How to Get Records with the Salesforce Sandbox API in PHP
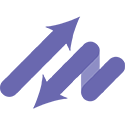
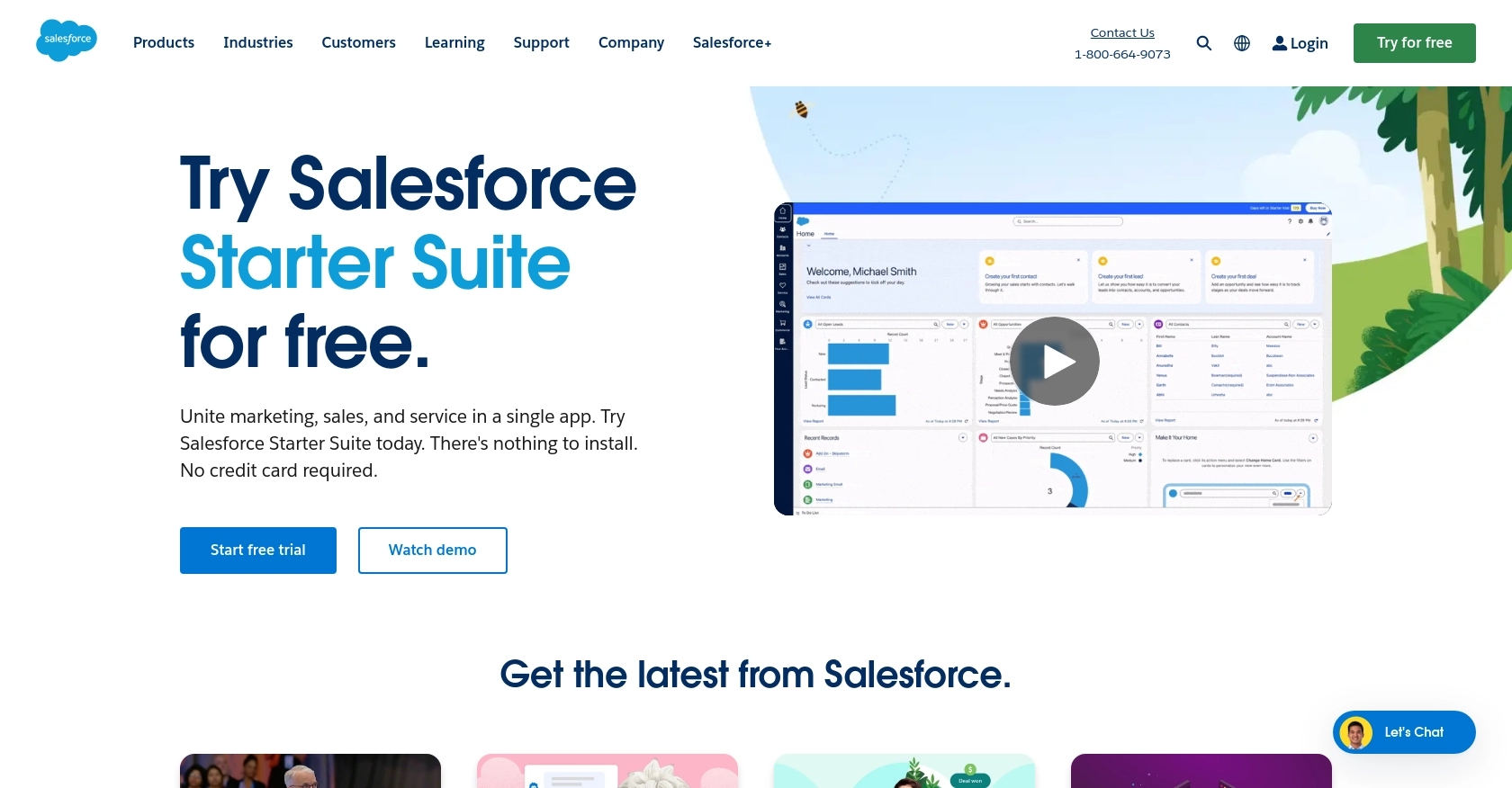
Introduction to Salesforce Sandbox API
Salesforce Sandbox is a powerful tool that allows developers to create and test applications in a secure environment that mirrors their production setup. It provides a safe space to experiment with new features, integrations, and customizations without affecting live data.
Integrating with the Salesforce Sandbox API is essential for developers who want to access and manipulate Salesforce data programmatically. For example, a developer might use the API to retrieve records such as accounts or contacts, enabling seamless data synchronization between Salesforce and other business applications.
This article will guide you through the process of using PHP to interact with the Salesforce Sandbox API, focusing on retrieving records efficiently and securely.
Setting Up Your Salesforce Sandbox Account
Before you can begin interacting with the Salesforce Sandbox API using PHP, you need to set up a Salesforce Sandbox account. This environment allows you to test and develop applications without impacting your live data, providing a secure and isolated space for experimentation.
Creating a Salesforce Sandbox Account
If you don't already have a Salesforce Sandbox account, you'll need to create one. Follow these steps to get started:
- Log in to your Salesforce production account.
- Navigate to the Setup menu by clicking on the gear icon in the top right corner.
- In the Quick Find box, type Sandboxes and select it from the dropdown.
- Click on New Sandbox to create a new sandbox environment.
- Choose the type of sandbox you need (e.g., Developer, Developer Pro) based on your requirements.
- Enter a name and description for your sandbox, then click Create.
Once your sandbox is created, you'll receive an email notification with login details.
Setting Up OAuth Authentication for Salesforce Sandbox
Salesforce uses OAuth 2.0 for authentication, which requires setting up a connected app. Follow these steps to configure OAuth authentication:
- In your Salesforce Sandbox account, navigate to Setup.
- In the Quick Find box, type App Manager and select it.
- Click on New Connected App in the top right corner.
- Fill in the required fields, such as Connected App Name and API Name.
- Under API (Enable OAuth Settings), check the box for Enable OAuth Settings.
- Enter a Callback URL. This is the URL where Salesforce will send the authorization code after a successful login.
- Select the appropriate OAuth Scopes that your application requires, such as Access and manage your data (api).
- Click Save to create the connected app.
After saving, you'll receive a Consumer Key and Consumer Secret. These credentials are essential for authenticating API requests.
For more detailed information on setting up a Salesforce Sandbox and OAuth authentication, refer to the official documentation: Salesforce Sandbox Setup and OAuth and Connected Apps.
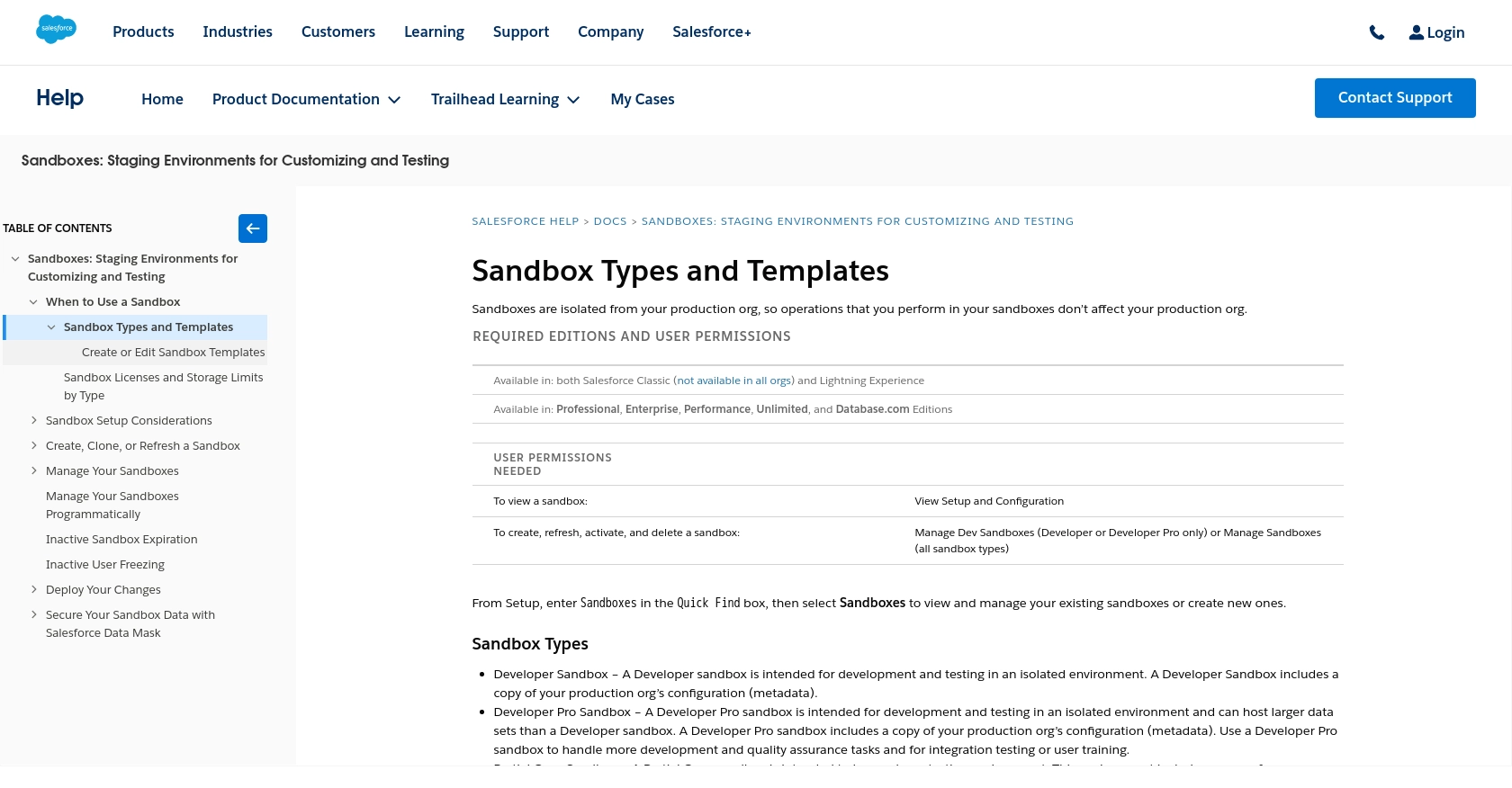
sbb-itb-96038d7
Making API Calls to Retrieve Records from Salesforce Sandbox Using PHP
Once you have set up your Salesforce Sandbox account and configured OAuth authentication, you can proceed to make API calls to retrieve records. This section will guide you through the process of using PHP to interact with the Salesforce Sandbox API, focusing on retrieving records such as accounts or contacts.
Prerequisites for PHP Integration with Salesforce Sandbox API
Before you begin, ensure that you have the following installed on your machine:
- PHP 7.4 or higher
- Composer, the PHP package manager
You'll also need to install the Guzzle HTTP client, which simplifies making HTTP requests in PHP. Run the following command to install Guzzle:
composer require guzzlehttp/guzzle
Example Code to Retrieve Records from Salesforce Sandbox
Below is a sample PHP script that demonstrates how to retrieve records from the Salesforce Sandbox API:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
// Set your Salesforce Sandbox credentials
$consumerKey = 'Your_Consumer_Key';
$consumerSecret = 'Your_Consumer_Secret';
$username = 'Your_Sandbox_Username';
$password = 'Your_Sandbox_Password';
$securityToken = 'Your_Security_Token';
// Obtain an access token
$response = $client->post('https://test.salesforce.com/services/oauth2/token', [
'form_params' => [
'grant_type' => 'password',
'client_id' => $consumerKey,
'client_secret' => $consumerSecret,
'username' => $username,
'password' => $password . $securityToken
]
]);
$accessToken = json_decode($response->getBody(), true)['access_token'];
// Make an API call to retrieve records
$response = $client->get('https://yourInstance.salesforce.com/services/data/vXX.X/sobjects/Account', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken
]
]);
$data = json_decode($response->getBody(), true);
// Output the retrieved records
foreach ($data['records'] as $record) {
echo 'Account Name: ' . $record['Name'] . "\n";
}
Replace Your_Consumer_Key
, Your_Consumer_Secret
, Your_Sandbox_Username
, Your_Sandbox_Password
, and Your_Security_Token
with your actual Salesforce Sandbox credentials. Also, replace yourInstance
with your Salesforce instance URL and vXX.X
with the appropriate API version.
Verifying Successful API Requests and Handling Errors
After running the script, you should see the account names printed in the console, indicating successful retrieval of records. To verify, you can log into your Salesforce Sandbox account and check the records.
Handling errors is crucial for robust integration. Common error codes include:
- 400 Bad Request: Check your request parameters.
- 401 Unauthorized: Verify your authentication credentials.
- 403 Forbidden: Ensure you have the necessary permissions.
For more detailed error handling, refer to the Salesforce API documentation.
Conclusion and Best Practices for Salesforce Sandbox API Integration
Integrating with the Salesforce Sandbox API using PHP provides developers with a robust way to access and manipulate Salesforce data securely. By following the steps outlined in this guide, you can efficiently retrieve records and ensure seamless data synchronization between Salesforce and other business applications.
Best Practices for Secure and Efficient Salesforce Sandbox API Integration
- Securely Store Credentials: Always store your OAuth credentials, such as the Consumer Key and Secret, securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handle Rate Limiting: Salesforce imposes rate limits on API requests. Monitor your API usage and implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that data fields are standardized and consistent across different systems to facilitate smooth data integration and reporting.
- Implement Robust Error Handling: Anticipate and handle common API errors, such as authentication failures and permission issues, to enhance the reliability of your integration.
Streamline Your Integration Process with Endgrate
While integrating with Salesforce Sandbox API can be rewarding, it may also be time-consuming and complex, especially if you need to manage multiple integrations. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Save time and resources by outsourcing integrations and ensuring a seamless connection between Salesforce and other platforms.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/salesforce-sandbox
- https://help.salesforce.com/s/articleView?id=sf.create_test_instance.htm&type=5
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/intro_oauth_and_connected_apps.htm
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/quickstart_dev_org.htm
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/resources_list.htm
Ready to get started?