Using the FreshDesk API to Create or Update Contacts in PHP
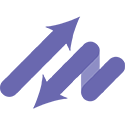
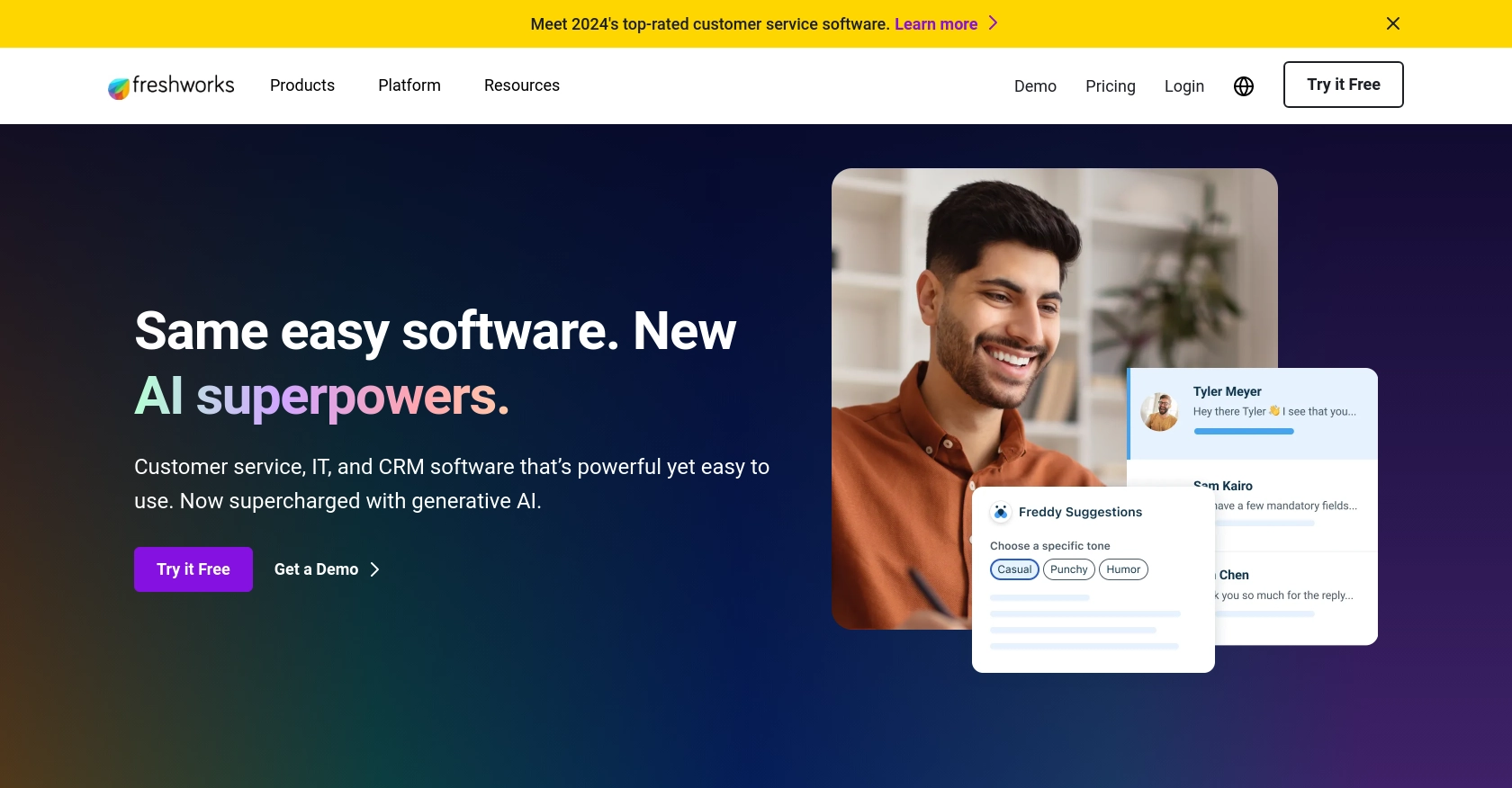
Introduction to FreshDesk API Integration
FreshDesk is a powerful customer support software that enables businesses to efficiently manage customer inquiries and support tickets. With its robust set of features, FreshDesk helps organizations streamline their customer service operations, improve response times, and enhance customer satisfaction.
Integrating with the FreshDesk API allows developers to automate and customize various support processes. For example, you can use the FreshDesk API to create or update contact information directly from your application, ensuring that your customer data remains accurate and up-to-date across all platforms.
This article will guide you through the process of using PHP to interact with the FreshDesk API, focusing on creating or updating contacts. By following these steps, developers can leverage FreshDesk's capabilities to enhance their customer support systems.
Setting Up Your FreshDesk Test Account
Before you can start integrating with the FreshDesk API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data.
Creating a FreshDesk Account
If you don't already have a FreshDesk account, you can sign up for a free trial on the FreshDesk website. This trial will give you access to all the features you need to test the API integration.
- Visit the FreshDesk signup page.
- Fill in the required information, such as your name, email, and company details.
- Follow the instructions to complete the registration process.
Generating an API Key for Authentication
FreshDesk uses a custom authentication method that involves an API key. Here's how you can generate your API key:
- Log in to your FreshDesk account.
- Navigate to your profile settings by clicking on your profile icon in the top right corner.
- Under the "API" section, you'll find your API key. Copy this key and store it securely, as you'll need it for authenticating your API requests.
Creating a FreshDesk App for API Access
To interact with the FreshDesk API, you may need to create an app within your FreshDesk account. This app will allow you to manage permissions and access levels for your API interactions.
- Go to the "Admin" section in your FreshDesk dashboard.
- Select "Apps" and click on "Create New App."
- Fill in the necessary details, such as the app name and description.
- Set the required permissions for accessing and modifying contact data.
- Save your app settings to finalize the creation process.
With your FreshDesk account and API key ready, you can now proceed to make API calls using PHP to create or update contacts. This setup ensures that you have the necessary credentials and permissions to interact with the FreshDesk API effectively.
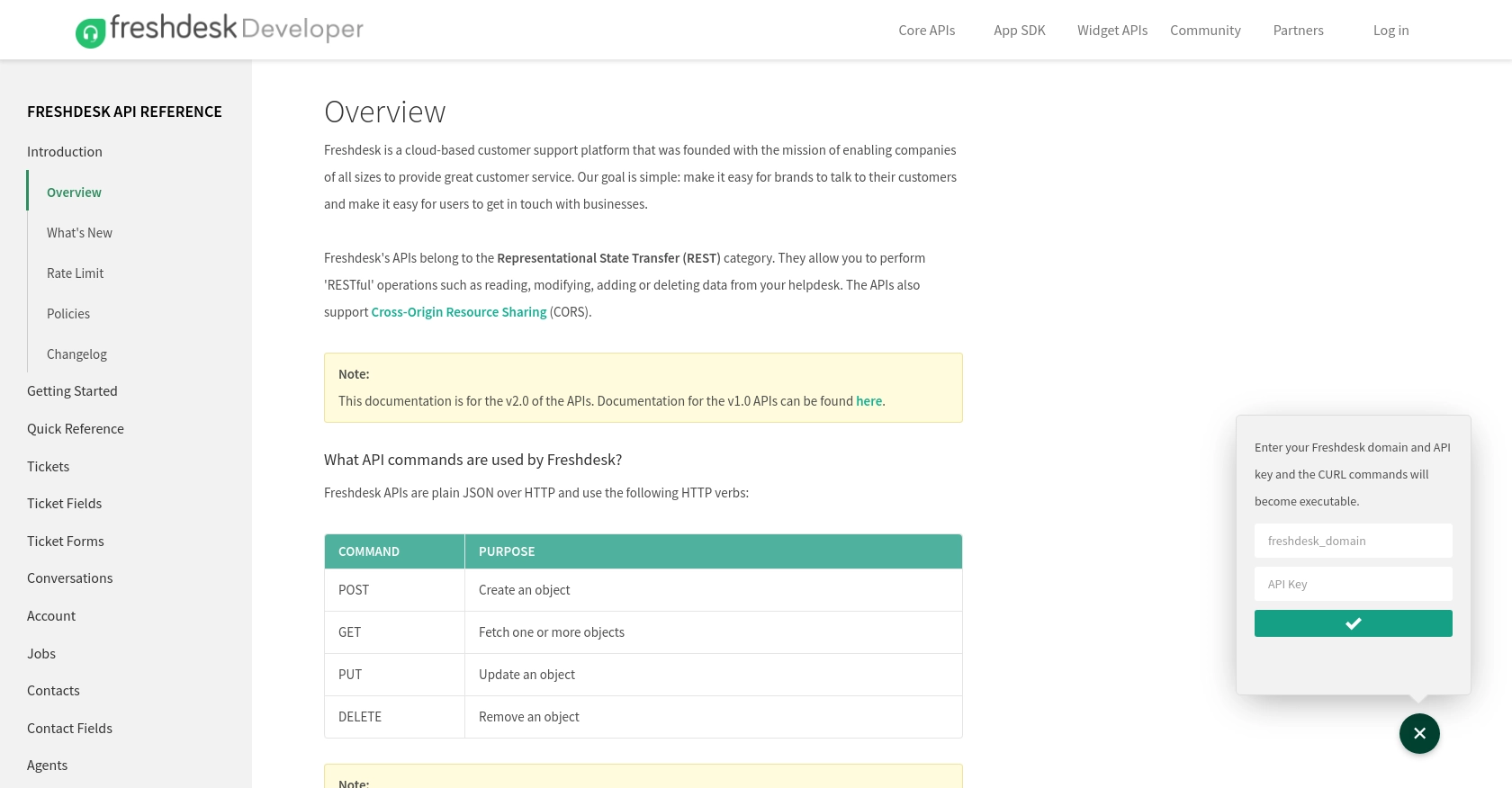
sbb-itb-96038d7
Making API Calls with PHP to FreshDesk for Contact Management
To interact with the FreshDesk API using PHP, you'll need to set up your development environment and write the necessary code to create or update contacts. This section will guide you through the process, ensuring you have everything you need to successfully make API calls.
Setting Up Your PHP Environment for FreshDesk API Integration
Before diving into the code, ensure that your PHP environment is properly configured. You'll need the following:
- PHP 7.4 or higher installed on your machine.
- The
cURL
extension enabled in your PHP configuration, as it will be used to make HTTP requests.
Installing Required PHP Dependencies
While PHP's built-in cURL
functions are sufficient for making API calls, you may also consider using a library like Guzzle for more advanced HTTP requests. If you choose to use Guzzle, you can install it via Composer:
composer require guzzlehttp/guzzle
Creating or Updating Contacts with FreshDesk API Using PHP
With your environment set up, you can now write the PHP code to interact with the FreshDesk API. Below is an example of how to create or update a contact:
<?php
// FreshDesk API endpoint for creating or updating contacts
$apiUrl = 'https://yourdomain.freshdesk.com/api/v2/contacts';
// Your FreshDesk API key
$apiKey = 'your_api_key_here';
// Contact data to be created or updated
$contactData = [
'name' => 'John Doe',
'email' => 'john.doe@example.com',
'phone' => '1234567890'
];
// Initialize cURL session
$ch = curl_init($apiUrl);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Content-Type: application/json'
]);
curl_setopt($ch, CURLOPT_USERPWD, $apiKey . ':X');
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($contactData));
// Execute cURL request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Decode and display response
$responseData = json_decode($response, true);
echo 'Contact ID: ' . $responseData['id'];
}
// Close cURL session
curl_close($ch);
?>
Replace yourdomain
with your FreshDesk domain and your_api_key_here
with your actual API key.
Verifying API Call Success in FreshDesk
After executing the PHP script, you can verify the success of the API call by checking the FreshDesk dashboard. Navigate to the Contacts section to see if the contact has been created or updated as expected.
Handling Errors and Troubleshooting
When making API calls, it's essential to handle potential errors. The FreshDesk API will return HTTP status codes to indicate success or failure:
- 200 OK: The request was successful.
- 201 Created: The contact was successfully created.
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 404 Not Found: The requested resource could not be found.
Ensure you check these status codes in your code to handle errors gracefully and provide meaningful feedback to users.
Conclusion and Best Practices for FreshDesk API Integration
Integrating with the FreshDesk API using PHP provides a powerful way to automate and enhance your customer support processes. By creating or updating contacts programmatically, you ensure that your customer data remains consistent and up-to-date across all platforms.
Storing FreshDesk API Credentials Securely
It's crucial to store your FreshDesk API credentials securely to prevent unauthorized access. Consider using environment variables or secure vaults to manage sensitive information like API keys.
Handling FreshDesk API Rate Limits
While the specific rate limits for FreshDesk API requests are not provided in the documentation, it's essential to implement error handling and retry logic to manage potential rate limiting. Monitor your API usage and adjust your request frequency accordingly to avoid hitting any limits.
Standardizing Data Fields for Consistency
When working with contact data, ensure that fields such as names, emails, and phone numbers are standardized. This consistency helps maintain data integrity and improves the efficiency of your support operations.
Enhancing Your Integration with Endgrate
For developers looking to streamline multiple integrations, Endgrate offers a unified API solution. By connecting to various platforms through a single endpoint, you can save time and resources, allowing you to focus on your core product development. Explore how Endgrate can simplify your integration processes and enhance your customer experience.
Read More
Ready to get started?