Using the Clickup API to Create Teams (with PHP examples)
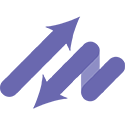
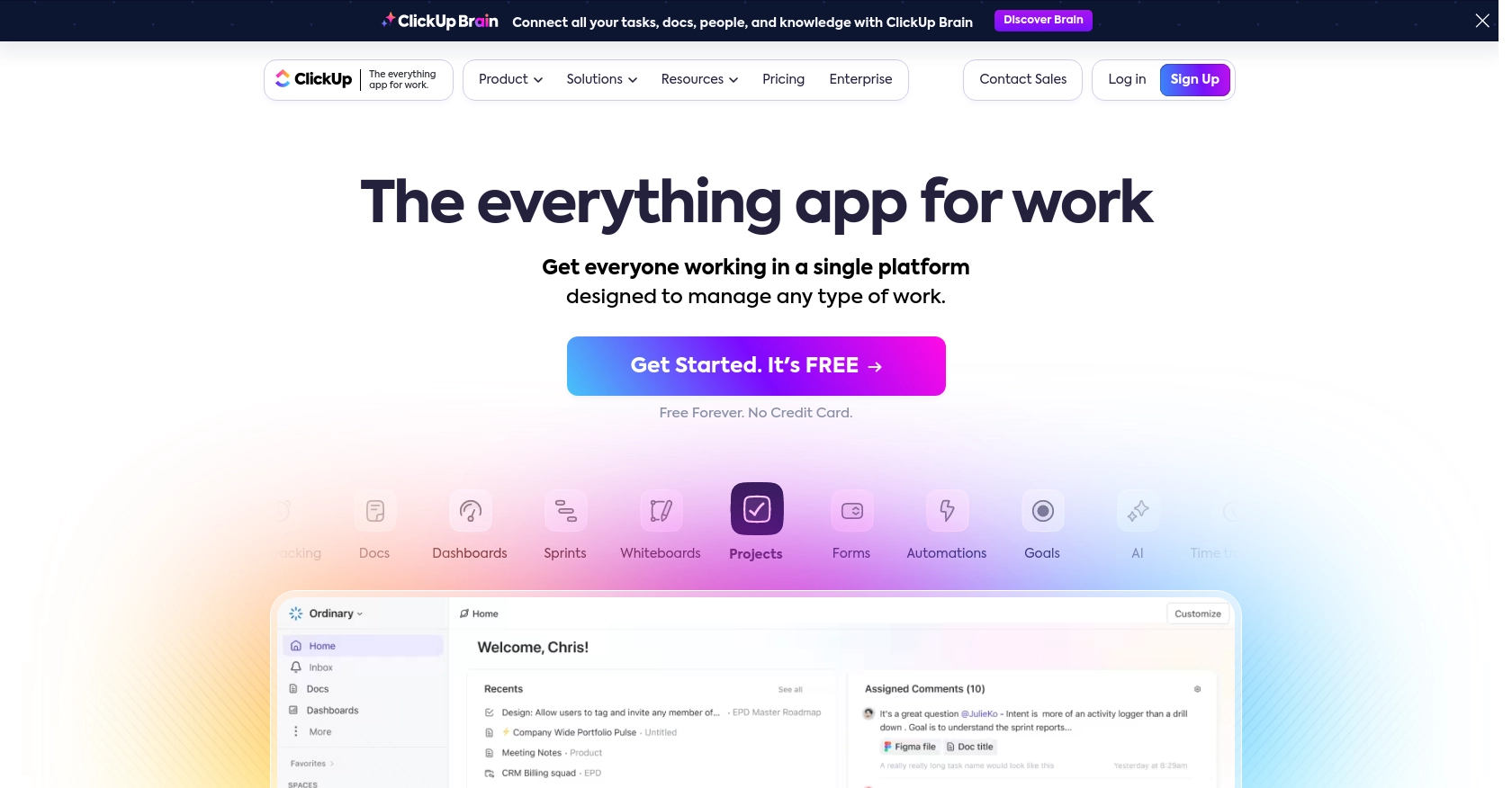
Introduction to ClickUp API Integration
ClickUp is a versatile project management and productivity tool designed to help teams organize tasks, manage workflows, and enhance collaboration. With its robust set of features, ClickUp is a popular choice for businesses seeking to streamline their operations and improve team efficiency.
Developers may want to integrate with ClickUp's API to automate and enhance their project management processes. For example, using the ClickUp API, a developer can create teams (user groups) within a workspace, allowing for better organization and assignment of tasks to specific groups of users.
This article will guide you through the process of using PHP to interact with the ClickUp API, specifically focusing on creating teams. By following this tutorial, you'll learn how to set up authentication, make API calls, and handle responses effectively.
Setting Up a ClickUp Test or Sandbox Account
Before diving into the ClickUp API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting your live data. ClickUp offers a straightforward process to get started with their API using OAuth authentication.
Creating a ClickUp Account
If you don't already have a ClickUp account, you can sign up for a free account on the ClickUp website. Follow the instructions to complete your registration. Once your account is set up, you can log in and access the necessary settings for API integration.
Generating a Personal API Token
For personal use or testing, you can generate a personal API token. Here's how:
- Log into your ClickUp account.
- In ClickUp 3.0, click your avatar in the upper-right corner and select Settings.
- Scroll down and click Apps in the sidebar.
- Under API Token, click Generate.
- Copy and save your personal API token for use in API requests.
Setting Up OAuth for Application Development
If you're developing an application for others, you'll need to use the OAuth flow. Follow these steps to create an OAuth app:
- Log into ClickUp and click your avatar in the lower-left corner.
- Select Integrations and then click ClickUp API.
- Click Create an App and provide a name and redirect URL for your app.
- Once created, you'll receive a
client_id
andclient_secret
.
For more details, refer to the ClickUp authentication documentation.
Retrieving an Authorization Code
To connect your app with a user's ClickUp account, share the following URL with them:
https://app.clickup.com/api?client_id={client_id}&redirect_uri={redirect_uri}
Replace {client_id}
and {redirect_uri}
with your app's details. After authorization, users will be redirected back to your app with the necessary query parameters to obtain an access token.
Requesting an Access Token
Use the authorization code to request an access token by calling the Get Access token endpoint with the client_id
, client_secret
, and code
parameters. This token will be used in the Authorization header for API requests.
With your ClickUp account and authentication set up, you're ready to start making API calls to create teams within your workspace.
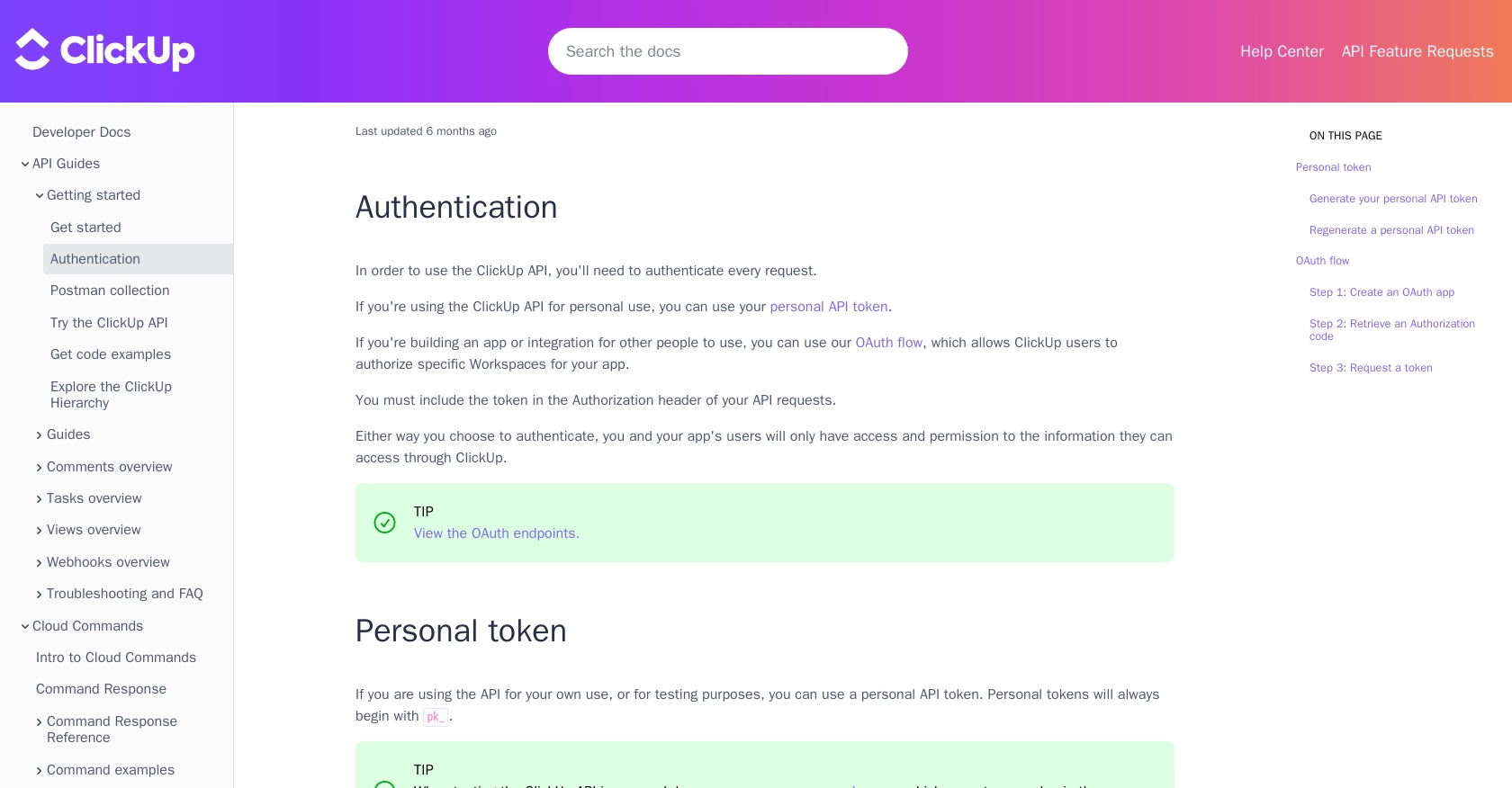
sbb-itb-96038d7
Making API Calls to Create Teams in ClickUp Using PHP
With your ClickUp account and authentication set up, you can now proceed to make API calls to create teams within your workspace. This section will guide you through the process of using PHP to interact with the ClickUp API, focusing on creating user groups (teams).
PHP Prerequisites and Setup
Before you begin, ensure you have the following installed on your machine:
- PHP 7.4 or higher
- cURL extension for PHP
These tools will allow you to make HTTP requests and handle API responses effectively.
Creating a Team in ClickUp Using PHP
To create a team in ClickUp, you'll need to make a POST request to the ClickUp API endpoint. Here's a step-by-step guide with example code:
<?php
// Set the API endpoint and authorization token
$endpoint = "https://api.clickup.com/api/v2/team/{team_id}/group";
$accessToken = "Your_Access_Token";
// Define the team data
$data = [
"name" => "New Team Name",
"members" => [123456, 987654] // Replace with actual member IDs
];
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Authorization: Bearer $accessToken",
"Content-Type: application/json"
]);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute cURL request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Parse and display the response
$responseData = json_decode($response, true);
echo "Team Created Successfully: " . $responseData['name'];
}
// Close cURL session
curl_close($ch);
?>
Replace Your_Access_Token
with the access token obtained through the OAuth process, and {team_id}
with the ID of your workspace.
Verifying the API Request Success
After running the PHP script, you should see a confirmation message indicating that the team was created successfully. To verify, log into your ClickUp account and navigate to the Teams section to see the newly created team.
Handling Errors and Common Error Codes
While making API calls, you may encounter errors. Here are some common error codes and their meanings:
- 400 Bad Request: The request was invalid. Check your data and parameters.
- 401 Unauthorized: The access token is missing or invalid. Ensure your token is correct.
- 403 Forbidden: You do not have permission to perform this action. Check your API permissions.
- 404 Not Found: The specified endpoint or resource could not be found.
For more detailed error handling, refer to the ClickUp API documentation.
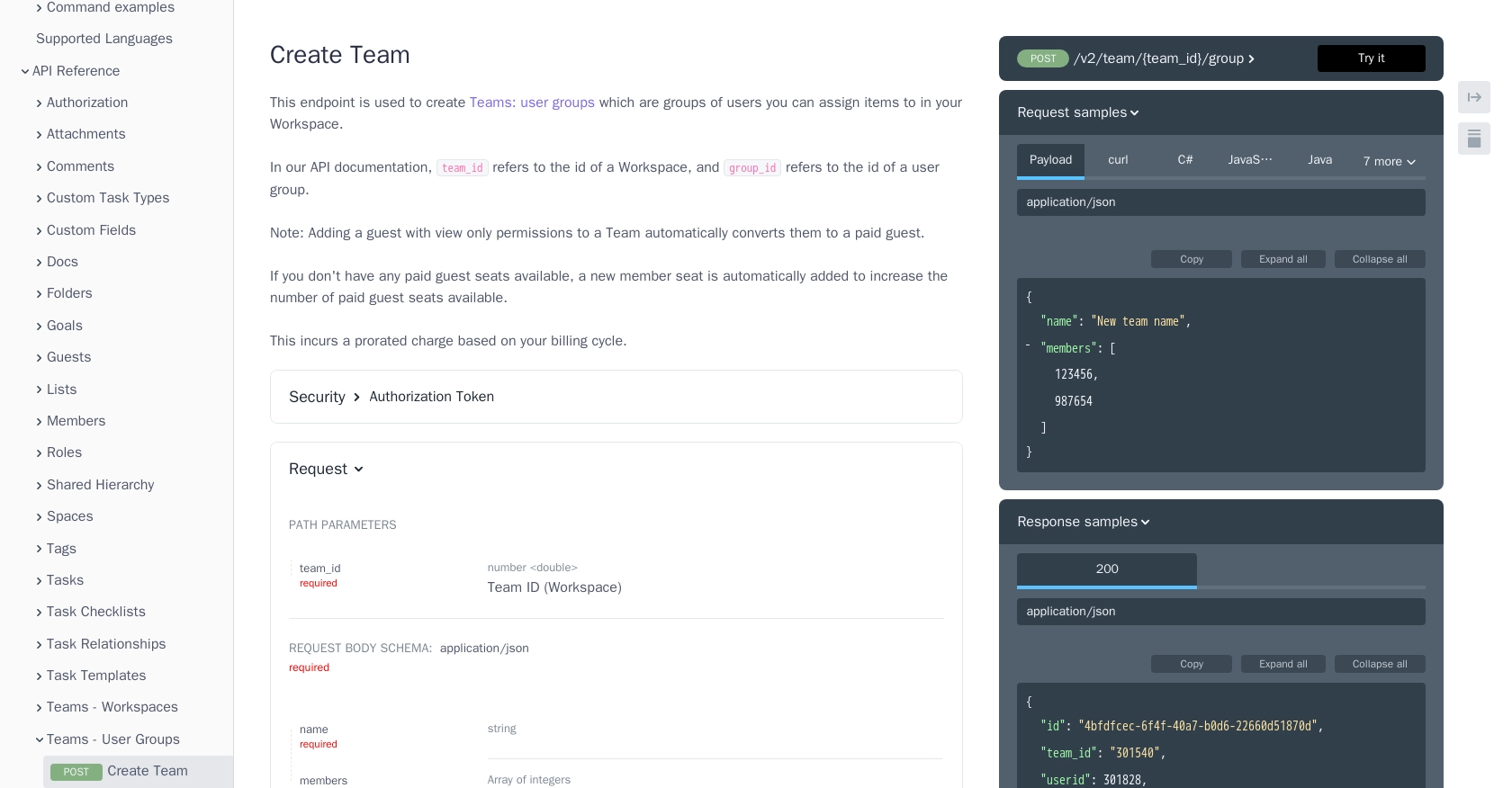
Conclusion and Best Practices for ClickUp API Integration
Integrating with the ClickUp API to create teams can significantly enhance your project management capabilities by automating the organization of user groups within your workspace. By following the steps outlined in this article, you can efficiently set up authentication, make API calls, and handle responses using PHP.
Best Practices for Secure and Efficient API Usage
- Secure Storage of Credentials: Always store your API tokens and client secrets securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be aware of ClickUp's rate limits to avoid exceeding them. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data fields are standardized and consistent across your application to facilitate smooth integration and data handling.
Enhance Your Integration Strategy with Endgrate
While building integrations with ClickUp and other platforms, consider leveraging Endgrate to streamline your process. Endgrate allows you to build once for each use case, reducing the need for multiple integrations and enabling you to focus on your core product. With Endgrate, you can offer an intuitive integration experience for your customers, saving time and resources.
Explore how Endgrate can simplify your integration needs by visiting Endgrate and discover the benefits of a unified API solution.
Read More
Ready to get started?