looking to enhance their customer engagement strategies.
Integrating with the Insightly API allows developers to access and manipulate data within the Insightly platform, enabling seamless integration with other systems. For example, a developer might use the Insightly API to retrieve organization data, which can then be used to generate detailed reports or synchronize with other business applications.
This article will guide you through the process of using Python to interact with the Insightly API, specifically focusing on retrieving organization information. By following this tutorial, you'll learn how to efficiently access and manage organization data within Insightly, enhancing your application's capabilities.
Setting Up Your Insightly Test Account
Before you can start interacting with the Insightly API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Insightly provides a straightforward process for setting up a test account, which is essential for development and testing purposes.
Creating an Insightly Account
If you don't already have an Insightly account, you can sign up for a free trial on the Insightly website. Follow the on-screen instructions to create your account. Once your account is set up, you can log in and access the Insightly dashboard.
Generating Your Insightly API Key
Insightly uses HTTP Basic authentication, which requires an API key to authenticate requests. Follow these steps to obtain your API key:
- Log in to your Insightly account.
- Navigate to your user profile by clicking on your user icon in the upper right corner.
- Select User Settings from the dropdown menu.
- In the API Key and URL section, you will find your API key. If it's not visible, click on Generate New API Key to create one.
- Copy the API key and store it securely, as you'll need it to authenticate your API requests.
For more detailed instructions, you can refer to the Insightly support documentation.
Configuring Your API Key for Authentication
To use your API key with the Insightly API, you need to include it in the Authorization header of your HTTP requests. The API key should be Base64-encoded and used as the username, with the password field left blank. Here's how you can set it up in Python:
import base64
# Your Insightly API key
api_key = 'your_api_key_here'
# Encode the API key
encoded_key = base64.b64encode(api_key.encode('utf-8')).decode('utf-8')
# Set the headers for the request
headers = {
'Authorization': f'Basic {encoded_key}'
}
By following these steps, you will be ready to make authenticated requests to the Insightly API using your test account. This setup ensures that you can safely develop and test your integration without impacting your live data.
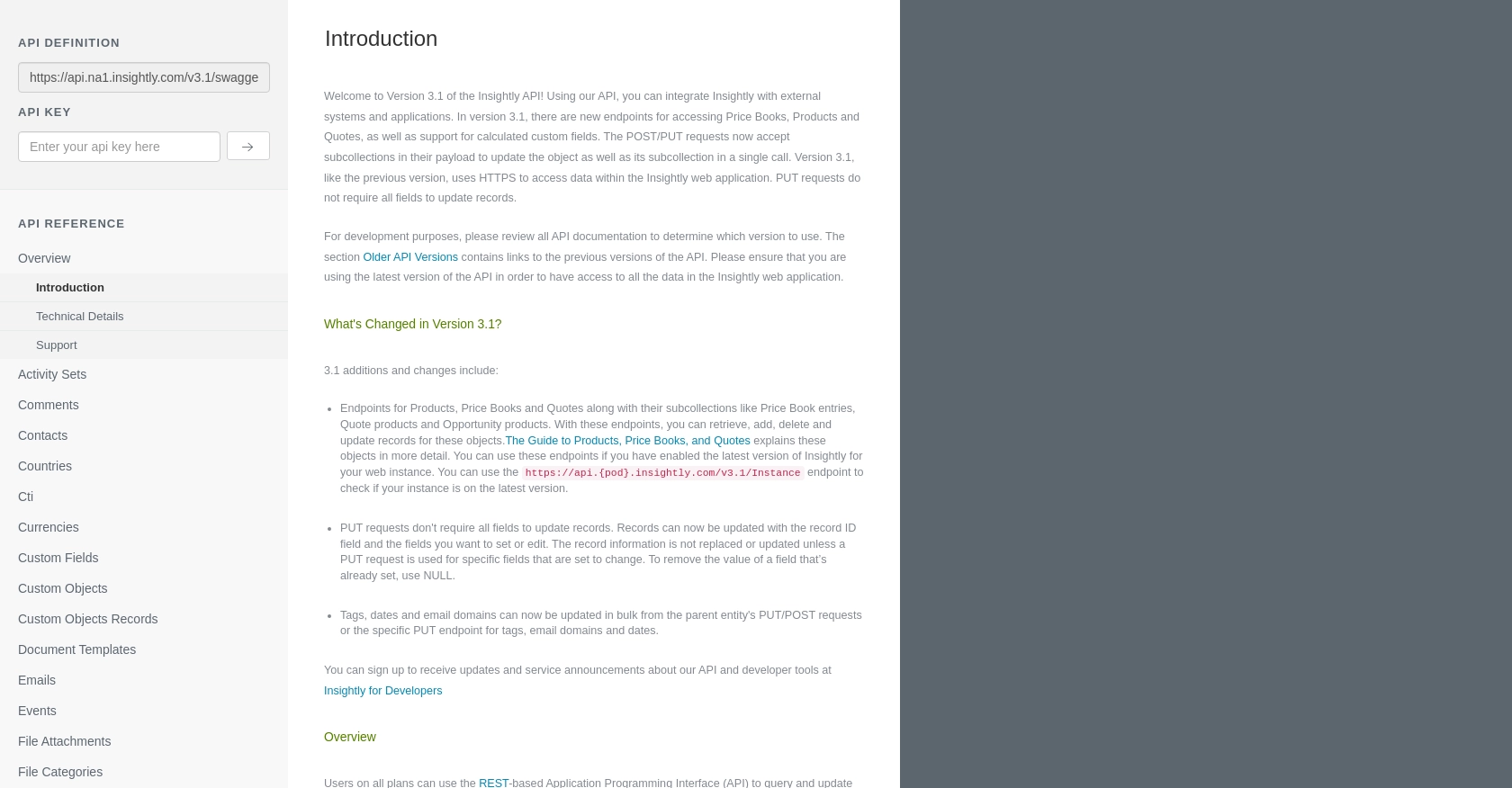
sbb-itb-96038d7
Making API Calls to Retrieve Organizations with Insightly API in Python
To interact with the Insightly API and retrieve organization data, you'll need to use Python to make HTTP requests. This section will guide you through the process of setting up your Python environment, writing the code to make API calls, and handling the responses effectively.
Setting Up Your Python Environment for Insightly API Integration
Before you begin, ensure that you have Python 3.x installed on your machine. You will also need the requests
library to handle HTTP requests. You can install it using pip:
pip install requests
Writing Python Code to Retrieve Organizations from Insightly
Once your environment is set up, you can write a Python script to make a GET request to the Insightly API and retrieve organization data. Here's a step-by-step guide:
import requests
import base64
# Your Insightly API key
api_key = 'your_api_key_here'
# Encode the API key
encoded_key = base64.b64encode(api_key.encode('utf-8')).decode('utf-8')
# Set the API endpoint and headers
endpoint = 'https://api.na1.insightly.com/v3.1/Organisations'
headers = {
'Authorization': f'Basic {encoded_key}'
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON data from the response
organizations = response.json()
# Loop through the organizations and print their information
for org in organizations:
print(f"Organization Name: {org['ORGANISATION_NAME']}")
else:
print(f"Failed to retrieve organizations. Status Code: {response.status_code}")
Replace your_api_key_here
with your actual Insightly API key. This script sets up the necessary headers for authentication and makes a GET request to the organizations endpoint. If successful, it prints the names of the organizations retrieved.
Verifying Successful API Requests and Handling Errors
After running your script, you should see a list of organization names printed in the console. This confirms that your API call was successful. If you encounter any errors, check the status code returned by the API:
- 200: Success
- 401: Authentication error (check your API key)
- 429: Rate limit exceeded (wait and try again later)
For more information on error codes, refer to the Insightly API documentation.
Handling Rate Limits and Optimizing API Calls
Insightly imposes rate limits on API requests to prevent abuse. The default limit is 10 requests per second, with daily limits based on your plan. If you exceed these limits, you'll receive a 429 status code. To handle rate limits:
- Implement retry logic with exponential backoff.
- Monitor the
X-RateLimit-Remaining
header to track your usage.
By following these best practices, you can efficiently manage your API calls and ensure smooth integration with Insightly.
Conclusion and Best Practices for Insightly API Integration
Integrating with the Insightly API using Python offers a powerful way to enhance your application's capabilities by accessing and managing organization data seamlessly. By following the steps outlined in this guide, you can efficiently retrieve organization information and ensure smooth interaction with the Insightly platform.
Best Practices for Secure and Efficient Insightly API Usage
- Secure Storage of API Keys: Always store your API keys securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handling Rate Limits: Implement retry logic with exponential backoff to manage rate limits effectively. Monitor the
X-RateLimit-Remaining
header to keep track of your API usage. - Data Transformation and Standardization: Ensure that data retrieved from Insightly is transformed and standardized to match your application's data structures for consistency.
Enhancing Integration Capabilities with Endgrate
While integrating with Insightly directly can be beneficial, using a tool like Endgrate can further streamline the process. Endgrate provides a unified API endpoint that connects to multiple platforms, including Insightly, allowing you to build integrations once and deploy them across various services. This approach saves time and resources, enabling you to focus on your core product development.
Explore how Endgrate can simplify your integration needs by visiting Endgrate and discover an intuitive integration experience for your customers.
Read More
Ready to get started?