How to Get Products with the Stamped API in PHP
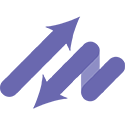
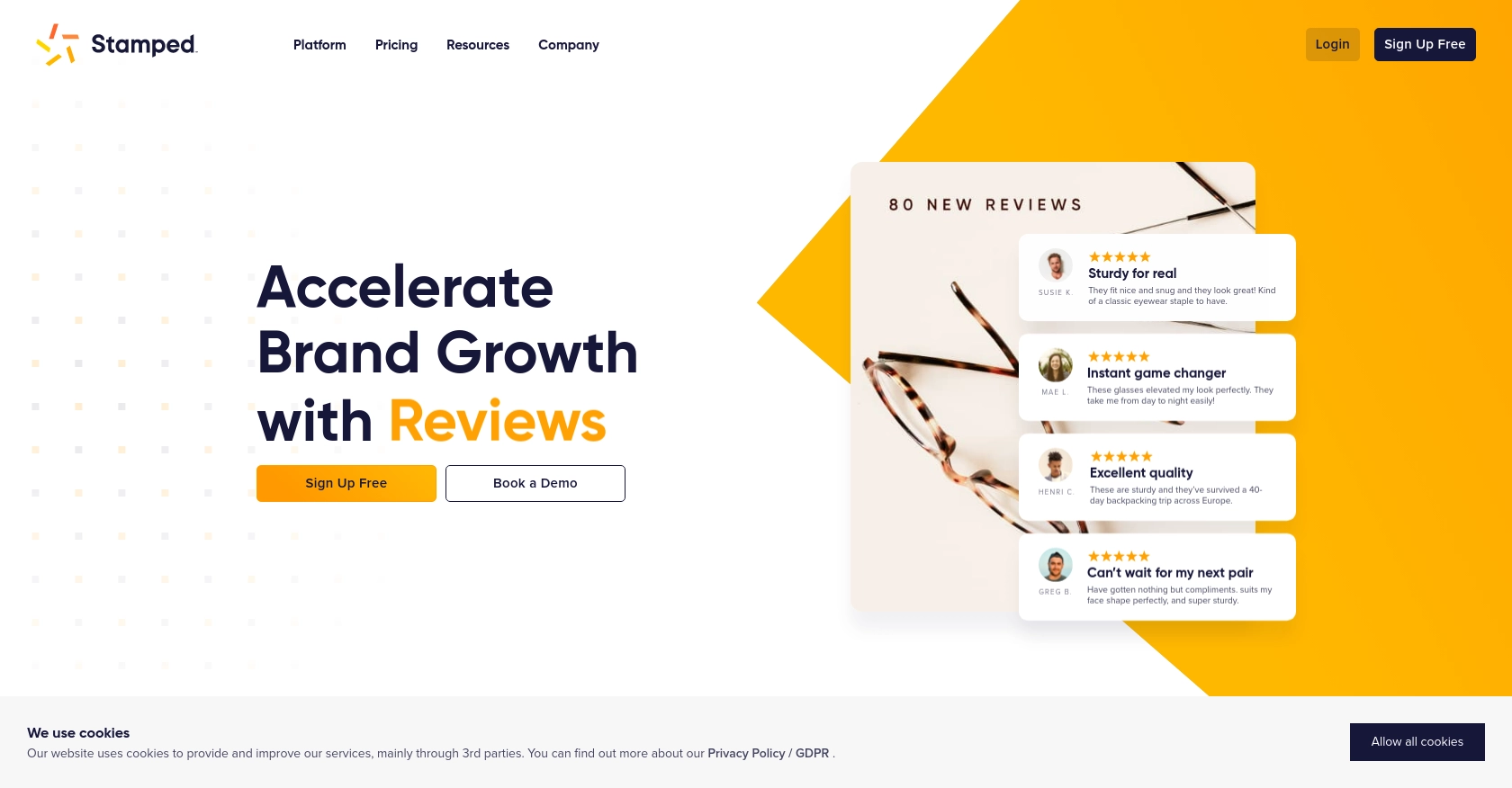
Introduction to Stamped API
Stamped is a powerful eCommerce marketing platform that enables businesses to enhance customer engagement through reviews, loyalty programs, and user-generated content. With over 75,000 brands leveraging its capabilities, Stamped provides a comprehensive suite of tools to drive growth and improve customer retention.
Developers may want to integrate with the Stamped API to access and manage product data efficiently. For example, using the Stamped API, a developer can retrieve detailed product information to synchronize inventory across multiple platforms, ensuring consistency and accuracy in product listings.
Setting Up Your Stamped API Account for Product Retrieval
Before you can start interacting with the Stamped API to retrieve product data, you need to set up your account and obtain the necessary authentication credentials. Stamped requires a Professional or Enterprise plan to access its API features, so ensure you have the appropriate subscription level.
Creating a Stamped Account and Accessing the API Keys
If you don't already have a Stamped account, sign up on the Stamped website. Once your account is active, follow these steps to access your API keys:
- Log in to your Stamped account and navigate to the API Keys section in the Control Panel.
- Here, you will find your public and private API keys. These keys are essential for authenticating your API requests.
- Keep these keys secure, as they grant access to your Stamped account's data.
Understanding Stamped API Authentication
Stamped uses HTTP Basic Auth for authenticating requests to private endpoints. Here's how you can set up authentication:
- Use your public API key as the username.
- Use your private API key as the password.
For public endpoints, you may only need the store hash or public key, depending on the specific API call.
Refer to the Stamped REST API documentation for more details on authentication.
Preparing Your PHP Environment
To interact with the Stamped API using PHP, ensure your development environment is set up correctly:
- Install PHP 7.4 or higher on your machine.
- Ensure you have access to a tool like Composer for managing PHP dependencies.
With your Stamped account and PHP environment ready, you're all set to start making API calls to retrieve product information.
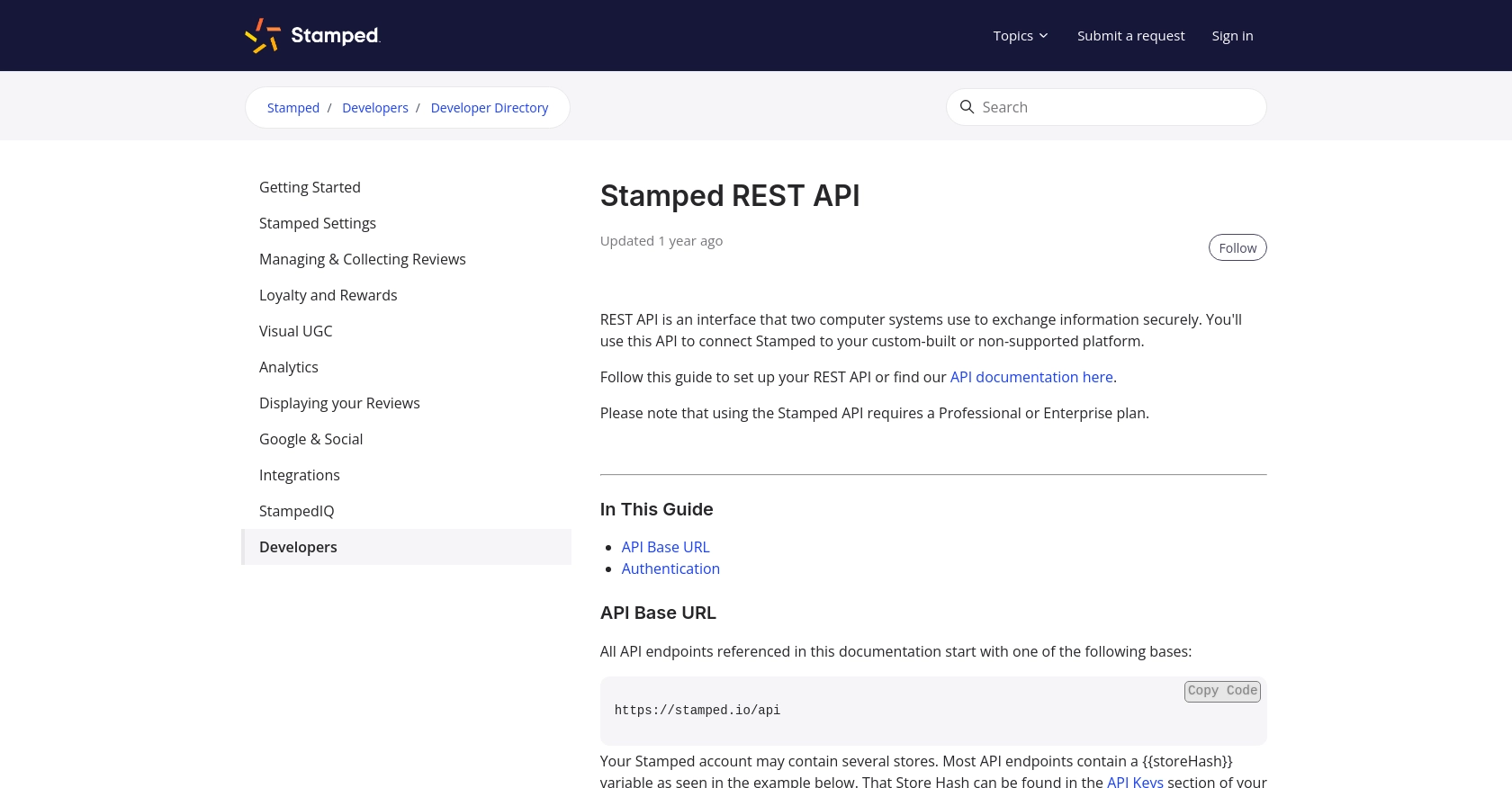
sbb-itb-96038d7
Making API Calls to Retrieve Products with Stamped API in PHP
Setting Up Your PHP Environment for Stamped API Integration
Before making API calls to the Stamped API, ensure your PHP environment is properly configured. You'll need PHP 7.4 or higher and Composer for managing dependencies. These tools will help you interact with the Stamped API seamlessly.
Installing Required PHP Dependencies
To make HTTP requests in PHP, you'll need the Guzzle HTTP client. Install it using Composer with the following command:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Product Data from Stamped API
With your environment set up, you can now write PHP code to interact with the Stamped API. Below is a sample script to retrieve product information:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$storeHash = 'your_store_hash';
$productId = 'your_product_id';
$publicKey = 'your_public_api_key';
$privateKey = 'your_private_api_key';
$response = $client->request('GET', "https://stamped.io/api/v3/merchant/shops/{$storeHash}/products/{$productId}", [
'auth' => [$publicKey, $privateKey]
]);
if ($response->getStatusCode() === 200) {
$productData = json_decode($response->getBody(), true);
echo 'Product Name: ' . $productData['name'] . "\n";
echo 'Product Description: ' . $productData['description'] . "\n";
} else {
echo 'Failed to retrieve product data. Status code: ' . $response->getStatusCode();
}
Replace your_store_hash
, your_product_id
, your_public_api_key
, and your_private_api_key
with your actual Stamped account details.
Understanding the API Response and Error Handling
After executing the script, you should see the product details printed in the console. If the request fails, the script will output the status code, helping you diagnose the issue.
For more information on error codes, refer to the Stamped API documentation.
Verifying API Call Success in Stamped Dashboard
To ensure the API call was successful, log in to your Stamped dashboard and verify the product details match the retrieved data. This step confirms that your integration is working correctly.
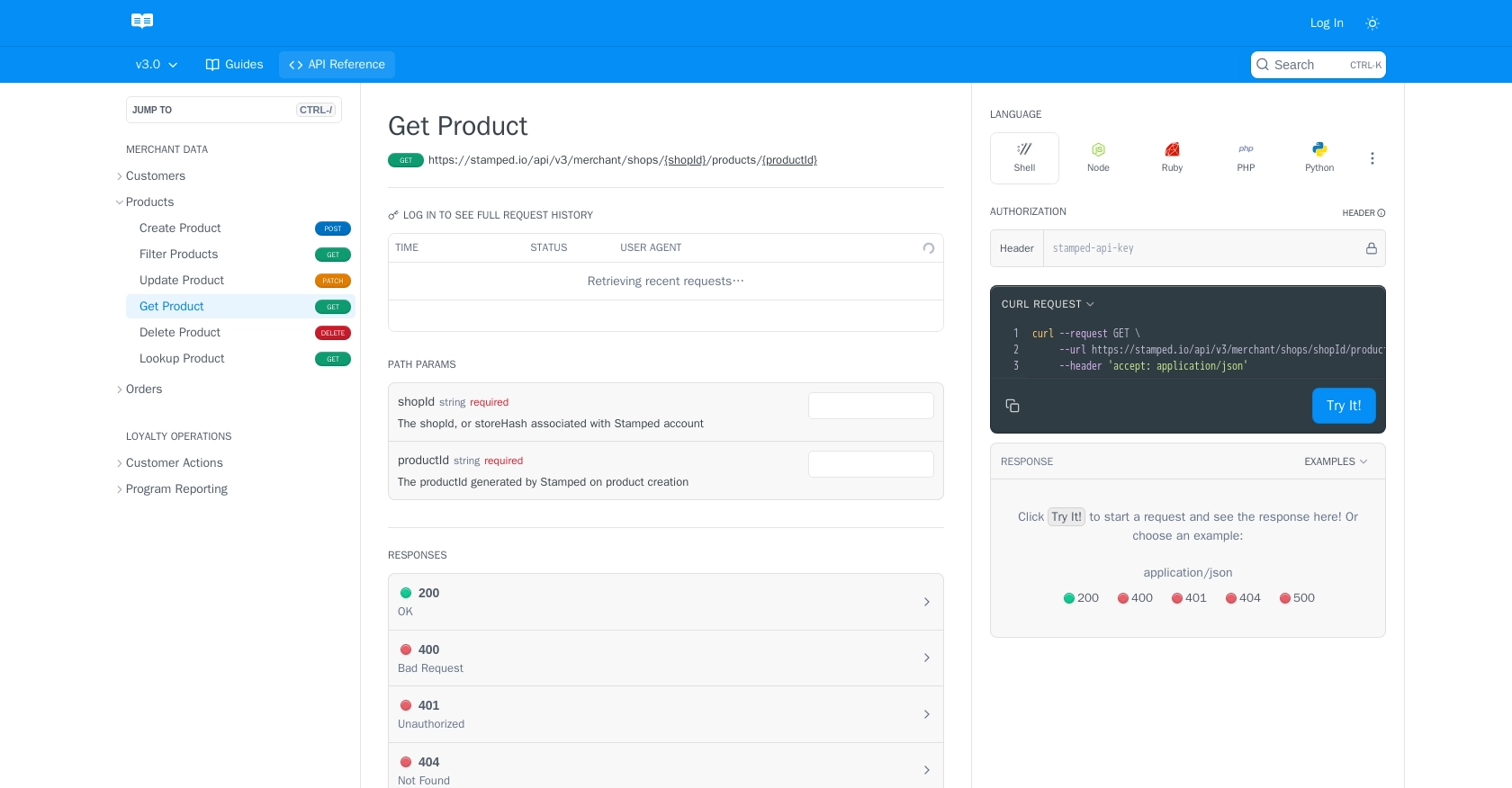
Best Practices for Using the Stamped API in PHP
When working with the Stamped API, it's essential to follow best practices to ensure secure and efficient integration. Here are some recommendations:
- Secure Storage of API Credentials: Always store your public and private API keys securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handling Rate Limits: Be aware of any rate limits imposed by the Stamped API to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage different HTTP status codes and API-specific errors. This will help in diagnosing issues quickly.
Streamlining Your Integration Process with Endgrate
Integrating with multiple platforms can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various services, including Stamped.
By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers, enhancing their satisfaction and engagement.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate today.
Read More
Ready to get started?