How to Create Pin with the igloohome API in Python
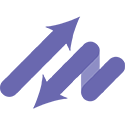
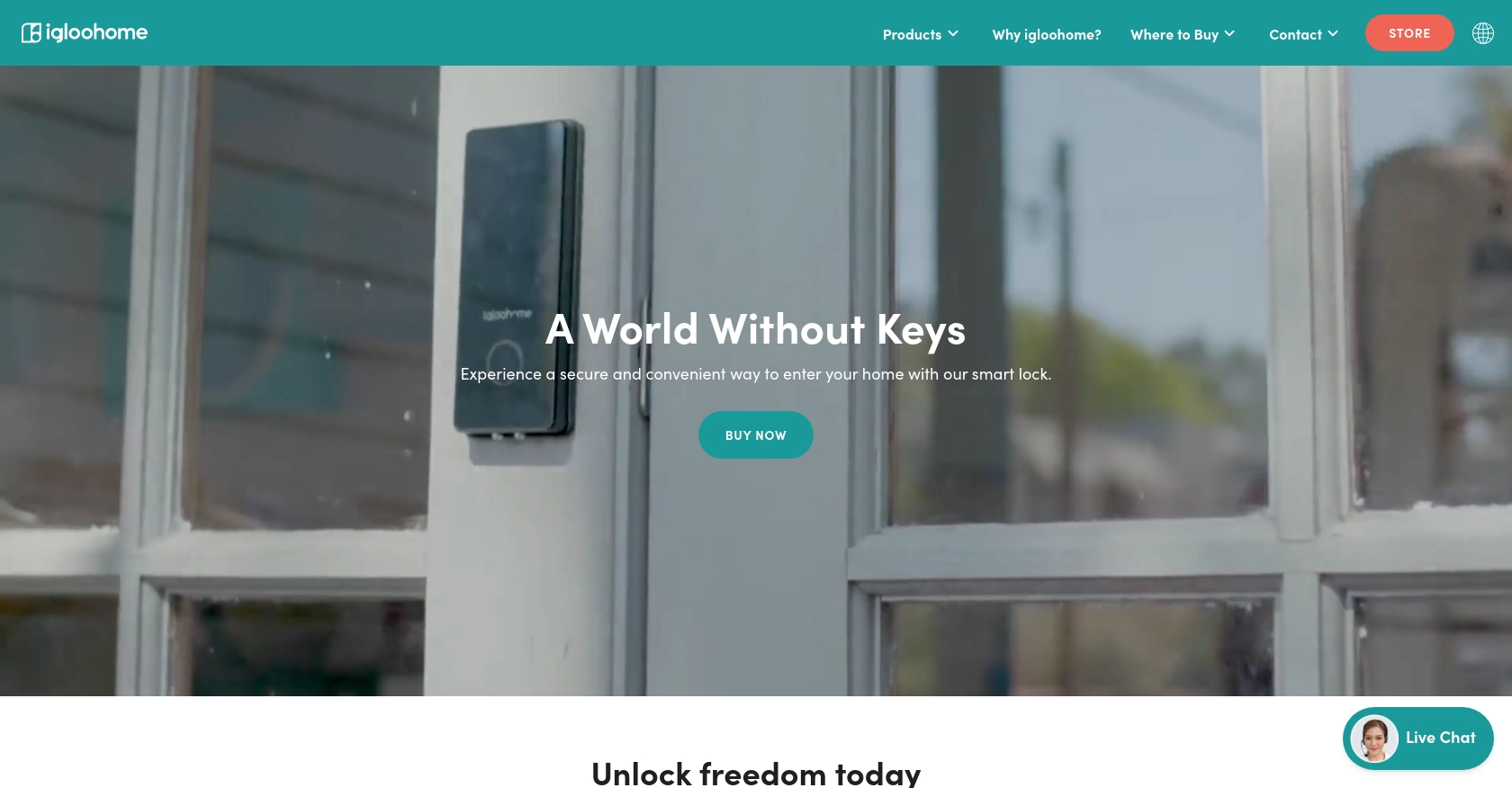
Introduction to igloohome API
igloohome is a leading provider of smart access solutions, offering a range of products that allow users to manage access to their properties remotely. With its innovative technology, igloohome provides secure and convenient access management for homes, offices, and other spaces.
Developers may want to integrate with the igloohome API to automate and streamline access control processes. For example, using the igloohome API, a developer can create a one-time PIN code for a smart lock, enabling temporary access for guests or service providers without the need for physical keys.
Setting Up Your igloohome Test/Sandbox Account
Before you can start creating PIN codes with the igloohome API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting any live data.
Registering for an igloohome API Trial
To begin, sign up for a 30-day trial of the igloohome API by visiting the following link: igloohome API Trial Registration. This trial provides access to the API's full functionality, allowing you to test and integrate with igloohome's smart access solutions.
Creating an igloohome App for OAuth Authentication
Since the igloohome API uses OAuth for authentication, you'll need to create an app within your sandbox account to obtain the necessary credentials. Follow these steps to set up your app:
- Log in to your igloohome account after registration.
- Navigate to the developer section and select "Create New App."
- Fill in the required details for your app, such as the app name and description.
- Once your app is created, you'll receive a Client ID and Client Secret. Keep these credentials secure, as they will be used to authenticate your API requests.
Configuring OAuth Scopes for igloohome API Access
To ensure your app has the correct permissions, configure the OAuth scopes as follows:
- Access to create and manage PIN codes.
- Read and write access to lock settings and status.
These scopes will allow your app to interact with the igloohome API effectively, enabling you to create and manage PIN codes for smart locks.
With your sandbox account and app set up, you're now ready to start integrating with the igloohome API using Python. In the next section, we'll walk through the process of making API calls to create a one-time PIN code.
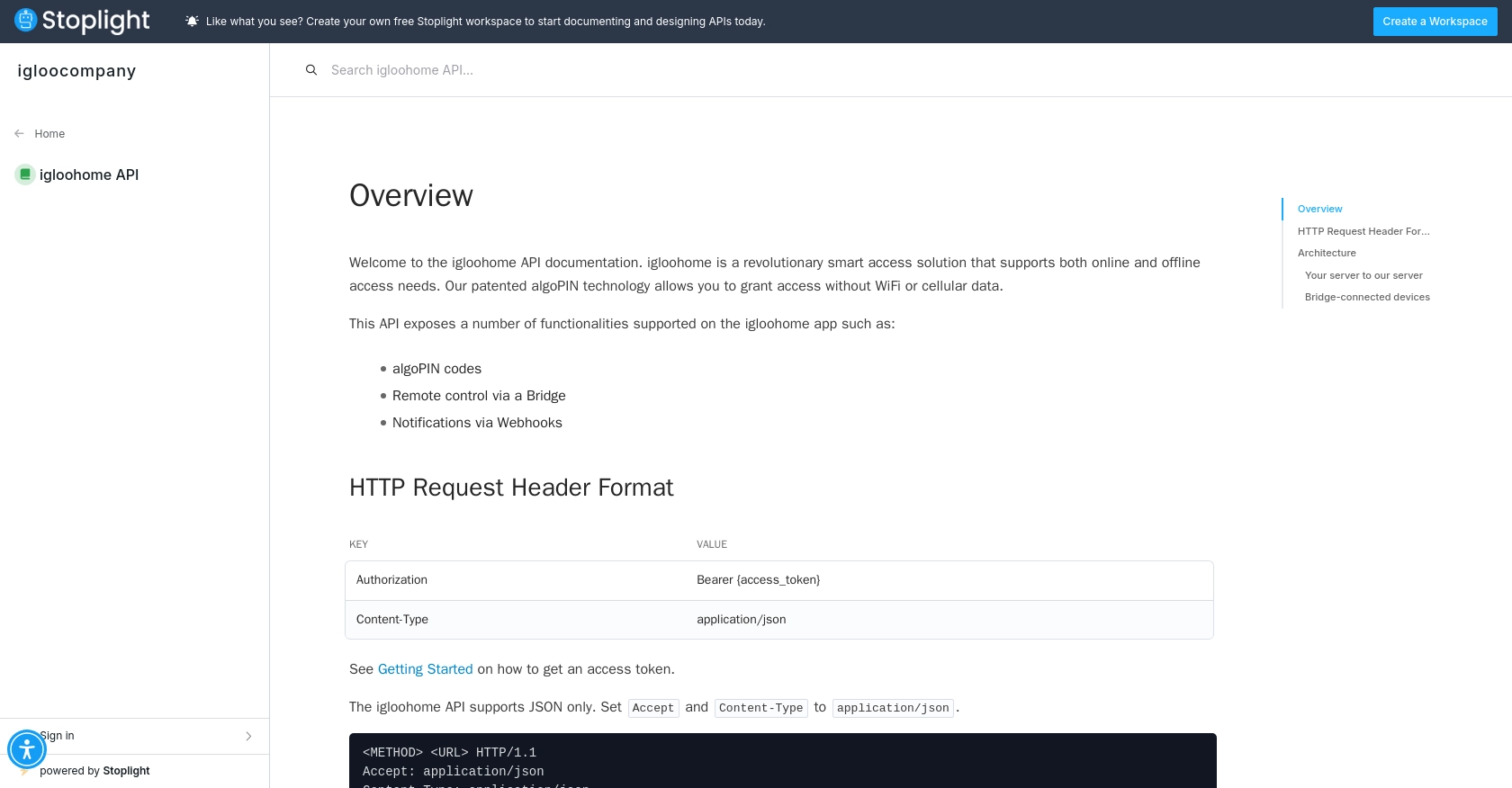
sbb-itb-96038d7
Making API Calls to Create a One-Time PIN Code with igloohome API in Python
To create a one-time PIN code using the igloohome API, you'll need to set up your Python environment and make the appropriate API call. This section will guide you through the necessary steps, including setting up Python, installing dependencies, and executing the API call.
Setting Up Your Python Environment for igloohome API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using the following command:
pip install requests
Creating a One-Time PIN Code with igloohome API
With your environment ready, you can proceed to create a one-time PIN code. The following Python script demonstrates how to make the API call:
import requests
# Define the API endpoint and headers
url = "https://api.igloohome.co/v1/pins"
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Define the payload for the PIN creation
payload = {
"lock_id": "your_lock_id",
"type": "one_time",
"start_time": "2023-10-01T00:00:00Z",
"end_time": "2023-10-01T23:59:59Z"
}
# Make the POST request to create the PIN
response = requests.post(url, json=payload, headers=headers)
# Check the response status and output the result
if response.status_code == 201:
print("PIN Created Successfully:", response.json())
else:
print("Failed to Create PIN:", response.status_code, response.json())
Replace Your_Access_Token
with the access token obtained from your igloohome app setup. Also, replace your_lock_id
with the ID of the lock you are managing.
Verifying the API Request and Handling Errors
After running the script, you should see a successful response with the details of the created PIN. If the request fails, the script will output the error code and message. Common error codes include:
- 400 Bad Request: Check the request payload for any missing or incorrect parameters.
- 401 Unauthorized: Ensure your access token is valid and correctly included in the headers.
- 404 Not Found: Verify the lock ID and endpoint URL.
For more detailed error handling, refer to the igloohome API documentation.
By following these steps, you can efficiently create one-time PIN codes using the igloohome API in Python, streamlining access management for your smart locks.
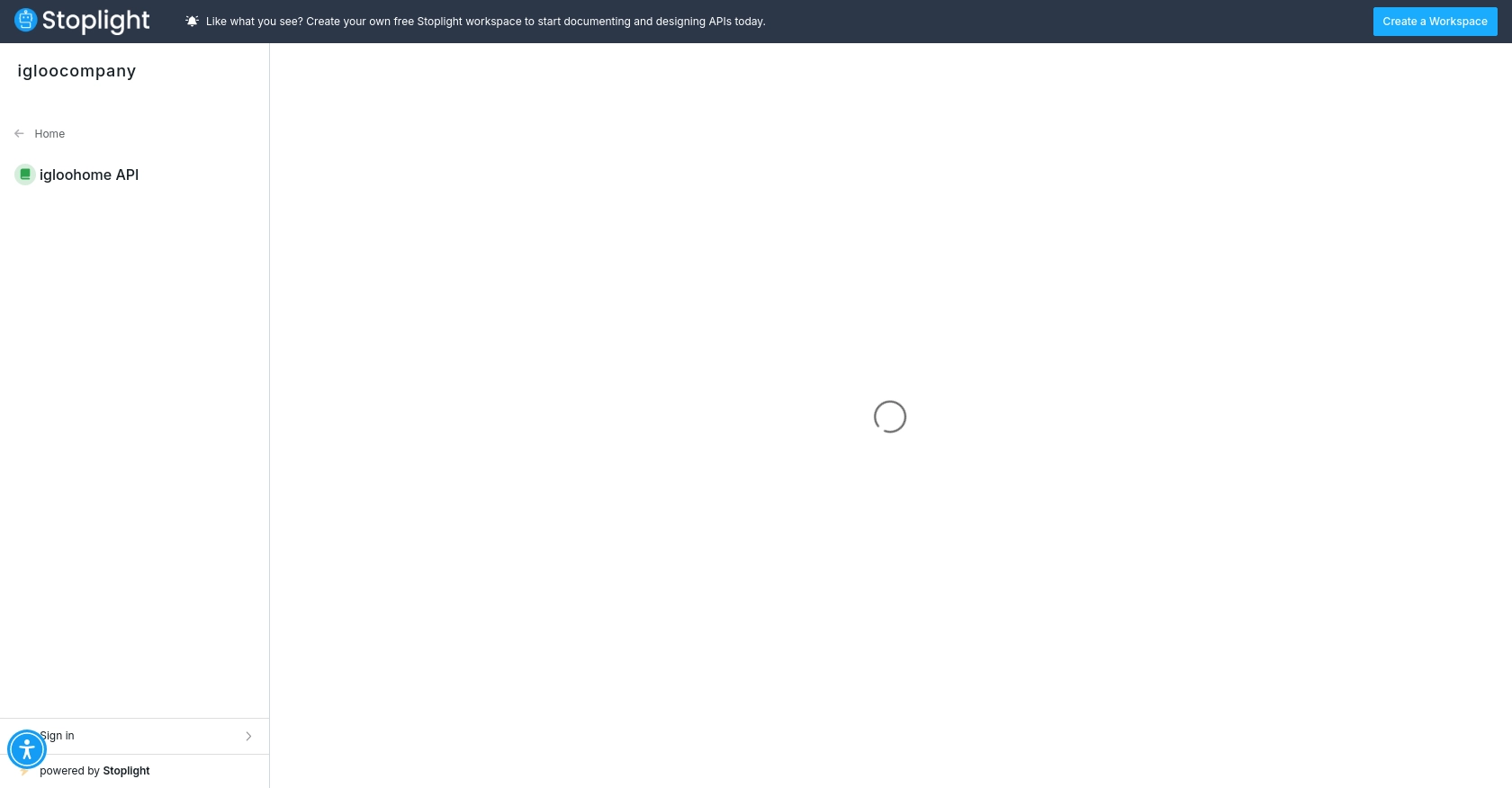
Conclusion and Best Practices for Using igloohome API in Python
Integrating with the igloohome API allows developers to efficiently manage smart access solutions, such as creating one-time PIN codes for locks. By following the steps outlined in this guide, you can seamlessly integrate igloohome's capabilities into your applications, enhancing security and convenience for users.
Best Practices for Secure and Efficient igloohome API Integration
- Secure Storage of Credentials: Always store your Client ID, Client Secret, and access tokens securely. Consider using environment variables or secure vaults to manage sensitive information.
- Handling Rate Limits: Be mindful of the API's rate limits to avoid throttling. Implement exponential backoff strategies to handle rate limit errors gracefully. Refer to the igloohome API documentation for specific rate limit details.
- Data Standardization: Ensure that data fields are standardized and consistent across your application to facilitate smooth integration and data handling.
Streamlining Integrations with Endgrate
For developers looking to simplify and scale their integration efforts, consider using Endgrate. With Endgrate, you can save time and resources by building once for each use case and leveraging a unified API endpoint for multiple platforms, including igloohome. This approach allows you to focus on your core product while providing an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?