Using the MongoDB API to Create or Update Records (with Javascript examples)
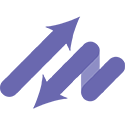
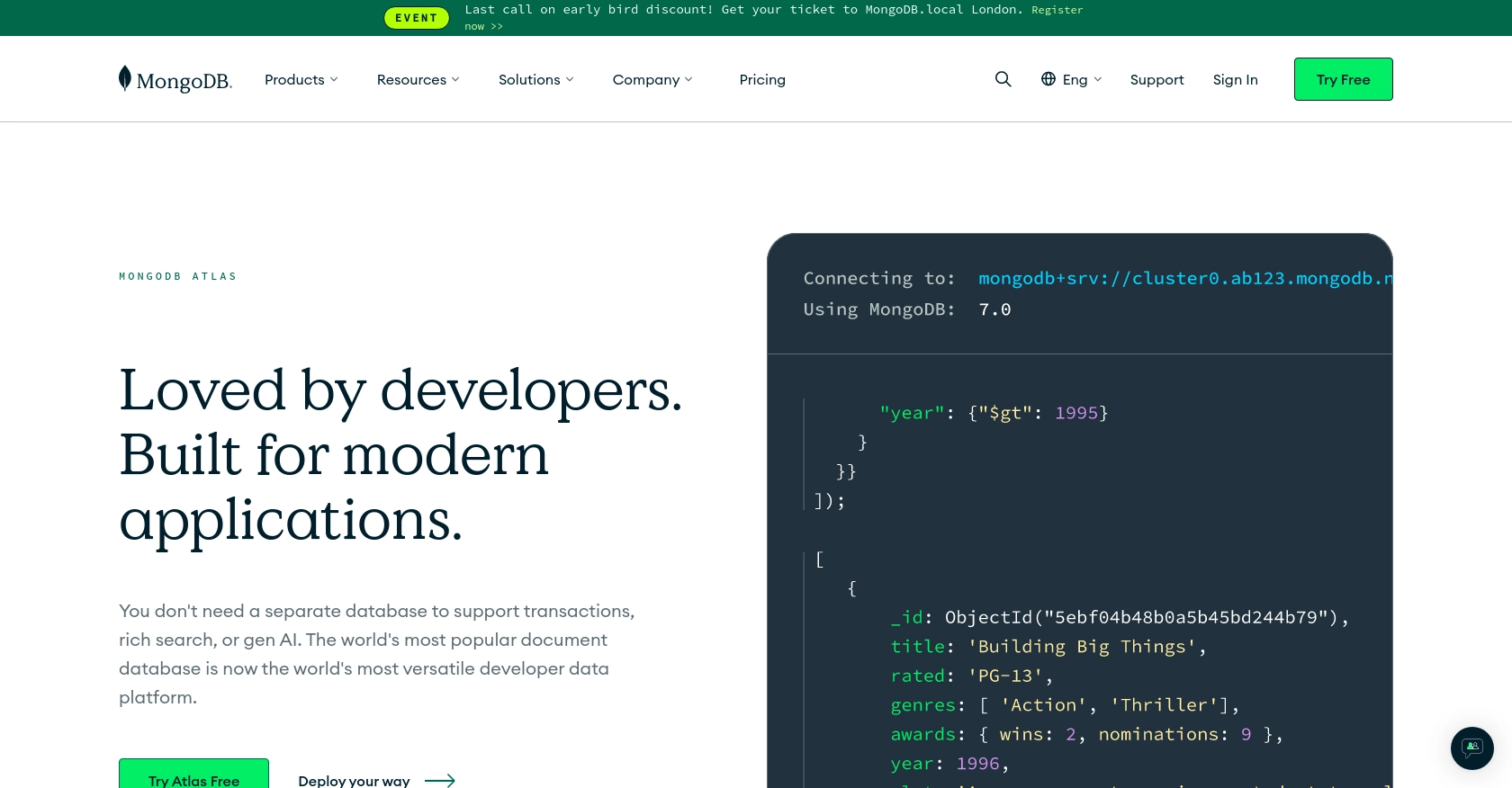
Introduction to MongoDB API Integration
MongoDB is a powerful, flexible, and scalable NoSQL database that allows developers to store and manage data in a document-oriented format. Known for its high performance and ability to handle large volumes of data, MongoDB is a popular choice for modern applications that require dynamic and complex data structures.
Integrating with the MongoDB API enables developers to perform CRUD (Create, Read, Update, Delete) operations programmatically, allowing for seamless data management and automation. For example, a developer might use the MongoDB API to create or update records in a database, facilitating real-time data synchronization between applications.
This article will guide you through using JavaScript to interact with the MongoDB API, focusing on creating and updating records. By the end of this tutorial, you'll have a solid understanding of how to leverage MongoDB's capabilities to enhance your application's data handling processes.
Setting Up Your MongoDB Test/Sandbox Account
Before you can start interacting with the MongoDB API using JavaScript, you need to set up a test or sandbox environment. This will allow you to safely experiment with API calls without affecting any production data.
Creating a MongoDB Atlas Account
MongoDB Atlas is a fully managed cloud database service that provides a free tier for developers to get started. Follow these steps to create your MongoDB Atlas account:
- Visit the MongoDB Atlas website and click on "Try Free".
- Sign up using your email address or an existing Google account.
- Once registered, log in to your MongoDB Atlas account.
Setting Up a Free Tier Cluster
After logging in, you'll need to set up a cluster to host your database:
- Click on "Build a Cluster" and select the free tier option.
- Choose your preferred cloud provider and region.
- Click "Create Cluster" to initiate the setup process. This may take a few minutes.
Configuring Database Access and Network Security
To interact with your MongoDB database, you'll need to configure access and security settings:
- Navigate to the "Database Access" section and create a new database user. Assign a username and password, and ensure the user has read and write permissions.
- Go to the "Network Access" section and add your IP address to the IP Whitelist. This allows your local machine to connect to the database.
Obtaining Connection String
With your cluster set up, you need the connection string to connect your application:
- In the "Clusters" view, click "Connect" next to your cluster.
- Select "Connect Your Application" and copy the provided connection string.
- Replace the placeholder username and password in the connection string with your database user's credentials.
With your MongoDB Atlas account and cluster configured, you're ready to start making API calls using JavaScript. This setup provides a secure and isolated environment to test your integration with MongoDB.
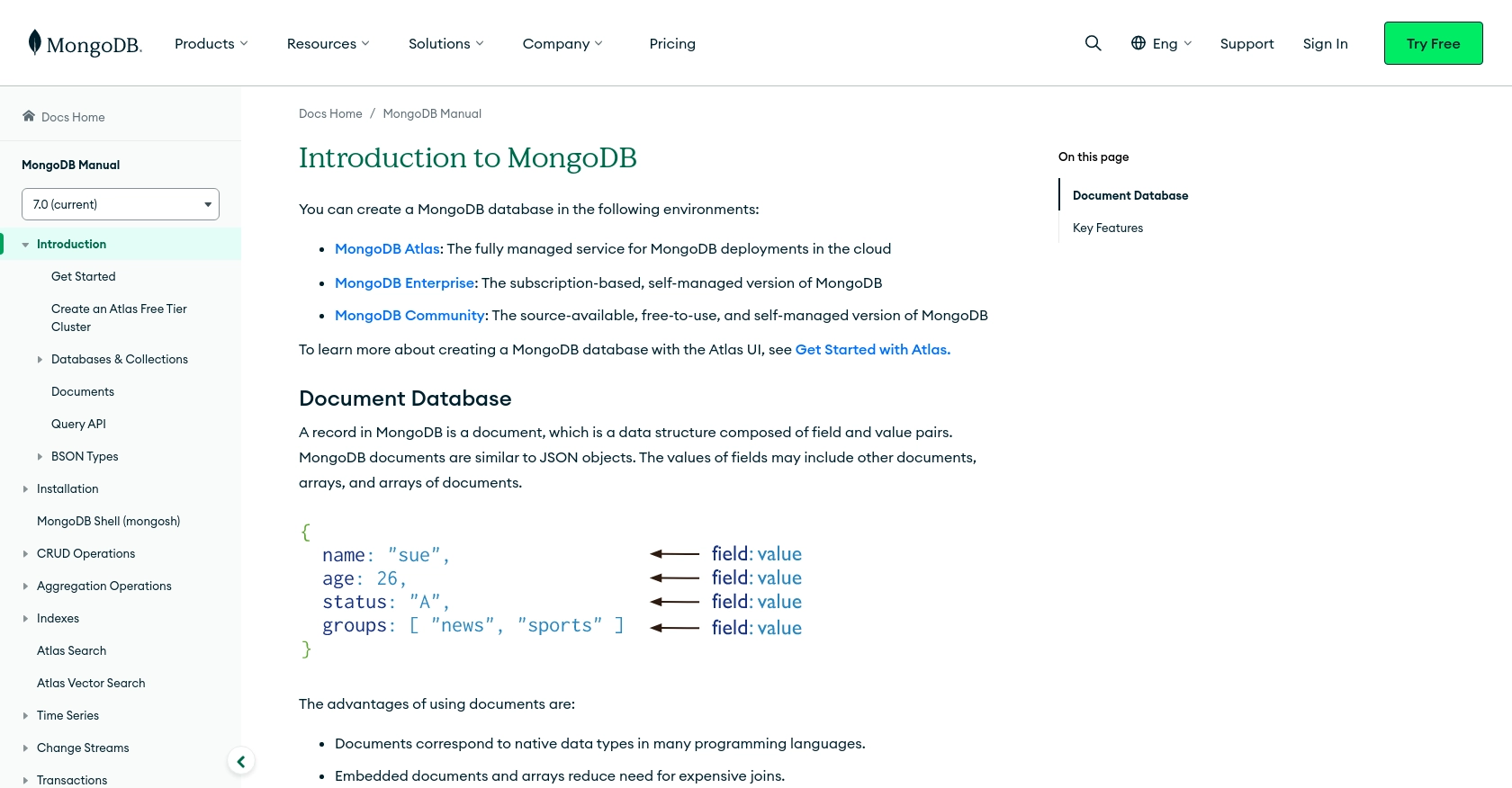
sbb-itb-96038d7
Making API Calls to MongoDB with JavaScript
To interact with MongoDB using JavaScript, you'll need to set up your environment and write code to perform API calls. This section will guide you through the process of creating and updating records in MongoDB using JavaScript.
Setting Up Your JavaScript Environment for MongoDB API
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides the runtime environment for executing JavaScript code outside a web browser.
- Download and install Node.js from the official website.
- Verify the installation by running
node -v
andnpm -v
in your terminal.
Next, you'll need to install the MongoDB Node.js driver, which allows your application to connect to MongoDB:
npm install mongodb
Connecting to MongoDB Using JavaScript
With the MongoDB driver installed, you can now connect to your MongoDB Atlas cluster using the connection string obtained earlier.
const { MongoClient } = require('mongodb');
const uri = "your_connection_string_here";
const client = new MongoClient(uri, { useNewUrlParser: true, useUnifiedTopology: true });
async function connectToMongoDB() {
try {
await client.connect();
console.log("Connected to MongoDB");
} catch (error) {
console.error("Error connecting to MongoDB:", error);
}
}
connectToMongoDB();
Replace your_connection_string_here
with your actual connection string, including your username and password.
Creating Records in MongoDB with JavaScript
Once connected, you can create new records in your MongoDB database. The following example demonstrates how to insert a document into a collection:
async function createRecord() {
try {
const database = client.db('your_database_name');
const collection = database.collection('your_collection_name');
const newRecord = {
name: "John Doe",
email: "john.doe@example.com",
age: 30
};
const result = await collection.insertOne(newRecord);
console.log(`New record created with ID: ${result.insertedId}`);
} catch (error) {
console.error("Error creating record:", error);
}
}
createRecord();
Replace your_database_name
and your_collection_name
with your actual database and collection names.
Updating Records in MongoDB with JavaScript
To update existing records, use the updateOne
or updateMany
methods. Here's an example of updating a record:
async function updateRecord() {
try {
const database = client.db('your_database_name');
const collection = database.collection('your_collection_name');
const filter = { email: "john.doe@example.com" };
const updateDoc = {
$set: {
age: 31
}
};
const result = await collection.updateOne(filter, updateDoc);
console.log(`${result.matchedCount} document(s) matched the filter, updated ${result.modifiedCount} document(s)`);
} catch (error) {
console.error("Error updating record:", error);
}
}
updateRecord();
This code updates the age of the record with the specified email address.
Verifying API Call Success and Handling Errors
After executing API calls, verify the success by checking the console output or querying the database directly. Handle errors gracefully by catching exceptions and logging them for further analysis.
For more detailed information on MongoDB API interactions, refer to the MongoDB documentation.
Conclusion and Best Practices for MongoDB API Integration with JavaScript
Integrating with the MongoDB API using JavaScript provides a robust solution for managing and manipulating data within your applications. By following the steps outlined in this article, you can efficiently create and update records in MongoDB, leveraging its powerful features to enhance your data handling capabilities.
Here are some best practices to consider when working with MongoDB API integrations:
- Securely Store Credentials: Ensure that your MongoDB connection strings and credentials are stored securely, using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by MongoDB Atlas and implement retry logic or exponential backoff strategies to handle API call limits gracefully.
- Data Validation and Sanitization: Always validate and sanitize data before inserting or updating records to maintain data integrity and prevent injection attacks.
- Monitor and Log API Calls: Implement logging and monitoring to track API call performance and errors, enabling quick identification and resolution of issues.
- Optimize Queries: Use indexes and optimize your queries to improve performance, especially when dealing with large datasets.
By adhering to these best practices, you can ensure a secure, efficient, and scalable integration with MongoDB.
For developers looking to streamline their integration processes further, consider using Endgrate. Endgrate offers a unified API endpoint that simplifies connecting to multiple platforms, allowing you to focus on building your core product while outsourcing the complexities of integration management. Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
Ready to get started?