How to Get Organizations with the Insightly API in PHP
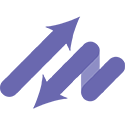
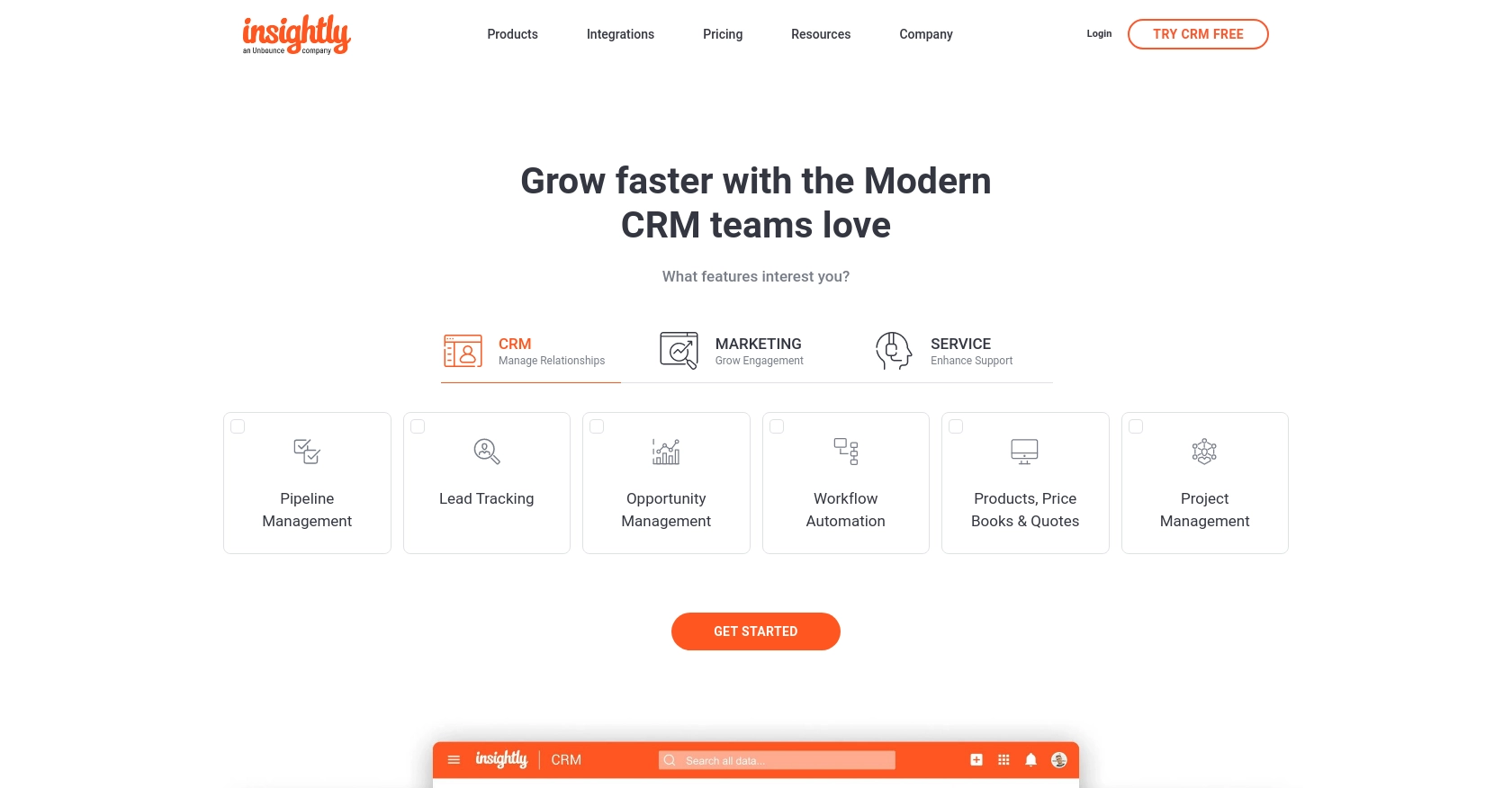
Introduction to Insightly CRM Integration
Insightly is a powerful CRM platform that helps businesses manage customer relationships, streamline workflows, and enhance productivity. With features like project management, lead tracking, and email integration, Insightly is a versatile tool for businesses aiming to improve their customer engagement strategies.
Integrating with the Insightly API allows developers to access and manipulate data within the CRM, enabling automation and customization of workflows. For example, a developer might want to retrieve organization data from Insightly to synchronize it with another system, ensuring that all customer information is up-to-date across platforms.
Setting Up Your Insightly Test Account
Before you can start interacting with the Insightly API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Creating an Insightly Account
If you don't already have an Insightly account, you can sign up for a free trial on the Insightly website. Follow the instructions to create your account. Once your account is set up, log in to access the Insightly dashboard.
Generating Your Insightly API Key
Insightly uses HTTP Basic authentication, which requires an API key to authenticate requests. Follow these steps to obtain your API key:
- Log in to your Insightly account.
- Click on your user profile in the upper right corner and select User Settings.
- Navigate to the API Key and URL section.
- Copy your API key from this section. You will use this key to authenticate your API requests.
For more details, refer to the Insightly API Key documentation.
Configuring Your API Key for Authentication
To authenticate your API requests, you need to include your API key as the Base64-encoded username in the HTTP Basic authentication header. The password field should be left blank. Here's how you can set it up in PHP:
$apiKey = 'your_api_key_here';
$authHeader = 'Authorization: Basic ' . base64_encode($apiKey . ':');
Ensure you replace your_api_key_here
with the actual API key you copied earlier.
Testing Your Setup
To verify that your setup is correct, you can make a simple API call to retrieve data. Use the following PHP code to test your connection:
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, 'https://api.na1.insightly.com/v3.1/Organisations');
curl_setopt($ch, CURLOPT_HTTPHEADER, array($authHeader));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
This code initializes a cURL session to the Insightly API endpoint for organizations, using your authentication header. If successful, it will return a list of organizations from your Insightly account.
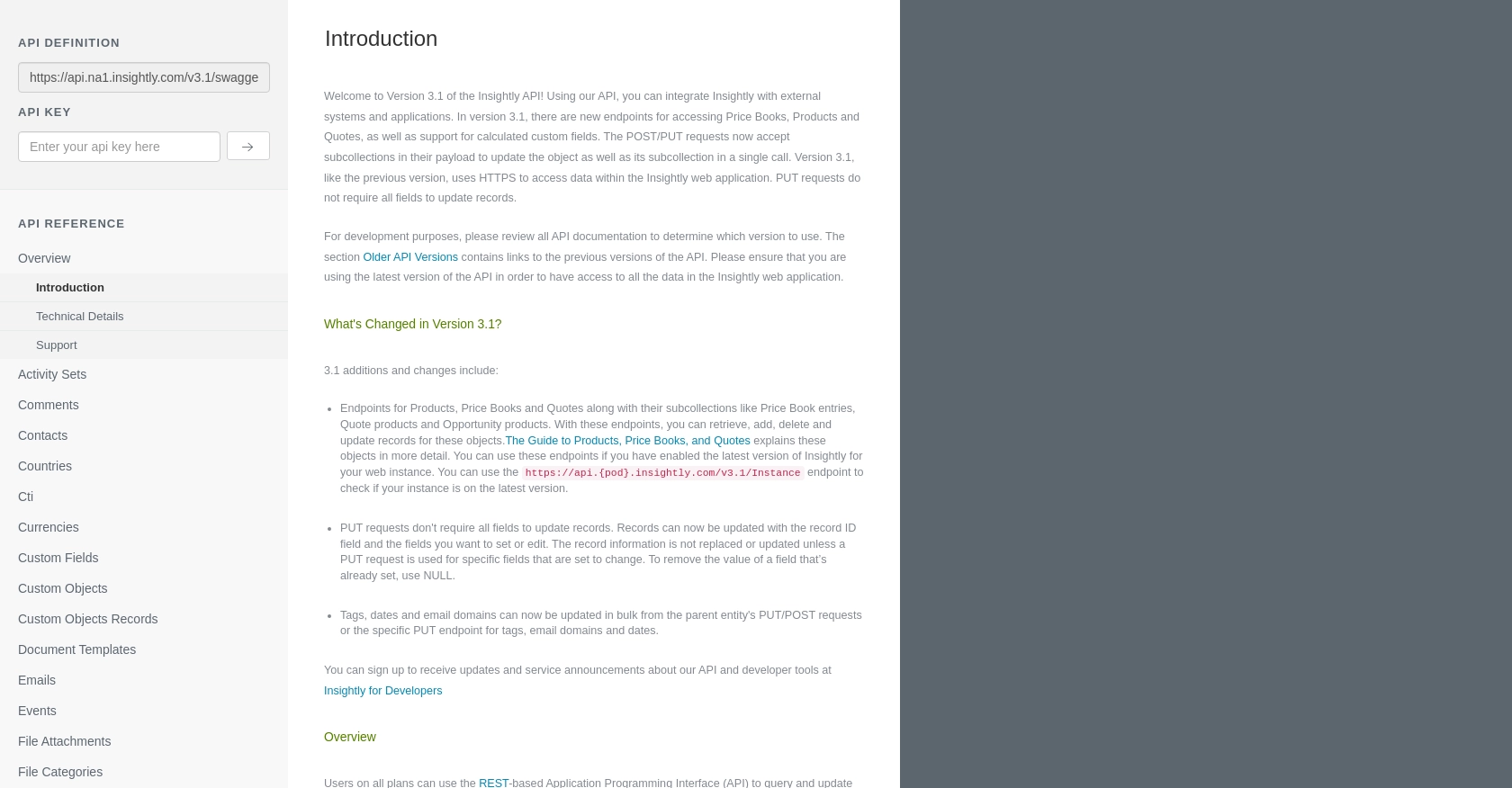
sbb-itb-96038d7
Making API Calls to Retrieve Organizations with Insightly API in PHP
To interact with the Insightly API using PHP, you'll need to ensure your environment is properly set up. This section will guide you through the necessary steps, including setting up PHP, installing dependencies, and executing API calls to retrieve organization data from Insightly.
Setting Up Your PHP Environment for Insightly API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or later
- cURL extension for PHP
To verify your PHP version and cURL installation, run the following commands in your terminal:
php -v
php -m | grep curl
Installing Necessary PHP Dependencies
For making HTTP requests, we'll use PHP's built-in cURL functions. Ensure that the cURL extension is enabled in your php.ini
file. If not, you can enable it by uncommenting the following line:
;extension=curl
Remove the semicolon to enable the extension:
extension=curl
Executing the Insightly API Call to Retrieve Organizations
With your environment ready, you can now proceed to make an API call to retrieve organizations from Insightly. Below is a sample PHP script demonstrating how to perform this task:
$apiKey = 'your_api_key_here';
$authHeader = 'Authorization: Basic ' . base64_encode($apiKey . ':');
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, 'https://api.na1.insightly.com/v3.1/Organisations');
curl_setopt($ch, CURLOPT_HTTPHEADER, array($authHeader));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
if ($httpCode == 200) {
$organizations = json_decode($response, true);
foreach ($organizations as $organization) {
echo 'Organization Name: ' . $organization['ORGANISATION_NAME'] . "<br>";
}
} else {
echo 'Failed to retrieve organizations. HTTP Status Code: ' . $httpCode;
}
}
curl_close($ch);
Replace your_api_key_here
with your actual Insightly API key. This script initializes a cURL session, sets the necessary options, and executes a GET request to the Insightly API endpoint for organizations. If successful, it will output the names of the organizations retrieved.
Handling Errors and Verifying API Call Success
It's crucial to handle potential errors when making API calls. The script above checks for cURL errors and HTTP status codes to ensure the request was successful. If the status code is 200, the request was successful, and the organization data is processed. Otherwise, an error message is displayed.
To verify the success of your API call, you can cross-check the retrieved data with the organizations listed in your Insightly account.
Understanding Insightly API Rate Limits
Keep in mind that Insightly imposes rate limits on API requests. According to the Insightly API documentation, the limit is 10 requests per second, with daily limits based on your plan. Exceeding these limits will result in a 429 status code. Plan your API usage accordingly to avoid hitting these limits.
Conclusion and Best Practices for Using the Insightly API in PHP
Integrating with the Insightly API using PHP allows developers to efficiently manage and synchronize organization data across platforms. By following the steps outlined in this guide, you can successfully authenticate and interact with the Insightly API to retrieve valuable information.
Best Practices for Secure and Efficient Insightly API Integration
- Securely Store API Keys: Always store your API keys securely, preferably in environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Insightly's rate limits. Implement logic to handle HTTP 429 status codes and consider using exponential backoff strategies to retry requests.
- Data Standardization: Ensure that data retrieved from Insightly is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement comprehensive error handling to manage potential API call failures and ensure robust application performance.
Enhance Your Integration Experience with Endgrate
While integrating with Insightly directly can be powerful, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and connect to multiple platforms effortlessly. Take advantage of an intuitive integration experience and save valuable time and resources. Visit Endgrate to learn more about how you can streamline your integration efforts.
Read More
Ready to get started?