Using the Zoho Books API to Create or Update Purchase Orders in Python
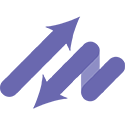
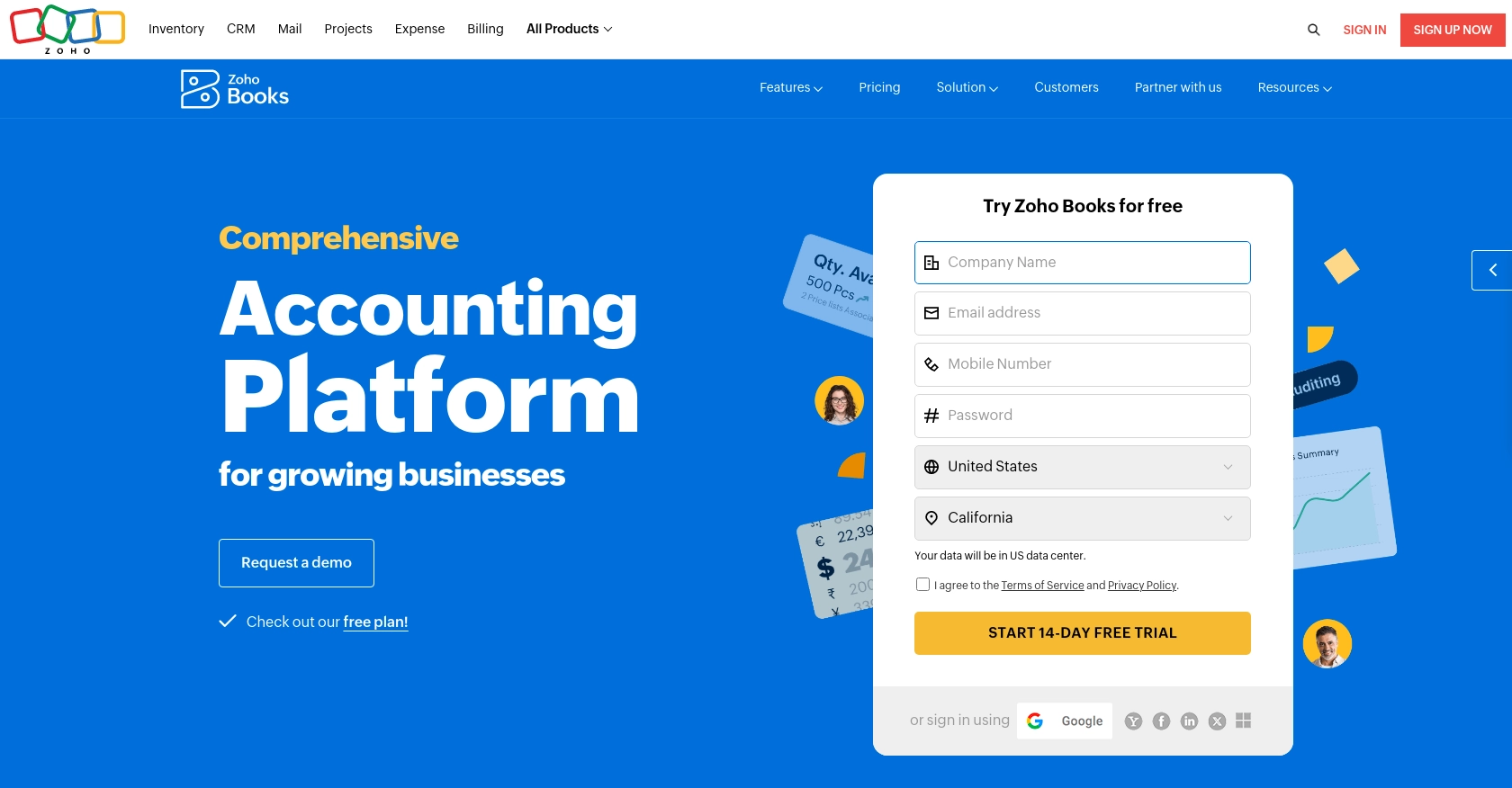
Introduction to Zoho Books API for Purchase Orders
Zoho Books is a comprehensive cloud-based accounting software designed to manage your finances, automate business workflows, and help you work collectively across departments. It offers a wide range of features including invoicing, expense tracking, inventory management, and more, making it a popular choice for businesses of all sizes.
Integrating with the Zoho Books API allows developers to streamline financial operations by automating tasks such as creating and updating purchase orders. For example, a developer might use the Zoho Books API to automatically generate purchase orders from a sales system, ensuring that inventory levels are maintained without manual intervention.
This article will guide you through using Python to interact with the Zoho Books API, focusing on creating and updating purchase orders. By the end of this tutorial, you'll be able to efficiently manage purchase orders within Zoho Books using Python, enhancing your business's operational efficiency.
Setting Up Your Zoho Books Test/Sandbox Account for API Integration
Before you can start creating or updating purchase orders using the Zoho Books API, you'll need to set up a test or sandbox account. This allows you to safely test your API interactions without affecting your live data.
Creating a Zoho Books Account
If you don't already have a Zoho Books account, you can sign up for a free trial on the Zoho Books website. Follow the on-screen instructions to create your account. Once your account is set up, you'll be able to access the Zoho Books dashboard.
Setting Up OAuth for Zoho Books API Access
The Zoho Books API uses OAuth 2.0 for authentication. Follow these steps to create an app and obtain the necessary credentials:
- Go to the Zoho Developer Console and click on "Add Client ID".
- Fill in the required details to register your application. Upon successful registration, you'll receive a Client ID and Client Secret.
- Keep these credentials secure, as they are essential for authenticating your API requests.
Generating OAuth Tokens
To interact with the Zoho Books API, you'll need to generate access and refresh tokens:
- Redirect users to the following authorization URL, replacing the placeholders with your details:
- After user consent, Zoho will redirect to your specified
redirect_uri
with acode
parameter. - Exchange this code for access and refresh tokens by making a POST request to:
- Store the access_token and refresh_token securely for future API calls.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.purchaseorders.CREATE,ZohoBooks.purchaseorders.UPDATE&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI&access_type=offline
https://accounts.zoho.com/oauth/v2/token?code=YOUR_CODE&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
For more detailed information, refer to the Zoho Books OAuth documentation.
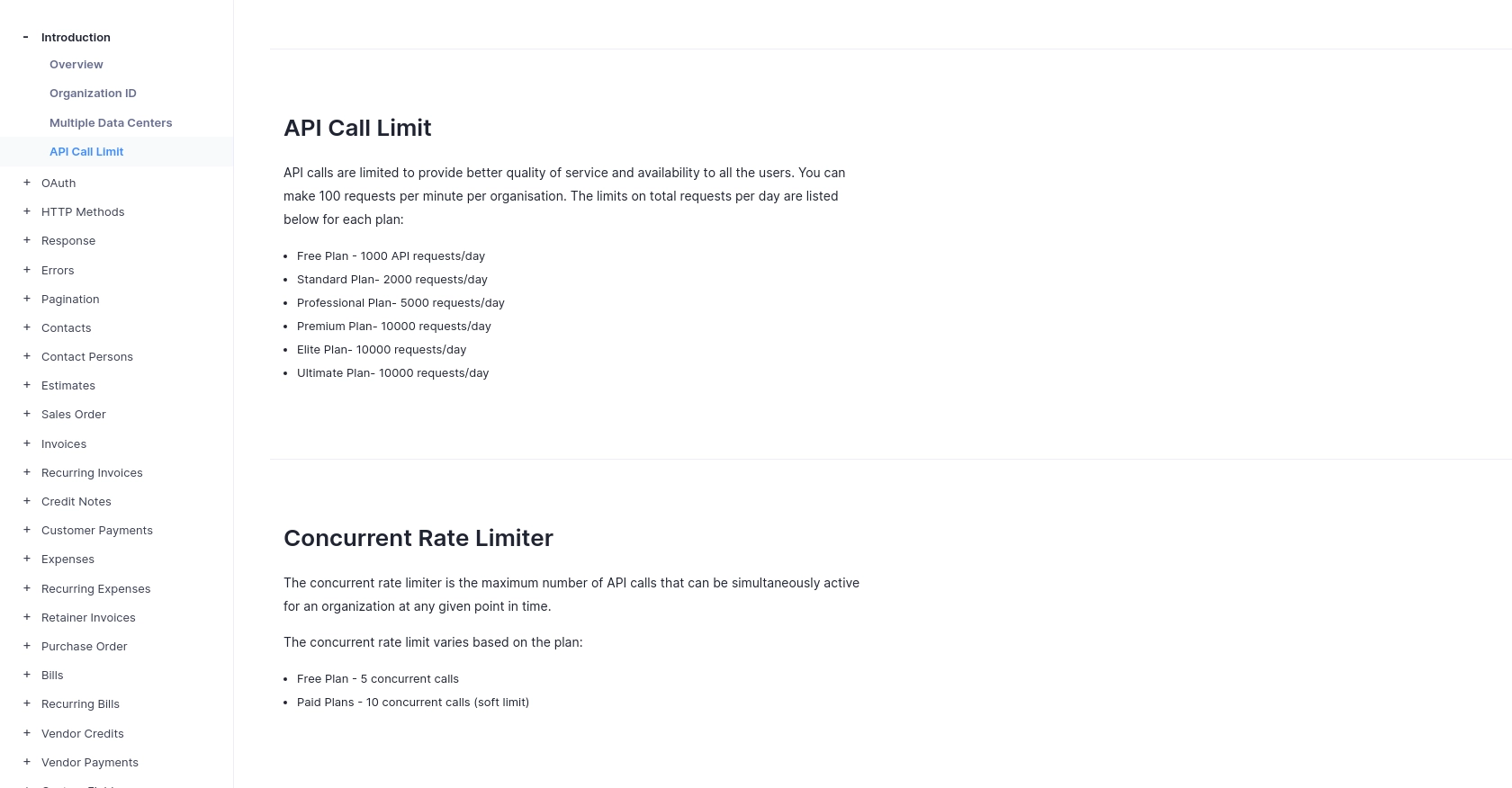
sbb-itb-96038d7
Making API Calls to Zoho Books for Purchase Orders Using Python
To interact with the Zoho Books API for creating or updating purchase orders, you'll need to set up your Python environment and make the necessary API calls. This section will guide you through the process, including setting up your Python environment, writing the code to make API requests, and handling responses.
Setting Up Your Python Environment for Zoho Books API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using the following command:
pip install requests
Creating a Purchase Order with Zoho Books API in Python
To create a purchase order, you'll need to make a POST request to the Zoho Books API endpoint. Here's a step-by-step guide:
- Create a new Python file named
create_purchase_order.py
. - Add the following code to the file:
import requests
# Set the API endpoint and headers
url = "https://www.zohoapis.com/books/v3/purchaseorders?organization_id=YOUR_ORG_ID"
headers = {
"Authorization": "Zoho-oauthtoken YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
# Define the purchase order data
purchase_order_data = {
"vendor_id": "460000000026049",
"currency_id": "460000000000099",
"line_items": [
{
"item_id": "460000000027009",
"rate": 112,
"quantity": 1
}
],
"purchaseorder_number": "PO-00001",
"date": "2023-10-01"
}
# Make the POST request
response = requests.post(url, json=purchase_order_data, headers=headers)
# Check the response
if response.status_code == 201:
print("Purchase Order Created Successfully")
else:
print(f"Failed to Create Purchase Order: {response.json()}")
Replace YOUR_ORG_ID
and YOUR_ACCESS_TOKEN
with your actual organization ID and access token. The code above sends a POST request to create a purchase order with specified details.
Updating a Purchase Order with Zoho Books API in Python
To update an existing purchase order, use a PUT request. Follow these steps:
- Create a new Python file named
update_purchase_order.py
. - Add the following code to the file:
import requests
# Set the API endpoint and headers
purchase_order_id = "460000000062001"
url = f"https://www.zohoapis.com/books/v3/purchaseorders/{purchase_order_id}?organization_id=YOUR_ORG_ID"
headers = {
"Authorization": "Zoho-oauthtoken YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
# Define the updated purchase order data
updated_data = {
"line_items": [
{
"line_item_id": "460000000074009",
"quantity": 2
}
]
}
# Make the PUT request
response = requests.put(url, json=updated_data, headers=headers)
# Check the response
if response.status_code == 200:
print("Purchase Order Updated Successfully")
else:
print(f"Failed to Update Purchase Order: {response.json()}")
Replace YOUR_ORG_ID
, YOUR_ACCESS_TOKEN
, and purchase_order_id
with your actual organization ID, access token, and the ID of the purchase order you wish to update. The code updates the quantity of a line item in the specified purchase order.
Handling API Responses and Errors
When making API calls, it's crucial to handle responses and potential errors effectively. Here are some common HTTP status codes you might encounter:
- 200 OK: The request was successful.
- 201 Created: The resource was successfully created.
- 400 Bad Request: The request was invalid. Check the request parameters.
- 401 Unauthorized: Authentication failed. Verify your access token.
- 429 Rate Limit Exceeded: Too many requests. Respect the rate limits.
For more information on error codes, refer to the Zoho Books Error Documentation.
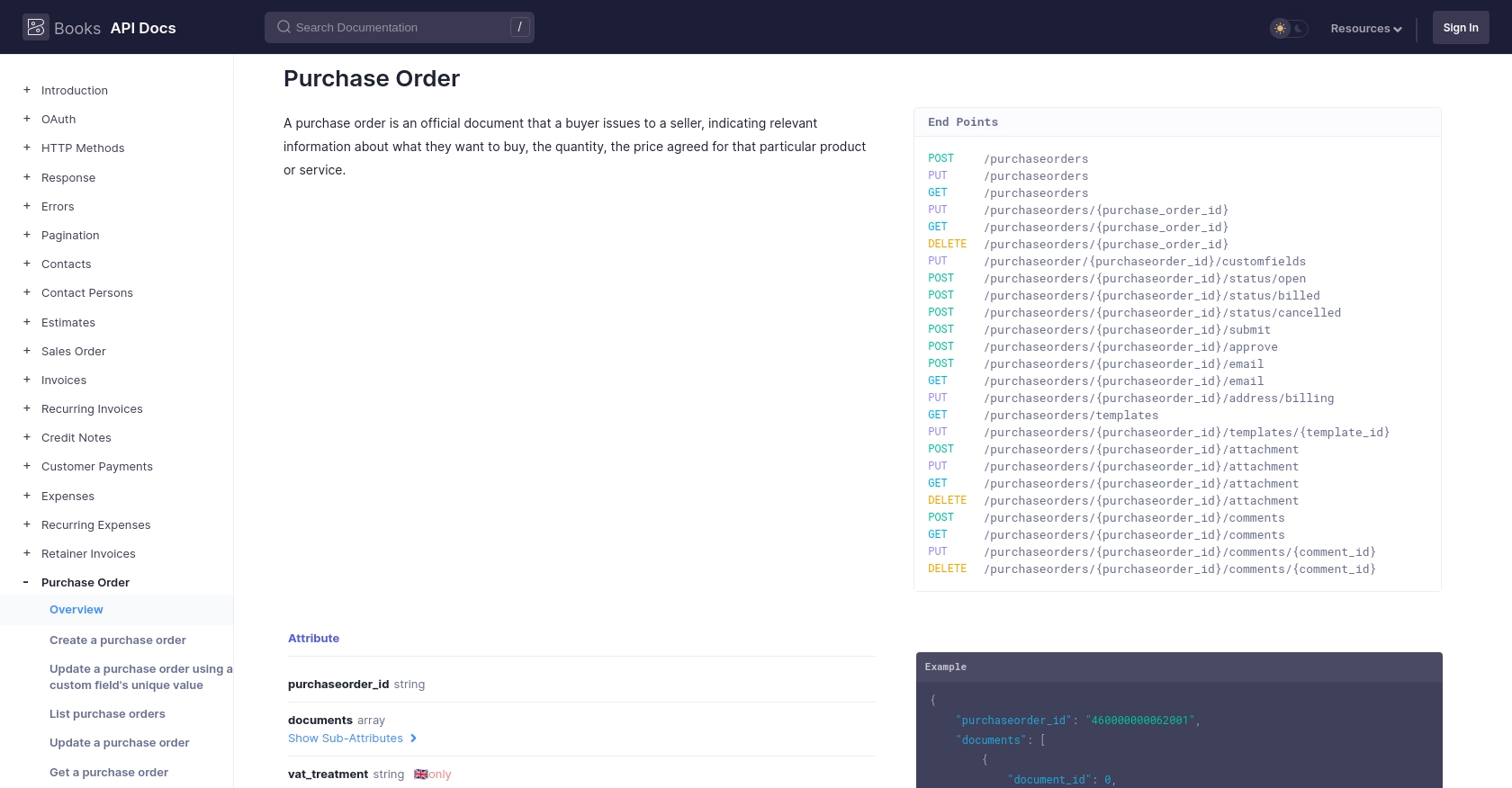
Best Practices for Zoho Books API Integration
When integrating with the Zoho Books API, it's essential to follow best practices to ensure security, efficiency, and reliability. Here are some key recommendations:
- Securely Store Credentials: Always store your OAuth tokens and client credentials securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Zoho Books imposes rate limits on API requests. The limit is 100 requests per minute per organization. Implement logic to handle rate limit responses gracefully and retry requests after a delay. For more details, refer to the API Call Limit Documentation.
- Standardize Data Fields: Ensure that data fields are consistent and standardized across your application and Zoho Books. This will help in maintaining data integrity and ease of integration.
- Implement Error Handling: Use robust error handling to manage API responses and errors. Log errors for debugging and provide meaningful messages to users when issues occur.
Enhancing Integration Efficiency with Endgrate
Building and maintaining integrations can be time-consuming and complex. Endgrate simplifies this process by offering a unified API endpoint that connects to multiple platforms, including Zoho Books. With Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the integration complexities.
- Build Once, Use Everywhere: Develop a single integration for each use case and apply it across different platforms.
- Provide a Seamless Experience: Offer your customers an intuitive and efficient integration experience.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/purchase-order/#overview
Ready to get started?