Using the Shopify API to Create or Update Products in Javascript
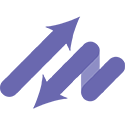
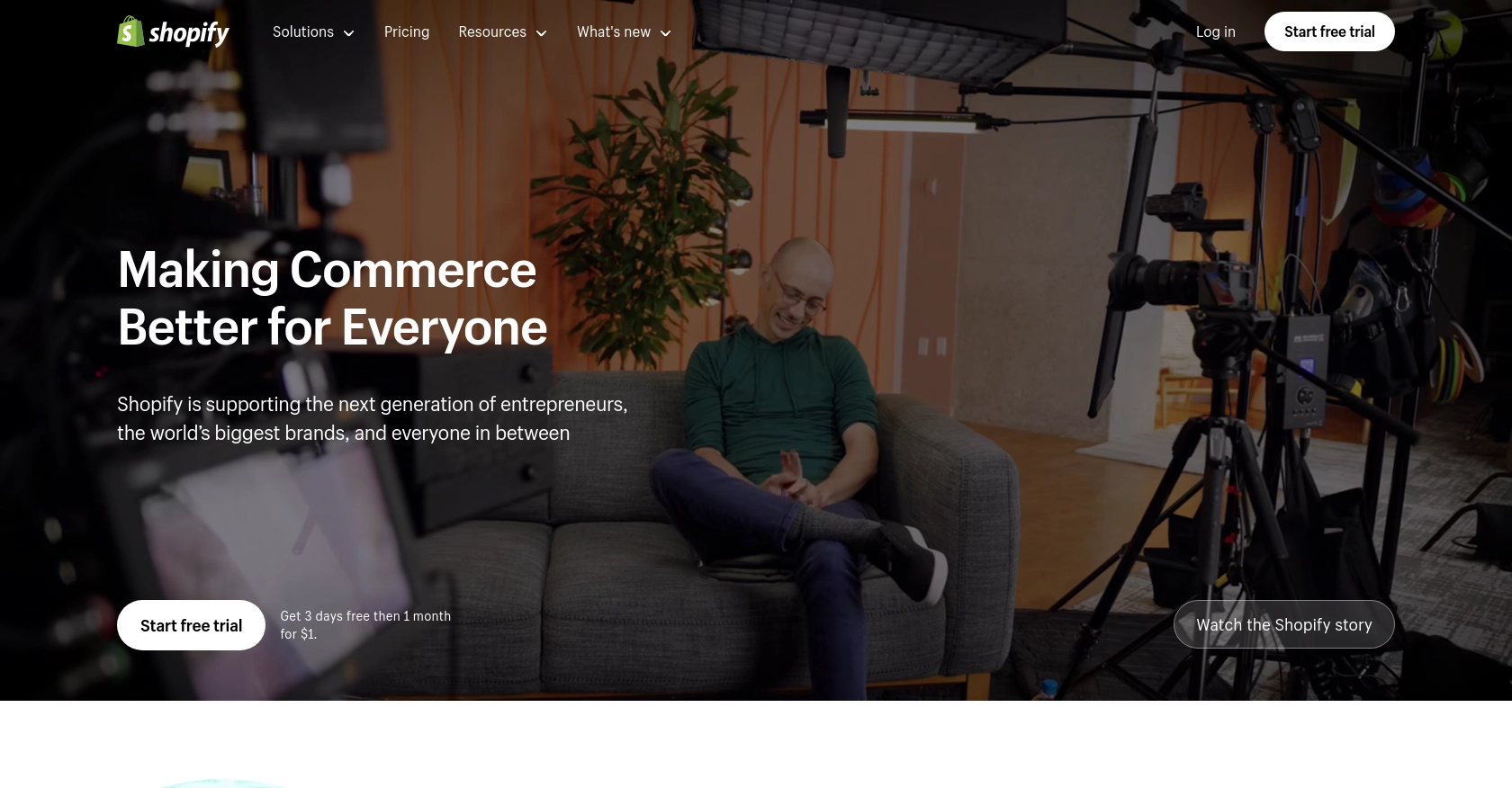
Introduction to Shopify API Integration
Shopify is a leading e-commerce platform that empowers businesses to create and manage their online stores with ease. It offers a robust suite of tools for managing products, processing orders, and handling customer interactions, making it a popular choice for businesses of all sizes.
Integrating with Shopify's API allows developers to automate and streamline various e-commerce operations. For example, you can use the Shopify API to create or update product listings directly from your application, ensuring that your store's inventory is always up-to-date and accurate.
This article will guide you through the process of using JavaScript to interact with the Shopify API, specifically focusing on creating and updating products. By leveraging this integration, developers can enhance their e-commerce solutions and provide a seamless experience for their users.
Setting Up Your Shopify Test or Sandbox Account
Before you can start integrating with the Shopify API, you'll need to set up a Shopify account. This will allow you to test your API interactions in a controlled environment without affecting a live store.
Create a Shopify Development Store
To begin, you'll need to create a Shopify development store. This type of store is free and specifically designed for testing and development purposes. Follow these steps to set up your development store:
- Visit the Shopify Partners page and sign up for a Shopify Partner account.
- Once your account is created, log in to the Shopify Partner Dashboard.
- Navigate to the "Stores" section and click on "Add store."
- Select "Development store" as the store type and fill in the required details.
- Click "Save" to create your development store.
Generate Shopify API Credentials
With your development store set up, the next step is to generate the necessary API credentials to authenticate your requests. Shopify uses OAuth for authentication, so you'll need to create a private app to obtain these credentials:
- Log in to your Shopify development store.
- Go to "Apps" in the left-hand menu and click on "Develop apps."
- Click "Create an app" and provide a name for your app.
- Under "Configuration," click "Configure Admin API scopes" and select the necessary scopes for product management, such as "write_products" and "read_products."
- Click "Save" to apply the changes.
- Once saved, navigate to the "API credentials" tab to find your API key and secret key.
Make sure to store these credentials securely, as they will be used to authenticate your API requests.
Authorize Your App with OAuth
To interact with the Shopify API, you'll need to authorize your app using OAuth. Follow these steps to complete the authorization process:
- Construct an authorization URL using your API key, scopes, and redirect URI.
- Direct your browser to this URL and log in with your Shopify store credentials.
- Once authorized, Shopify will redirect you to the specified redirect URI with a temporary code.
- Exchange this code for a permanent access token by making a POST request to the Shopify token endpoint.
With the access token, you can now make authenticated requests to the Shopify API.
For more detailed information on authentication, refer to the Shopify API authentication documentation.
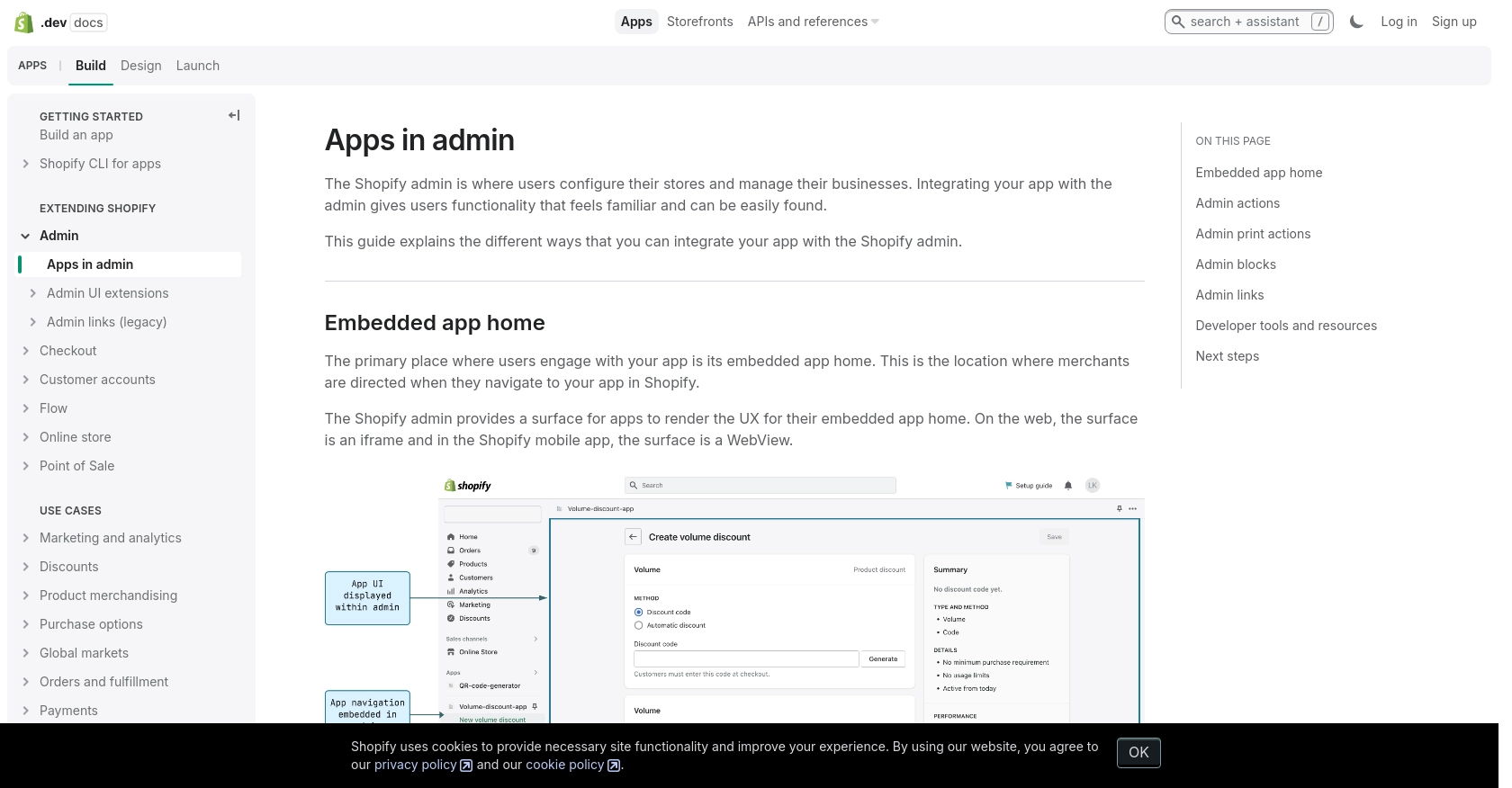
sbb-itb-96038d7
Making API Calls to Shopify for Product Management Using JavaScript
To interact with the Shopify API for creating or updating products, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling responses and errors effectively.
Setting Up Your JavaScript Environment for Shopify API Integration
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides the runtime environment for executing JavaScript outside the browser. You can download it from the official Node.js website.
Once Node.js is installed, you can use npm (Node Package Manager) to install the Axios library, which simplifies making HTTP requests:
npm install axios
Creating a New Product in Shopify Using JavaScript
To create a new product, you'll need to send a POST request to the Shopify API endpoint. Below is a sample code snippet demonstrating how to create a product:
const axios = require('axios');
const createProduct = async () => {
const url = 'https://your-development-store.myshopify.com/admin/api/2024-07/products.json';
const accessToken = 'your_access_token';
const productData = {
product: {
title: 'New Product',
body_html: 'Great product!',
vendor: 'Your Vendor',
product_type: 'Type',
status: 'active'
}
};
try {
const response = await axios.post(url, productData, {
headers: {
'Content-Type': 'application/json',
'X-Shopify-Access-Token': accessToken
}
});
console.log('Product created:', response.data);
} catch (error) {
console.error('Error creating product:', error.response.data);
}
};
createProduct();
Replace your_access_token
with the access token obtained during the OAuth process. This code sends a POST request to create a new product with the specified details. If successful, it logs the created product's information.
Updating an Existing Product in Shopify Using JavaScript
To update a product, you'll need to send a PUT request to the Shopify API. Here's how you can update a product:
const updateProduct = async (productId) => {
const url = `https://your-development-store.myshopify.com/admin/api/2024-07/products/${productId}.json`;
const accessToken = 'your_access_token';
const updatedProductData = {
product: {
id: productId,
title: 'Updated Product Title'
}
};
try {
const response = await axios.put(url, updatedProductData, {
headers: {
'Content-Type': 'application/json',
'X-Shopify-Access-Token': accessToken
}
});
console.log('Product updated:', response.data);
} catch (error) {
console.error('Error updating product:', error.response.data);
}
};
updateProduct('product_id_here');
Replace product_id_here
with the ID of the product you wish to update. This code updates the product's title and logs the updated product information if successful.
Handling Shopify API Responses and Errors
When making API calls, it's crucial to handle responses and errors properly. Shopify API responses include status codes that indicate the success or failure of the request. Common status codes include:
- 200 OK: The request was successful.
- 201 Created: The resource was successfully created.
- 401 Unauthorized: Authentication failed. Check your access token.
- 429 Too Many Requests: Rate limit exceeded. Wait before retrying.
For more detailed information on status codes and error handling, refer to the Shopify API documentation.
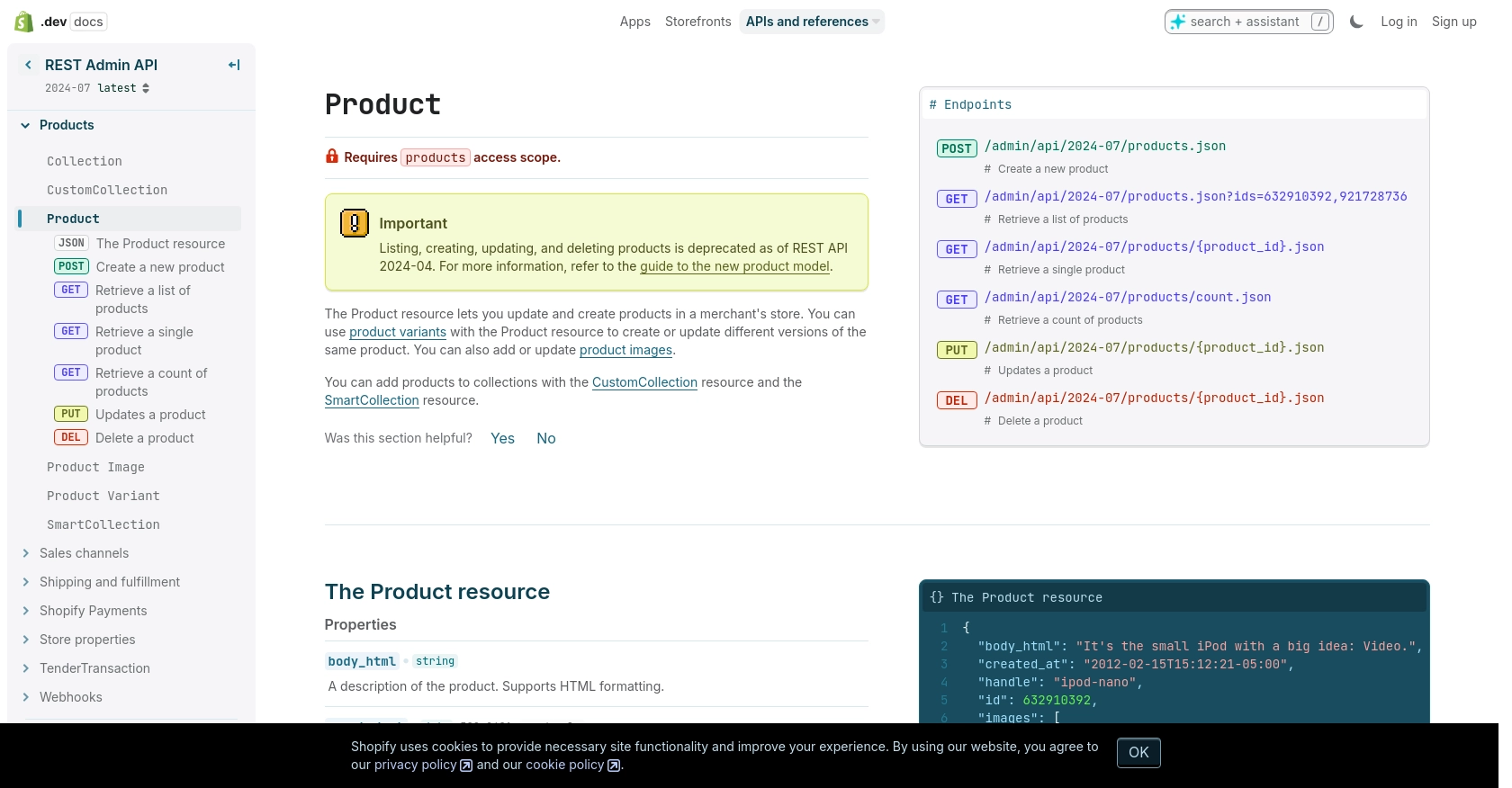
Conclusion and Best Practices for Shopify API Integration
Integrating with the Shopify API using JavaScript provides a powerful way to manage your e-commerce store's products programmatically. By following the steps outlined in this article, you can create and update products efficiently, ensuring your store's inventory remains accurate and up-to-date.
Best Practices for Secure and Efficient Shopify API Usage
- Securely Store Credentials: Always store your API credentials securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limits: Shopify's API has a rate limit of 40 requests per app per store per minute. Implement logic to handle the
429 Too Many Requests
error by checking theRetry-After
header and pausing requests accordingly. For more details, refer to the Shopify API documentation. - Data Transformation: Ensure that data sent to and received from the API is properly transformed and standardized to match your application's requirements.
- Monitor API Usage: Regularly monitor your API usage and error logs to identify and address any issues promptly.
Enhancing E-commerce Solutions with Endgrate
While integrating with Shopify's API can significantly enhance your e-commerce solutions, managing multiple integrations can be complex and time-consuming. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to various platforms, including Shopify.
With Endgrate, you can save time and resources by building once for each use case instead of multiple times for different integrations. This allows you to focus on your core product while offering an intuitive integration experience for your customers. Explore how Endgrate can simplify your integration needs by visiting Endgrate's website.
Read More
Ready to get started?