Using the FTP API to Export Data in Javascript
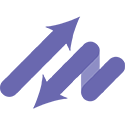
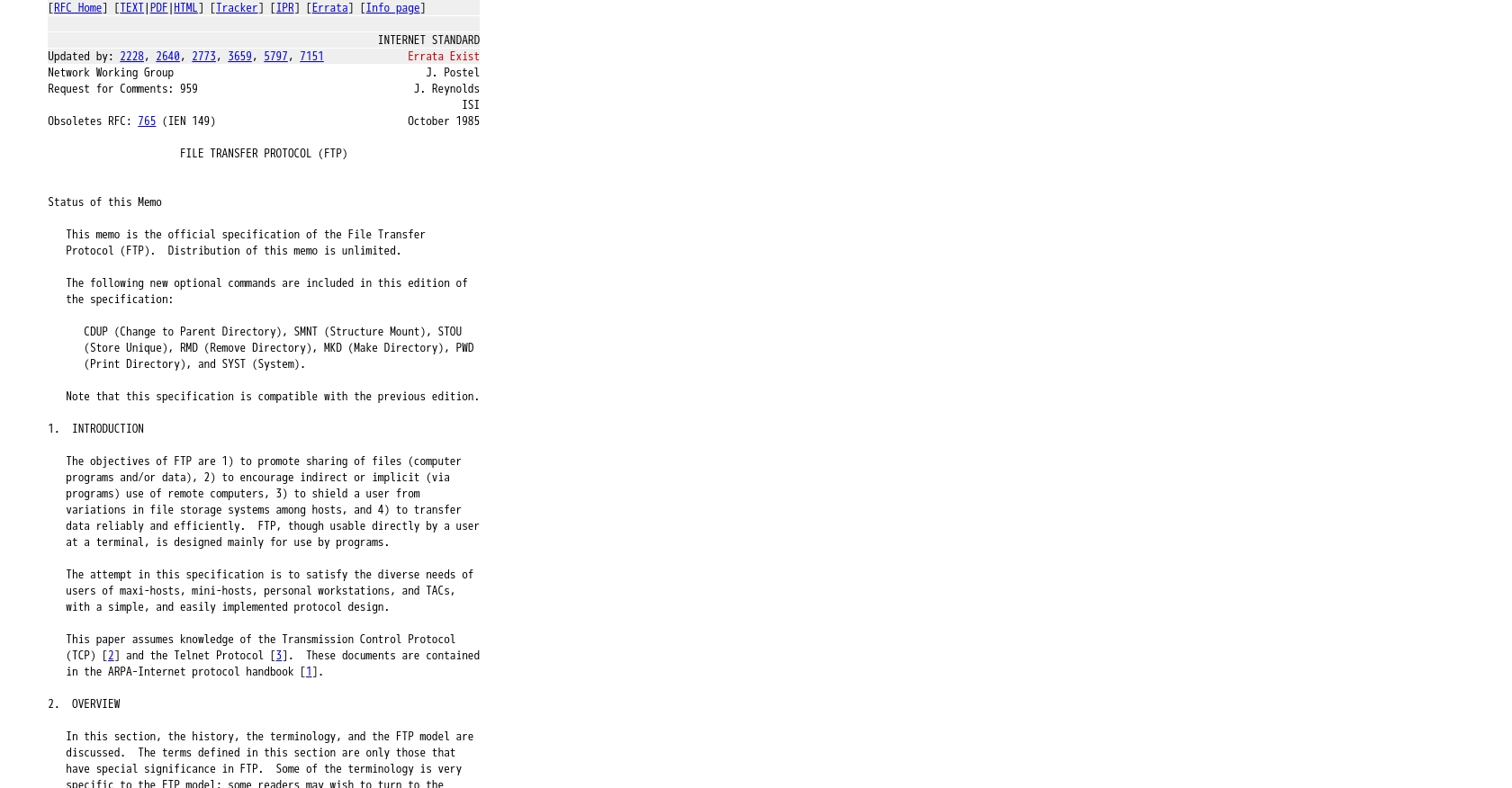
Introduction to FTP Data Export
File Transfer Protocol (FTP) is a standard network protocol used to transfer files between a client and server on a computer network. It is widely used for uploading and downloading files, making it an essential tool for businesses that need to manage large volumes of data efficiently.
Developers often need to integrate with FTP servers to automate data export processes. For example, a developer might use the FTP API to export sales data from a company's database to an external server for backup or analysis. This integration can streamline data management and ensure that critical information is securely stored and easily accessible.
Setting Up Your FTP Test Environment
Before diving into exporting data using the FTP API in JavaScript, it's crucial to set up a test environment. This will allow you to safely experiment with FTP operations without affecting live data. Here's how to get started:
Create a Test FTP Account
To begin, you'll need access to an FTP server. Many hosting providers offer free FTP accounts for testing purposes. Alternatively, you can set up a local FTP server on your machine using software like FileZilla Server or vsftpd.
- Download and install your chosen FTP server software.
- Create a new FTP user account with a username and password.
- Set the home directory for this user to a specific folder on your system where test files will be stored.
Configure FTP Server Settings
Ensure your FTP server is configured correctly to allow connections. Key settings include:
- Enable passive mode for better compatibility with firewalls.
- Set appropriate permissions for the test user to read and write files in the designated directory.
- Ensure the FTP server is running and accessible from your network.
Using a JavaScript FTP Library
To interact with the FTP server using JavaScript, you'll need a language-specific library. One popular choice is ftp, a Node.js module that simplifies FTP operations.
npm install ftp
Once installed, you can use this library to connect to your FTP server and perform data export operations.
Authentication with FTP
FTP uses a straightforward authentication method involving a username and password. When connecting to the server, you'll provide these credentials to establish a session. Here's a basic example of how to authenticate using the ftp
library:
const FTPClient = require('ftp');
const client = new FTPClient();
client.on('ready', function() {
console.log('Connected to FTP server');
// Proceed with data export operations
});
client.connect({
host: 'ftp.example.com',
user: 'your_username',
password: 'your_password'
});
Replace ftp.example.com
, your_username
, and your_password
with your FTP server's details.
With your test environment set up, you're ready to explore exporting data using the FTP API in JavaScript. This setup ensures you can safely test and refine your integration before deploying it in a production environment.
Executing FTP API Calls for Data Export in JavaScript
To effectively export data using the FTP API in JavaScript, you'll need to understand how to establish a connection and perform file operations. This section will guide you through the process of making API calls using the ftp
library in Node.js.
Setting Up Your JavaScript Environment for FTP Operations
Before making API calls, ensure your environment is ready. You'll need Node.js installed on your machine. If you haven't already, install the ftp
library:
npm install ftp
Connecting to the FTP Server
To start, you'll need to connect to your FTP server using the credentials set up in your test environment. Here's how you can establish a connection:
const FTPClient = require('ftp');
const client = new FTPClient();
client.on('ready', function() {
console.log('Connected to FTP server');
// Proceed with further operations
});
client.connect({
host: 'ftp.example.com',
user: 'your_username',
password: 'your_password'
});
Replace ftp.example.com
, your_username
, and your_password
with your actual FTP server details.
Exporting Data Files from FTP Server
Once connected, you can export data files from the FTP server. Here's an example of how to download a file:
client.get('remote/file/path.txt', function(err, stream) {
if (err) throw err;
stream.once('close', function() { client.end(); });
stream.pipe(fs.createWriteStream('local/file/path.txt'));
});
This code retrieves a file from the FTP server and saves it locally. Ensure you specify the correct remote and local file paths.
Handling FTP API Errors and Verifying Success
It's crucial to handle errors gracefully and verify the success of your operations. The ftp
library provides error handling through callbacks:
client.on('error', function(err) {
console.error('FTP connection error:', err);
});
Always check the existence and integrity of the exported files in your local directory to confirm successful data export.
Best Practices for FTP Data Export
- Ensure your FTP credentials are stored securely and not hardcoded in your scripts.
- Implement logging to track successful and failed operations for auditing purposes.
- Consider using secure FTP (SFTP) for enhanced security during data transfer.
By following these steps, you can efficiently export data using the FTP API in JavaScript, ensuring a smooth and secure integration process.
Conclusion: Best Practices for FTP Data Export in JavaScript
Exporting data using the FTP API in JavaScript can significantly enhance your data management processes, providing a reliable method for transferring files between servers. To ensure a seamless integration, consider the following best practices:
- Secure Credential Management: Store FTP credentials securely, using environment variables or secure vaults, to prevent unauthorized access.
- Implement Error Handling: Use robust error handling to manage connection issues and file transfer errors, ensuring that your application can recover gracefully.
- Monitor and Log Operations: Implement logging to track file transfers, which aids in auditing and troubleshooting any issues that arise during the export process.
- Consider Secure FTP (SFTP): For enhanced security, consider using SFTP, which encrypts data during transfer, protecting sensitive information from interception.
- Manage Rate Limits: Be aware of any rate limits imposed by your FTP server to avoid disruptions in service. Implement retry logic and backoff strategies if necessary.
By adhering to these practices, you can optimize your FTP data export processes, ensuring efficiency and security. For developers looking to streamline integration efforts further, consider leveraging Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Explore how Endgrate can simplify your integration experience by visiting Endgrate.
Read More
Ready to get started?