Using the Younium API to Get Orders in Javascript
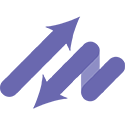
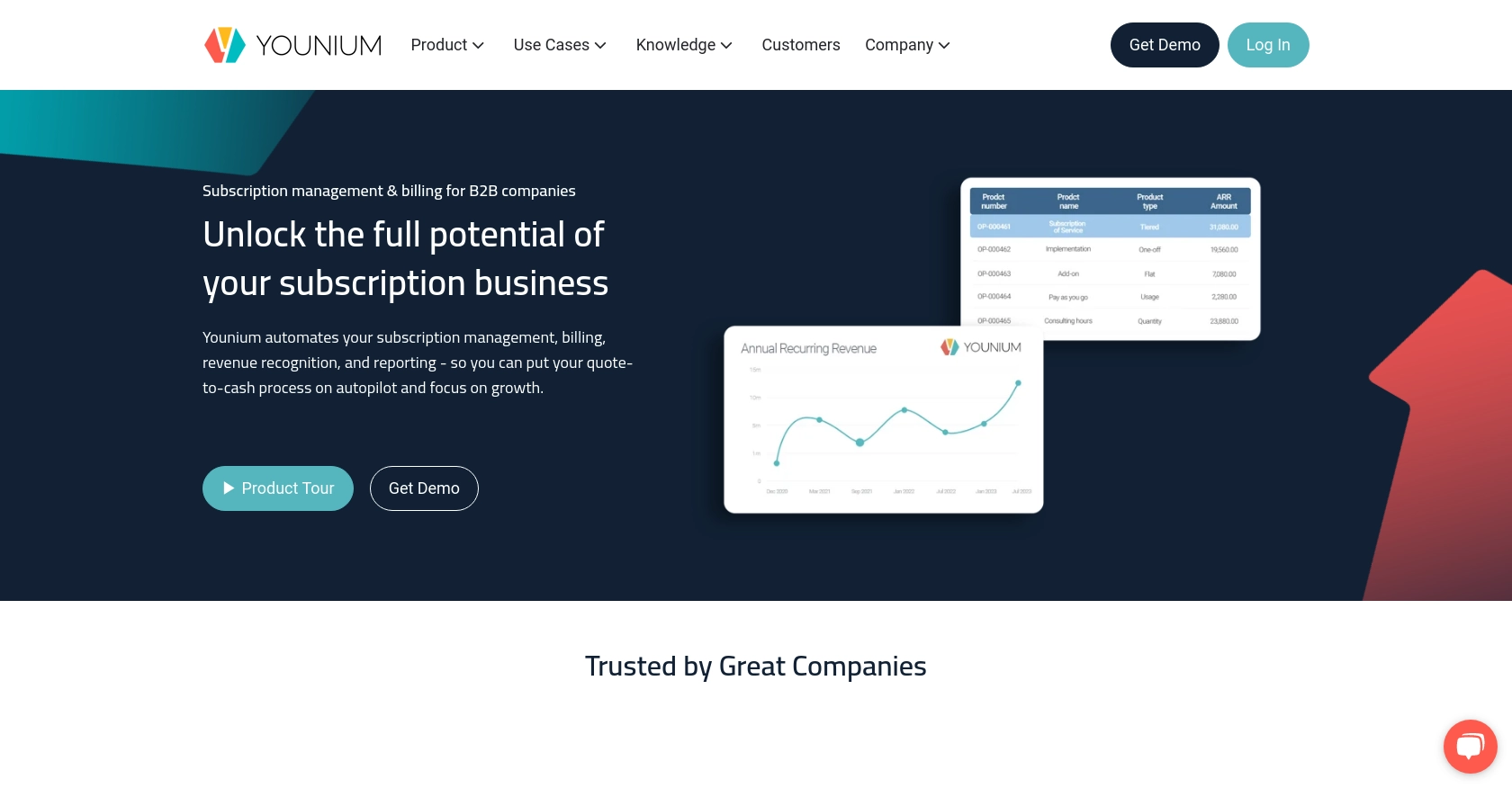
Introduction to Younium API for Order Management
Younium is a comprehensive subscription management platform designed to streamline billing and financial operations for B2B SaaS companies. It offers robust features for managing subscriptions, invoicing, and financial reporting, making it an essential tool for businesses looking to optimize their revenue operations.
Integrating with the Younium API allows developers to automate and enhance their order management processes. For example, by using the Younium API, developers can efficiently retrieve and manage sales orders, enabling seamless synchronization with other business systems. This integration can significantly reduce manual data entry, improve accuracy, and enhance overall operational efficiency.
Setting Up Your Younium Sandbox Account for API Integration
Before you can start using the Younium API to manage orders, you'll need to set up a sandbox account. This environment allows you to test and develop your integration without affecting live data. Follow these steps to get started:
Creating a Younium Sandbox Account
- Visit the Younium Developer Portal and sign up for a sandbox account if you haven't already.
- Follow the on-screen instructions to complete your registration and log in to your sandbox environment.
Generating API Credentials for Younium
To authenticate your API requests, you'll need to generate an API token and client credentials. Here's how:
- Click on your profile name in the top right corner and select "Privacy & Security."
- Navigate to "Personal Tokens" and click "Generate Token."
- Provide a relevant description and click "Create."
- Copy the generated Client ID and Secret Key. These credentials are crucial for obtaining your JWT access token.
Acquiring a JWT Access Token
With your client credentials ready, you can now generate a JWT access token:
// Example POST request to obtain JWT token
const axios = require('axios');
const getToken = async () => {
try {
const response = await axios.post('https://api.sandbox.younium.com/auth/token', {
clientId: 'Your_Client_ID',
secret: 'Your_Secret_Key'
}, {
headers: {
'Content-Type': 'application/json'
}
});
console.log('Access Token:', response.data.accessToken);
} catch (error) {
console.error('Error fetching token:', error.response.data);
}
};
getToken();
Replace Your_Client_ID
and Your_Secret_Key
with the credentials you generated earlier. This script will return an access token valid for 24 hours.
Handling Authentication Errors
If you encounter errors during authentication, refer to the following common issues:
- 401 Unauthorized: Indicates an expired or incorrect access token.
- 403 Forbidden: May occur if the legal entity is incorrect or permissions are insufficient.
For more details, visit the Younium Authentication Documentation.
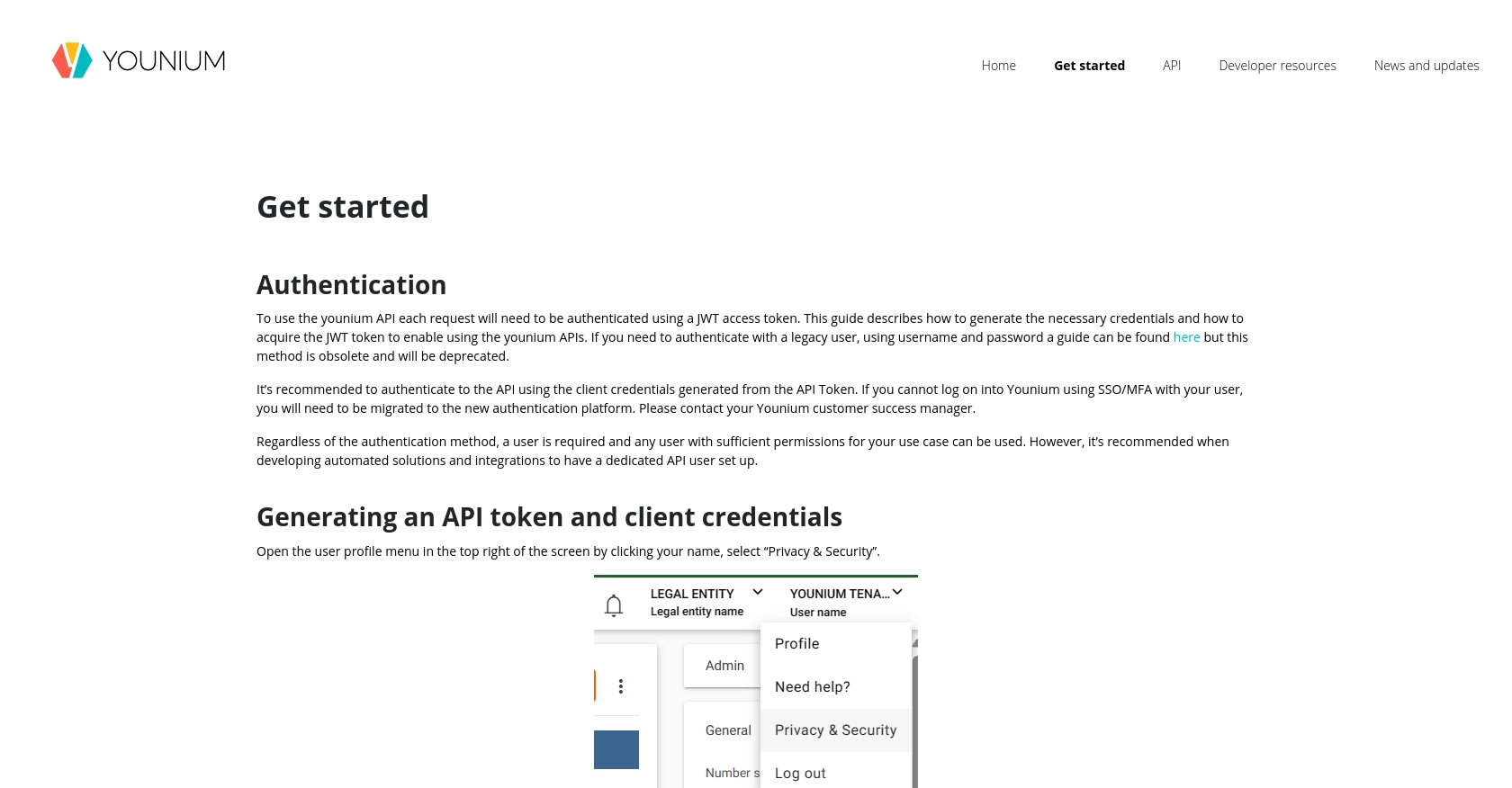
sbb-itb-96038d7
Making API Calls to Retrieve Younium Orders Using JavaScript
To interact with the Younium API and retrieve sales orders, you'll need to make authenticated API calls using JavaScript. This section will guide you through the process of setting up your environment, writing the necessary code, and handling potential errors.
Setting Up Your JavaScript Environment for Younium API Integration
Before making API calls, ensure you have Node.js installed on your machine. You will also need the Axios library to handle HTTP requests. Install Axios using the following command:
npm install axios
Writing JavaScript Code to Retrieve Younium Sales Orders
With your environment ready, you can now write the code to fetch sales orders from Younium. Create a file named getYouniumOrders.js
and add the following code:
const axios = require('axios');
const getOrders = async () => {
try {
const tokenResponse = await axios.post('https://api.sandbox.younium.com/auth/token', {
clientId: 'Your_Client_ID',
secret: 'Your_Secret_Key'
}, {
headers: {
'Content-Type': 'application/json'
}
});
const accessToken = tokenResponse.data.accessToken;
const ordersResponse = await axios.get('https://api.sandbox.younium.com/orders', {
headers: {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json',
'api-version': '2.1'
}
});
console.log('Sales Orders:', ordersResponse.data);
} catch (error) {
console.error('Error fetching orders:', error.response.data);
}
};
getOrders();
Replace Your_Client_ID
and Your_Secret_Key
with your actual credentials. This script first obtains a JWT access token and then uses it to fetch sales orders from the Younium API.
Verifying Successful API Requests in Younium Sandbox
After running the script, you should see the list of sales orders printed in your console. To verify the request's success, you can log into your Younium sandbox account and check the orders section to ensure the data matches.
Handling Errors and Common Issues with Younium API Calls
When making API calls, you may encounter errors. Here are some common issues and their solutions:
- 400 Bad Request: Check the request parameters and headers for any mistakes.
- 401 Unauthorized: Ensure your access token is valid and not expired.
- 403 Forbidden: Verify the legal entity specified in the headers is correct and that you have the necessary permissions.
For more detailed error handling, refer to the Younium API Documentation.
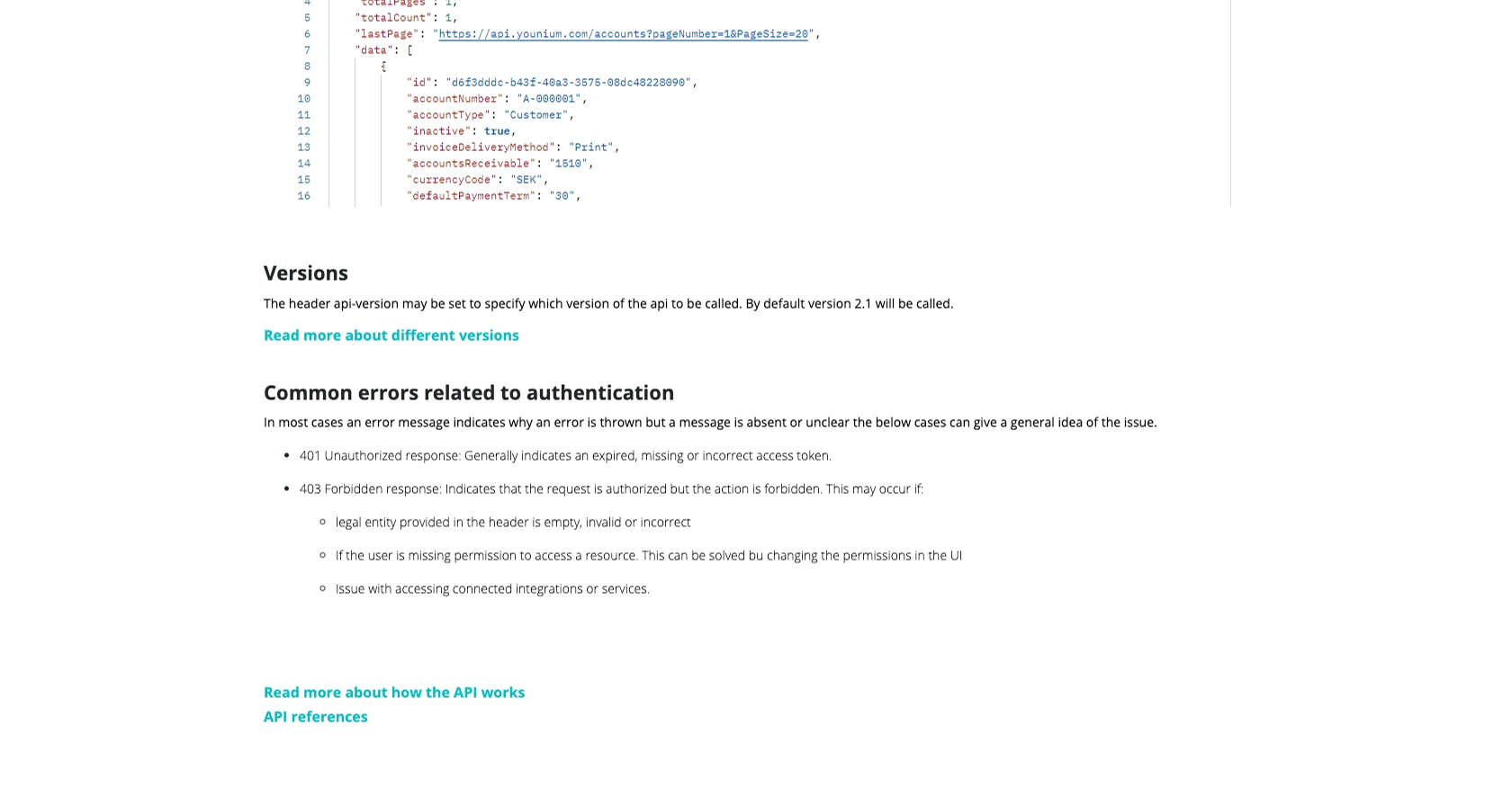
Conclusion and Best Practices for Using Younium API with JavaScript
Integrating with the Younium API using JavaScript can significantly enhance your order management processes by automating tasks and ensuring seamless data synchronization. By following the steps outlined in this guide, you can efficiently retrieve sales orders and integrate them with your existing systems.
Best Practices for Secure and Efficient Younium API Integration
- Securely Store Credentials: Always store your Client ID and Secret Key securely. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Standardize Data Fields: Ensure that the data retrieved from the API is standardized and transformed as needed to fit your application's data model.
- Monitor API Usage: Regularly monitor your API usage and performance to identify any potential issues or areas for optimization.
Leverage Endgrate for Streamlined Integration Solutions
While integrating with the Younium API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including Younium. By using Endgrate, you can save time and resources, allowing you to focus on your core product development while providing an intuitive integration experience for your customers.
Explore how Endgrate can help streamline your integration efforts by visiting Endgrate's website.
Read More
Ready to get started?