How to Create or Update Applicants with the JazzHR API in Javascript
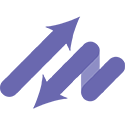
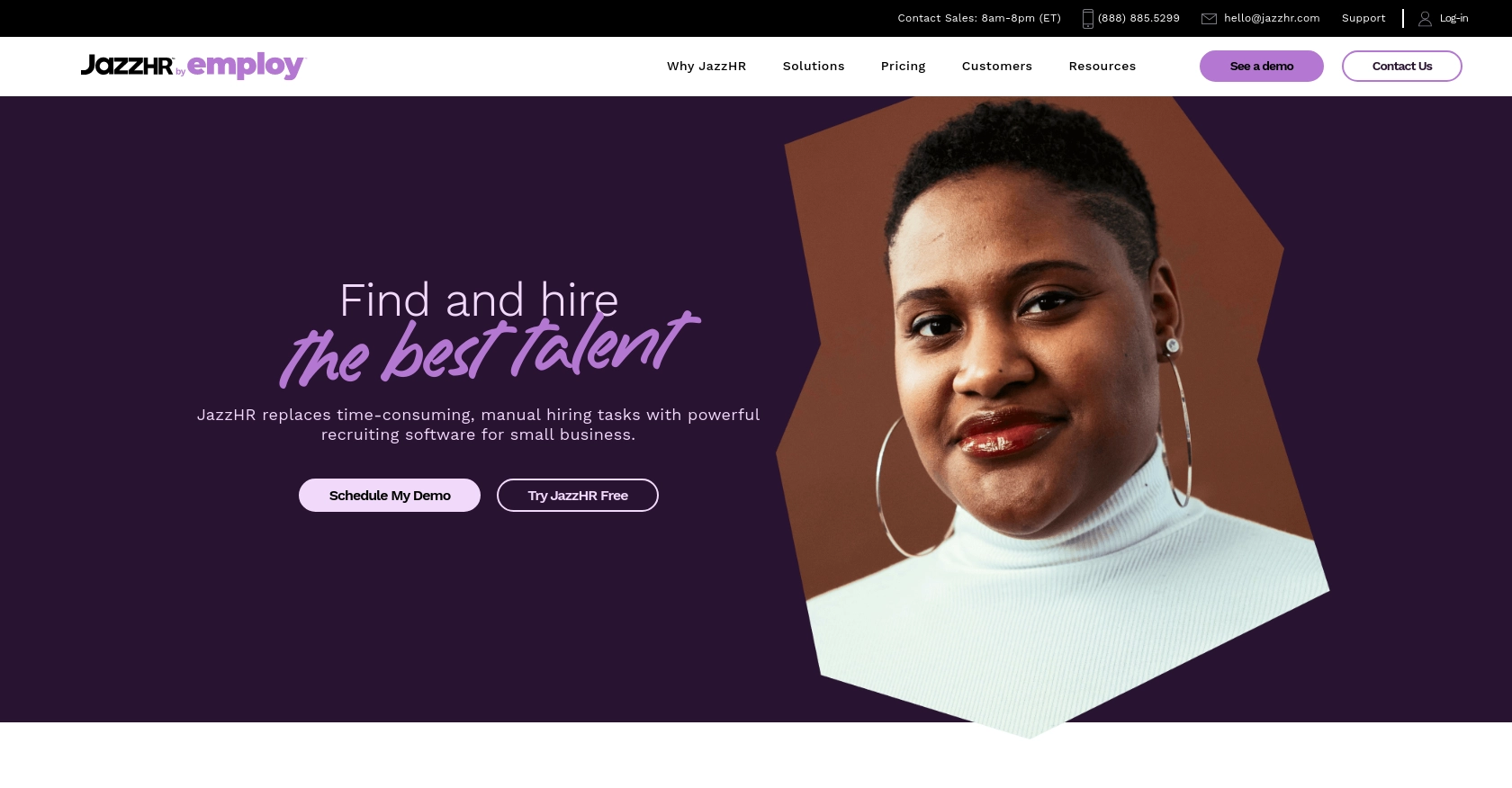
Introduction to JazzHR API Integration
JazzHR is a powerful recruiting software designed to streamline the hiring process for businesses of all sizes. It offers a comprehensive suite of tools to manage job postings, track applicants, and collaborate with hiring teams, making it an essential platform for modern recruitment.
Integrating with the JazzHR API allows developers to automate and enhance recruitment workflows. For example, you can create or update applicant information directly from your custom application, ensuring that your recruitment data is always up-to-date and synchronized across platforms.
This article will guide you through the process of using JavaScript to interact with the JazzHR API, specifically focusing on creating or updating applicants. By the end of this tutorial, you'll be equipped to leverage JazzHR's capabilities to optimize your recruitment processes.
Setting Up Your JazzHR Test Account for API Integration
Before you can start interacting with the JazzHR API using JavaScript, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Creating a JazzHR Account
If you don't already have a JazzHR account, you can sign up for a free trial on the JazzHR website. This will give you access to the platform's features and allow you to generate an API key for integration purposes.
- Visit the JazzHR website and click on the "Free Trial" button.
- Fill out the registration form with the required information and submit it.
- Once your account is created, log in to access the JazzHR dashboard.
Generating Your JazzHR API Key
To authenticate your API requests, you'll need an API key from JazzHR. Follow these steps to obtain it:
- Navigate to the "Integrations" section in your JazzHR account settings.
- Locate the API key section and generate a new API key if one hasn't been created yet.
- Copy the API key and store it securely, as you'll need it to authenticate your API calls.
For more detailed instructions, refer to the JazzHR API Overview.
Understanding JazzHR API Rate Limits
When working with the JazzHR API, it's important to be aware of the rate limits to ensure smooth operation. Each API call is limited to 100 results per page. If you need to retrieve more results, you can paginate by appending "/page/#" to the request URI, where # is the page number.
Keep these limits in mind as you develop your integration to avoid hitting the rate cap and ensure efficient data retrieval.
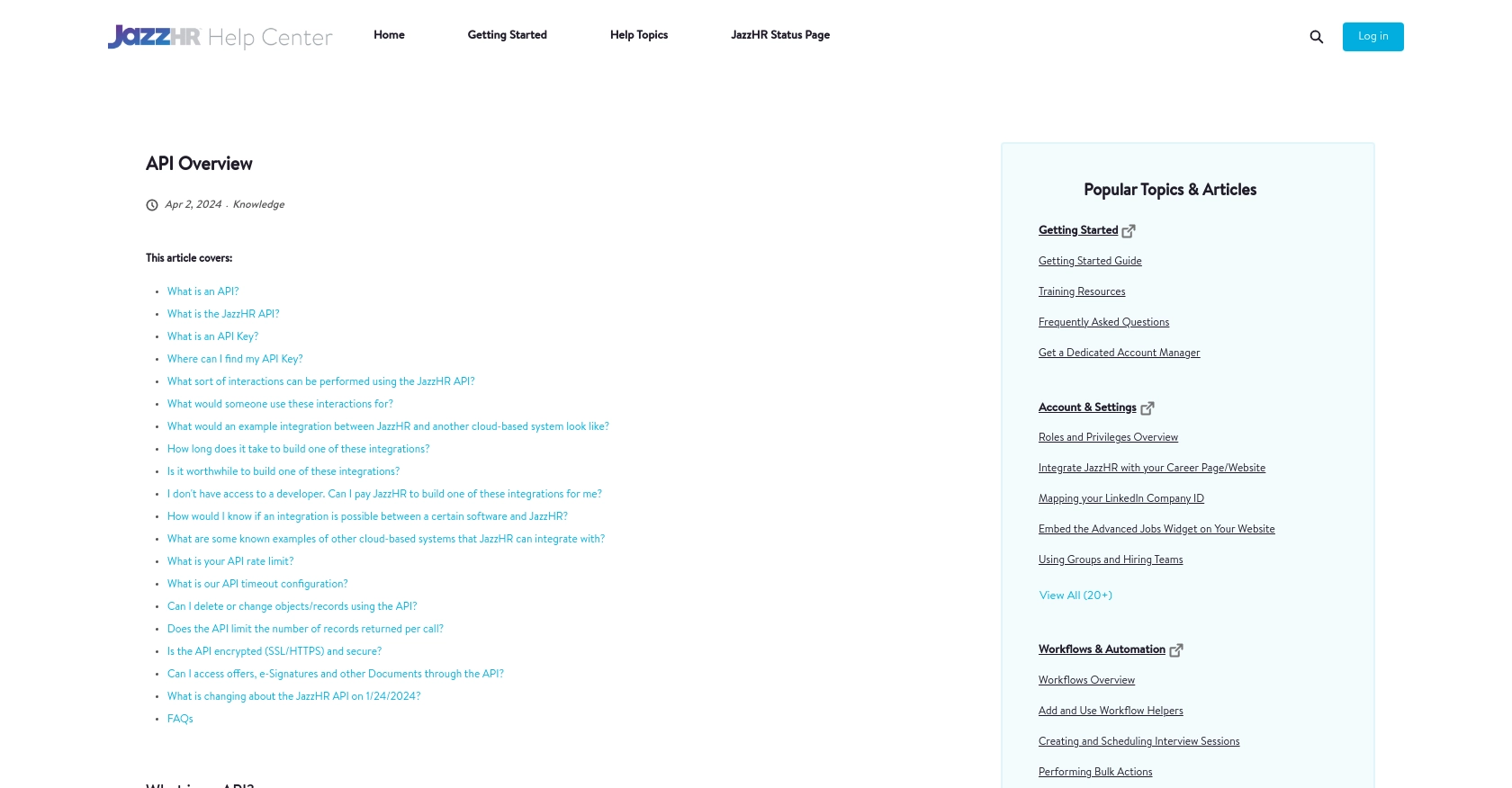
sbb-itb-96038d7
Making API Calls to JazzHR Using JavaScript
To interact with the JazzHR API and manage applicants, you'll need to make HTTP requests using JavaScript. This section will guide you through the process of setting up your environment, writing the code to create or update applicants, and handling potential errors.
Setting Up Your JavaScript Environment for JazzHR API Integration
Before you begin coding, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A code editor, such as Visual Studio Code.
- The
axios
library for making HTTP requests. You can install it using npm:
npm install axios
Creating or Updating Applicants with JazzHR API
Now, let's write the JavaScript code to create or update applicants using the JazzHR API. Start by creating a new file named jazzhr_applicants.js
and add the following code:
const axios = require('axios');
// Your JazzHR API key
const apiKey = 'Your_API_Key';
// Function to create or update an applicant
async function createOrUpdateApplicant(applicantData) {
const url = 'https://api.resumatorapi.com/v1/applicants';
const headers = {
'Content-Type': 'application/json',
'Authorization': `Bearer ${apiKey}`
};
try {
const response = await axios.post(url, applicantData, { headers });
console.log('Applicant Created/Updated Successfully:', response.data);
} catch (error) {
console.error('Error Creating/Updating Applicant:', error.response ? error.response.data : error.message);
}
}
// Sample applicant data
const applicantData = {
first_name: 'John',
last_name: 'Doe',
email: 'johndoe@example.com',
phone: '123-456-7890'
};
// Call the function
createOrUpdateApplicant(applicantData);
Replace Your_API_Key
with the API key you generated from your JazzHR account. The applicantData
object contains the applicant's information you wish to create or update.
Verifying API Call Success and Handling Errors
After running the script, you should see a success message in the console if the applicant is created or updated successfully. If there's an error, the error message will be logged, helping you diagnose the issue.
To verify the changes, log in to your JazzHR test account and check the applicants' section to see if the new or updated applicant appears.
Handling JazzHR API Rate Limits
Remember that the JazzHR API has a rate limit of 100 results per page. If you encounter rate limit errors, consider implementing a delay between requests or optimizing your data retrieval strategy.
For more information on handling errors and rate limits, refer to the JazzHR API Overview.
Conclusion and Best Practices for JazzHR API Integration
Integrating with the JazzHR API using JavaScript can significantly enhance your recruitment processes by automating applicant management. By following the steps outlined in this guide, you can efficiently create or update applicant information, ensuring your data remains synchronized and up-to-date.
Best Practices for Secure and Efficient JazzHR API Usage
- Secure API Key Storage: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of JazzHR's rate limits. Implement strategies such as request throttling or batching to avoid exceeding these limits and ensure smooth API interactions.
- Data Standardization: Ensure that applicant data is standardized before sending it to the API. This helps maintain consistency and reduces the likelihood of errors.
- Error Handling: Implement robust error handling to manage API call failures gracefully. Log errors for troubleshooting and provide meaningful feedback to users.
Streamlining Integrations with Endgrate
While integrating with individual APIs like JazzHR can be powerful, managing multiple integrations can become complex. Endgrate offers a unified API solution that simplifies this process, allowing you to focus on your core product development. By using Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers.
Explore how Endgrate can save you time and resources by visiting Endgrate and discover the benefits of outsourcing your integration needs.
Read More
Ready to get started?