Using the Younium API to Get Products in PHP
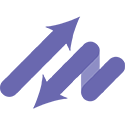
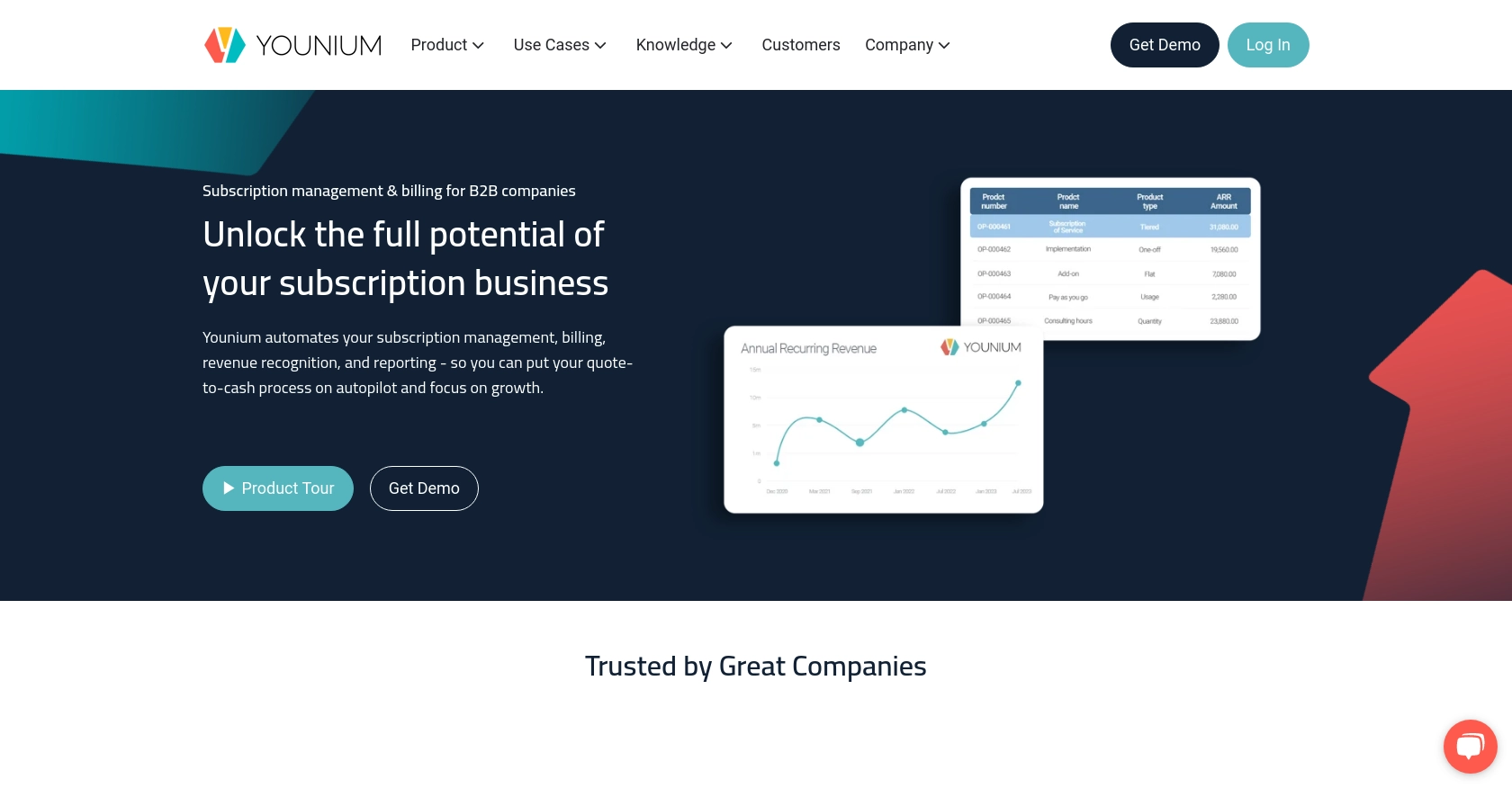
Introduction to Younium API Integration
Younium is a comprehensive subscription management platform designed to streamline billing, invoicing, and financial operations for B2B SaaS companies. It offers a robust set of tools that help businesses manage their recurring revenue and subscription lifecycle efficiently.
Integrating with the Younium API allows developers to automate and enhance their subscription management processes. For example, you might want to retrieve product information from Younium to display in your application, enabling seamless synchronization of product data across platforms.
Setting Up Your Younium Sandbox Account for API Integration
Before you can start interacting with the Younium API, you'll need to set up a sandbox account. This environment allows you to test and develop your integration without affecting your live data. Follow these steps to get started:
Creating a Younium Sandbox Account
If you don't already have a Younium account, you can sign up for a sandbox account on the Younium website. This account will provide you with the necessary environment to test API interactions.
- Visit the Younium Developer Portal.
- Follow the instructions to create a sandbox account.
- Once your account is created, log in to access the sandbox environment.
Generating API Tokens and Client Credentials
To authenticate your API requests, you'll need to generate API tokens and client credentials. Here's how to do it:
- Log in to your Younium sandbox account.
- Open the user profile menu by clicking your name in the top right corner and select "Privacy & Security."
- In the left panel, click on "Personal Tokens" and then "Generate Token."
- Provide a relevant description and click "Create."
- Copy the generated Client ID and Secret Key. These credentials are crucial for generating your JWT access token and will not be visible again.
Acquiring a JWT Access Token
With your client credentials in hand, you can now generate a JWT access token to authenticate your API requests:
$clientId = 'Your_Client_ID';
$secret = 'Your_Secret_Key';
$url = 'https://api.sandbox.younium.com/auth/token';
$headers = [
'Content-Type: application/json'
];
$body = json_encode([
'clientId' => $clientId,
'secret' => $secret
]);
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $body);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
$data = json_decode($response, true);
$accessToken = $data['accessToken'];
Replace Your_Client_ID
and Your_Secret_Key
with the credentials you generated earlier. This code snippet sends a POST request to the Younium authentication endpoint to obtain a JWT access token.
Once you have your access token, you can use it to authenticate your API calls to Younium. Remember, the token is valid for 24 hours, so you'll need to refresh it as needed.
For more detailed information, refer to the Younium Authentication Documentation.
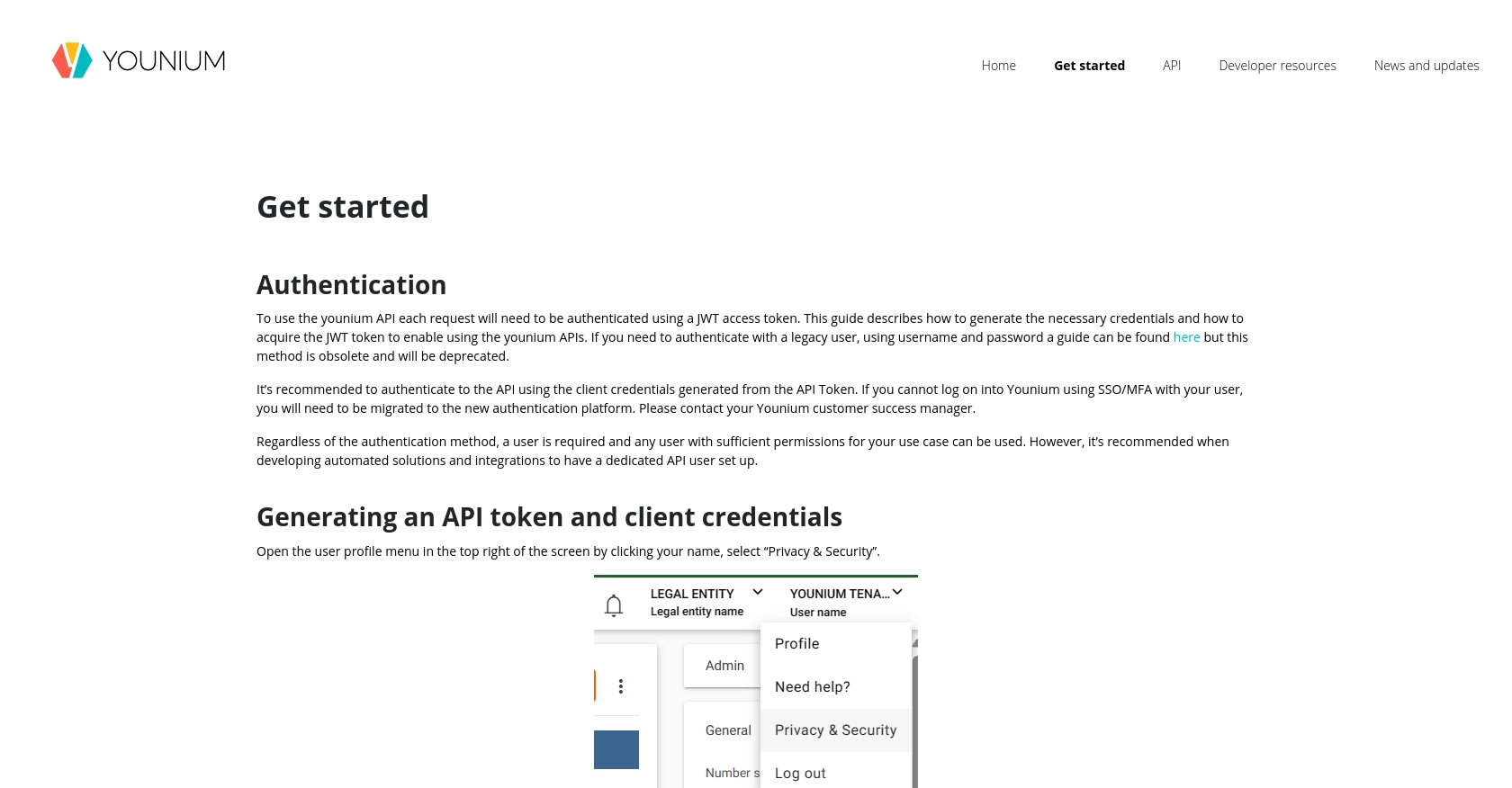
sbb-itb-96038d7
Making API Calls to Retrieve Products from Younium Using PHP
Now that you have your JWT access token, you can proceed to make authenticated API calls to Younium. In this section, we'll guide you through the process of retrieving product information using PHP. This will allow you to seamlessly integrate Younium's product data into your application.
Setting Up Your PHP Environment for Younium API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need:
- PHP 7.4 or later
- cURL extension enabled
These prerequisites will allow you to execute HTTP requests and handle JSON responses effectively.
Retrieving Products from Younium API
To retrieve products, you'll need to make a GET request to the Younium API's products endpoint. Here's a step-by-step guide:
$accessToken = 'Your_Access_Token';
$url = 'https://api.sandbox.younium.com/products';
$headers = [
'Authorization: Bearer ' . $accessToken,
'Content-Type: application/json',
'api-version: 2.1'
];
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
$data = json_decode($response, true);
if (isset($data['data'])) {
foreach ($data['data'] as $product) {
echo 'Product Name: ' . $product['name'] . "\n";
echo 'Product ID: ' . $product['id'] . "\n";
echo 'Description: ' . $product['description'] . "\n\n";
}
} else {
echo 'Failed to retrieve products. Please check your access token and API endpoint.';
}
Replace Your_Access_Token
with the JWT token you obtained earlier. This code snippet sends a GET request to the Younium products endpoint and processes the JSON response to display product details.
Verifying API Call Success and Handling Errors
After executing the code, you should see a list of products with their names, IDs, and descriptions. If the request fails, ensure your access token is valid and the API endpoint is correct.
Common error codes include:
- 401 Unauthorized: Indicates an expired or incorrect access token.
- 403 Forbidden: Suggests insufficient permissions or incorrect legal entity specification.
For more details on error handling, refer to the Younium API Documentation.
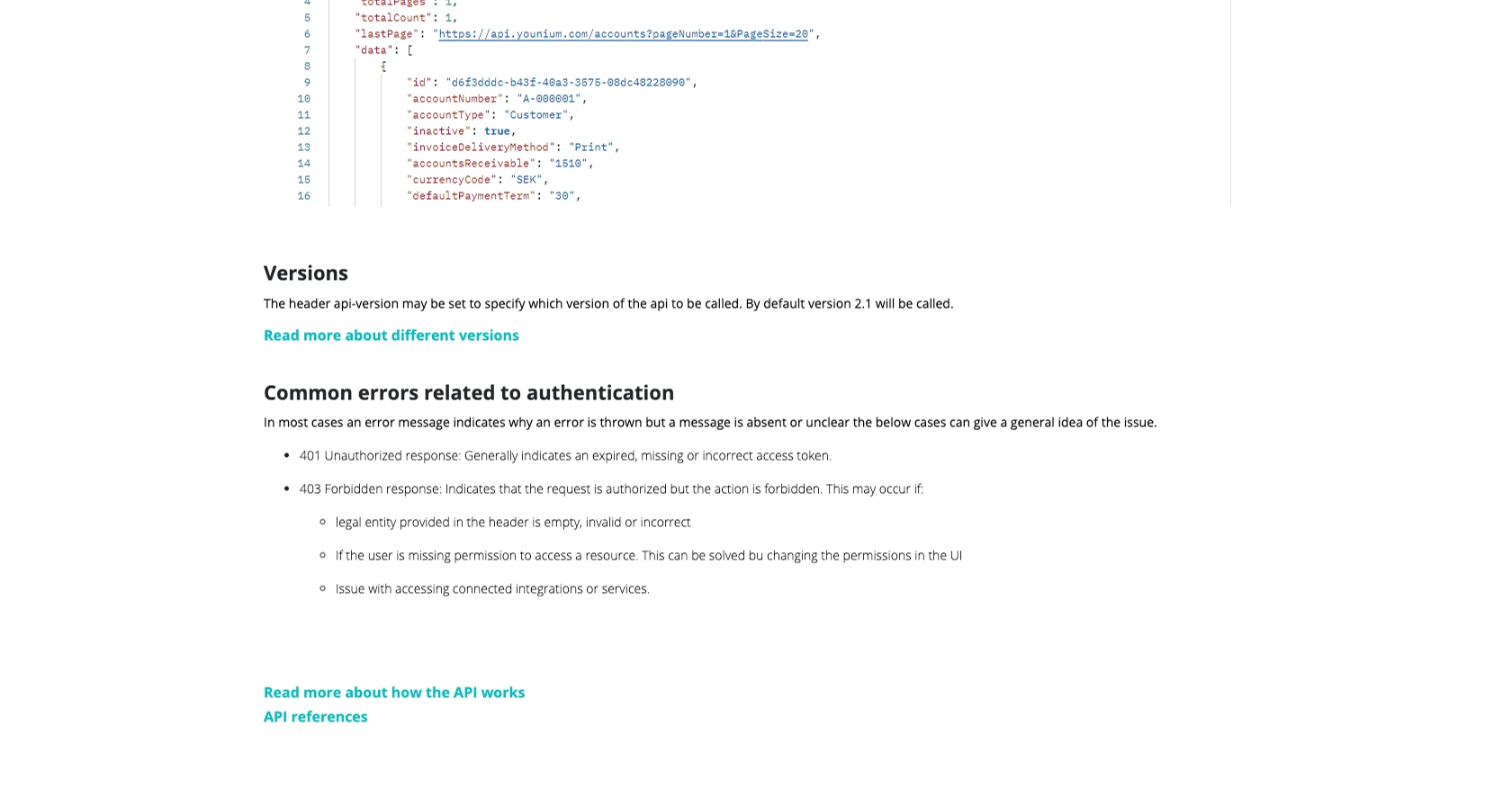
Conclusion and Best Practices for Younium API Integration in PHP
Integrating with the Younium API can significantly enhance your subscription management capabilities by automating product data retrieval and synchronization. By following the steps outlined in this guide, you can efficiently connect your PHP application to Younium and leverage its robust features.
Best Practices for Secure and Efficient Younium API Integration
- Securely Store Credentials: Always store your client credentials and JWT tokens securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Be mindful of API rate limits to avoid throttling. Implement retry logic with exponential backoff to manage rate limit responses gracefully.
- Refresh Tokens Regularly: Since JWT tokens are valid for 24 hours, ensure your application can refresh tokens automatically to maintain uninterrupted access.
- Standardize Data Fields: Transform and standardize data fields to ensure consistency across different platforms and services.
Streamlining Integrations with Endgrate
While integrating with Younium directly is powerful, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Younium. By using Endgrate, you can:
- Save time and resources by outsourcing integration development and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an easy, intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website.
Read More
Ready to get started?